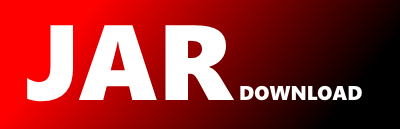
com.google.api.services.compute.model.HttpRouteRule Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* An HttpRouteRule specifies how to match an HTTP request and the corresponding routing action that
* load balancing proxies will perform.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class HttpRouteRule extends com.google.api.client.json.GenericJson {
/**
* The short description conveying the intent of this routeRule. The description can have a
* maximum length of 1024 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Specifies changes to request and response headers that need to take effect for the selected
* backendService. The headerAction specified here are applied before the matching
* pathMatchers[].headerAction and after pathMatchers[].routeRules[].routeAction.weightedBackendSe
* rvice.backendServiceWeightAction[].headerAction
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private HttpHeaderAction headerAction;
/**
* Outbound route specific configuration for networkservices.HttpFilter resources enabled by
* Traffic Director. httpFilterConfigs only applies for Loadbalancers with loadBalancingScheme set
* to INTERNAL_SELF_MANAGED. See ForwardingRule for more details.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List httpFilterConfigs;
static {
// hack to force ProGuard to consider HttpFilterConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(HttpFilterConfig.class);
}
/**
* Outbound route specific metadata supplied to networkservices.HttpFilter resources enabled by
* Traffic Director. httpFilterMetadata only applies for Loadbalancers with loadBalancingScheme
* set to INTERNAL_SELF_MANAGED. See ForwardingRule for more details. The only configTypeUrl
* supported is type.googleapis.com/google.protobuf.Struct
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List httpFilterMetadata;
static {
// hack to force ProGuard to consider HttpFilterConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(HttpFilterConfig.class);
}
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List matchRules;
/**
* For routeRules within a given pathMatcher, priority determines the order in which load balancer
* will interpret routeRules. RouteRules are evaluated in order of priority, from the lowest to
* highest number. The priority of a rule decreases as its number increases (1, 2, 3, N+1). The
* first rule that matches the request is applied. You cannot configure two or more routeRules
* with the same priority. Priority for each rule must be set to a number between 0 and 2147483647
* inclusive. Priority numbers can have gaps, which enable you to add or remove rules in the
* future without affecting the rest of the rules. For example, 1, 2, 3, 4, 5, 9, 12, 16 is a
* valid series of priority numbers to which you could add rules numbered from 6 to 8, 10 to 11,
* and 13 to 15 in the future without any impact on existing rules.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer priority;
/**
* In response to a matching matchRule, the load balancer performs advanced routing actions like
* URL rewrites, header transformations, etc. prior to forwarding the request to the selected
* backend. If routeAction specifies any weightedBackendServices, service must not be set.
* Conversely if service is set, routeAction cannot contain any weightedBackendServices. Only one
* of urlRedirect, service or routeAction.weightedBackendService must be set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private HttpRouteAction routeAction;
/**
* The full or partial URL of the backend service resource to which traffic is directed if this
* rule is matched. If routeAction is additionally specified, advanced routing actions like URL
* Rewrites, etc. take effect prior to sending the request to the backend. However, if service is
* specified, routeAction cannot contain any weightedBackendService s. Conversely, if routeAction
* specifies any weightedBackendServices, service must not be specified. Only one of urlRedirect,
* service or routeAction.weightedBackendService must be set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String service;
/**
* When this rule is matched, the request is redirected to a URL specified by urlRedirect. If
* urlRedirect is specified, service or routeAction must not be set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private HttpRedirectAction urlRedirect;
/**
* The short description conveying the intent of this routeRule. The description can have a
* maximum length of 1024 characters.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The short description conveying the intent of this routeRule. The description can have a
* maximum length of 1024 characters.
* @param description description or {@code null} for none
*/
public HttpRouteRule setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Specifies changes to request and response headers that need to take effect for the selected
* backendService. The headerAction specified here are applied before the matching
* pathMatchers[].headerAction and after pathMatchers[].routeRules[].routeAction.weightedBackendSe
* rvice.backendServiceWeightAction[].headerAction
* @return value or {@code null} for none
*/
public HttpHeaderAction getHeaderAction() {
return headerAction;
}
/**
* Specifies changes to request and response headers that need to take effect for the selected
* backendService. The headerAction specified here are applied before the matching
* pathMatchers[].headerAction and after pathMatchers[].routeRules[].routeAction.weightedBackendSe
* rvice.backendServiceWeightAction[].headerAction
* @param headerAction headerAction or {@code null} for none
*/
public HttpRouteRule setHeaderAction(HttpHeaderAction headerAction) {
this.headerAction = headerAction;
return this;
}
/**
* Outbound route specific configuration for networkservices.HttpFilter resources enabled by
* Traffic Director. httpFilterConfigs only applies for Loadbalancers with loadBalancingScheme set
* to INTERNAL_SELF_MANAGED. See ForwardingRule for more details.
* @return value or {@code null} for none
*/
public java.util.List getHttpFilterConfigs() {
return httpFilterConfigs;
}
/**
* Outbound route specific configuration for networkservices.HttpFilter resources enabled by
* Traffic Director. httpFilterConfigs only applies for Loadbalancers with loadBalancingScheme set
* to INTERNAL_SELF_MANAGED. See ForwardingRule for more details.
* @param httpFilterConfigs httpFilterConfigs or {@code null} for none
*/
public HttpRouteRule setHttpFilterConfigs(java.util.List httpFilterConfigs) {
this.httpFilterConfigs = httpFilterConfigs;
return this;
}
/**
* Outbound route specific metadata supplied to networkservices.HttpFilter resources enabled by
* Traffic Director. httpFilterMetadata only applies for Loadbalancers with loadBalancingScheme
* set to INTERNAL_SELF_MANAGED. See ForwardingRule for more details. The only configTypeUrl
* supported is type.googleapis.com/google.protobuf.Struct
* @return value or {@code null} for none
*/
public java.util.List getHttpFilterMetadata() {
return httpFilterMetadata;
}
/**
* Outbound route specific metadata supplied to networkservices.HttpFilter resources enabled by
* Traffic Director. httpFilterMetadata only applies for Loadbalancers with loadBalancingScheme
* set to INTERNAL_SELF_MANAGED. See ForwardingRule for more details. The only configTypeUrl
* supported is type.googleapis.com/google.protobuf.Struct
* @param httpFilterMetadata httpFilterMetadata or {@code null} for none
*/
public HttpRouteRule setHttpFilterMetadata(java.util.List httpFilterMetadata) {
this.httpFilterMetadata = httpFilterMetadata;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getMatchRules() {
return matchRules;
}
/**
* @param matchRules matchRules or {@code null} for none
*/
public HttpRouteRule setMatchRules(java.util.List matchRules) {
this.matchRules = matchRules;
return this;
}
/**
* For routeRules within a given pathMatcher, priority determines the order in which load balancer
* will interpret routeRules. RouteRules are evaluated in order of priority, from the lowest to
* highest number. The priority of a rule decreases as its number increases (1, 2, 3, N+1). The
* first rule that matches the request is applied. You cannot configure two or more routeRules
* with the same priority. Priority for each rule must be set to a number between 0 and 2147483647
* inclusive. Priority numbers can have gaps, which enable you to add or remove rules in the
* future without affecting the rest of the rules. For example, 1, 2, 3, 4, 5, 9, 12, 16 is a
* valid series of priority numbers to which you could add rules numbered from 6 to 8, 10 to 11,
* and 13 to 15 in the future without any impact on existing rules.
* @return value or {@code null} for none
*/
public java.lang.Integer getPriority() {
return priority;
}
/**
* For routeRules within a given pathMatcher, priority determines the order in which load balancer
* will interpret routeRules. RouteRules are evaluated in order of priority, from the lowest to
* highest number. The priority of a rule decreases as its number increases (1, 2, 3, N+1). The
* first rule that matches the request is applied. You cannot configure two or more routeRules
* with the same priority. Priority for each rule must be set to a number between 0 and 2147483647
* inclusive. Priority numbers can have gaps, which enable you to add or remove rules in the
* future without affecting the rest of the rules. For example, 1, 2, 3, 4, 5, 9, 12, 16 is a
* valid series of priority numbers to which you could add rules numbered from 6 to 8, 10 to 11,
* and 13 to 15 in the future without any impact on existing rules.
* @param priority priority or {@code null} for none
*/
public HttpRouteRule setPriority(java.lang.Integer priority) {
this.priority = priority;
return this;
}
/**
* In response to a matching matchRule, the load balancer performs advanced routing actions like
* URL rewrites, header transformations, etc. prior to forwarding the request to the selected
* backend. If routeAction specifies any weightedBackendServices, service must not be set.
* Conversely if service is set, routeAction cannot contain any weightedBackendServices. Only one
* of urlRedirect, service or routeAction.weightedBackendService must be set.
* @return value or {@code null} for none
*/
public HttpRouteAction getRouteAction() {
return routeAction;
}
/**
* In response to a matching matchRule, the load balancer performs advanced routing actions like
* URL rewrites, header transformations, etc. prior to forwarding the request to the selected
* backend. If routeAction specifies any weightedBackendServices, service must not be set.
* Conversely if service is set, routeAction cannot contain any weightedBackendServices. Only one
* of urlRedirect, service or routeAction.weightedBackendService must be set.
* @param routeAction routeAction or {@code null} for none
*/
public HttpRouteRule setRouteAction(HttpRouteAction routeAction) {
this.routeAction = routeAction;
return this;
}
/**
* The full or partial URL of the backend service resource to which traffic is directed if this
* rule is matched. If routeAction is additionally specified, advanced routing actions like URL
* Rewrites, etc. take effect prior to sending the request to the backend. However, if service is
* specified, routeAction cannot contain any weightedBackendService s. Conversely, if routeAction
* specifies any weightedBackendServices, service must not be specified. Only one of urlRedirect,
* service or routeAction.weightedBackendService must be set.
* @return value or {@code null} for none
*/
public java.lang.String getService() {
return service;
}
/**
* The full or partial URL of the backend service resource to which traffic is directed if this
* rule is matched. If routeAction is additionally specified, advanced routing actions like URL
* Rewrites, etc. take effect prior to sending the request to the backend. However, if service is
* specified, routeAction cannot contain any weightedBackendService s. Conversely, if routeAction
* specifies any weightedBackendServices, service must not be specified. Only one of urlRedirect,
* service or routeAction.weightedBackendService must be set.
* @param service service or {@code null} for none
*/
public HttpRouteRule setService(java.lang.String service) {
this.service = service;
return this;
}
/**
* When this rule is matched, the request is redirected to a URL specified by urlRedirect. If
* urlRedirect is specified, service or routeAction must not be set.
* @return value or {@code null} for none
*/
public HttpRedirectAction getUrlRedirect() {
return urlRedirect;
}
/**
* When this rule is matched, the request is redirected to a URL specified by urlRedirect. If
* urlRedirect is specified, service or routeAction must not be set.
* @param urlRedirect urlRedirect or {@code null} for none
*/
public HttpRouteRule setUrlRedirect(HttpRedirectAction urlRedirect) {
this.urlRedirect = urlRedirect;
return this;
}
@Override
public HttpRouteRule set(String fieldName, Object value) {
return (HttpRouteRule) super.set(fieldName, value);
}
@Override
public HttpRouteRule clone() {
return (HttpRouteRule) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy