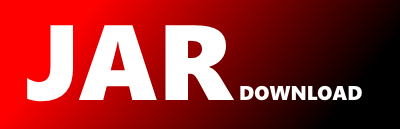
target.apidocs.com.google.api.services.compute.model.Address.html Maven / Gradle / Ivy
Address (Compute Engine API alpha-rev20200526-1.30.9)
com.google.api.services.compute.model
Class Address
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.Address
-
public final class Address
extends GenericJson
Use global external addresses for GFE-based external HTTP(S) load balancers in Premium Tier.
Use global internal addresses for reserved peering network range.
Use regional external addresses for the following resources:
- External IP addresses for VM instances - Regional external forwarding rules - Cloud NAT
external IP addresses - GFE based LBs in Standard Tier - Network LBs in Premium or Standard Tier
- Cloud VPN gateways (both Classic and HA)
Use regional internal IP addresses for subnet IP ranges (primary and secondary). This includes:
- Internal IP addresses for VM instances - Alias IP ranges of VM instances (/32 only) - Regional
internal forwarding rules - Internal TCP/UDP load balancer addresses - Internal HTTP(S) load
balancer addresses - Cloud DNS inbound forwarding IP addresses
For more information, read reserved IP address.
(== resource_for {$api_version}.addresses ==) (== resource_for {$api_version}.globalAddresses ==)
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Address()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Address
clone()
byte[]
decodeLabelFingerprint()
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking.
Address
encodeLabelFingerprint(byte[] labelFingerprint)
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking.
String
getAddress()
The static IP address represented by this resource.
String
getAddressType()
The type of address to reserve, either INTERNAL or EXTERNAL.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional description of this resource.
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getIpVersion()
The IP version that will be used by this address.
String
getKind()
[Output Only] Type of the resource.
String
getLabelFingerprint()
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking.
Map<String,String>
getLabels()
Labels for this resource.
String
getName()
Name of the resource.
String
getNetwork()
The URL of the network in which to reserve the address.
String
getNetworkTier()
This signifies the networking tier used for configuring this address and can only take the
following values: PREMIUM or STANDARD.
Integer
getPrefixLength()
The prefix length if the resource reprensents an IP range.
String
getPurpose()
The purpose of this resource, which can be one of the following values: - `GCE_ENDPOINT` for
addresses that are used by VM instances, alias IP ranges, internal load balancers, and similar
resources.
String
getRegion()
[Output Only] The URL of the region where the regional address resides.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
String
getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
String
getStatus()
[Output Only] The status of the address, which can be one of RESERVING, RESERVED, or IN_USE.
String
getSubnetwork()
The URL of the subnetwork in which to reserve the address.
List<String>
getUsers()
[Output Only] The URLs of the resources that are using this address.
Address
set(String fieldName,
Object value)
Address
setAddress(String address)
The static IP address represented by this resource.
Address
setAddressType(String addressType)
The type of address to reserve, either INTERNAL or EXTERNAL.
Address
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
Address
setDescription(String description)
An optional description of this resource.
Address
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
Address
setIpVersion(String ipVersion)
The IP version that will be used by this address.
Address
setKind(String kind)
[Output Only] Type of the resource.
Address
setLabelFingerprint(String labelFingerprint)
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking.
Address
setLabels(Map<String,String> labels)
Labels for this resource.
Address
setName(String name)
Name of the resource.
Address
setNetwork(String network)
The URL of the network in which to reserve the address.
Address
setNetworkTier(String networkTier)
This signifies the networking tier used for configuring this address and can only take the
following values: PREMIUM or STANDARD.
Address
setPrefixLength(Integer prefixLength)
The prefix length if the resource reprensents an IP range.
Address
setPurpose(String purpose)
The purpose of this resource, which can be one of the following values: - `GCE_ENDPOINT` for
addresses that are used by VM instances, alias IP ranges, internal load balancers, and similar
resources.
Address
setRegion(String region)
[Output Only] The URL of the region where the regional address resides.
Address
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
Address
setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
Address
setStatus(String status)
[Output Only] The status of the address, which can be one of RESERVING, RESERVED, or IN_USE.
Address
setSubnetwork(String subnetwork)
The URL of the subnetwork in which to reserve the address.
Address
setUsers(List<String> users)
[Output Only] The URLs of the resources that are using this address.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAddress
public String getAddress()
The static IP address represented by this resource.
- Returns:
- value or
null
for none
-
setAddress
public Address setAddress(String address)
The static IP address represented by this resource.
- Parameters:
address
- address or null
for none
-
getAddressType
public String getAddressType()
The type of address to reserve, either INTERNAL or EXTERNAL. If unspecified, defaults to
EXTERNAL.
- Returns:
- value or
null
for none
-
setAddressType
public Address setAddressType(String addressType)
The type of address to reserve, either INTERNAL or EXTERNAL. If unspecified, defaults to
EXTERNAL.
- Parameters:
addressType
- addressType or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public Address setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this field when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public Address setDescription(String description)
An optional description of this resource. Provide this field when you create the resource.
- Parameters:
description
- description or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public Address setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getIpVersion
public String getIpVersion()
The IP version that will be used by this address. Valid options are IPV4 or IPV6. This can only
be specified for a global address.
- Returns:
- value or
null
for none
-
setIpVersion
public Address setIpVersion(String ipVersion)
The IP version that will be used by this address. Valid options are IPV4 or IPV6. This can only
be specified for a global address.
- Parameters:
ipVersion
- ipVersion or null
for none
-
getKind
public String getKind()
[Output Only] Type of the resource. Always compute#address for addresses.
- Returns:
- value or
null
for none
-
setKind
public Address setKind(String kind)
[Output Only] Type of the resource. Always compute#address for addresses.
- Parameters:
kind
- kind or null
for none
-
getLabelFingerprint
public String getLabelFingerprint()
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking. The fingerprint is initially generated by Compute
Engine and changes after every request to modify or update labels. You must always provide an
up-to-date fingerprint hash in order to update or change labels, otherwise the request will
fail with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve an Address.
- Returns:
- value or
null
for none
- See Also:
decodeLabelFingerprint()
-
decodeLabelFingerprint
public byte[] decodeLabelFingerprint()
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking. The fingerprint is initially generated by Compute
Engine and changes after every request to modify or update labels. You must always provide an
up-to-date fingerprint hash in order to update or change labels, otherwise the request will
fail with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve an Address.
- Returns:
- Base64 decoded value or
null
for none
- Since:
- 1.14
- See Also:
getLabelFingerprint()
-
setLabelFingerprint
public Address setLabelFingerprint(String labelFingerprint)
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking. The fingerprint is initially generated by Compute
Engine and changes after every request to modify or update labels. You must always provide an
up-to-date fingerprint hash in order to update or change labels, otherwise the request will
fail with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve an Address.
- Parameters:
labelFingerprint
- labelFingerprint or null
for none
- See Also:
#encodeLabelFingerprint()
-
encodeLabelFingerprint
public Address encodeLabelFingerprint(byte[] labelFingerprint)
A fingerprint for the labels being applied to this Address, which is essentially a hash of the
labels set used for optimistic locking. The fingerprint is initially generated by Compute
Engine and changes after every request to modify or update labels. You must always provide an
up-to-date fingerprint hash in order to update or change labels, otherwise the request will
fail with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve an Address.
- Since:
- 1.14
- See Also:
The value is encoded Base64 or {@code null} for none.
-
getLabels
public Map<String,String> getLabels()
Labels for this resource. These can only be added or modified by the setLabels method. Each
label key/value pair must comply with RFC1035. Label values may be empty.
- Returns:
- value or
null
for none
-
setLabels
public Address setLabels(Map<String,String> labels)
Labels for this resource. These can only be added or modified by the setLabels method. Each
label key/value pair must comply with RFC1035. Label values may be empty.
- Parameters:
labels
- labels or null
for none
-
getName
public String getName()
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be
a lowercase letter, and all following characters (except for the last character) must be a
dash, lowercase letter, or digit. The last character must be a lowercase letter or digit.
- Returns:
- value or
null
for none
-
setName
public Address setName(String name)
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be
a lowercase letter, and all following characters (except for the last character) must be a
dash, lowercase letter, or digit. The last character must be a lowercase letter or digit.
- Parameters:
name
- name or null
for none
-
getNetwork
public String getNetwork()
The URL of the network in which to reserve the address. This field can only be used with
INTERNAL type with the VPC_PEERING purpose.
- Returns:
- value or
null
for none
-
setNetwork
public Address setNetwork(String network)
The URL of the network in which to reserve the address. This field can only be used with
INTERNAL type with the VPC_PEERING purpose.
- Parameters:
network
- network or null
for none
-
getNetworkTier
public String getNetworkTier()
This signifies the networking tier used for configuring this address and can only take the
following values: PREMIUM or STANDARD. Global forwarding rules can only be Premium Tier.
Regional forwarding rules can be either Premium or Standard Tier. Standard Tier addresses
applied to regional forwarding rules can be used with any external load balancer. Regional
forwarding rules in Premium Tier can only be used with a network load balancer.
If this field is not specified, it is assumed to be PREMIUM.
- Returns:
- value or
null
for none
-
setNetworkTier
public Address setNetworkTier(String networkTier)
This signifies the networking tier used for configuring this address and can only take the
following values: PREMIUM or STANDARD. Global forwarding rules can only be Premium Tier.
Regional forwarding rules can be either Premium or Standard Tier. Standard Tier addresses
applied to regional forwarding rules can be used with any external load balancer. Regional
forwarding rules in Premium Tier can only be used with a network load balancer.
If this field is not specified, it is assumed to be PREMIUM.
- Parameters:
networkTier
- networkTier or null
for none
-
getPrefixLength
public Integer getPrefixLength()
The prefix length if the resource reprensents an IP range.
- Returns:
- value or
null
for none
-
setPrefixLength
public Address setPrefixLength(Integer prefixLength)
The prefix length if the resource reprensents an IP range.
- Parameters:
prefixLength
- prefixLength or null
for none
-
getPurpose
public String getPurpose()
The purpose of this resource, which can be one of the following values: - `GCE_ENDPOINT` for
addresses that are used by VM instances, alias IP ranges, internal load balancers, and similar
resources. - `DNS_RESOLVER` for a DNS resolver address in a subnetwork - `VPC_PEERING` for
addresses that are reserved for VPC peer networks. - `NAT_AUTO` for addresses that are
external IP addresses automatically reserved for Cloud NAT.
- Returns:
- value or
null
for none
-
setPurpose
public Address setPurpose(String purpose)
The purpose of this resource, which can be one of the following values: - `GCE_ENDPOINT` for
addresses that are used by VM instances, alias IP ranges, internal load balancers, and similar
resources. - `DNS_RESOLVER` for a DNS resolver address in a subnetwork - `VPC_PEERING` for
addresses that are reserved for VPC peer networks. - `NAT_AUTO` for addresses that are
external IP addresses automatically reserved for Cloud NAT.
- Parameters:
purpose
- purpose or null
for none
-
getRegion
public String getRegion()
[Output Only] The URL of the region where the regional address resides. This field is not
applicable to global addresses. You must specify this field as part of the HTTP request URL.
- Returns:
- value or
null
for none
-
setRegion
public Address setRegion(String region)
[Output Only] The URL of the region where the regional address resides. This field is not
applicable to global addresses. You must specify this field as part of the HTTP request URL.
- Parameters:
region
- region or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public Address setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getSelfLinkWithId
public String getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
- Returns:
- value or
null
for none
-
setSelfLinkWithId
public Address setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
- Parameters:
selfLinkWithId
- selfLinkWithId or null
for none
-
getStatus
public String getStatus()
[Output Only] The status of the address, which can be one of RESERVING, RESERVED, or IN_USE. An
address that is RESERVING is currently in the process of being reserved. A RESERVED address is
currently reserved and available to use. An IN_USE address is currently being used by another
resource and is not available.
- Returns:
- value or
null
for none
-
setStatus
public Address setStatus(String status)
[Output Only] The status of the address, which can be one of RESERVING, RESERVED, or IN_USE. An
address that is RESERVING is currently in the process of being reserved. A RESERVED address is
currently reserved and available to use. An IN_USE address is currently being used by another
resource and is not available.
- Parameters:
status
- status or null
for none
-
getSubnetwork
public String getSubnetwork()
The URL of the subnetwork in which to reserve the address. If an IP address is specified, it
must be within the subnetwork's IP range. This field can only be used with INTERNAL type with a
GCE_ENDPOINT or DNS_RESOLVER purpose.
- Returns:
- value or
null
for none
-
setSubnetwork
public Address setSubnetwork(String subnetwork)
The URL of the subnetwork in which to reserve the address. If an IP address is specified, it
must be within the subnetwork's IP range. This field can only be used with INTERNAL type with a
GCE_ENDPOINT or DNS_RESOLVER purpose.
- Parameters:
subnetwork
- subnetwork or null
for none
-
getUsers
public List<String> getUsers()
[Output Only] The URLs of the resources that are using this address.
- Returns:
- value or
null
for none
-
setUsers
public Address setUsers(List<String> users)
[Output Only] The URLs of the resources that are using this address.
- Parameters:
users
- users or null
for none
-
set
public Address set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Address clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy