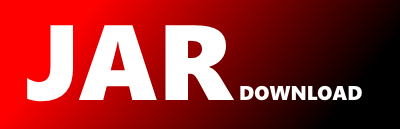
com.google.api.services.cloudresourcemanager.CloudResourceManager Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudresourcemanager;
/**
* Service definition for CloudResourceManager (v1).
*
*
* Creates, reads, and updates metadata for Google Cloud Platform resource containers.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudResourceManagerRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudResourceManager extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.30.9 of the Cloud Resource Manager API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://cloudresourcemanager.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudResourceManager(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudResourceManager(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Folders collection.
*
* The typical use is:
*
* {@code CloudResourceManager cloudresourcemanager = new CloudResourceManager(...);}
* {@code CloudResourceManager.Folders.List request = cloudresourcemanager.folders().list(parameters ...)}
*
*
* @return the resource collection
*/
public Folders folders() {
return new Folders();
}
/**
* The "folders" collection of methods.
*/
public class Folders {
/**
* Clears a `Policy` from a resource.
*
* Create a request for the method "folders.clearOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ClearOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource for the `Policy` to clear.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest}
* @return the request
*/
public ClearOrgPolicy clearOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest content) throws java.io.IOException {
ClearOrgPolicy result = new ClearOrgPolicy(resource, content);
initialize(result);
return result;
}
public class ClearOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:clearOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Clears a `Policy` from a resource.
*
* Create a request for the method "folders.clearOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ClearOrgPolicy#execute()} method to invoke the remote
* operation. {@link ClearOrgPolicy#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param resource Name of the resource for the `Policy` to clear.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest}
* @since 1.13
*/
protected ClearOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Empty.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public ClearOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (ClearOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public ClearOrgPolicy setAlt(java.lang.String alt) {
return (ClearOrgPolicy) super.setAlt(alt);
}
@Override
public ClearOrgPolicy setCallback(java.lang.String callback) {
return (ClearOrgPolicy) super.setCallback(callback);
}
@Override
public ClearOrgPolicy setFields(java.lang.String fields) {
return (ClearOrgPolicy) super.setFields(fields);
}
@Override
public ClearOrgPolicy setKey(java.lang.String key) {
return (ClearOrgPolicy) super.setKey(key);
}
@Override
public ClearOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ClearOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public ClearOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (ClearOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public ClearOrgPolicy setUploadType(java.lang.String uploadType) {
return (ClearOrgPolicy) super.setUploadType(uploadType);
}
@Override
public ClearOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (ClearOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource for the `Policy` to clear. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource for the `Policy` to clear.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource for the `Policy` to clear. */
public ClearOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ClearOrgPolicy set(String parameterName, Object value) {
return (ClearOrgPolicy) super.set(parameterName, value);
}
}
/**
* Gets the effective `Policy` on a resource. This is the result of merging `Policies` in the
* resource hierarchy. The returned `Policy` will not have an `etag`set because it is a computed
* `Policy` across multiple resources. Subtrees of Resource Manager resource hierarchy with 'under:'
* prefix will not be expanded.
*
* Create a request for the method "folders.getEffectiveOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetEffectiveOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource The name of the resource to start computing the effective `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest}
* @return the request
*/
public GetEffectiveOrgPolicy getEffectiveOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest content) throws java.io.IOException {
GetEffectiveOrgPolicy result = new GetEffectiveOrgPolicy(resource, content);
initialize(result);
return result;
}
public class GetEffectiveOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getEffectiveOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Gets the effective `Policy` on a resource. This is the result of merging `Policies` in the
* resource hierarchy. The returned `Policy` will not have an `etag`set because it is a computed
* `Policy` across multiple resources. Subtrees of Resource Manager resource hierarchy with
* 'under:' prefix will not be expanded.
*
* Create a request for the method "folders.getEffectiveOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetEffectiveOrgPolicy#execute()} method to invoke the
* remote operation. {@link GetEffectiveOrgPolicy#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource The name of the resource to start computing the effective `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest}
* @since 1.13
*/
protected GetEffectiveOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public GetEffectiveOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetEffectiveOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetEffectiveOrgPolicy setAlt(java.lang.String alt) {
return (GetEffectiveOrgPolicy) super.setAlt(alt);
}
@Override
public GetEffectiveOrgPolicy setCallback(java.lang.String callback) {
return (GetEffectiveOrgPolicy) super.setCallback(callback);
}
@Override
public GetEffectiveOrgPolicy setFields(java.lang.String fields) {
return (GetEffectiveOrgPolicy) super.setFields(fields);
}
@Override
public GetEffectiveOrgPolicy setKey(java.lang.String key) {
return (GetEffectiveOrgPolicy) super.setKey(key);
}
@Override
public GetEffectiveOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetEffectiveOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetEffectiveOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetEffectiveOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetEffectiveOrgPolicy setUploadType(java.lang.String uploadType) {
return (GetEffectiveOrgPolicy) super.setUploadType(uploadType);
}
@Override
public GetEffectiveOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetEffectiveOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** The name of the resource to start computing the effective `Policy`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** The name of the resource to start computing the effective `Policy`.
*/
public java.lang.String getResource() {
return resource;
}
/** The name of the resource to start computing the effective `Policy`. */
public GetEffectiveOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetEffectiveOrgPolicy set(String parameterName, Object value) {
return (GetEffectiveOrgPolicy) super.set(parameterName, value);
}
}
/**
* Gets a `Policy` on a resource.
*
* If no `Policy` is set on the resource, a `Policy` is returned with default values including
* `POLICY_TYPE_NOT_SET` for the `policy_type oneof`. The `etag` value can be used with
* `SetOrgPolicy()` to create or update a `Policy` during read-modify-write.
*
* Create a request for the method "folders.getOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource the `Policy` is set on.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest}
* @return the request
*/
public GetOrgPolicy getOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest content) throws java.io.IOException {
GetOrgPolicy result = new GetOrgPolicy(resource, content);
initialize(result);
return result;
}
public class GetOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Gets a `Policy` on a resource.
*
* If no `Policy` is set on the resource, a `Policy` is returned with default values including
* `POLICY_TYPE_NOT_SET` for the `policy_type oneof`. The `etag` value can be used with
* `SetOrgPolicy()` to create or update a `Policy` during read-modify-write.
*
* Create a request for the method "folders.getOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetOrgPolicy#execute()} method to invoke the remote
* operation. {@link
* GetOrgPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource Name of the resource the `Policy` is set on.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest}
* @since 1.13
*/
protected GetOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public GetOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetOrgPolicy setAlt(java.lang.String alt) {
return (GetOrgPolicy) super.setAlt(alt);
}
@Override
public GetOrgPolicy setCallback(java.lang.String callback) {
return (GetOrgPolicy) super.setCallback(callback);
}
@Override
public GetOrgPolicy setFields(java.lang.String fields) {
return (GetOrgPolicy) super.setFields(fields);
}
@Override
public GetOrgPolicy setKey(java.lang.String key) {
return (GetOrgPolicy) super.setKey(key);
}
@Override
public GetOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetOrgPolicy setUploadType(java.lang.String uploadType) {
return (GetOrgPolicy) super.setUploadType(uploadType);
}
@Override
public GetOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource the `Policy` is set on. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource the `Policy` is set on.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource the `Policy` is set on. */
public GetOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetOrgPolicy set(String parameterName, Object value) {
return (GetOrgPolicy) super.set(parameterName, value);
}
}
/**
* Lists `Constraints` that could be applied on the specified resource.
*
* Create a request for the method "folders.listAvailableOrgPolicyConstraints".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ListAvailableOrgPolicyConstraints#execute()} method to
* invoke the remote operation.
*
* @param resource Name of the resource to list `Constraints` for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest}
* @return the request
*/
public ListAvailableOrgPolicyConstraints listAvailableOrgPolicyConstraints(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest content) throws java.io.IOException {
ListAvailableOrgPolicyConstraints result = new ListAvailableOrgPolicyConstraints(resource, content);
initialize(result);
return result;
}
public class ListAvailableOrgPolicyConstraints extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:listAvailableOrgPolicyConstraints";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Lists `Constraints` that could be applied on the specified resource.
*
* Create a request for the method "folders.listAvailableOrgPolicyConstraints".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ListAvailableOrgPolicyConstraints#execute()} method to
* invoke the remote operation. {@link ListAvailableOrgPolicyConstraints#initialize(com.google
* .api.client.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this
* instance immediately after invoking the constructor.
*
* @param resource Name of the resource to list `Constraints` for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest}
* @since 1.13
*/
protected ListAvailableOrgPolicyConstraints(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public ListAvailableOrgPolicyConstraints set$Xgafv(java.lang.String $Xgafv) {
return (ListAvailableOrgPolicyConstraints) super.set$Xgafv($Xgafv);
}
@Override
public ListAvailableOrgPolicyConstraints setAlt(java.lang.String alt) {
return (ListAvailableOrgPolicyConstraints) super.setAlt(alt);
}
@Override
public ListAvailableOrgPolicyConstraints setCallback(java.lang.String callback) {
return (ListAvailableOrgPolicyConstraints) super.setCallback(callback);
}
@Override
public ListAvailableOrgPolicyConstraints setFields(java.lang.String fields) {
return (ListAvailableOrgPolicyConstraints) super.setFields(fields);
}
@Override
public ListAvailableOrgPolicyConstraints setKey(java.lang.String key) {
return (ListAvailableOrgPolicyConstraints) super.setKey(key);
}
@Override
public ListAvailableOrgPolicyConstraints setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListAvailableOrgPolicyConstraints) super.setPrettyPrint(prettyPrint);
}
@Override
public ListAvailableOrgPolicyConstraints setQuotaUser(java.lang.String quotaUser) {
return (ListAvailableOrgPolicyConstraints) super.setQuotaUser(quotaUser);
}
@Override
public ListAvailableOrgPolicyConstraints setUploadType(java.lang.String uploadType) {
return (ListAvailableOrgPolicyConstraints) super.setUploadType(uploadType);
}
@Override
public ListAvailableOrgPolicyConstraints setUploadProtocol(java.lang.String uploadProtocol) {
return (ListAvailableOrgPolicyConstraints) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource to list `Constraints` for. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource to list `Constraints` for.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource to list `Constraints` for. */
public ListAvailableOrgPolicyConstraints setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ListAvailableOrgPolicyConstraints set(String parameterName, Object value) {
return (ListAvailableOrgPolicyConstraints) super.set(parameterName, value);
}
}
/**
* Lists all the `Policies` set for a particular resource.
*
* Create a request for the method "folders.listOrgPolicies".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ListOrgPolicies#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource to list Policies for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest}
* @return the request
*/
public ListOrgPolicies listOrgPolicies(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest content) throws java.io.IOException {
ListOrgPolicies result = new ListOrgPolicies(resource, content);
initialize(result);
return result;
}
public class ListOrgPolicies extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:listOrgPolicies";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Lists all the `Policies` set for a particular resource.
*
* Create a request for the method "folders.listOrgPolicies".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ListOrgPolicies#execute()} method to invoke the remote
* operation. {@link ListOrgPolicies#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param resource Name of the resource to list Policies for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest}
* @since 1.13
*/
protected ListOrgPolicies(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public ListOrgPolicies set$Xgafv(java.lang.String $Xgafv) {
return (ListOrgPolicies) super.set$Xgafv($Xgafv);
}
@Override
public ListOrgPolicies setAlt(java.lang.String alt) {
return (ListOrgPolicies) super.setAlt(alt);
}
@Override
public ListOrgPolicies setCallback(java.lang.String callback) {
return (ListOrgPolicies) super.setCallback(callback);
}
@Override
public ListOrgPolicies setFields(java.lang.String fields) {
return (ListOrgPolicies) super.setFields(fields);
}
@Override
public ListOrgPolicies setKey(java.lang.String key) {
return (ListOrgPolicies) super.setKey(key);
}
@Override
public ListOrgPolicies setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListOrgPolicies) super.setPrettyPrint(prettyPrint);
}
@Override
public ListOrgPolicies setQuotaUser(java.lang.String quotaUser) {
return (ListOrgPolicies) super.setQuotaUser(quotaUser);
}
@Override
public ListOrgPolicies setUploadType(java.lang.String uploadType) {
return (ListOrgPolicies) super.setUploadType(uploadType);
}
@Override
public ListOrgPolicies setUploadProtocol(java.lang.String uploadProtocol) {
return (ListOrgPolicies) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource to list Policies for. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource to list Policies for.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource to list Policies for. */
public ListOrgPolicies setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ListOrgPolicies set(String parameterName, Object value) {
return (ListOrgPolicies) super.set(parameterName, value);
}
}
/**
* Updates the specified `Policy` on the resource. Creates a new `Policy` for that `Constraint` on
* the resource if one does not exist.
*
* Not supplying an `etag` on the request `Policy` results in an unconditional write of the
* `Policy`.
*
* Create a request for the method "folders.setOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link SetOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Resource name of the resource to attach the `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest}
* @return the request
*/
public SetOrgPolicy setOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest content) throws java.io.IOException {
SetOrgPolicy result = new SetOrgPolicy(resource, content);
initialize(result);
return result;
}
public class SetOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:setOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Updates the specified `Policy` on the resource. Creates a new `Policy` for that `Constraint` on
* the resource if one does not exist.
*
* Not supplying an `etag` on the request `Policy` results in an unconditional write of the
* `Policy`.
*
* Create a request for the method "folders.setOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link SetOrgPolicy#execute()} method to invoke the remote
* operation. {@link
* SetOrgPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource Resource name of the resource to attach the `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest}
* @since 1.13
*/
protected SetOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public SetOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetOrgPolicy setAlt(java.lang.String alt) {
return (SetOrgPolicy) super.setAlt(alt);
}
@Override
public SetOrgPolicy setCallback(java.lang.String callback) {
return (SetOrgPolicy) super.setCallback(callback);
}
@Override
public SetOrgPolicy setFields(java.lang.String fields) {
return (SetOrgPolicy) super.setFields(fields);
}
@Override
public SetOrgPolicy setKey(java.lang.String key) {
return (SetOrgPolicy) super.setKey(key);
}
@Override
public SetOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetOrgPolicy setUploadType(java.lang.String uploadType) {
return (SetOrgPolicy) super.setUploadType(uploadType);
}
@Override
public SetOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Resource name of the resource to attach the `Policy`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Resource name of the resource to attach the `Policy`.
*/
public java.lang.String getResource() {
return resource;
}
/** Resource name of the resource to attach the `Policy`. */
public SetOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetOrgPolicy set(String parameterName, Object value) {
return (SetOrgPolicy) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Liens collection.
*
* The typical use is:
*
* {@code CloudResourceManager cloudresourcemanager = new CloudResourceManager(...);}
* {@code CloudResourceManager.Liens.List request = cloudresourcemanager.liens().list(parameters ...)}
*
*
* @return the resource collection
*/
public Liens liens() {
return new Liens();
}
/**
* The "liens" collection of methods.
*/
public class Liens {
/**
* Create a Lien which applies to the resource denoted by the `parent` field.
*
* Callers of this method will require permission on the `parent` resource. For example, applying to
* `projects/1234` requires permission `resourcemanager.projects.updateLiens`.
*
* NOTE: Some resources may limit the number of Liens which may be applied.
*
* Create a request for the method "liens.create".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudresourcemanager.model.Lien}
* @return the request
*/
public Create create(com.google.api.services.cloudresourcemanager.model.Lien content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/liens";
/**
* Create a Lien which applies to the resource denoted by the `parent` field.
*
* Callers of this method will require permission on the `parent` resource. For example, applying
* to `projects/1234` requires permission `resourcemanager.projects.updateLiens`.
*
* NOTE: Some resources may limit the number of Liens which may be applied.
*
* Create a request for the method "liens.create".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudresourcemanager.model.Lien}
* @since 1.13
*/
protected Create(com.google.api.services.cloudresourcemanager.model.Lien content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Lien.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete a Lien by `name`.
*
* Callers of this method will require permission on the `parent` resource. For example, a Lien with
* a `parent` of `projects/1234` requires permission `resourcemanager.projects.updateLiens`.
*
* Create a request for the method "liens.delete".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name/identifier of the Lien to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^liens/.*$");
/**
* Delete a Lien by `name`.
*
* Callers of this method will require permission on the `parent` resource. For example, a Lien
* with a `parent` of `projects/1234` requires permission `resourcemanager.projects.updateLiens`.
*
* Create a request for the method "liens.delete".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name/identifier of the Lien to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudResourceManager.this, "DELETE", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^liens/.*$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name/identifier of the Lien to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name/identifier of the Lien to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name/identifier of the Lien to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^liens/.*$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieve a Lien by `name`.
*
* Callers of this method will require permission on the `parent` resource. For example, a Lien with
* a `parent` of `projects/1234` requires permission requires permission
* `resourcemanager.projects.get` or `resourcemanager.projects.updateLiens`.
*
* Create a request for the method "liens.get".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name/identifier of the Lien.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^liens/.*$");
/**
* Retrieve a Lien by `name`.
*
* Callers of this method will require permission on the `parent` resource. For example, a Lien
* with a `parent` of `projects/1234` requires permission requires permission
* `resourcemanager.projects.get` or `resourcemanager.projects.updateLiens`.
*
* Create a request for the method "liens.get".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name/identifier of the Lien.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudResourceManager.this, "GET", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.Lien.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^liens/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name/identifier of the Lien. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name/identifier of the Lien.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name/identifier of the Lien. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^liens/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all Liens applied to the `parent` resource.
*
* Callers of this method will require permission on the `parent` resource. For example, a Lien with
* a `parent` of `projects/1234` requires permission `resourcemanager.projects.get`.
*
* Create a request for the method "liens.list".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/liens";
/**
* List all Liens applied to the `parent` resource.
*
* Callers of this method will require permission on the `parent` resource. For example, a Lien
* with a `parent` of `projects/1234` requires permission `resourcemanager.projects.get`.
*
* Create a request for the method "liens.list".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudResourceManager.this, "GET", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.ListLiensResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The maximum number of items to return. This is a suggestion for the server. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return. This is a suggestion for the server.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of items to return. This is a suggestion for the server. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The `next_page_token` value returned from a previous List request, if any. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The `next_page_token` value returned from a previous List request, if any. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. The name of the resource to list all attached Liens. For example,
* `projects/1234`.
*
* (google.api.field_policy).resource_type annotation is not set since the parent depends on
* the meta api implementation. This field could be a project or other sub project resources.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the resource to list all attached Liens. For example, `projects/1234`.
(google.api.field_policy).resource_type annotation is not set since the parent depends on the meta
api implementation. This field could be a project or other sub project resources.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the resource to list all attached Liens. For example,
* `projects/1234`.
*
* (google.api.field_policy).resource_type annotation is not set since the parent depends on
* the meta api implementation. This field could be a project or other sub project resources.
*/
public List setParent(java.lang.String parent) {
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudResourceManager cloudresourcemanager = new CloudResourceManager(...);}
* {@code CloudResourceManager.Operations.List request = cloudresourcemanager.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudResourceManager.this, "GET", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Organizations collection.
*
* The typical use is:
*
* {@code CloudResourceManager cloudresourcemanager = new CloudResourceManager(...);}
* {@code CloudResourceManager.Organizations.List request = cloudresourcemanager.organizations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Organizations organizations() {
return new Organizations();
}
/**
* The "organizations" collection of methods.
*/
public class Organizations {
/**
* Clears a `Policy` from a resource.
*
* Create a request for the method "organizations.clearOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ClearOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource for the `Policy` to clear.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest}
* @return the request
*/
public ClearOrgPolicy clearOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest content) throws java.io.IOException {
ClearOrgPolicy result = new ClearOrgPolicy(resource, content);
initialize(result);
return result;
}
public class ClearOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:clearOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Clears a `Policy` from a resource.
*
* Create a request for the method "organizations.clearOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ClearOrgPolicy#execute()} method to invoke the remote
* operation. {@link ClearOrgPolicy#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param resource Name of the resource for the `Policy` to clear.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest}
* @since 1.13
*/
protected ClearOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Empty.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public ClearOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (ClearOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public ClearOrgPolicy setAlt(java.lang.String alt) {
return (ClearOrgPolicy) super.setAlt(alt);
}
@Override
public ClearOrgPolicy setCallback(java.lang.String callback) {
return (ClearOrgPolicy) super.setCallback(callback);
}
@Override
public ClearOrgPolicy setFields(java.lang.String fields) {
return (ClearOrgPolicy) super.setFields(fields);
}
@Override
public ClearOrgPolicy setKey(java.lang.String key) {
return (ClearOrgPolicy) super.setKey(key);
}
@Override
public ClearOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ClearOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public ClearOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (ClearOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public ClearOrgPolicy setUploadType(java.lang.String uploadType) {
return (ClearOrgPolicy) super.setUploadType(uploadType);
}
@Override
public ClearOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (ClearOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource for the `Policy` to clear. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource for the `Policy` to clear.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource for the `Policy` to clear. */
public ClearOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ClearOrgPolicy set(String parameterName, Object value) {
return (ClearOrgPolicy) super.set(parameterName, value);
}
}
/**
* Fetches an Organization resource identified by the specified resource name.
*
* Create a request for the method "organizations.get".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The resource name of the Organization to fetch. This is the organization's
relative path in the API,
* formatted as "organizations/[organizationId]".
For example, "organizations/1234".
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Fetches an Organization resource identified by the specified resource name.
*
* Create a request for the method "organizations.get".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource name of the Organization to fetch. This is the organization's
relative path in the API,
* formatted as "organizations/[organizationId]".
For example, "organizations/1234".
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudResourceManager.this, "GET", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.Organization.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the Organization to fetch. This is the organization's relative path in
* the API, formatted as "organizations/[organizationId]". For example, "organizations/1234".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the Organization to fetch. This is the organization's relative path in the
API, formatted as "organizations/[organizationId]". For example, "organizations/1234".
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the Organization to fetch. This is the organization's relative path in
* the API, formatted as "organizations/[organizationId]". For example, "organizations/1234".
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the effective `Policy` on a resource. This is the result of merging `Policies` in the
* resource hierarchy. The returned `Policy` will not have an `etag`set because it is a computed
* `Policy` across multiple resources. Subtrees of Resource Manager resource hierarchy with 'under:'
* prefix will not be expanded.
*
* Create a request for the method "organizations.getEffectiveOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetEffectiveOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource The name of the resource to start computing the effective `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest}
* @return the request
*/
public GetEffectiveOrgPolicy getEffectiveOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest content) throws java.io.IOException {
GetEffectiveOrgPolicy result = new GetEffectiveOrgPolicy(resource, content);
initialize(result);
return result;
}
public class GetEffectiveOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getEffectiveOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Gets the effective `Policy` on a resource. This is the result of merging `Policies` in the
* resource hierarchy. The returned `Policy` will not have an `etag`set because it is a computed
* `Policy` across multiple resources. Subtrees of Resource Manager resource hierarchy with
* 'under:' prefix will not be expanded.
*
* Create a request for the method "organizations.getEffectiveOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetEffectiveOrgPolicy#execute()} method to invoke the
* remote operation. {@link GetEffectiveOrgPolicy#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource The name of the resource to start computing the effective `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest}
* @since 1.13
*/
protected GetEffectiveOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public GetEffectiveOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetEffectiveOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetEffectiveOrgPolicy setAlt(java.lang.String alt) {
return (GetEffectiveOrgPolicy) super.setAlt(alt);
}
@Override
public GetEffectiveOrgPolicy setCallback(java.lang.String callback) {
return (GetEffectiveOrgPolicy) super.setCallback(callback);
}
@Override
public GetEffectiveOrgPolicy setFields(java.lang.String fields) {
return (GetEffectiveOrgPolicy) super.setFields(fields);
}
@Override
public GetEffectiveOrgPolicy setKey(java.lang.String key) {
return (GetEffectiveOrgPolicy) super.setKey(key);
}
@Override
public GetEffectiveOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetEffectiveOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetEffectiveOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetEffectiveOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetEffectiveOrgPolicy setUploadType(java.lang.String uploadType) {
return (GetEffectiveOrgPolicy) super.setUploadType(uploadType);
}
@Override
public GetEffectiveOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetEffectiveOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** The name of the resource to start computing the effective `Policy`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** The name of the resource to start computing the effective `Policy`.
*/
public java.lang.String getResource() {
return resource;
}
/** The name of the resource to start computing the effective `Policy`. */
public GetEffectiveOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetEffectiveOrgPolicy set(String parameterName, Object value) {
return (GetEffectiveOrgPolicy) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for an Organization resource. May be empty if no such policy or
* resource exists. The `resource` field should be the organization's resource name, e.g.
* "organizations/123".
*
* Authorization requires the Google IAM permission `resourcemanager.organizations.getIamPolicy` on
* the specified organization
*
* Create a request for the method "organizations.getIamPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Gets the access control policy for an Organization resource. May be empty if no such policy or
* resource exists. The `resource` field should be the organization's resource name, e.g.
* "organizations/123".
*
* Authorization requires the Google IAM permission `resourcemanager.organizations.getIamPolicy`
* on the specified organization
*
* Create a request for the method "organizations.getIamPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Gets a `Policy` on a resource.
*
* If no `Policy` is set on the resource, a `Policy` is returned with default values including
* `POLICY_TYPE_NOT_SET` for the `policy_type oneof`. The `etag` value can be used with
* `SetOrgPolicy()` to create or update a `Policy` during read-modify-write.
*
* Create a request for the method "organizations.getOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource the `Policy` is set on.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest}
* @return the request
*/
public GetOrgPolicy getOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest content) throws java.io.IOException {
GetOrgPolicy result = new GetOrgPolicy(resource, content);
initialize(result);
return result;
}
public class GetOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Gets a `Policy` on a resource.
*
* If no `Policy` is set on the resource, a `Policy` is returned with default values including
* `POLICY_TYPE_NOT_SET` for the `policy_type oneof`. The `etag` value can be used with
* `SetOrgPolicy()` to create or update a `Policy` during read-modify-write.
*
* Create a request for the method "organizations.getOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetOrgPolicy#execute()} method to invoke the remote
* operation. {@link
* GetOrgPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource Name of the resource the `Policy` is set on.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest}
* @since 1.13
*/
protected GetOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public GetOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetOrgPolicy setAlt(java.lang.String alt) {
return (GetOrgPolicy) super.setAlt(alt);
}
@Override
public GetOrgPolicy setCallback(java.lang.String callback) {
return (GetOrgPolicy) super.setCallback(callback);
}
@Override
public GetOrgPolicy setFields(java.lang.String fields) {
return (GetOrgPolicy) super.setFields(fields);
}
@Override
public GetOrgPolicy setKey(java.lang.String key) {
return (GetOrgPolicy) super.setKey(key);
}
@Override
public GetOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetOrgPolicy setUploadType(java.lang.String uploadType) {
return (GetOrgPolicy) super.setUploadType(uploadType);
}
@Override
public GetOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource the `Policy` is set on. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource the `Policy` is set on.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource the `Policy` is set on. */
public GetOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetOrgPolicy set(String parameterName, Object value) {
return (GetOrgPolicy) super.set(parameterName, value);
}
}
/**
* Lists `Constraints` that could be applied on the specified resource.
*
* Create a request for the method "organizations.listAvailableOrgPolicyConstraints".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ListAvailableOrgPolicyConstraints#execute()} method to
* invoke the remote operation.
*
* @param resource Name of the resource to list `Constraints` for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest}
* @return the request
*/
public ListAvailableOrgPolicyConstraints listAvailableOrgPolicyConstraints(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest content) throws java.io.IOException {
ListAvailableOrgPolicyConstraints result = new ListAvailableOrgPolicyConstraints(resource, content);
initialize(result);
return result;
}
public class ListAvailableOrgPolicyConstraints extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:listAvailableOrgPolicyConstraints";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists `Constraints` that could be applied on the specified resource.
*
* Create a request for the method "organizations.listAvailableOrgPolicyConstraints".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ListAvailableOrgPolicyConstraints#execute()} method to
* invoke the remote operation. {@link ListAvailableOrgPolicyConstraints#initialize(com.google
* .api.client.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this
* instance immediately after invoking the constructor.
*
* @param resource Name of the resource to list `Constraints` for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest}
* @since 1.13
*/
protected ListAvailableOrgPolicyConstraints(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public ListAvailableOrgPolicyConstraints set$Xgafv(java.lang.String $Xgafv) {
return (ListAvailableOrgPolicyConstraints) super.set$Xgafv($Xgafv);
}
@Override
public ListAvailableOrgPolicyConstraints setAlt(java.lang.String alt) {
return (ListAvailableOrgPolicyConstraints) super.setAlt(alt);
}
@Override
public ListAvailableOrgPolicyConstraints setCallback(java.lang.String callback) {
return (ListAvailableOrgPolicyConstraints) super.setCallback(callback);
}
@Override
public ListAvailableOrgPolicyConstraints setFields(java.lang.String fields) {
return (ListAvailableOrgPolicyConstraints) super.setFields(fields);
}
@Override
public ListAvailableOrgPolicyConstraints setKey(java.lang.String key) {
return (ListAvailableOrgPolicyConstraints) super.setKey(key);
}
@Override
public ListAvailableOrgPolicyConstraints setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListAvailableOrgPolicyConstraints) super.setPrettyPrint(prettyPrint);
}
@Override
public ListAvailableOrgPolicyConstraints setQuotaUser(java.lang.String quotaUser) {
return (ListAvailableOrgPolicyConstraints) super.setQuotaUser(quotaUser);
}
@Override
public ListAvailableOrgPolicyConstraints setUploadType(java.lang.String uploadType) {
return (ListAvailableOrgPolicyConstraints) super.setUploadType(uploadType);
}
@Override
public ListAvailableOrgPolicyConstraints setUploadProtocol(java.lang.String uploadProtocol) {
return (ListAvailableOrgPolicyConstraints) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource to list `Constraints` for. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource to list `Constraints` for.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource to list `Constraints` for. */
public ListAvailableOrgPolicyConstraints setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ListAvailableOrgPolicyConstraints set(String parameterName, Object value) {
return (ListAvailableOrgPolicyConstraints) super.set(parameterName, value);
}
}
/**
* Lists all the `Policies` set for a particular resource.
*
* Create a request for the method "organizations.listOrgPolicies".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ListOrgPolicies#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource to list Policies for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest}
* @return the request
*/
public ListOrgPolicies listOrgPolicies(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest content) throws java.io.IOException {
ListOrgPolicies result = new ListOrgPolicies(resource, content);
initialize(result);
return result;
}
public class ListOrgPolicies extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:listOrgPolicies";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists all the `Policies` set for a particular resource.
*
* Create a request for the method "organizations.listOrgPolicies".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ListOrgPolicies#execute()} method to invoke the remote
* operation. {@link ListOrgPolicies#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param resource Name of the resource to list Policies for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest}
* @since 1.13
*/
protected ListOrgPolicies(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public ListOrgPolicies set$Xgafv(java.lang.String $Xgafv) {
return (ListOrgPolicies) super.set$Xgafv($Xgafv);
}
@Override
public ListOrgPolicies setAlt(java.lang.String alt) {
return (ListOrgPolicies) super.setAlt(alt);
}
@Override
public ListOrgPolicies setCallback(java.lang.String callback) {
return (ListOrgPolicies) super.setCallback(callback);
}
@Override
public ListOrgPolicies setFields(java.lang.String fields) {
return (ListOrgPolicies) super.setFields(fields);
}
@Override
public ListOrgPolicies setKey(java.lang.String key) {
return (ListOrgPolicies) super.setKey(key);
}
@Override
public ListOrgPolicies setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListOrgPolicies) super.setPrettyPrint(prettyPrint);
}
@Override
public ListOrgPolicies setQuotaUser(java.lang.String quotaUser) {
return (ListOrgPolicies) super.setQuotaUser(quotaUser);
}
@Override
public ListOrgPolicies setUploadType(java.lang.String uploadType) {
return (ListOrgPolicies) super.setUploadType(uploadType);
}
@Override
public ListOrgPolicies setUploadProtocol(java.lang.String uploadProtocol) {
return (ListOrgPolicies) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource to list Policies for. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource to list Policies for.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource to list Policies for. */
public ListOrgPolicies setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ListOrgPolicies set(String parameterName, Object value) {
return (ListOrgPolicies) super.set(parameterName, value);
}
}
/**
* Searches Organization resources that are visible to the user and satisfy the specified filter.
* This method returns Organizations in an unspecified order. New Organizations do not necessarily
* appear at the end of the results.
*
* Search will only return organizations on which the user has the permission
* `resourcemanager.organizations.get`
*
* Create a request for the method "organizations.search".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SearchOrganizationsRequest}
* @return the request
*/
public Search search(com.google.api.services.cloudresourcemanager.model.SearchOrganizationsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/organizations:search";
/**
* Searches Organization resources that are visible to the user and satisfy the specified filter.
* This method returns Organizations in an unspecified order. New Organizations do not necessarily
* appear at the end of the results.
*
* Search will only return organizations on which the user has the permission
* `resourcemanager.organizations.get`
*
* Create a request for the method "organizations.search".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Search#execute()} method to invoke the remote
* operation. {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SearchOrganizationsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.cloudresourcemanager.model.SearchOrganizationsRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.SearchOrganizationsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on an Organization resource. Replaces any existing policy. The
* `resource` field should be the organization's resource name, e.g. "organizations/123".
*
* Authorization requires the Google IAM permission `resourcemanager.organizations.setIamPolicy` on
* the specified organization
*
* Create a request for the method "organizations.setIamPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Sets the access control policy on an Organization resource. Replaces any existing policy. The
* `resource` field should be the organization's resource name, e.g. "organizations/123".
*
* Authorization requires the Google IAM permission `resourcemanager.organizations.setIamPolicy`
* on the specified organization
*
* Create a request for the method "organizations.setIamPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Updates the specified `Policy` on the resource. Creates a new `Policy` for that `Constraint` on
* the resource if one does not exist.
*
* Not supplying an `etag` on the request `Policy` results in an unconditional write of the
* `Policy`.
*
* Create a request for the method "organizations.setOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link SetOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Resource name of the resource to attach the `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest}
* @return the request
*/
public SetOrgPolicy setOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest content) throws java.io.IOException {
SetOrgPolicy result = new SetOrgPolicy(resource, content);
initialize(result);
return result;
}
public class SetOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:setOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Updates the specified `Policy` on the resource. Creates a new `Policy` for that `Constraint` on
* the resource if one does not exist.
*
* Not supplying an `etag` on the request `Policy` results in an unconditional write of the
* `Policy`.
*
* Create a request for the method "organizations.setOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link SetOrgPolicy#execute()} method to invoke the remote
* operation. {@link
* SetOrgPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource Resource name of the resource to attach the `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest}
* @since 1.13
*/
protected SetOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public SetOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetOrgPolicy setAlt(java.lang.String alt) {
return (SetOrgPolicy) super.setAlt(alt);
}
@Override
public SetOrgPolicy setCallback(java.lang.String callback) {
return (SetOrgPolicy) super.setCallback(callback);
}
@Override
public SetOrgPolicy setFields(java.lang.String fields) {
return (SetOrgPolicy) super.setFields(fields);
}
@Override
public SetOrgPolicy setKey(java.lang.String key) {
return (SetOrgPolicy) super.setKey(key);
}
@Override
public SetOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetOrgPolicy setUploadType(java.lang.String uploadType) {
return (SetOrgPolicy) super.setUploadType(uploadType);
}
@Override
public SetOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Resource name of the resource to attach the `Policy`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Resource name of the resource to attach the `Policy`.
*/
public java.lang.String getResource() {
return resource;
}
/** Resource name of the resource to attach the `Policy`. */
public SetOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetOrgPolicy set(String parameterName, Object value) {
return (SetOrgPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified Organization. The `resource` field should
* be the organization's resource name, e.g. "organizations/123".
*
* There are no permissions required for making this API call.
*
* Create a request for the method "organizations.testIamPermissions".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested.
See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Returns permissions that a caller has on the specified Organization. The `resource` field
* should be the organization's resource name, e.g. "organizations/123".
*
* There are no permissions required for making this API call.
*
* Create a request for the method "organizations.testIamPermissions".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested.
See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code CloudResourceManager cloudresourcemanager = new CloudResourceManager(...);}
* {@code CloudResourceManager.Projects.List request = cloudresourcemanager.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* Clears a `Policy` from a resource.
*
* Create a request for the method "projects.clearOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ClearOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource for the `Policy` to clear.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest}
* @return the request
*/
public ClearOrgPolicy clearOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest content) throws java.io.IOException {
ClearOrgPolicy result = new ClearOrgPolicy(resource, content);
initialize(result);
return result;
}
public class ClearOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:clearOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Clears a `Policy` from a resource.
*
* Create a request for the method "projects.clearOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ClearOrgPolicy#execute()} method to invoke the remote
* operation. {@link ClearOrgPolicy#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param resource Name of the resource for the `Policy` to clear.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest}
* @since 1.13
*/
protected ClearOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ClearOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Empty.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public ClearOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (ClearOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public ClearOrgPolicy setAlt(java.lang.String alt) {
return (ClearOrgPolicy) super.setAlt(alt);
}
@Override
public ClearOrgPolicy setCallback(java.lang.String callback) {
return (ClearOrgPolicy) super.setCallback(callback);
}
@Override
public ClearOrgPolicy setFields(java.lang.String fields) {
return (ClearOrgPolicy) super.setFields(fields);
}
@Override
public ClearOrgPolicy setKey(java.lang.String key) {
return (ClearOrgPolicy) super.setKey(key);
}
@Override
public ClearOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ClearOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public ClearOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (ClearOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public ClearOrgPolicy setUploadType(java.lang.String uploadType) {
return (ClearOrgPolicy) super.setUploadType(uploadType);
}
@Override
public ClearOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (ClearOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource for the `Policy` to clear. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource for the `Policy` to clear.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource for the `Policy` to clear. */
public ClearOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ClearOrgPolicy set(String parameterName, Object value) {
return (ClearOrgPolicy) super.set(parameterName, value);
}
}
/**
* Request that a new Project be created. The result is an Operation which can be used to track the
* creation process. This process usually takes a few seconds, but can sometimes take much longer.
* The tracking Operation is automatically deleted after a few hours, so there is no need to call
* DeleteOperation.
*
* Authorization requires the Google IAM permission `resourcemanager.projects.create` on the
* specified parent for the new project. The parent is identified by a specified ResourceId, which
* must include both an ID and a type, such as organization.
*
* This method does not associate the new project with a billing account. You can set or update the
* billing account associated with a project using the [`projects.updateBillingInfo`]
* (/billing/reference/rest/v1/projects/updateBillingInfo) method.
*
* Create a request for the method "projects.create".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.cloudresourcemanager.model.Project}
* @return the request
*/
public Create create(com.google.api.services.cloudresourcemanager.model.Project content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects";
/**
* Request that a new Project be created. The result is an Operation which can be used to track
* the creation process. This process usually takes a few seconds, but can sometimes take much
* longer. The tracking Operation is automatically deleted after a few hours, so there is no need
* to call DeleteOperation.
*
* Authorization requires the Google IAM permission `resourcemanager.projects.create` on the
* specified parent for the new project. The parent is identified by a specified ResourceId, which
* must include both an ID and a type, such as organization.
*
* This method does not associate the new project with a billing account. You can set or update
* the billing account associated with a project using the [`projects.updateBillingInfo`]
* (/billing/reference/rest/v1/projects/updateBillingInfo) method.
*
* Create a request for the method "projects.create".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.cloudresourcemanager.model.Project}
* @since 1.13
*/
protected Create(com.google.api.services.cloudresourcemanager.model.Project content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Operation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Marks the Project identified by the specified `project_id` (for example, `my-project-123`) for
* deletion. This method will only affect the Project if it has a lifecycle state of ACTIVE.
*
* This method changes the Project's lifecycle state from ACTIVE to DELETE_REQUESTED. The deletion
* starts at an unspecified time, at which point the Project is no longer accessible.
*
* Until the deletion completes, you can check the lifecycle state checked by retrieving the Project
* with GetProject, and the Project remains visible to ListProjects. However, you cannot update the
* project.
*
* After the deletion completes, the Project is not retrievable by the GetProject and ListProjects
* methods.
*
* The caller must have modify permissions for this Project.
*
* Create a request for the method "projects.delete".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param projectId The Project ID (for example, `foo-bar-123`).
Required.
* @return the request
*/
public Delete delete(java.lang.String projectId) throws java.io.IOException {
Delete result = new Delete(projectId);
initialize(result);
return result;
}
public class Delete extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{projectId}";
/**
* Marks the Project identified by the specified `project_id` (for example, `my-project-123`) for
* deletion. This method will only affect the Project if it has a lifecycle state of ACTIVE.
*
* This method changes the Project's lifecycle state from ACTIVE to DELETE_REQUESTED. The deletion
* starts at an unspecified time, at which point the Project is no longer accessible.
*
* Until the deletion completes, you can check the lifecycle state checked by retrieving the
* Project with GetProject, and the Project remains visible to ListProjects. However, you cannot
* update the project.
*
* After the deletion completes, the Project is not retrievable by the GetProject and
* ListProjects methods.
*
* The caller must have modify permissions for this Project.
*
* Create a request for the method "projects.delete".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The Project ID (for example, `foo-bar-123`).
Required.
* @since 1.13
*/
protected Delete(java.lang.String projectId) {
super(CloudResourceManager.this, "DELETE", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.Empty.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The Project ID (for example, `foo-bar-123`).
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The Project ID (for example, `foo-bar-123`).
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The Project ID (for example, `foo-bar-123`).
*
* Required.
*/
public Delete setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the Project identified by the specified `project_id` (for example, `my-project-123`).
*
* The caller must have read permissions for this Project.
*
* Create a request for the method "projects.get".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId The Project ID (for example, `my-project-123`).
Required.
* @return the request
*/
public Get get(java.lang.String projectId) throws java.io.IOException {
Get result = new Get(projectId);
initialize(result);
return result;
}
public class Get extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{projectId}";
/**
* Retrieves the Project identified by the specified `project_id` (for example, `my-project-123`).
*
* The caller must have read permissions for this Project.
*
* Create a request for the method "projects.get".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The Project ID (for example, `my-project-123`).
Required.
* @since 1.13
*/
protected Get(java.lang.String projectId) {
super(CloudResourceManager.this, "GET", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.Project.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The Project ID (for example, `my-project-123`).
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The Project ID (for example, `my-project-123`).
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The Project ID (for example, `my-project-123`).
*
* Required.
*/
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets a list of ancestors in the resource hierarchy for the Project identified by the specified
* `project_id` (for example, `my-project-123`).
*
* The caller must have read permissions for this Project.
*
* Create a request for the method "projects.getAncestry".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetAncestry#execute()} method to invoke the remote
* operation.
*
* @param projectId The Project ID (for example, `my-project-123`).
Required.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetAncestryRequest}
* @return the request
*/
public GetAncestry getAncestry(java.lang.String projectId, com.google.api.services.cloudresourcemanager.model.GetAncestryRequest content) throws java.io.IOException {
GetAncestry result = new GetAncestry(projectId, content);
initialize(result);
return result;
}
public class GetAncestry extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{projectId}:getAncestry";
/**
* Gets a list of ancestors in the resource hierarchy for the Project identified by the specified
* `project_id` (for example, `my-project-123`).
*
* The caller must have read permissions for this Project.
*
* Create a request for the method "projects.getAncestry".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetAncestry#execute()} method to invoke the remote
* operation. {@link
* GetAncestry#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The Project ID (for example, `my-project-123`).
Required.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetAncestryRequest}
* @since 1.13
*/
protected GetAncestry(java.lang.String projectId, com.google.api.services.cloudresourcemanager.model.GetAncestryRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.GetAncestryResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public GetAncestry set$Xgafv(java.lang.String $Xgafv) {
return (GetAncestry) super.set$Xgafv($Xgafv);
}
@Override
public GetAncestry setAlt(java.lang.String alt) {
return (GetAncestry) super.setAlt(alt);
}
@Override
public GetAncestry setCallback(java.lang.String callback) {
return (GetAncestry) super.setCallback(callback);
}
@Override
public GetAncestry setFields(java.lang.String fields) {
return (GetAncestry) super.setFields(fields);
}
@Override
public GetAncestry setKey(java.lang.String key) {
return (GetAncestry) super.setKey(key);
}
@Override
public GetAncestry setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAncestry) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAncestry setQuotaUser(java.lang.String quotaUser) {
return (GetAncestry) super.setQuotaUser(quotaUser);
}
@Override
public GetAncestry setUploadType(java.lang.String uploadType) {
return (GetAncestry) super.setUploadType(uploadType);
}
@Override
public GetAncestry setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAncestry) super.setUploadProtocol(uploadProtocol);
}
/**
* The Project ID (for example, `my-project-123`).
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The Project ID (for example, `my-project-123`).
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The Project ID (for example, `my-project-123`).
*
* Required.
*/
public GetAncestry setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public GetAncestry set(String parameterName, Object value) {
return (GetAncestry) super.set(parameterName, value);
}
}
/**
* Gets the effective `Policy` on a resource. This is the result of merging `Policies` in the
* resource hierarchy. The returned `Policy` will not have an `etag`set because it is a computed
* `Policy` across multiple resources. Subtrees of Resource Manager resource hierarchy with 'under:'
* prefix will not be expanded.
*
* Create a request for the method "projects.getEffectiveOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetEffectiveOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource The name of the resource to start computing the effective `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest}
* @return the request
*/
public GetEffectiveOrgPolicy getEffectiveOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest content) throws java.io.IOException {
GetEffectiveOrgPolicy result = new GetEffectiveOrgPolicy(resource, content);
initialize(result);
return result;
}
public class GetEffectiveOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getEffectiveOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Gets the effective `Policy` on a resource. This is the result of merging `Policies` in the
* resource hierarchy. The returned `Policy` will not have an `etag`set because it is a computed
* `Policy` across multiple resources. Subtrees of Resource Manager resource hierarchy with
* 'under:' prefix will not be expanded.
*
* Create a request for the method "projects.getEffectiveOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetEffectiveOrgPolicy#execute()} method to invoke the
* remote operation. {@link GetEffectiveOrgPolicy#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource The name of the resource to start computing the effective `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest}
* @since 1.13
*/
protected GetEffectiveOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetEffectiveOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public GetEffectiveOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetEffectiveOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetEffectiveOrgPolicy setAlt(java.lang.String alt) {
return (GetEffectiveOrgPolicy) super.setAlt(alt);
}
@Override
public GetEffectiveOrgPolicy setCallback(java.lang.String callback) {
return (GetEffectiveOrgPolicy) super.setCallback(callback);
}
@Override
public GetEffectiveOrgPolicy setFields(java.lang.String fields) {
return (GetEffectiveOrgPolicy) super.setFields(fields);
}
@Override
public GetEffectiveOrgPolicy setKey(java.lang.String key) {
return (GetEffectiveOrgPolicy) super.setKey(key);
}
@Override
public GetEffectiveOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetEffectiveOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetEffectiveOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetEffectiveOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetEffectiveOrgPolicy setUploadType(java.lang.String uploadType) {
return (GetEffectiveOrgPolicy) super.setUploadType(uploadType);
}
@Override
public GetEffectiveOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetEffectiveOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** The name of the resource to start computing the effective `Policy`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** The name of the resource to start computing the effective `Policy`.
*/
public java.lang.String getResource() {
return resource;
}
/** The name of the resource to start computing the effective `Policy`. */
public GetEffectiveOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetEffectiveOrgPolicy set(String parameterName, Object value) {
return (GetEffectiveOrgPolicy) super.set(parameterName, value);
}
}
/**
* Returns the IAM access control policy for the specified Project. Permission is denied if the
* policy or the resource does not exist.
*
* Authorization requires the Google IAM permission `resourcemanager.projects.getIamPolicy` on the
* project.
*
* For additional information about resource structure and identification, see [Resource
* Names](/apis/design/resource_names).
*
* Create a request for the method "projects.getIamPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{resource}:getIamPolicy";
/**
* Returns the IAM access control policy for the specified Project. Permission is denied if the
* policy or the resource does not exist.
*
* Authorization requires the Google IAM permission `resourcemanager.projects.getIamPolicy` on the
* project.
*
* For additional information about resource structure and identification, see [Resource
* Names](/apis/design/resource_names).
*
* Create a request for the method "projects.getIamPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetIamPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Gets a `Policy` on a resource.
*
* If no `Policy` is set on the resource, a `Policy` is returned with default values including
* `POLICY_TYPE_NOT_SET` for the `policy_type oneof`. The `etag` value can be used with
* `SetOrgPolicy()` to create or update a `Policy` during read-modify-write.
*
* Create a request for the method "projects.getOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link GetOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource the `Policy` is set on.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest}
* @return the request
*/
public GetOrgPolicy getOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest content) throws java.io.IOException {
GetOrgPolicy result = new GetOrgPolicy(resource, content);
initialize(result);
return result;
}
public class GetOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Gets a `Policy` on a resource.
*
* If no `Policy` is set on the resource, a `Policy` is returned with default values including
* `POLICY_TYPE_NOT_SET` for the `policy_type oneof`. The `etag` value can be used with
* `SetOrgPolicy()` to create or update a `Policy` during read-modify-write.
*
* Create a request for the method "projects.getOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link GetOrgPolicy#execute()} method to invoke the remote
* operation. {@link
* GetOrgPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource Name of the resource the `Policy` is set on.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest}
* @since 1.13
*/
protected GetOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.GetOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public GetOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetOrgPolicy setAlt(java.lang.String alt) {
return (GetOrgPolicy) super.setAlt(alt);
}
@Override
public GetOrgPolicy setCallback(java.lang.String callback) {
return (GetOrgPolicy) super.setCallback(callback);
}
@Override
public GetOrgPolicy setFields(java.lang.String fields) {
return (GetOrgPolicy) super.setFields(fields);
}
@Override
public GetOrgPolicy setKey(java.lang.String key) {
return (GetOrgPolicy) super.setKey(key);
}
@Override
public GetOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetOrgPolicy setUploadType(java.lang.String uploadType) {
return (GetOrgPolicy) super.setUploadType(uploadType);
}
@Override
public GetOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource the `Policy` is set on. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource the `Policy` is set on.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource the `Policy` is set on. */
public GetOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetOrgPolicy set(String parameterName, Object value) {
return (GetOrgPolicy) super.set(parameterName, value);
}
}
/**
* Lists Projects that the caller has the `resourcemanager.projects.get` permission on and satisfy
* the specified filter.
*
* This method returns Projects in an unspecified order. This method is eventually consistent with
* project mutations; this means that a newly created project may not appear in the results or
* recent updates to an existing project may not be reflected in the results. To retrieve the latest
* state of a project, use the GetProject method.
*
* NOTE: If the request filter contains a `parent.type` and `parent.id` and the caller has the
* `resourcemanager.projects.list` permission on the parent, the results will be drawn from an
* alternate index which provides more consistent results. In future versions of this API, this List
* method will be split into List and Search to properly capture the behavorial difference.
*
* Create a request for the method "projects.list".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects";
/**
* Lists Projects that the caller has the `resourcemanager.projects.get` permission on and satisfy
* the specified filter.
*
* This method returns Projects in an unspecified order. This method is eventually consistent with
* project mutations; this means that a newly created project may not appear in the results or
* recent updates to an existing project may not be reflected in the results. To retrieve the
* latest state of a project, use the GetProject method.
*
* NOTE: If the request filter contains a `parent.type` and `parent.id` and the caller has the
* `resourcemanager.projects.list` permission on the parent, the results will be drawn from an
* alternate index which provides more consistent results. In future versions of this API, this
* List method will be split into List and Search to properly capture the behavorial difference.
*
* Create a request for the method "projects.list".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(CloudResourceManager.this, "GET", REST_PATH, null, com.google.api.services.cloudresourcemanager.model.ListProjectsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* An expression for filtering the results of the request. Filter rules are case insensitive.
* The fields eligible for filtering are:
*
* + `name` + `id` + `labels.` (where *key* is the name of a label) + `parent.type` +
* `parent.id`
*
* Some examples of using labels as filters:
*
* | Filter | Description |
* |------------------|-----------------------------------------------------| | name:how*
* | The project's name starts with "how". | | name:Howl | The project's
* name is `Howl` or `howl`. | | name:HOWL | Equivalent to above.
* | | NAME:howl | Equivalent to above. | |
* labels.color:* | The project has the label `color`. | | labels.color:red
* | The project's label `color` has the value `red`. | | labels.color:redlabels.size:big
* |The project's label `color` has the value `red` and its label `size` has the value `big`.
* |
*
* If no filter is specified, the call will return projects for which the user has the
* `resourcemanager.projects.get` permission.
*
* NOTE: To perform a by-parent query (eg., what projects are directly in a Folder), the
* caller must have the `resourcemanager.projects.list` permission on the parent and the
* filter must contain both a `parent.type` and a `parent.id` restriction (example:
* "parent.type:folder parent.id:123"). In this case an alternate search index is used which
* provides more consistent results.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An expression for filtering the results of the request. Filter rules are case insensitive. The
fields eligible for filtering are:
+ `name` + `id` + `labels.` (where *key* is the name of a label) + `parent.type` + `parent.id`
Some examples of using labels as filters:
| Filter | Description |
|------------------|-----------------------------------------------------| | name:how* | The
project's name starts with "how". | | name:Howl | The project's name is `Howl`
or `howl`. | | name:HOWL | Equivalent to above. |
| NAME:howl | Equivalent to above. | | labels.color:* | The
project has the label `color`. | | labels.color:red | The project's label `color`
has the value `red`. | | labels.color:redlabels.size:big |The project's label `color` has the
value `red` and its label `size` has the value `big`. |
If no filter is specified, the call will return projects for which the user has the
`resourcemanager.projects.get` permission.
NOTE: To perform a by-parent query (eg., what projects are directly in a Folder), the caller must
have the `resourcemanager.projects.list` permission on the parent and the filter must contain both
a `parent.type` and a `parent.id` restriction (example: "parent.type:folder parent.id:123"). In
this case an alternate search index is used which provides more consistent results.
Optional.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An expression for filtering the results of the request. Filter rules are case insensitive.
* The fields eligible for filtering are:
*
* + `name` + `id` + `labels.` (where *key* is the name of a label) + `parent.type` +
* `parent.id`
*
* Some examples of using labels as filters:
*
* | Filter | Description |
* |------------------|-----------------------------------------------------| | name:how*
* | The project's name starts with "how". | | name:Howl | The project's
* name is `Howl` or `howl`. | | name:HOWL | Equivalent to above.
* | | NAME:howl | Equivalent to above. | |
* labels.color:* | The project has the label `color`. | | labels.color:red
* | The project's label `color` has the value `red`. | | labels.color:redlabels.size:big
* |The project's label `color` has the value `red` and its label `size` has the value `big`.
* |
*
* If no filter is specified, the call will return projects for which the user has the
* `resourcemanager.projects.get` permission.
*
* NOTE: To perform a by-parent query (eg., what projects are directly in a Folder), the
* caller must have the `resourcemanager.projects.list` permission on the parent and the
* filter must contain both a `parent.type` and a `parent.id` restriction (example:
* "parent.type:folder parent.id:123"). In this case an alternate search index is used which
* provides more consistent results.
*
* Optional.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of Projects to return in the response. The server can return fewer
* Projects than requested. If unspecified, server picks an appropriate default.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of Projects to return in the response. The server can return fewer Projects than
requested. If unspecified, server picks an appropriate default.
Optional.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of Projects to return in the response. The server can return fewer
* Projects than requested. If unspecified, server picks an appropriate default.
*
* Optional.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A pagination token returned from a previous call to ListProjects that indicates from where
* listing should continue.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A pagination token returned from a previous call to ListProjects that indicates from where listing
should continue.
Optional.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A pagination token returned from a previous call to ListProjects that indicates from where
* listing should continue.
*
* Optional.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists `Constraints` that could be applied on the specified resource.
*
* Create a request for the method "projects.listAvailableOrgPolicyConstraints".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ListAvailableOrgPolicyConstraints#execute()} method to
* invoke the remote operation.
*
* @param resource Name of the resource to list `Constraints` for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest}
* @return the request
*/
public ListAvailableOrgPolicyConstraints listAvailableOrgPolicyConstraints(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest content) throws java.io.IOException {
ListAvailableOrgPolicyConstraints result = new ListAvailableOrgPolicyConstraints(resource, content);
initialize(result);
return result;
}
public class ListAvailableOrgPolicyConstraints extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:listAvailableOrgPolicyConstraints";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists `Constraints` that could be applied on the specified resource.
*
* Create a request for the method "projects.listAvailableOrgPolicyConstraints".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ListAvailableOrgPolicyConstraints#execute()} method to
* invoke the remote operation. {@link ListAvailableOrgPolicyConstraints#initialize(com.google
* .api.client.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this
* instance immediately after invoking the constructor.
*
* @param resource Name of the resource to list `Constraints` for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest}
* @since 1.13
*/
protected ListAvailableOrgPolicyConstraints(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.ListAvailableOrgPolicyConstraintsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public ListAvailableOrgPolicyConstraints set$Xgafv(java.lang.String $Xgafv) {
return (ListAvailableOrgPolicyConstraints) super.set$Xgafv($Xgafv);
}
@Override
public ListAvailableOrgPolicyConstraints setAlt(java.lang.String alt) {
return (ListAvailableOrgPolicyConstraints) super.setAlt(alt);
}
@Override
public ListAvailableOrgPolicyConstraints setCallback(java.lang.String callback) {
return (ListAvailableOrgPolicyConstraints) super.setCallback(callback);
}
@Override
public ListAvailableOrgPolicyConstraints setFields(java.lang.String fields) {
return (ListAvailableOrgPolicyConstraints) super.setFields(fields);
}
@Override
public ListAvailableOrgPolicyConstraints setKey(java.lang.String key) {
return (ListAvailableOrgPolicyConstraints) super.setKey(key);
}
@Override
public ListAvailableOrgPolicyConstraints setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListAvailableOrgPolicyConstraints) super.setPrettyPrint(prettyPrint);
}
@Override
public ListAvailableOrgPolicyConstraints setQuotaUser(java.lang.String quotaUser) {
return (ListAvailableOrgPolicyConstraints) super.setQuotaUser(quotaUser);
}
@Override
public ListAvailableOrgPolicyConstraints setUploadType(java.lang.String uploadType) {
return (ListAvailableOrgPolicyConstraints) super.setUploadType(uploadType);
}
@Override
public ListAvailableOrgPolicyConstraints setUploadProtocol(java.lang.String uploadProtocol) {
return (ListAvailableOrgPolicyConstraints) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource to list `Constraints` for. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource to list `Constraints` for.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource to list `Constraints` for. */
public ListAvailableOrgPolicyConstraints setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ListAvailableOrgPolicyConstraints set(String parameterName, Object value) {
return (ListAvailableOrgPolicyConstraints) super.set(parameterName, value);
}
}
/**
* Lists all the `Policies` set for a particular resource.
*
* Create a request for the method "projects.listOrgPolicies".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link ListOrgPolicies#execute()} method to invoke the remote
* operation.
*
* @param resource Name of the resource to list Policies for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest}
* @return the request
*/
public ListOrgPolicies listOrgPolicies(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest content) throws java.io.IOException {
ListOrgPolicies result = new ListOrgPolicies(resource, content);
initialize(result);
return result;
}
public class ListOrgPolicies extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:listOrgPolicies";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists all the `Policies` set for a particular resource.
*
* Create a request for the method "projects.listOrgPolicies".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link ListOrgPolicies#execute()} method to invoke the remote
* operation. {@link ListOrgPolicies#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param resource Name of the resource to list Policies for.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest}
* @since 1.13
*/
protected ListOrgPolicies(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.ListOrgPoliciesResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public ListOrgPolicies set$Xgafv(java.lang.String $Xgafv) {
return (ListOrgPolicies) super.set$Xgafv($Xgafv);
}
@Override
public ListOrgPolicies setAlt(java.lang.String alt) {
return (ListOrgPolicies) super.setAlt(alt);
}
@Override
public ListOrgPolicies setCallback(java.lang.String callback) {
return (ListOrgPolicies) super.setCallback(callback);
}
@Override
public ListOrgPolicies setFields(java.lang.String fields) {
return (ListOrgPolicies) super.setFields(fields);
}
@Override
public ListOrgPolicies setKey(java.lang.String key) {
return (ListOrgPolicies) super.setKey(key);
}
@Override
public ListOrgPolicies setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListOrgPolicies) super.setPrettyPrint(prettyPrint);
}
@Override
public ListOrgPolicies setQuotaUser(java.lang.String quotaUser) {
return (ListOrgPolicies) super.setQuotaUser(quotaUser);
}
@Override
public ListOrgPolicies setUploadType(java.lang.String uploadType) {
return (ListOrgPolicies) super.setUploadType(uploadType);
}
@Override
public ListOrgPolicies setUploadProtocol(java.lang.String uploadProtocol) {
return (ListOrgPolicies) super.setUploadProtocol(uploadProtocol);
}
/** Name of the resource to list Policies for. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name of the resource to list Policies for.
*/
public java.lang.String getResource() {
return resource;
}
/** Name of the resource to list Policies for. */
public ListOrgPolicies setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public ListOrgPolicies set(String parameterName, Object value) {
return (ListOrgPolicies) super.set(parameterName, value);
}
}
/**
* Sets the IAM access control policy for the specified Project.
*
* CAUTION: This method will replace the existing policy, and cannot be used to append additional
* IAM settings.
*
* NOTE: Removing service accounts from policies or changing their roles can render services
* completely inoperable. It is important to understand how the service account is being used before
* removing or updating its roles.
*
* The following constraints apply when using `setIamPolicy()`:
*
* + Project does not support `allUsers` and `allAuthenticatedUsers` as `members` in a `Binding` of
* a `Policy`.
*
* + The owner role can be granted to a `user`, `serviceAccount`, or a group that is part of an
* organization. For example, [email protected] could be added as an owner to a project
* in the myownpersonaldomain.com organization, but not the examplepetstore.com organization.
*
* + Service accounts can be made owners of a project directly without any restrictions. However, to
* be added as an owner, a user must be invited via Cloud Platform console and must accept the
* invitation.
*
* + A user cannot be granted the owner role using `setIamPolicy()`. The user must be granted the
* owner role using the Cloud Platform Console and must explicitly accept the invitation.
*
* + You can only grant ownership of a project to a member by using the GCP Console. Inviting a
* member will deliver an invitation email that they must accept. An invitation email is not
* generated if you are granting a role other than owner, or if both the member you are inviting and
* the project are part of your organization.
*
* + Membership changes that leave the project without any owners that have accepted the Terms of
* Service (ToS) will be rejected.
*
* + If the project is not part of an organization, there must be at least one owner who has
* accepted the Terms of Service (ToS) agreement in the policy. Calling `setIamPolicy()` to remove
* the last ToS-accepted owner from the policy will fail. This restriction also applies to legacy
* projects that no longer have owners who have accepted the ToS. Edits to IAM policies will be
* rejected until the lack of a ToS-accepting owner is rectified.
*
* Authorization requires the Google IAM permission `resourcemanager.projects.setIamPolicy` on the
* project
*
* Create a request for the method "projects.setIamPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{resource}:setIamPolicy";
/**
* Sets the IAM access control policy for the specified Project.
*
* CAUTION: This method will replace the existing policy, and cannot be used to append additional
* IAM settings.
*
* NOTE: Removing service accounts from policies or changing their roles can render services
* completely inoperable. It is important to understand how the service account is being used
* before removing or updating its roles.
*
* The following constraints apply when using `setIamPolicy()`:
*
* + Project does not support `allUsers` and `allAuthenticatedUsers` as `members` in a `Binding`
* of a `Policy`.
*
* + The owner role can be granted to a `user`, `serviceAccount`, or a group that is part of an
* organization. For example, [email protected] could be added as an owner to a
* project in the myownpersonaldomain.com organization, but not the examplepetstore.com
* organization.
*
* + Service accounts can be made owners of a project directly without any restrictions. However,
* to be added as an owner, a user must be invited via Cloud Platform console and must accept the
* invitation.
*
* + A user cannot be granted the owner role using `setIamPolicy()`. The user must be granted the
* owner role using the Cloud Platform Console and must explicitly accept the invitation.
*
* + You can only grant ownership of a project to a member by using the GCP Console. Inviting a
* member will deliver an invitation email that they must accept. An invitation email is not
* generated if you are granting a role other than owner, or if both the member you are inviting
* and the project are part of your organization.
*
* + Membership changes that leave the project without any owners that have accepted the Terms of
* Service (ToS) will be rejected.
*
* + If the project is not part of an organization, there must be at least one owner who has
* accepted the Terms of Service (ToS) agreement in the policy. Calling `setIamPolicy()` to remove
* the last ToS-accepted owner from the policy will fail. This restriction also applies to legacy
* projects that no longer have owners who have accepted the ToS. Edits to IAM policies will be
* rejected until the lack of a ToS-accepting owner is rectified.
*
* Authorization requires the Google IAM permission `resourcemanager.projects.setIamPolicy` on the
* project
*
* Create a request for the method "projects.setIamPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetIamPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Updates the specified `Policy` on the resource. Creates a new `Policy` for that `Constraint` on
* the resource if one does not exist.
*
* Not supplying an `etag` on the request `Policy` results in an unconditional write of the
* `Policy`.
*
* Create a request for the method "projects.setOrgPolicy".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link SetOrgPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource Resource name of the resource to attach the `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest}
* @return the request
*/
public SetOrgPolicy setOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest content) throws java.io.IOException {
SetOrgPolicy result = new SetOrgPolicy(resource, content);
initialize(result);
return result;
}
public class SetOrgPolicy extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/{+resource}:setOrgPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Updates the specified `Policy` on the resource. Creates a new `Policy` for that `Constraint` on
* the resource if one does not exist.
*
* Not supplying an `etag` on the request `Policy` results in an unconditional write of the
* `Policy`.
*
* Create a request for the method "projects.setOrgPolicy".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link SetOrgPolicy#execute()} method to invoke the remote
* operation. {@link
* SetOrgPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource Resource name of the resource to attach the `Policy`.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest}
* @since 1.13
*/
protected SetOrgPolicy(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.SetOrgPolicyRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.OrgPolicy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public SetOrgPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetOrgPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetOrgPolicy setAlt(java.lang.String alt) {
return (SetOrgPolicy) super.setAlt(alt);
}
@Override
public SetOrgPolicy setCallback(java.lang.String callback) {
return (SetOrgPolicy) super.setCallback(callback);
}
@Override
public SetOrgPolicy setFields(java.lang.String fields) {
return (SetOrgPolicy) super.setFields(fields);
}
@Override
public SetOrgPolicy setKey(java.lang.String key) {
return (SetOrgPolicy) super.setKey(key);
}
@Override
public SetOrgPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetOrgPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetOrgPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetOrgPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetOrgPolicy setUploadType(java.lang.String uploadType) {
return (SetOrgPolicy) super.setUploadType(uploadType);
}
@Override
public SetOrgPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetOrgPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Resource name of the resource to attach the `Policy`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Resource name of the resource to attach the `Policy`.
*/
public java.lang.String getResource() {
return resource;
}
/** Resource name of the resource to attach the `Policy`. */
public SetOrgPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetOrgPolicy set(String parameterName, Object value) {
return (SetOrgPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified Project.
*
* There are no permissions required for making this API call.
*
* Create a request for the method "projects.testIamPermissions".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested.
See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{resource}:testIamPermissions";
/**
* Returns permissions that a caller has on the specified Project.
*
* There are no permissions required for making this API call.
*
* Create a request for the method "projects.testIamPermissions".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested.
See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.cloudresourcemanager.model.TestIamPermissionsRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Restores the Project identified by the specified `project_id` (for example, `my-project-123`).
* You can only use this method for a Project that has a lifecycle state of DELETE_REQUESTED. After
* deletion starts, the Project cannot be restored.
*
* The caller must have modify permissions for this Project.
*
* Create a request for the method "projects.undelete".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param projectId The project ID (for example, `foo-bar-123`).
Required.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.UndeleteProjectRequest}
* @return the request
*/
public Undelete undelete(java.lang.String projectId, com.google.api.services.cloudresourcemanager.model.UndeleteProjectRequest content) throws java.io.IOException {
Undelete result = new Undelete(projectId, content);
initialize(result);
return result;
}
public class Undelete extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{projectId}:undelete";
/**
* Restores the Project identified by the specified `project_id` (for example, `my-project-123`).
* You can only use this method for a Project that has a lifecycle state of DELETE_REQUESTED.
* After deletion starts, the Project cannot be restored.
*
* The caller must have modify permissions for this Project.
*
* Create a request for the method "projects.undelete".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Undelete#execute()} method to invoke the remote
* operation. {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The project ID (for example, `foo-bar-123`).
Required.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.UndeleteProjectRequest}
* @since 1.13
*/
protected Undelete(java.lang.String projectId, com.google.api.services.cloudresourcemanager.model.UndeleteProjectRequest content) {
super(CloudResourceManager.this, "POST", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Empty.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID (for example, `foo-bar-123`).
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The project ID (for example, `foo-bar-123`).
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The project ID (for example, `foo-bar-123`).
*
* Required.
*/
public Undelete setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
/**
* Updates the attributes of the Project identified by the specified `project_id` (for example, `my-
* project-123`).
*
* The caller must have modify permissions for this Project.
*
* Create a request for the method "projects.update".
*
* This request holds the parameters needed by the cloudresourcemanager server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param projectId The project ID (for example, `my-project-123`).
Required.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.Project}
* @return the request
*/
public Update update(java.lang.String projectId, com.google.api.services.cloudresourcemanager.model.Project content) throws java.io.IOException {
Update result = new Update(projectId, content);
initialize(result);
return result;
}
public class Update extends CloudResourceManagerRequest {
private static final String REST_PATH = "v1/projects/{projectId}";
/**
* Updates the attributes of the Project identified by the specified `project_id` (for example,
* `my-project-123`).
*
* The caller must have modify permissions for this Project.
*
* Create a request for the method "projects.update".
*
* This request holds the parameters needed by the the cloudresourcemanager server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The project ID (for example, `my-project-123`).
Required.
* @param content the {@link com.google.api.services.cloudresourcemanager.model.Project}
* @since 1.13
*/
protected Update(java.lang.String projectId, com.google.api.services.cloudresourcemanager.model.Project content) {
super(CloudResourceManager.this, "PUT", REST_PATH, content, com.google.api.services.cloudresourcemanager.model.Project.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID (for example, `my-project-123`).
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The project ID (for example, `my-project-123`).
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The project ID (for example, `my-project-123`).
*
* Required.
*/
public Update setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link CloudResourceManager}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudResourceManager}. */
@Override
public CloudResourceManager build() {
return new CloudResourceManager(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudResourceManagerRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudResourceManagerRequestInitializer(
CloudResourceManagerRequestInitializer cloudresourcemanagerRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudresourcemanagerRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}