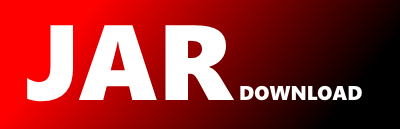
com.google.api.services.cloudtrace.v2beta1.CloudTrace Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.cloudtrace.v2beta1;
/**
* Service definition for CloudTrace (v2beta1).
*
*
* Sends application trace data to Cloud Trace for viewing. Trace data is collected for all App Engine applications by default. Trace data from other applications can be provided using this API. This library is used to interact with the Cloud Trace API directly. If you are looking to instrument your application for Cloud Trace, we recommend using OpenCensus.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudTraceRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudTrace extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1)),
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"1.31.0 of the Cloud Trace API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://cloudtrace.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://cloudtrace.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudTrace(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudTrace(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code CloudTrace cloudtrace = new CloudTrace(...);}
* {@code CloudTrace.Projects.List request = cloudtrace.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the TraceSinks collection.
*
* The typical use is:
*
* {@code CloudTrace cloudtrace = new CloudTrace(...);}
* {@code CloudTrace.TraceSinks.List request = cloudtrace.traceSinks().list(parameters ...)}
*
*
* @return the resource collection
*/
public TraceSinks traceSinks() {
return new TraceSinks();
}
/**
* The "traceSinks" collection of methods.
*/
public class TraceSinks {
/**
* Creates a sink that exports trace spans to a destination. The export of newly-ingested traces
* begins immediately, unless the sink's `writer_identity` is not permitted to write to the
* destination. A sink can export traces only from the resource owning the sink (the 'parent').
*
* Create a request for the method "traceSinks.create".
*
* This request holds the parameters needed by the cloudtrace server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the sink (currently only project sinks are supported):
* "projects/[PROJECT_ID]" Examples: `"projects/my-trace-project"`, `"projects/123456789"`.
* @param content the {@link com.google.api.services.cloudtrace.v2beta1.model.TraceSink}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.cloudtrace.v2beta1.model.TraceSink content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudTraceRequest {
private static final String REST_PATH = "v2beta1/{+parent}/traceSinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Creates a sink that exports trace spans to a destination. The export of newly-ingested traces
* begins immediately, unless the sink's `writer_identity` is not permitted to write to the
* destination. A sink can export traces only from the resource owning the sink (the 'parent').
*
* Create a request for the method "traceSinks.create".
*
* This request holds the parameters needed by the the cloudtrace server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the sink (currently only project sinks are supported):
* "projects/[PROJECT_ID]" Examples: `"projects/my-trace-project"`, `"projects/123456789"`.
* @param content the {@link com.google.api.services.cloudtrace.v2beta1.model.TraceSink}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.cloudtrace.v2beta1.model.TraceSink content) {
super(CloudTrace.this, "POST", REST_PATH, content, com.google.api.services.cloudtrace.v2beta1.model.TraceSink.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the sink (currently only project sinks are
* supported): "projects/[PROJECT_ID]" Examples: `"projects/my-trace-project"`,
* `"projects/123456789"`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the sink (currently only project sinks are supported):
"projects/[PROJECT_ID]" Examples: `"projects/my-trace-project"`, `"projects/123456789"`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the sink (currently only project sinks are
* supported): "projects/[PROJECT_ID]" Examples: `"projects/my-trace-project"`,
* `"projects/123456789"`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a sink.
*
* Create a request for the method "traceSinks.delete".
*
* This request holds the parameters needed by the cloudtrace server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudTraceRequest {
private static final String REST_PATH = "v2beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/traceSinks/[^/]+$");
/**
* Deletes a sink.
*
* Create a request for the method "traceSinks.delete".
*
* This request holds the parameters needed by the the cloudtrace server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudTrace.this, "DELETE", REST_PATH, null, com.google.api.services.cloudtrace.v2beta1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/traceSinks/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the sink to delete, including the parent resource and the sink
identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example: `"projects/12345/traceSinks
/my-sink-id"`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/traceSinks/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Get a trace sink by name under the parent resource (GCP project).
*
* Create a request for the method "traceSinks.get".
*
* This request holds the parameters needed by the cloudtrace server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the sink: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudTraceRequest {
private static final String REST_PATH = "v2beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/traceSinks/[^/]+$");
/**
* Get a trace sink by name under the parent resource (GCP project).
*
* Create a request for the method "traceSinks.get".
*
* This request holds the parameters needed by the the cloudtrace server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the sink: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudTrace.this, "GET", REST_PATH, null, com.google.api.services.cloudtrace.v2beta1.model.TraceSink.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/traceSinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]"
* Example: `"projects/12345/traceSinks/my-sink-id"`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the sink: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
`"projects/12345/traceSinks/my-sink-id"`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]"
* Example: `"projects/12345/traceSinks/my-sink-id"`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/traceSinks/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all sinks for the parent resource (GCP project).
*
* Create a request for the method "traceSinks.list".
*
* This request holds the parameters needed by the cloudtrace server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose sinks are to be listed (currently only project parent resources
* are supported): "projects/[PROJECT_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudTraceRequest {
private static final String REST_PATH = "v2beta1/{+parent}/traceSinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* List all sinks for the parent resource (GCP project).
*
* Create a request for the method "traceSinks.list".
*
* This request holds the parameters needed by the the cloudtrace server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose sinks are to be listed (currently only project parent resources
* are supported): "projects/[PROJECT_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudTrace.this, "GET", REST_PATH, null, com.google.api.services.cloudtrace.v2beta1.model.ListTraceSinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose sinks are to be listed (currently only project parent
* resources are supported): "projects/[PROJECT_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose sinks are to be listed (currently only project parent resources
are supported): "projects/[PROJECT_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose sinks are to be listed (currently only project parent
* resources are supported): "projects/[PROJECT_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of `nextPageToken` in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of `nextPageToken` in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of `nextPageToken` in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. `pageToken` must be the value of `nextPageToken` from the previous response.
* The values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. `pageToken` must be the value of `nextPageToken` from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. `pageToken` must be the value of `nextPageToken` from the previous response.
* The values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method updates fields in the existing sink according to the provided update
* mask. The sink's name cannot be changed nor any output-only fields (e.g. the writer_identity).
*
* Create a request for the method "traceSinks.patch".
*
* This request holds the parameters needed by the cloudtrace server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
* @param content the {@link com.google.api.services.cloudtrace.v2beta1.model.TraceSink}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.cloudtrace.v2beta1.model.TraceSink content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudTraceRequest {
private static final String REST_PATH = "v2beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/traceSinks/[^/]+$");
/**
* Updates a sink. This method updates fields in the existing sink according to the provided
* update mask. The sink's name cannot be changed nor any output-only fields (e.g. the
* writer_identity).
*
* Create a request for the method "traceSinks.patch".
*
* This request holds the parameters needed by the the cloudtrace server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
* @param content the {@link com.google.api.services.cloudtrace.v2beta1.model.TraceSink}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.cloudtrace.v2beta1.model.TraceSink content) {
super(CloudTrace.this, "PATCH", REST_PATH, content, com.google.api.services.cloudtrace.v2beta1.model.TraceSink.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/traceSinks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example: `"projects/12345/traceSinks
/my-sink-id"`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_NUMBER]/traceSinks/[SINK_ID]" Example:
* `"projects/12345/traceSinks/my-sink-id"`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/traceSinks/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in `trace_sink` that are to be updated. A
* sink field is overwritten if, and only if, it is in the update mask. `name` and
* `writer_identity` fields cannot be updated. An empty updateMask is considered an error.
* For a detailed `FieldMask` definition, see https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask Example: `updateMask=output_config`.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in `trace_sink` that are to be updated. A sink field
is overwritten if, and only if, it is in the update mask. `name` and `writer_identity` fields
cannot be updated. An empty updateMask is considered an error. For a detailed `FieldMask`
definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask Example: `updateMask=output_config`.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in `trace_sink` that are to be updated. A
* sink field is overwritten if, and only if, it is in the update mask. `name` and
* `writer_identity` fields cannot be updated. An empty updateMask is considered an error.
* For a detailed `FieldMask` definition, see https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask Example: `updateMask=output_config`.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link CloudTrace}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudTrace}. */
@Override
public CloudTrace build() {
return new CloudTrace(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudTraceRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudTraceRequestInitializer(
CloudTraceRequestInitializer cloudtraceRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudtraceRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}