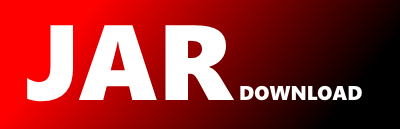
com.google.api.services.composer.v1.model.PrivateClusterConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.composer.v1.model;
/**
* Configuration options for the private GKE cluster in a Cloud Composer environment.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Composer API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PrivateClusterConfig extends com.google.api.client.json.GenericJson {
/**
* Optional. If `true`, access to the public endpoint of the GKE cluster is denied.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enablePrivateEndpoint;
/**
* Optional. The CIDR block from which IPv4 range for GKE master will be reserved. If left blank,
* the default value of '172.16.0.0/23' is used.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String masterIpv4CidrBlock;
/**
* Output only. The IP range in CIDR notation to use for the hosted master network. This range is
* used for assigning internal IP addresses to the GKE cluster master or set of masters and to the
* internal load balancer virtual IP. This range must not overlap with any other ranges in use
* within the cluster's network.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String masterIpv4ReservedRange;
/**
* Optional. If `true`, access to the public endpoint of the GKE cluster is denied.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnablePrivateEndpoint() {
return enablePrivateEndpoint;
}
/**
* Optional. If `true`, access to the public endpoint of the GKE cluster is denied.
* @param enablePrivateEndpoint enablePrivateEndpoint or {@code null} for none
*/
public PrivateClusterConfig setEnablePrivateEndpoint(java.lang.Boolean enablePrivateEndpoint) {
this.enablePrivateEndpoint = enablePrivateEndpoint;
return this;
}
/**
* Optional. The CIDR block from which IPv4 range for GKE master will be reserved. If left blank,
* the default value of '172.16.0.0/23' is used.
* @return value or {@code null} for none
*/
public java.lang.String getMasterIpv4CidrBlock() {
return masterIpv4CidrBlock;
}
/**
* Optional. The CIDR block from which IPv4 range for GKE master will be reserved. If left blank,
* the default value of '172.16.0.0/23' is used.
* @param masterIpv4CidrBlock masterIpv4CidrBlock or {@code null} for none
*/
public PrivateClusterConfig setMasterIpv4CidrBlock(java.lang.String masterIpv4CidrBlock) {
this.masterIpv4CidrBlock = masterIpv4CidrBlock;
return this;
}
/**
* Output only. The IP range in CIDR notation to use for the hosted master network. This range is
* used for assigning internal IP addresses to the GKE cluster master or set of masters and to the
* internal load balancer virtual IP. This range must not overlap with any other ranges in use
* within the cluster's network.
* @return value or {@code null} for none
*/
public java.lang.String getMasterIpv4ReservedRange() {
return masterIpv4ReservedRange;
}
/**
* Output only. The IP range in CIDR notation to use for the hosted master network. This range is
* used for assigning internal IP addresses to the GKE cluster master or set of masters and to the
* internal load balancer virtual IP. This range must not overlap with any other ranges in use
* within the cluster's network.
* @param masterIpv4ReservedRange masterIpv4ReservedRange or {@code null} for none
*/
public PrivateClusterConfig setMasterIpv4ReservedRange(java.lang.String masterIpv4ReservedRange) {
this.masterIpv4ReservedRange = masterIpv4ReservedRange;
return this;
}
@Override
public PrivateClusterConfig set(String fieldName, Object value) {
return (PrivateClusterConfig) super.set(fieldName, value);
}
@Override
public PrivateClusterConfig clone() {
return (PrivateClusterConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy