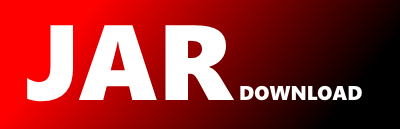
target.apidocs.com.google.api.services.cloudkms.v1.CloudKMS.Projects.Locations.KeyRings.CryptoKeys.html Maven / Gradle / Ivy
CloudKMS.Projects.Locations.KeyRings.CryptoKeys (Cloud Key Management Service (KMS) API v1-rev20240801-2.0.0)
com.google.api.services.cloudkms.v1
Class CloudKMS.Projects.Locations.KeyRings.CryptoKeys
- java.lang.Object
-
- com.google.api.services.cloudkms.v1.CloudKMS.Projects.Locations.KeyRings.CryptoKeys
-
- Enclosing class:
- CloudKMS.Projects.Locations.KeyRings
public class CloudKMS.Projects.Locations.KeyRings.CryptoKeys
extends Object
The "cryptoKeys" collection of methods.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Create
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.CryptoKeyVersions
The "cryptoKeyVersions" collection of methods.
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Decrypt
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Encrypt
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Get
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.GetIamPolicy
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.List
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Patch
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.SetIamPolicy
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.TestIamPermissions
class
CloudKMS.Projects.Locations.KeyRings.CryptoKeys.UpdatePrimaryVersion
-
Constructor Summary
Constructors
Constructor and Description
CryptoKeys()
-
Method Summary
-
-
Method Detail
-
create
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Create create(String parent,
CryptoKey content)
throws IOException
Create a new CryptoKey within a KeyRing. CryptoKey.purpose and
CryptoKey.version_template.algorithm are required.
Create a request for the method "cryptoKeys.create".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
parent
- Required. The name of the KeyRing associated with the CryptoKeys.
content
- the CryptoKey
- Returns:
- the request
- Throws:
IOException
-
decrypt
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Decrypt decrypt(String name,
DecryptRequest content)
throws IOException
Decrypts data that was protected by Encrypt. The CryptoKey.purpose must be ENCRYPT_DECRYPT.
Create a request for the method "cryptoKeys.decrypt".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Required. The resource name of the CryptoKey to use for decryption. The server will choose the
appropriate version.
content
- the DecryptRequest
- Returns:
- the request
- Throws:
IOException
-
encrypt
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Encrypt encrypt(String name,
EncryptRequest content)
throws IOException
Encrypts data, so that it can only be recovered by a call to Decrypt. The CryptoKey.purpose must
be ENCRYPT_DECRYPT.
Create a request for the method "cryptoKeys.encrypt".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Required. The resource name of the CryptoKey or CryptoKeyVersion to use for encryption. If a
CryptoKey is specified, the server will use its primary version.
content
- the EncryptRequest
- Returns:
- the request
- Throws:
IOException
-
get
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Get get(String name)
throws IOException
Returns metadata for a given CryptoKey, as well as its primary CryptoKeyVersion.
Create a request for the method "cryptoKeys.get".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Required. The name of the CryptoKey to get.
- Returns:
- the request
- Throws:
IOException
-
getIamPolicy
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.GetIamPolicy getIamPolicy(String resource)
throws IOException
Gets the access control policy for a resource. Returns an empty policy if the resource exists and
does not have a policy set.
Create a request for the method "cryptoKeys.getIamPolicy".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
resource
- REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
this field.
- Returns:
- the request
- Throws:
IOException
-
list
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.List list(String parent)
throws IOException
Lists CryptoKeys.
Create a request for the method "cryptoKeys.list".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
parent
- Required. The resource name of the KeyRing to list, in the format `projects/locations/keyRings`.
- Returns:
- the request
- Throws:
IOException
-
patch
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.Patch patch(String name,
CryptoKey content)
throws IOException
Update a CryptoKey.
Create a request for the method "cryptoKeys.patch".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Output only. The resource name for this CryptoKey in the format
`projects/locations/keyRings/cryptoKeys`.
content
- the CryptoKey
- Returns:
- the request
- Throws:
IOException
-
setIamPolicy
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.SetIamPolicy setIamPolicy(String resource,
SetIamPolicyRequest content)
throws IOException
Sets the access control policy on the specified resource. Replaces any existing policy. Can
return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
Create a request for the method "cryptoKeys.setIamPolicy".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
resource
- REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
this field.
content
- the SetIamPolicyRequest
- Returns:
- the request
- Throws:
IOException
-
testIamPermissions
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.TestIamPermissions testIamPermissions(String resource,
TestIamPermissionsRequest content)
throws IOException
Returns permissions that a caller has on the specified resource. If the resource does not exist,
this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
designed to be used for building permission-aware UIs and command-line tools, not for
authorization checking. This operation may "fail open" without warning.
Create a request for the method "cryptoKeys.testIamPermissions".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
resource
- REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
this field.
content
- the TestIamPermissionsRequest
- Returns:
- the request
- Throws:
IOException
-
updatePrimaryVersion
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.UpdatePrimaryVersion updatePrimaryVersion(String name,
UpdateCryptoKeyPrimaryVersionRequest content)
throws IOException
Update the version of a CryptoKey that will be used in Encrypt. Returns an error if called on a
key whose purpose is not ENCRYPT_DECRYPT.
Create a request for the method "cryptoKeys.updatePrimaryVersion".
This request holds the parameters needed by the cloudkms server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote
operation.
- Parameters:
name
- Required. The resource name of the CryptoKey to update.
content
- the UpdateCryptoKeyPrimaryVersionRequest
- Returns:
- the request
- Throws:
IOException
-
cryptoKeyVersions
public CloudKMS.Projects.Locations.KeyRings.CryptoKeys.CryptoKeyVersions cryptoKeyVersions()
An accessor for creating requests from the CryptoKeyVersions collection.
The typical use is:
CloudKMS cloudkms = new CloudKMS(...);
CloudKMS.CryptoKeyVersions.List request = cloudkms.cryptoKeyVersions().list(parameters ...)
- Returns:
- the resource collection
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy