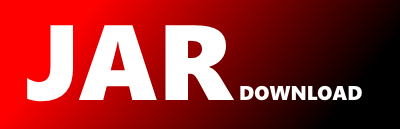
target.apidocs.com.google.api.services.cloudresourcemanager.model.Project.html Maven / Gradle / Ivy
Project (Cloud Resource Manager API v1-rev20200608-1.30.9)
com.google.api.services.cloudresourcemanager.model
Class Project
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.cloudresourcemanager.model.Project
-
public final class Project
extends GenericJson
A Project is a high-level Google Cloud Platform entity. It is a container for ACLs, APIs, App
Engine Apps, VMs, and other Google Cloud Platform resources.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Cloud Resource Manager API. For a detailed
explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Project()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Project
clone()
String
getCreateTime()
Creation time.
Map<String,String>
getLabels()
The labels associated with this Project.
String
getLifecycleState()
The Project lifecycle state.
String
getName()
The optional user-assigned display name of the Project.
ResourceId
getParent()
An optional reference to a parent Resource.
String
getProjectId()
The unique, user-assigned ID of the Project.
Long
getProjectNumber()
The number uniquely identifying the project.
Project
set(String fieldName,
Object value)
Project
setCreateTime(String createTime)
Creation time.
Project
setLabels(Map<String,String> labels)
The labels associated with this Project.
Project
setLifecycleState(String lifecycleState)
The Project lifecycle state.
Project
setName(String name)
The optional user-assigned display name of the Project.
Project
setParent(ResourceId parent)
An optional reference to a parent Resource.
Project
setProjectId(String projectId)
The unique, user-assigned ID of the Project.
Project
setProjectNumber(Long projectNumber)
The number uniquely identifying the project.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCreateTime
public String getCreateTime()
Creation time.
Read-only.
- Returns:
- value or
null
for none
-
setCreateTime
public Project setCreateTime(String createTime)
Creation time.
Read-only.
- Parameters:
createTime
- createTime or null
for none
-
getLabels
public Map<String,String> getLabels()
The labels associated with this Project.
Label keys must be between 1 and 63 characters long and must conform to the following regular
expression: \[a-z\](\[-a-z0-9\]*\[a-z0-9\])?.
Label values must be between 0 and 63 characters long and must conform to the regular
expression (\[a-z\](\[-a-z0-9\]*\[a-z0-9\])?)?. A label value can be empty.
No more than 256 labels can be associated with a given resource.
Clients should store labels in a representation such as JSON that does not depend on specific
characters being disallowed.
Example: "environment" : "dev" Read-write.
- Returns:
- value or
null
for none
-
setLabels
public Project setLabels(Map<String,String> labels)
The labels associated with this Project.
Label keys must be between 1 and 63 characters long and must conform to the following regular
expression: \[a-z\](\[-a-z0-9\]*\[a-z0-9\])?.
Label values must be between 0 and 63 characters long and must conform to the regular
expression (\[a-z\](\[-a-z0-9\]*\[a-z0-9\])?)?. A label value can be empty.
No more than 256 labels can be associated with a given resource.
Clients should store labels in a representation such as JSON that does not depend on specific
characters being disallowed.
Example: "environment" : "dev" Read-write.
- Parameters:
labels
- labels or null
for none
-
getLifecycleState
public String getLifecycleState()
The Project lifecycle state.
Read-only.
- Returns:
- value or
null
for none
-
setLifecycleState
public Project setLifecycleState(String lifecycleState)
The Project lifecycle state.
Read-only.
- Parameters:
lifecycleState
- lifecycleState or null
for none
-
getName
public String getName()
The optional user-assigned display name of the Project. When present it must be between 4 to 30
characters. Allowed characters are: lowercase and uppercase letters, numbers, hyphen, single-
quote, double-quote, space, and exclamation point.
Example: My Project Read-write.
- Returns:
- value or
null
for none
-
setName
public Project setName(String name)
The optional user-assigned display name of the Project. When present it must be between 4 to 30
characters. Allowed characters are: lowercase and uppercase letters, numbers, hyphen, single-
quote, double-quote, space, and exclamation point.
Example: My Project Read-write.
- Parameters:
name
- name or null
for none
-
getParent
public ResourceId getParent()
An optional reference to a parent Resource.
Supported parent types include "organization" and "folder". Once set, the parent cannot be
cleared. The `parent` can be set on creation or using the `UpdateProject` method; the end user
must have the `resourcemanager.projects.create` permission on the parent.
Read-write.
- Returns:
- value or
null
for none
-
setParent
public Project setParent(ResourceId parent)
An optional reference to a parent Resource.
Supported parent types include "organization" and "folder". Once set, the parent cannot be
cleared. The `parent` can be set on creation or using the `UpdateProject` method; the end user
must have the `resourcemanager.projects.create` permission on the parent.
Read-write.
- Parameters:
parent
- parent or null
for none
-
getProjectId
public String getProjectId()
The unique, user-assigned ID of the Project. It must be 6 to 30 lowercase letters, digits, or
hyphens. It must start with a letter. Trailing hyphens are prohibited.
Example: tokyo-rain-123 Read-only after creation.
- Returns:
- value or
null
for none
-
setProjectId
public Project setProjectId(String projectId)
The unique, user-assigned ID of the Project. It must be 6 to 30 lowercase letters, digits, or
hyphens. It must start with a letter. Trailing hyphens are prohibited.
Example: tokyo-rain-123 Read-only after creation.
- Parameters:
projectId
- projectId or null
for none
-
getProjectNumber
public Long getProjectNumber()
The number uniquely identifying the project.
Example: 415104041262 Read-only.
- Returns:
- value or
null
for none
-
setProjectNumber
public Project setProjectNumber(Long projectNumber)
The number uniquely identifying the project.
Example: 415104041262 Read-only.
- Parameters:
projectNumber
- projectNumber or null
for none
-
set
public Project set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Project clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy