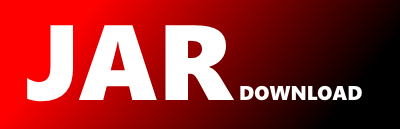
target.apidocs.com.google.api.services.compute.model.License.html Maven / Gradle / Ivy
License (Compute Engine API alpha-rev20200526-1.30.9)
com.google.api.services.compute.model
Class License
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.License
-
public final class License
extends GenericJson
Represents a License resource.
A License represents billing and aggregate usage data for public and marketplace images. Caution
This resource is intended for use only by third-party partners who are creating Cloud Marketplace
images. (== resource_for {$api_version}.licenses ==)
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
License()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
License
clone()
Boolean
getChargesUseFee()
[Output Only] Deprecated.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional textual description of the resource; provided by the client when the resource is
created.
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getKind()
[Output Only] Type of resource.
BigInteger
getLicenseCode()
[Output Only] The unique code used to attach this license to images, snapshots, and disks.
String
getName()
Name of the resource.
LicenseResourceRequirements
getResourceRequirements()
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
String
getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
Boolean
getTransferable()
If false, licenses will not be copied from the source resource when creating an image from a
disk, disk from snapshot, or snapshot from disk.
License
set(String fieldName,
Object value)
License
setChargesUseFee(Boolean chargesUseFee)
[Output Only] Deprecated.
License
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
License
setDescription(String description)
An optional textual description of the resource; provided by the client when the resource is
created.
License
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
License
setKind(String kind)
[Output Only] Type of resource.
License
setLicenseCode(BigInteger licenseCode)
[Output Only] The unique code used to attach this license to images, snapshots, and disks.
License
setName(String name)
Name of the resource.
License
setResourceRequirements(LicenseResourceRequirements resourceRequirements)
License
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
License
setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
License
setTransferable(Boolean transferable)
If false, licenses will not be copied from the source resource when creating an image from a
disk, disk from snapshot, or snapshot from disk.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getChargesUseFee
public Boolean getChargesUseFee()
[Output Only] Deprecated. This field no longer reflects whether a license charges a usage fee.
- Returns:
- value or
null
for none
-
setChargesUseFee
public License setChargesUseFee(Boolean chargesUseFee)
[Output Only] Deprecated. This field no longer reflects whether a license charges a usage fee.
- Parameters:
chargesUseFee
- chargesUseFee or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public License setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional textual description of the resource; provided by the client when the resource is
created.
- Returns:
- value or
null
for none
-
setDescription
public License setDescription(String description)
An optional textual description of the resource; provided by the client when the resource is
created.
- Parameters:
description
- description or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public License setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output Only] Type of resource. Always compute#license for licenses.
- Returns:
- value or
null
for none
-
setKind
public License setKind(String kind)
[Output Only] Type of resource. Always compute#license for licenses.
- Parameters:
kind
- kind or null
for none
-
getLicenseCode
public BigInteger getLicenseCode()
[Output Only] The unique code used to attach this license to images, snapshots, and disks.
- Returns:
- value or
null
for none
-
setLicenseCode
public License setLicenseCode(BigInteger licenseCode)
[Output Only] The unique code used to attach this license to images, snapshots, and disks.
- Parameters:
licenseCode
- licenseCode or null
for none
-
getName
public String getName()
Name of the resource. The name must be 1-63 characters long and comply with RFC1035.
- Returns:
- value or
null
for none
-
setName
public License setName(String name)
Name of the resource. The name must be 1-63 characters long and comply with RFC1035.
- Parameters:
name
- name or null
for none
-
getResourceRequirements
public LicenseResourceRequirements getResourceRequirements()
- Returns:
- value or
null
for none
-
setResourceRequirements
public License setResourceRequirements(LicenseResourceRequirements resourceRequirements)
- Parameters:
resourceRequirements
- resourceRequirements or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public License setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getSelfLinkWithId
public String getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
- Returns:
- value or
null
for none
-
setSelfLinkWithId
public License setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
- Parameters:
selfLinkWithId
- selfLinkWithId or null
for none
-
getTransferable
public Boolean getTransferable()
If false, licenses will not be copied from the source resource when creating an image from a
disk, disk from snapshot, or snapshot from disk.
- Returns:
- value or
null
for none
-
setTransferable
public License setTransferable(Boolean transferable)
If false, licenses will not be copied from the source resource when creating an image from a
disk, disk from snapshot, or snapshot from disk.
- Parameters:
transferable
- transferable or null
for none
-
set
public License set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public License clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy