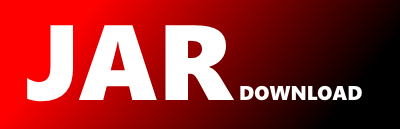
target.apidocs.com.google.api.services.compute.model.MachineImage.html Maven / Gradle / Ivy
MachineImage (Compute Engine API alpha-rev20200526-1.30.9)
com.google.api.services.compute.model
Class MachineImage
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.MachineImage
-
public final class MachineImage
extends GenericJson
Represents a machine image resource.
A machine image is a Compute Engine resource that stores all the configuration, metadata,
permissions, and data from one or more disks required to create a Virtual machine (VM) instance.
For more information, see Machine images. (== resource_for {$api_version}.machineImages ==)
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
MachineImage()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
MachineImage
clone()
String
getCreationTimestamp()
[Output Only] The creation timestamp for this machine image in RFC3339 text format.
String
getDescription()
An optional description of this resource.
Boolean
getGuestFlush()
[Input Only] Specifies to create an application consistent machine image by informing the OS to
prepare for the snapshot process.
BigInteger
getId()
[Output Only] A unique identifier for this machine image.
String
getKind()
[Output Only] The resource type, which is always compute#machineImage for machine image.
CustomerEncryptionKey
getMachineImageEncryptionKey()
Encrypts the machine image using a customer-supplied encryption key.
String
getName()
Name of the resource; provided by the client when the resource is created.
String
getSelfLink()
[Output Only] The URL for this machine image.
String
getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
List<SourceDiskEncryptionKey>
getSourceDiskEncryptionKeys()
[Input Only] The customer-supplied encryption key of the disks attached to the source instance.
String
getSourceInstance()
The source instance used to create the machine image.
SourceInstanceProperties
getSourceInstanceProperties()
[Output Only] Properties of source instance.
String
getStatus()
[Output Only] The status of the machine image.
List<String>
getStorageLocations()
The regional or multi-regional Cloud Storage bucket location where the machine image is stored.
Long
getTotalStorageBytes()
[Output Only] Total size of the storage used by the machine image.
MachineImage
set(String fieldName,
Object value)
MachineImage
setCreationTimestamp(String creationTimestamp)
[Output Only] The creation timestamp for this machine image in RFC3339 text format.
MachineImage
setDescription(String description)
An optional description of this resource.
MachineImage
setGuestFlush(Boolean guestFlush)
[Input Only] Specifies to create an application consistent machine image by informing the OS to
prepare for the snapshot process.
MachineImage
setId(BigInteger id)
[Output Only] A unique identifier for this machine image.
MachineImage
setKind(String kind)
[Output Only] The resource type, which is always compute#machineImage for machine image.
MachineImage
setMachineImageEncryptionKey(CustomerEncryptionKey machineImageEncryptionKey)
Encrypts the machine image using a customer-supplied encryption key.
MachineImage
setName(String name)
Name of the resource; provided by the client when the resource is created.
MachineImage
setSelfLink(String selfLink)
[Output Only] The URL for this machine image.
MachineImage
setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
MachineImage
setSourceDiskEncryptionKeys(List<SourceDiskEncryptionKey> sourceDiskEncryptionKeys)
[Input Only] The customer-supplied encryption key of the disks attached to the source instance.
MachineImage
setSourceInstance(String sourceInstance)
The source instance used to create the machine image.
MachineImage
setSourceInstanceProperties(SourceInstanceProperties sourceInstanceProperties)
[Output Only] Properties of source instance.
MachineImage
setStatus(String status)
[Output Only] The status of the machine image.
MachineImage
setStorageLocations(List<String> storageLocations)
The regional or multi-regional Cloud Storage bucket location where the machine image is stored.
MachineImage
setTotalStorageBytes(Long totalStorageBytes)
[Output Only] Total size of the storage used by the machine image.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] The creation timestamp for this machine image in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public MachineImage setCreationTimestamp(String creationTimestamp)
[Output Only] The creation timestamp for this machine image in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public MachineImage setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getGuestFlush
public Boolean getGuestFlush()
[Input Only] Specifies to create an application consistent machine image by informing the OS to
prepare for the snapshot process. Currently only supported on Windows instances using the
Volume Shadow Copy Service (VSS).
- Returns:
- value or
null
for none
-
setGuestFlush
public MachineImage setGuestFlush(Boolean guestFlush)
[Input Only] Specifies to create an application consistent machine image by informing the OS to
prepare for the snapshot process. Currently only supported on Windows instances using the
Volume Shadow Copy Service (VSS).
- Parameters:
guestFlush
- guestFlush or null
for none
-
getId
public BigInteger getId()
[Output Only] A unique identifier for this machine image. The server defines this identifier.
- Returns:
- value or
null
for none
-
setId
public MachineImage setId(BigInteger id)
[Output Only] A unique identifier for this machine image. The server defines this identifier.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output Only] The resource type, which is always compute#machineImage for machine image.
- Returns:
- value or
null
for none
-
setKind
public MachineImage setKind(String kind)
[Output Only] The resource type, which is always compute#machineImage for machine image.
- Parameters:
kind
- kind or null
for none
-
getMachineImageEncryptionKey
public CustomerEncryptionKey getMachineImageEncryptionKey()
Encrypts the machine image using a customer-supplied encryption key.
After you encrypt a machine image using a customer-supplied key, you must provide the same key
if you use the machine image later. For example, you must provide the encryption key when you
create an instance from the encrypted machine image in a future request.
Customer-supplied encryption keys do not protect access to metadata of the machine image.
If you do not provide an encryption key when creating the machine image, then the machine image
will be encrypted using an automatically generated key and you do not need to provide a key to
use the machine image later.
- Returns:
- value or
null
for none
-
setMachineImageEncryptionKey
public MachineImage setMachineImageEncryptionKey(CustomerEncryptionKey machineImageEncryptionKey)
Encrypts the machine image using a customer-supplied encryption key.
After you encrypt a machine image using a customer-supplied key, you must provide the same key
if you use the machine image later. For example, you must provide the encryption key when you
create an instance from the encrypted machine image in a future request.
Customer-supplied encryption keys do not protect access to metadata of the machine image.
If you do not provide an encryption key when creating the machine image, then the machine image
will be encrypted using an automatically generated key and you do not need to provide a key to
use the machine image later.
- Parameters:
machineImageEncryptionKey
- machineImageEncryptionKey or null
for none
-
getName
public String getName()
Name of the resource; provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Returns:
- value or
null
for none
-
setName
public MachineImage setName(String name)
Name of the resource; provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Parameters:
name
- name or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] The URL for this machine image. The server defines this URL.
- Returns:
- value or
null
for none
-
setSelfLink
public MachineImage setSelfLink(String selfLink)
[Output Only] The URL for this machine image. The server defines this URL.
- Parameters:
selfLink
- selfLink or null
for none
-
getSelfLinkWithId
public String getSelfLinkWithId()
[Output Only] Server-defined URL for this resource with the resource id.
- Returns:
- value or
null
for none
-
setSelfLinkWithId
public MachineImage setSelfLinkWithId(String selfLinkWithId)
[Output Only] Server-defined URL for this resource with the resource id.
- Parameters:
selfLinkWithId
- selfLinkWithId or null
for none
-
getSourceDiskEncryptionKeys
public List<SourceDiskEncryptionKey> getSourceDiskEncryptionKeys()
[Input Only] The customer-supplied encryption key of the disks attached to the source instance.
Required if the source disk is protected by a customer-supplied encryption key.
- Returns:
- value or
null
for none
-
setSourceDiskEncryptionKeys
public MachineImage setSourceDiskEncryptionKeys(List<SourceDiskEncryptionKey> sourceDiskEncryptionKeys)
[Input Only] The customer-supplied encryption key of the disks attached to the source instance.
Required if the source disk is protected by a customer-supplied encryption key.
- Parameters:
sourceDiskEncryptionKeys
- sourceDiskEncryptionKeys or null
for none
-
getSourceInstance
public String getSourceInstance()
The source instance used to create the machine image. You can provide this as a partial or full
URL to the resource. For example, the following are valid values: -
https://www.googleapis.com/compute/v1/projects/project/zones/zone/instances/instance -
projects/project/zones/zone/instances/instance
- Returns:
- value or
null
for none
-
setSourceInstance
public MachineImage setSourceInstance(String sourceInstance)
The source instance used to create the machine image. You can provide this as a partial or full
URL to the resource. For example, the following are valid values: -
https://www.googleapis.com/compute/v1/projects/project/zones/zone/instances/instance -
projects/project/zones/zone/instances/instance
- Parameters:
sourceInstance
- sourceInstance or null
for none
-
getSourceInstanceProperties
public SourceInstanceProperties getSourceInstanceProperties()
[Output Only] Properties of source instance.
- Returns:
- value or
null
for none
-
setSourceInstanceProperties
public MachineImage setSourceInstanceProperties(SourceInstanceProperties sourceInstanceProperties)
[Output Only] Properties of source instance.
- Parameters:
sourceInstanceProperties
- sourceInstanceProperties or null
for none
-
getStatus
public String getStatus()
[Output Only] The status of the machine image. One of the following values: INVALID, CREATING,
READY, DELETING, and UPLOADING.
- Returns:
- value or
null
for none
-
setStatus
public MachineImage setStatus(String status)
[Output Only] The status of the machine image. One of the following values: INVALID, CREATING,
READY, DELETING, and UPLOADING.
- Parameters:
status
- status or null
for none
-
getStorageLocations
public List<String> getStorageLocations()
The regional or multi-regional Cloud Storage bucket location where the machine image is stored.
- Returns:
- value or
null
for none
-
setStorageLocations
public MachineImage setStorageLocations(List<String> storageLocations)
The regional or multi-regional Cloud Storage bucket location where the machine image is stored.
- Parameters:
storageLocations
- storageLocations or null
for none
-
getTotalStorageBytes
public Long getTotalStorageBytes()
[Output Only] Total size of the storage used by the machine image.
- Returns:
- value or
null
for none
-
setTotalStorageBytes
public MachineImage setTotalStorageBytes(Long totalStorageBytes)
[Output Only] Total size of the storage used by the machine image.
- Parameters:
totalStorageBytes
- totalStorageBytes or null
for none
-
set
public MachineImage set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public MachineImage clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy