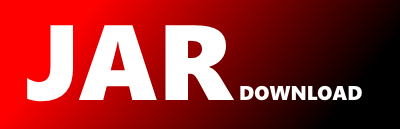
com.google.api.services.compute.model.ForwardingRule Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Represents a Forwarding Rule resource.
*
* Forwarding rule resources in GCP can be either regional or global in scope:
*
* * [Global](/compute/docs/reference/rest/{$api_version}/globalForwardingRules) *
* [Regional](/compute/docs/reference/rest/{$api_version}/forwardingRules)
*
* A forwarding rule and its corresponding IP address represent the frontend configuration of a
* Google Cloud Platform load balancer. Forwarding rules can also reference target instances and
* Cloud VPN Classic gateways (targetVpnGateway).
*
* For more information, read Forwarding rule concepts and Using protocol forwarding.
*
* (== resource_for {$api_version}.forwardingRules ==) (== resource_for
* {$api_version}.globalForwardingRules ==) (== resource_for {$api_version}.regionForwardingRules
* ==)
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ForwardingRule extends com.google.api.client.json.GenericJson {
/**
* IP address that this forwarding rule serves. When a client sends traffic to this IP address,
* the forwarding rule directs the traffic to the target that you specify in the forwarding rule.
*
* If you don't specify a reserved IP address, an ephemeral IP address is assigned. Methods for
* specifying an IP address:
*
* * IPv4 dotted decimal, as in `100.1.2.3` * Full URL, as in
* https://www.googleapis.com/compute/v1/projects/project_id/regions/region/addresses/address-name
* * Partial URL or by name, as in: * projects/project_id/regions/region/addresses/address-name *
* regions/region/addresses/address-name * global/addresses/address-name * address-name
*
* The loadBalancingScheme and the forwarding rule's target determine the type of IP address that
* you can use. For detailed information, refer to [IP address specifications](/load-
* balancing/docs/forwarding-rule-concepts#ip_address_specifications).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("IPAddress")
private java.lang.String iPAddress;
/**
* The IP protocol to which this rule applies. For protocol forwarding, valid options are TCP,
* UDP, ESP, AH, SCTP or ICMP.
*
* For Internal TCP/UDP Load Balancing, the load balancing scheme is INTERNAL, and one of TCP or
* UDP are valid. For Traffic Director, the load balancing scheme is INTERNAL_SELF_MANAGED, and
* only TCPis valid. For Internal HTTP(S) Load Balancing, the load balancing scheme is
* INTERNAL_MANAGED, and only TCP is valid. For HTTP(S), SSL Proxy, and TCP Proxy Load Balancing,
* the load balancing scheme is EXTERNAL and only TCP is valid. For Network TCP/UDP Load
* Balancing, the load balancing scheme is EXTERNAL, and one of TCP or UDP is valid.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("IPProtocol")
private java.lang.String iPProtocol;
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. This field cannot be used with port or portRange
* fields.
*
* When the load balancing scheme is INTERNAL and protocol is TCP/UDP, specify this field to allow
* packets addressed to any ports will be forwarded to the backends configured with this
* forwarding rule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allPorts;
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. If the field is set to TRUE, clients can access ILB
* from all regions. Otherwise only allows access from clients in the same region as the internal
* load balancer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowGlobalAccess;
/**
* This field is only used for INTERNAL load balancing.
*
* For internal load balancing, this field identifies the BackendService resource to receive the
* matched traffic.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String backendService;
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creationTimestamp;
/**
* An optional description of this resource. Provide this property when you create the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fingerprint;
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger id;
/**
* The IP Version that will be used by this forwarding rule. Valid options are IPV4 or IPV6. This
* can only be specified for an external global forwarding rule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ipVersion;
/**
* Indicates whether or not this load balancer can be used as a collector for packet mirroring. To
* prevent mirroring loops, instances behind this load balancer will not have their traffic
* mirrored even if a PacketMirroring rule applies to them. This can only be set to true for load
* balancers that have their loadBalancingScheme set to INTERNAL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isMirroringCollector;
/**
* [Output Only] Type of the resource. Always compute#forwardingRule for Forwarding Rule
* resources.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String labelFingerprint;
/**
* Labels for this resource. These can only be added or modified by the setLabels method. Each
* label key/value pair must comply with RFC1035. Label values may be empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Specifies the forwarding rule type.
*
* - EXTERNAL is used for: - Classic Cloud VPN gateways - Protocol forwarding to VMs from an
* external IP address - The following load balancers: HTTP(S), SSL Proxy, TCP Proxy, and Network
* TCP/UDP - INTERNAL is used for: - Protocol forwarding to VMs from an internal IP address
* - Internal TCP/UDP load balancers - INTERNAL_MANAGED is used for: - Internal HTTP(S) load
* balancers - INTERNAL_SELF_MANAGED is used for: - Traffic Director
*
* For more information about forwarding rules, refer to Forwarding rule concepts.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String loadBalancingScheme;
/**
* Opaque filter criteria used by Loadbalancer to restrict routing configuration to a limited set
* of xDS compliant clients. In their xDS requests to Loadbalancer, xDS clients present node
* metadata. If a match takes place, the relevant configuration is made available to those
* proxies. Otherwise, all the resources (e.g. TargetHttpProxy, UrlMap) referenced by the
* ForwardingRule will not be visible to those proxies. For each metadataFilter in this list, if
* its filterMatchCriteria is set to MATCH_ANY, at least one of the filterLabels must match the
* corresponding label provided in the metadata. If its filterMatchCriteria is set to MATCH_ALL,
* then all of its filterLabels must match with corresponding labels provided in the metadata.
* metadataFilters specified here will be applifed before those specified in the UrlMap that this
* ForwardingRule references. metadataFilters only applies to Loadbalancers that have their
* loadBalancingScheme set to INTERNAL_SELF_MANAGED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List metadataFilters;
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* This field is not used for external load balancing.
*
* For INTERNAL and INTERNAL_SELF_MANAGED load balancing, this field identifies the network that
* the load balanced IP should belong to for this Forwarding Rule. If this field is not specified,
* the default network will be used.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String network;
/**
* This signifies the networking tier used for configuring this load balancer and can only take
* the following values: PREMIUM, STANDARD.
*
* For regional ForwardingRule, the valid values are PREMIUM and STANDARD. For
* GlobalForwardingRule, the valid value is PREMIUM.
*
* If this field is not specified, it is assumed to be PREMIUM. If IPAddress is specified, this
* value must be equal to the networkTier of the Address.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String networkTier;
/**
* When the load balancing scheme is EXTERNAL, INTERNAL_SELF_MANAGED and INTERNAL_MANAGED, you can
* specify a port_range. Use with a forwarding rule that points to a target proxy or a target
* pool. Do not use with a forwarding rule that points to a backend service. This field is used
* along with the target field for TargetHttpProxy, TargetHttpsProxy, TargetSslProxy,
* TargetTcpProxy, TargetVpnGateway, TargetPool, TargetInstance.
*
* Applicable only when IPProtocol is TCP, UDP, or SCTP, only packets addressed to ports in the
* specified range will be forwarded to target. Forwarding rules with the same [IPAddress,
* IPProtocol] pair must have disjoint port ranges.
*
* Some types of forwarding target have constraints on the acceptable ports: - TargetHttpProxy:
* 80, 8080 - TargetHttpsProxy: 443 - TargetTcpProxy: 25, 43, 110, 143, 195, 443, 465, 587, 700,
* 993, 995, 1688, 1883, 5222 - TargetSslProxy: 25, 43, 110, 143, 195, 443, 465, 587, 700, 993,
* 995, 1688, 1883, 5222 - TargetVpnGateway: 500, 4500
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String portRange;
/**
* This field is used along with the backend_service field for internal load balancing.
*
* When the load balancing scheme is INTERNAL, a list of ports can be configured, for example,
* ['80'], ['8000','9000']. Only packets addressed to these ports are forwarded to the backends
* configured with the forwarding rule.
*
* If the forwarding rule's loadBalancingScheme is INTERNAL, you can specify ports in one of the
* following ways:
*
* * A list of up to five ports, which can be non-contiguous * Keyword ALL, which causes the
* forwarding rule to forward traffic on any port of the forwarding rule's protocol.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List ports;
/**
* [Output Only] URL of the region where the regional forwarding rule resides. This field is not
* applicable to global forwarding rules. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String region;
/**
* [Output Only] Server-defined URL for the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* [Output Only] Server-defined URL for this resource with the resource id.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLinkWithId;
/**
* An optional prefix to the service name for this Forwarding Rule. If specified, the prefix is
* the first label of the fully qualified service name.
*
* The label must be 1-63 characters long, and comply with RFC1035. Specifically, the label must
* be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which
* means the first character must be a lowercase letter, and all following characters must be a
* dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
* This field is only used for internal load balancing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceLabel;
/**
* [Output Only] The internal fully qualified service name for this Forwarding Rule.
*
* This field is only used for internal load balancing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceName;
/**
* This field is only used for INTERNAL load balancing.
*
* For internal load balancing, this field identifies the subnetwork that the load balanced IP
* should belong to for this Forwarding Rule.
*
* If the network specified is in auto subnet mode, this field is optional. However, if the
* network is in custom subnet mode, a subnetwork must be specified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String subnetwork;
/**
* The URL of the target resource to receive the matched traffic. For regional forwarding rules,
* this target must live in the same region as the forwarding rule. For global forwarding rules,
* this target must be a global load balancing resource. The forwarded traffic must be of a type
* appropriate to the target object. For INTERNAL_SELF_MANAGED load balancing, only
* targetHttpProxy is valid, not targetHttpsProxy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String target;
/**
* IP address that this forwarding rule serves. When a client sends traffic to this IP address,
* the forwarding rule directs the traffic to the target that you specify in the forwarding rule.
*
* If you don't specify a reserved IP address, an ephemeral IP address is assigned. Methods for
* specifying an IP address:
*
* * IPv4 dotted decimal, as in `100.1.2.3` * Full URL, as in
* https://www.googleapis.com/compute/v1/projects/project_id/regions/region/addresses/address-name
* * Partial URL or by name, as in: * projects/project_id/regions/region/addresses/address-name *
* regions/region/addresses/address-name * global/addresses/address-name * address-name
*
* The loadBalancingScheme and the forwarding rule's target determine the type of IP address that
* you can use. For detailed information, refer to [IP address specifications](/load-
* balancing/docs/forwarding-rule-concepts#ip_address_specifications).
* @return value or {@code null} for none
*/
public java.lang.String getIPAddress() {
return iPAddress;
}
/**
* IP address that this forwarding rule serves. When a client sends traffic to this IP address,
* the forwarding rule directs the traffic to the target that you specify in the forwarding rule.
*
* If you don't specify a reserved IP address, an ephemeral IP address is assigned. Methods for
* specifying an IP address:
*
* * IPv4 dotted decimal, as in `100.1.2.3` * Full URL, as in
* https://www.googleapis.com/compute/v1/projects/project_id/regions/region/addresses/address-name
* * Partial URL or by name, as in: * projects/project_id/regions/region/addresses/address-name *
* regions/region/addresses/address-name * global/addresses/address-name * address-name
*
* The loadBalancingScheme and the forwarding rule's target determine the type of IP address that
* you can use. For detailed information, refer to [IP address specifications](/load-
* balancing/docs/forwarding-rule-concepts#ip_address_specifications).
* @param iPAddress iPAddress or {@code null} for none
*/
public ForwardingRule setIPAddress(java.lang.String iPAddress) {
this.iPAddress = iPAddress;
return this;
}
/**
* The IP protocol to which this rule applies. For protocol forwarding, valid options are TCP,
* UDP, ESP, AH, SCTP or ICMP.
*
* For Internal TCP/UDP Load Balancing, the load balancing scheme is INTERNAL, and one of TCP or
* UDP are valid. For Traffic Director, the load balancing scheme is INTERNAL_SELF_MANAGED, and
* only TCPis valid. For Internal HTTP(S) Load Balancing, the load balancing scheme is
* INTERNAL_MANAGED, and only TCP is valid. For HTTP(S), SSL Proxy, and TCP Proxy Load Balancing,
* the load balancing scheme is EXTERNAL and only TCP is valid. For Network TCP/UDP Load
* Balancing, the load balancing scheme is EXTERNAL, and one of TCP or UDP is valid.
* @return value or {@code null} for none
*/
public java.lang.String getIPProtocol() {
return iPProtocol;
}
/**
* The IP protocol to which this rule applies. For protocol forwarding, valid options are TCP,
* UDP, ESP, AH, SCTP or ICMP.
*
* For Internal TCP/UDP Load Balancing, the load balancing scheme is INTERNAL, and one of TCP or
* UDP are valid. For Traffic Director, the load balancing scheme is INTERNAL_SELF_MANAGED, and
* only TCPis valid. For Internal HTTP(S) Load Balancing, the load balancing scheme is
* INTERNAL_MANAGED, and only TCP is valid. For HTTP(S), SSL Proxy, and TCP Proxy Load Balancing,
* the load balancing scheme is EXTERNAL and only TCP is valid. For Network TCP/UDP Load
* Balancing, the load balancing scheme is EXTERNAL, and one of TCP or UDP is valid.
* @param iPProtocol iPProtocol or {@code null} for none
*/
public ForwardingRule setIPProtocol(java.lang.String iPProtocol) {
this.iPProtocol = iPProtocol;
return this;
}
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. This field cannot be used with port or portRange
* fields.
*
* When the load balancing scheme is INTERNAL and protocol is TCP/UDP, specify this field to allow
* packets addressed to any ports will be forwarded to the backends configured with this
* forwarding rule.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllPorts() {
return allPorts;
}
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. This field cannot be used with port or portRange
* fields.
*
* When the load balancing scheme is INTERNAL and protocol is TCP/UDP, specify this field to allow
* packets addressed to any ports will be forwarded to the backends configured with this
* forwarding rule.
* @param allPorts allPorts or {@code null} for none
*/
public ForwardingRule setAllPorts(java.lang.Boolean allPorts) {
this.allPorts = allPorts;
return this;
}
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. If the field is set to TRUE, clients can access ILB
* from all regions. Otherwise only allows access from clients in the same region as the internal
* load balancer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllowGlobalAccess() {
return allowGlobalAccess;
}
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. If the field is set to TRUE, clients can access ILB
* from all regions. Otherwise only allows access from clients in the same region as the internal
* load balancer.
* @param allowGlobalAccess allowGlobalAccess or {@code null} for none
*/
public ForwardingRule setAllowGlobalAccess(java.lang.Boolean allowGlobalAccess) {
this.allowGlobalAccess = allowGlobalAccess;
return this;
}
/**
* This field is only used for INTERNAL load balancing.
*
* For internal load balancing, this field identifies the BackendService resource to receive the
* matched traffic.
* @return value or {@code null} for none
*/
public java.lang.String getBackendService() {
return backendService;
}
/**
* This field is only used for INTERNAL load balancing.
*
* For internal load balancing, this field identifies the BackendService resource to receive the
* matched traffic.
* @param backendService backendService or {@code null} for none
*/
public ForwardingRule setBackendService(java.lang.String backendService) {
this.backendService = backendService;
return this;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @return value or {@code null} for none
*/
public java.lang.String getCreationTimestamp() {
return creationTimestamp;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public ForwardingRule setCreationTimestamp(java.lang.String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @param description description or {@code null} for none
*/
public ForwardingRule setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #decodeFingerprint()
* @return value or {@code null} for none
*/
public java.lang.String getFingerprint() {
return fingerprint;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #getFingerprint()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeFingerprint() {
return com.google.api.client.util.Base64.decodeBase64(fingerprint);
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #encodeFingerprint()
* @param fingerprint fingerprint or {@code null} for none
*/
public ForwardingRule setFingerprint(java.lang.String fingerprint) {
this.fingerprint = fingerprint;
return this;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #setFingerprint()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public ForwardingRule encodeFingerprint(byte[] fingerprint) {
this.fingerprint = com.google.api.client.util.Base64.encodeBase64URLSafeString(fingerprint);
return this;
}
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* @return value or {@code null} for none
*/
public java.math.BigInteger getId() {
return id;
}
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* @param id id or {@code null} for none
*/
public ForwardingRule setId(java.math.BigInteger id) {
this.id = id;
return this;
}
/**
* The IP Version that will be used by this forwarding rule. Valid options are IPV4 or IPV6. This
* can only be specified for an external global forwarding rule.
* @return value or {@code null} for none
*/
public java.lang.String getIpVersion() {
return ipVersion;
}
/**
* The IP Version that will be used by this forwarding rule. Valid options are IPV4 or IPV6. This
* can only be specified for an external global forwarding rule.
* @param ipVersion ipVersion or {@code null} for none
*/
public ForwardingRule setIpVersion(java.lang.String ipVersion) {
this.ipVersion = ipVersion;
return this;
}
/**
* Indicates whether or not this load balancer can be used as a collector for packet mirroring. To
* prevent mirroring loops, instances behind this load balancer will not have their traffic
* mirrored even if a PacketMirroring rule applies to them. This can only be set to true for load
* balancers that have their loadBalancingScheme set to INTERNAL.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsMirroringCollector() {
return isMirroringCollector;
}
/**
* Indicates whether or not this load balancer can be used as a collector for packet mirroring. To
* prevent mirroring loops, instances behind this load balancer will not have their traffic
* mirrored even if a PacketMirroring rule applies to them. This can only be set to true for load
* balancers that have their loadBalancingScheme set to INTERNAL.
* @param isMirroringCollector isMirroringCollector or {@code null} for none
*/
public ForwardingRule setIsMirroringCollector(java.lang.Boolean isMirroringCollector) {
this.isMirroringCollector = isMirroringCollector;
return this;
}
/**
* [Output Only] Type of the resource. Always compute#forwardingRule for Forwarding Rule
* resources.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* [Output Only] Type of the resource. Always compute#forwardingRule for Forwarding Rule
* resources.
* @param kind kind or {@code null} for none
*/
public ForwardingRule setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #decodeLabelFingerprint()
* @return value or {@code null} for none
*/
public java.lang.String getLabelFingerprint() {
return labelFingerprint;
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #getLabelFingerprint()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeLabelFingerprint() {
return com.google.api.client.util.Base64.decodeBase64(labelFingerprint);
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #encodeLabelFingerprint()
* @param labelFingerprint labelFingerprint or {@code null} for none
*/
public ForwardingRule setLabelFingerprint(java.lang.String labelFingerprint) {
this.labelFingerprint = labelFingerprint;
return this;
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet.
*
* To see the latest fingerprint, make a get() request to retrieve a ForwardingRule.
* @see #setLabelFingerprint()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public ForwardingRule encodeLabelFingerprint(byte[] labelFingerprint) {
this.labelFingerprint = com.google.api.client.util.Base64.encodeBase64URLSafeString(labelFingerprint);
return this;
}
/**
* Labels for this resource. These can only be added or modified by the setLabels method. Each
* label key/value pair must comply with RFC1035. Label values may be empty.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Labels for this resource. These can only be added or modified by the setLabels method. Each
* label key/value pair must comply with RFC1035. Label values may be empty.
* @param labels labels or {@code null} for none
*/
public ForwardingRule setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Specifies the forwarding rule type.
*
* - EXTERNAL is used for: - Classic Cloud VPN gateways - Protocol forwarding to VMs from an
* external IP address - The following load balancers: HTTP(S), SSL Proxy, TCP Proxy, and Network
* TCP/UDP - INTERNAL is used for: - Protocol forwarding to VMs from an internal IP address
* - Internal TCP/UDP load balancers - INTERNAL_MANAGED is used for: - Internal HTTP(S) load
* balancers - INTERNAL_SELF_MANAGED is used for: - Traffic Director
*
* For more information about forwarding rules, refer to Forwarding rule concepts.
* @return value or {@code null} for none
*/
public java.lang.String getLoadBalancingScheme() {
return loadBalancingScheme;
}
/**
* Specifies the forwarding rule type.
*
* - EXTERNAL is used for: - Classic Cloud VPN gateways - Protocol forwarding to VMs from an
* external IP address - The following load balancers: HTTP(S), SSL Proxy, TCP Proxy, and Network
* TCP/UDP - INTERNAL is used for: - Protocol forwarding to VMs from an internal IP address
* - Internal TCP/UDP load balancers - INTERNAL_MANAGED is used for: - Internal HTTP(S) load
* balancers - INTERNAL_SELF_MANAGED is used for: - Traffic Director
*
* For more information about forwarding rules, refer to Forwarding rule concepts.
* @param loadBalancingScheme loadBalancingScheme or {@code null} for none
*/
public ForwardingRule setLoadBalancingScheme(java.lang.String loadBalancingScheme) {
this.loadBalancingScheme = loadBalancingScheme;
return this;
}
/**
* Opaque filter criteria used by Loadbalancer to restrict routing configuration to a limited set
* of xDS compliant clients. In their xDS requests to Loadbalancer, xDS clients present node
* metadata. If a match takes place, the relevant configuration is made available to those
* proxies. Otherwise, all the resources (e.g. TargetHttpProxy, UrlMap) referenced by the
* ForwardingRule will not be visible to those proxies. For each metadataFilter in this list, if
* its filterMatchCriteria is set to MATCH_ANY, at least one of the filterLabels must match the
* corresponding label provided in the metadata. If its filterMatchCriteria is set to MATCH_ALL,
* then all of its filterLabels must match with corresponding labels provided in the metadata.
* metadataFilters specified here will be applifed before those specified in the UrlMap that this
* ForwardingRule references. metadataFilters only applies to Loadbalancers that have their
* loadBalancingScheme set to INTERNAL_SELF_MANAGED.
* @return value or {@code null} for none
*/
public java.util.List getMetadataFilters() {
return metadataFilters;
}
/**
* Opaque filter criteria used by Loadbalancer to restrict routing configuration to a limited set
* of xDS compliant clients. In their xDS requests to Loadbalancer, xDS clients present node
* metadata. If a match takes place, the relevant configuration is made available to those
* proxies. Otherwise, all the resources (e.g. TargetHttpProxy, UrlMap) referenced by the
* ForwardingRule will not be visible to those proxies. For each metadataFilter in this list, if
* its filterMatchCriteria is set to MATCH_ANY, at least one of the filterLabels must match the
* corresponding label provided in the metadata. If its filterMatchCriteria is set to MATCH_ALL,
* then all of its filterLabels must match with corresponding labels provided in the metadata.
* metadataFilters specified here will be applifed before those specified in the UrlMap that this
* ForwardingRule references. metadataFilters only applies to Loadbalancers that have their
* loadBalancingScheme set to INTERNAL_SELF_MANAGED.
* @param metadataFilters metadataFilters or {@code null} for none
*/
public ForwardingRule setMetadataFilters(java.util.List metadataFilters) {
this.metadataFilters = metadataFilters;
return this;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* @param name name or {@code null} for none
*/
public ForwardingRule setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* This field is not used for external load balancing.
*
* For INTERNAL and INTERNAL_SELF_MANAGED load balancing, this field identifies the network that
* the load balanced IP should belong to for this Forwarding Rule. If this field is not specified,
* the default network will be used.
* @return value or {@code null} for none
*/
public java.lang.String getNetwork() {
return network;
}
/**
* This field is not used for external load balancing.
*
* For INTERNAL and INTERNAL_SELF_MANAGED load balancing, this field identifies the network that
* the load balanced IP should belong to for this Forwarding Rule. If this field is not specified,
* the default network will be used.
* @param network network or {@code null} for none
*/
public ForwardingRule setNetwork(java.lang.String network) {
this.network = network;
return this;
}
/**
* This signifies the networking tier used for configuring this load balancer and can only take
* the following values: PREMIUM, STANDARD.
*
* For regional ForwardingRule, the valid values are PREMIUM and STANDARD. For
* GlobalForwardingRule, the valid value is PREMIUM.
*
* If this field is not specified, it is assumed to be PREMIUM. If IPAddress is specified, this
* value must be equal to the networkTier of the Address.
* @return value or {@code null} for none
*/
public java.lang.String getNetworkTier() {
return networkTier;
}
/**
* This signifies the networking tier used for configuring this load balancer and can only take
* the following values: PREMIUM, STANDARD.
*
* For regional ForwardingRule, the valid values are PREMIUM and STANDARD. For
* GlobalForwardingRule, the valid value is PREMIUM.
*
* If this field is not specified, it is assumed to be PREMIUM. If IPAddress is specified, this
* value must be equal to the networkTier of the Address.
* @param networkTier networkTier or {@code null} for none
*/
public ForwardingRule setNetworkTier(java.lang.String networkTier) {
this.networkTier = networkTier;
return this;
}
/**
* When the load balancing scheme is EXTERNAL, INTERNAL_SELF_MANAGED and INTERNAL_MANAGED, you can
* specify a port_range. Use with a forwarding rule that points to a target proxy or a target
* pool. Do not use with a forwarding rule that points to a backend service. This field is used
* along with the target field for TargetHttpProxy, TargetHttpsProxy, TargetSslProxy,
* TargetTcpProxy, TargetVpnGateway, TargetPool, TargetInstance.
*
* Applicable only when IPProtocol is TCP, UDP, or SCTP, only packets addressed to ports in the
* specified range will be forwarded to target. Forwarding rules with the same [IPAddress,
* IPProtocol] pair must have disjoint port ranges.
*
* Some types of forwarding target have constraints on the acceptable ports: - TargetHttpProxy:
* 80, 8080 - TargetHttpsProxy: 443 - TargetTcpProxy: 25, 43, 110, 143, 195, 443, 465, 587, 700,
* 993, 995, 1688, 1883, 5222 - TargetSslProxy: 25, 43, 110, 143, 195, 443, 465, 587, 700, 993,
* 995, 1688, 1883, 5222 - TargetVpnGateway: 500, 4500
* @return value or {@code null} for none
*/
public java.lang.String getPortRange() {
return portRange;
}
/**
* When the load balancing scheme is EXTERNAL, INTERNAL_SELF_MANAGED and INTERNAL_MANAGED, you can
* specify a port_range. Use with a forwarding rule that points to a target proxy or a target
* pool. Do not use with a forwarding rule that points to a backend service. This field is used
* along with the target field for TargetHttpProxy, TargetHttpsProxy, TargetSslProxy,
* TargetTcpProxy, TargetVpnGateway, TargetPool, TargetInstance.
*
* Applicable only when IPProtocol is TCP, UDP, or SCTP, only packets addressed to ports in the
* specified range will be forwarded to target. Forwarding rules with the same [IPAddress,
* IPProtocol] pair must have disjoint port ranges.
*
* Some types of forwarding target have constraints on the acceptable ports: - TargetHttpProxy:
* 80, 8080 - TargetHttpsProxy: 443 - TargetTcpProxy: 25, 43, 110, 143, 195, 443, 465, 587, 700,
* 993, 995, 1688, 1883, 5222 - TargetSslProxy: 25, 43, 110, 143, 195, 443, 465, 587, 700, 993,
* 995, 1688, 1883, 5222 - TargetVpnGateway: 500, 4500
* @param portRange portRange or {@code null} for none
*/
public ForwardingRule setPortRange(java.lang.String portRange) {
this.portRange = portRange;
return this;
}
/**
* This field is used along with the backend_service field for internal load balancing.
*
* When the load balancing scheme is INTERNAL, a list of ports can be configured, for example,
* ['80'], ['8000','9000']. Only packets addressed to these ports are forwarded to the backends
* configured with the forwarding rule.
*
* If the forwarding rule's loadBalancingScheme is INTERNAL, you can specify ports in one of the
* following ways:
*
* * A list of up to five ports, which can be non-contiguous * Keyword ALL, which causes the
* forwarding rule to forward traffic on any port of the forwarding rule's protocol.
* @return value or {@code null} for none
*/
public java.util.List getPorts() {
return ports;
}
/**
* This field is used along with the backend_service field for internal load balancing.
*
* When the load balancing scheme is INTERNAL, a list of ports can be configured, for example,
* ['80'], ['8000','9000']. Only packets addressed to these ports are forwarded to the backends
* configured with the forwarding rule.
*
* If the forwarding rule's loadBalancingScheme is INTERNAL, you can specify ports in one of the
* following ways:
*
* * A list of up to five ports, which can be non-contiguous * Keyword ALL, which causes the
* forwarding rule to forward traffic on any port of the forwarding rule's protocol.
* @param ports ports or {@code null} for none
*/
public ForwardingRule setPorts(java.util.List ports) {
this.ports = ports;
return this;
}
/**
* [Output Only] URL of the region where the regional forwarding rule resides. This field is not
* applicable to global forwarding rules. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* @return value or {@code null} for none
*/
public java.lang.String getRegion() {
return region;
}
/**
* [Output Only] URL of the region where the regional forwarding rule resides. This field is not
* applicable to global forwarding rules. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* @param region region or {@code null} for none
*/
public ForwardingRule setRegion(java.lang.String region) {
this.region = region;
return this;
}
/**
* [Output Only] Server-defined URL for the resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* [Output Only] Server-defined URL for the resource.
* @param selfLink selfLink or {@code null} for none
*/
public ForwardingRule setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* [Output Only] Server-defined URL for this resource with the resource id.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLinkWithId() {
return selfLinkWithId;
}
/**
* [Output Only] Server-defined URL for this resource with the resource id.
* @param selfLinkWithId selfLinkWithId or {@code null} for none
*/
public ForwardingRule setSelfLinkWithId(java.lang.String selfLinkWithId) {
this.selfLinkWithId = selfLinkWithId;
return this;
}
/**
* An optional prefix to the service name for this Forwarding Rule. If specified, the prefix is
* the first label of the fully qualified service name.
*
* The label must be 1-63 characters long, and comply with RFC1035. Specifically, the label must
* be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which
* means the first character must be a lowercase letter, and all following characters must be a
* dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
* This field is only used for internal load balancing.
* @return value or {@code null} for none
*/
public java.lang.String getServiceLabel() {
return serviceLabel;
}
/**
* An optional prefix to the service name for this Forwarding Rule. If specified, the prefix is
* the first label of the fully qualified service name.
*
* The label must be 1-63 characters long, and comply with RFC1035. Specifically, the label must
* be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which
* means the first character must be a lowercase letter, and all following characters must be a
* dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
* This field is only used for internal load balancing.
* @param serviceLabel serviceLabel or {@code null} for none
*/
public ForwardingRule setServiceLabel(java.lang.String serviceLabel) {
this.serviceLabel = serviceLabel;
return this;
}
/**
* [Output Only] The internal fully qualified service name for this Forwarding Rule.
*
* This field is only used for internal load balancing.
* @return value or {@code null} for none
*/
public java.lang.String getServiceName() {
return serviceName;
}
/**
* [Output Only] The internal fully qualified service name for this Forwarding Rule.
*
* This field is only used for internal load balancing.
* @param serviceName serviceName or {@code null} for none
*/
public ForwardingRule setServiceName(java.lang.String serviceName) {
this.serviceName = serviceName;
return this;
}
/**
* This field is only used for INTERNAL load balancing.
*
* For internal load balancing, this field identifies the subnetwork that the load balanced IP
* should belong to for this Forwarding Rule.
*
* If the network specified is in auto subnet mode, this field is optional. However, if the
* network is in custom subnet mode, a subnetwork must be specified.
* @return value or {@code null} for none
*/
public java.lang.String getSubnetwork() {
return subnetwork;
}
/**
* This field is only used for INTERNAL load balancing.
*
* For internal load balancing, this field identifies the subnetwork that the load balanced IP
* should belong to for this Forwarding Rule.
*
* If the network specified is in auto subnet mode, this field is optional. However, if the
* network is in custom subnet mode, a subnetwork must be specified.
* @param subnetwork subnetwork or {@code null} for none
*/
public ForwardingRule setSubnetwork(java.lang.String subnetwork) {
this.subnetwork = subnetwork;
return this;
}
/**
* The URL of the target resource to receive the matched traffic. For regional forwarding rules,
* this target must live in the same region as the forwarding rule. For global forwarding rules,
* this target must be a global load balancing resource. The forwarded traffic must be of a type
* appropriate to the target object. For INTERNAL_SELF_MANAGED load balancing, only
* targetHttpProxy is valid, not targetHttpsProxy.
* @return value or {@code null} for none
*/
public java.lang.String getTarget() {
return target;
}
/**
* The URL of the target resource to receive the matched traffic. For regional forwarding rules,
* this target must live in the same region as the forwarding rule. For global forwarding rules,
* this target must be a global load balancing resource. The forwarded traffic must be of a type
* appropriate to the target object. For INTERNAL_SELF_MANAGED load balancing, only
* targetHttpProxy is valid, not targetHttpsProxy.
* @param target target or {@code null} for none
*/
public ForwardingRule setTarget(java.lang.String target) {
this.target = target;
return this;
}
@Override
public ForwardingRule set(String fieldName, Object value) {
return (ForwardingRule) super.set(fieldName, value);
}
@Override
public ForwardingRule clone() {
return (ForwardingRule) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy