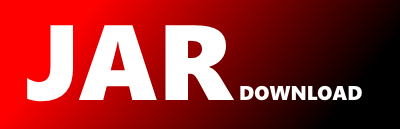
target.apidocs.com.google.api.services.compute.model.OutlierDetection.html Maven / Gradle / Ivy
OutlierDetection (Compute Engine API alpha-rev20200526-1.30.9)
com.google.api.services.compute.model
Class OutlierDetection
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.OutlierDetection
-
public final class OutlierDetection
extends GenericJson
Settings controlling the eviction of unhealthy hosts from the load balancing pool for the backend
service.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
OutlierDetection()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
OutlierDetection
clone()
Duration
getBaseEjectionTime()
The base time that a host is ejected for.
Integer
getConsecutiveErrors()
Number of errors before a host is ejected from the connection pool.
Integer
getConsecutiveGatewayFailure()
The number of consecutive gateway failures (502, 503, 504 status or connection errors that are
mapped to one of those status codes) before a consecutive gateway failure ejection occurs.
Integer
getEnforcingConsecutiveErrors()
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive 5xx.
Integer
getEnforcingConsecutiveGatewayFailure()
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive gateway failures.
Integer
getEnforcingSuccessRate()
The percentage chance that a host will be actually ejected when an outlier status is detected
through success rate statistics.
Duration
getInterval()
Time interval between ejection analysis sweeps.
Integer
getMaxEjectionPercent()
Maximum percentage of hosts in the load balancing pool for the backend service that can be
ejected.
Integer
getSuccessRateMinimumHosts()
The number of hosts in a cluster that must have enough request volume to detect success rate
outliers.
Integer
getSuccessRateRequestVolume()
The minimum number of total requests that must be collected in one interval (as defined by the
interval duration above) to include this host in success rate based outlier detection.
Integer
getSuccessRateStdevFactor()
This factor is used to determine the ejection threshold for success rate outlier ejection.
OutlierDetection
set(String fieldName,
Object value)
OutlierDetection
setBaseEjectionTime(Duration baseEjectionTime)
The base time that a host is ejected for.
OutlierDetection
setConsecutiveErrors(Integer consecutiveErrors)
Number of errors before a host is ejected from the connection pool.
OutlierDetection
setConsecutiveGatewayFailure(Integer consecutiveGatewayFailure)
The number of consecutive gateway failures (502, 503, 504 status or connection errors that are
mapped to one of those status codes) before a consecutive gateway failure ejection occurs.
OutlierDetection
setEnforcingConsecutiveErrors(Integer enforcingConsecutiveErrors)
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive 5xx.
OutlierDetection
setEnforcingConsecutiveGatewayFailure(Integer enforcingConsecutiveGatewayFailure)
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive gateway failures.
OutlierDetection
setEnforcingSuccessRate(Integer enforcingSuccessRate)
The percentage chance that a host will be actually ejected when an outlier status is detected
through success rate statistics.
OutlierDetection
setInterval(Duration interval)
Time interval between ejection analysis sweeps.
OutlierDetection
setMaxEjectionPercent(Integer maxEjectionPercent)
Maximum percentage of hosts in the load balancing pool for the backend service that can be
ejected.
OutlierDetection
setSuccessRateMinimumHosts(Integer successRateMinimumHosts)
The number of hosts in a cluster that must have enough request volume to detect success rate
outliers.
OutlierDetection
setSuccessRateRequestVolume(Integer successRateRequestVolume)
The minimum number of total requests that must be collected in one interval (as defined by the
interval duration above) to include this host in success rate based outlier detection.
OutlierDetection
setSuccessRateStdevFactor(Integer successRateStdevFactor)
This factor is used to determine the ejection threshold for success rate outlier ejection.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getBaseEjectionTime
public Duration getBaseEjectionTime()
The base time that a host is ejected for. The real ejection time is equal to the base ejection
time multiplied by the number of times the host has been ejected. Defaults to 30000ms or 30s.
- Returns:
- value or
null
for none
-
setBaseEjectionTime
public OutlierDetection setBaseEjectionTime(Duration baseEjectionTime)
The base time that a host is ejected for. The real ejection time is equal to the base ejection
time multiplied by the number of times the host has been ejected. Defaults to 30000ms or 30s.
- Parameters:
baseEjectionTime
- baseEjectionTime or null
for none
-
getConsecutiveErrors
public Integer getConsecutiveErrors()
Number of errors before a host is ejected from the connection pool. When the backend host is
accessed over HTTP, a 5xx return code qualifies as an error. Defaults to 5.
- Returns:
- value or
null
for none
-
setConsecutiveErrors
public OutlierDetection setConsecutiveErrors(Integer consecutiveErrors)
Number of errors before a host is ejected from the connection pool. When the backend host is
accessed over HTTP, a 5xx return code qualifies as an error. Defaults to 5.
- Parameters:
consecutiveErrors
- consecutiveErrors or null
for none
-
getConsecutiveGatewayFailure
public Integer getConsecutiveGatewayFailure()
The number of consecutive gateway failures (502, 503, 504 status or connection errors that are
mapped to one of those status codes) before a consecutive gateway failure ejection occurs.
Defaults to 3.
- Returns:
- value or
null
for none
-
setConsecutiveGatewayFailure
public OutlierDetection setConsecutiveGatewayFailure(Integer consecutiveGatewayFailure)
The number of consecutive gateway failures (502, 503, 504 status or connection errors that are
mapped to one of those status codes) before a consecutive gateway failure ejection occurs.
Defaults to 3.
- Parameters:
consecutiveGatewayFailure
- consecutiveGatewayFailure or null
for none
-
getEnforcingConsecutiveErrors
public Integer getEnforcingConsecutiveErrors()
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive 5xx. This setting can be used to disable ejection or to ramp it up slowly.
Defaults to 0.
- Returns:
- value or
null
for none
-
setEnforcingConsecutiveErrors
public OutlierDetection setEnforcingConsecutiveErrors(Integer enforcingConsecutiveErrors)
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive 5xx. This setting can be used to disable ejection or to ramp it up slowly.
Defaults to 0.
- Parameters:
enforcingConsecutiveErrors
- enforcingConsecutiveErrors or null
for none
-
getEnforcingConsecutiveGatewayFailure
public Integer getEnforcingConsecutiveGatewayFailure()
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive gateway failures. This setting can be used to disable ejection or to ramp
it up slowly. Defaults to 100.
- Returns:
- value or
null
for none
-
setEnforcingConsecutiveGatewayFailure
public OutlierDetection setEnforcingConsecutiveGatewayFailure(Integer enforcingConsecutiveGatewayFailure)
The percentage chance that a host will be actually ejected when an outlier status is detected
through consecutive gateway failures. This setting can be used to disable ejection or to ramp
it up slowly. Defaults to 100.
- Parameters:
enforcingConsecutiveGatewayFailure
- enforcingConsecutiveGatewayFailure or null
for none
-
getEnforcingSuccessRate
public Integer getEnforcingSuccessRate()
The percentage chance that a host will be actually ejected when an outlier status is detected
through success rate statistics. This setting can be used to disable ejection or to ramp it up
slowly. Defaults to 100.
- Returns:
- value or
null
for none
-
setEnforcingSuccessRate
public OutlierDetection setEnforcingSuccessRate(Integer enforcingSuccessRate)
The percentage chance that a host will be actually ejected when an outlier status is detected
through success rate statistics. This setting can be used to disable ejection or to ramp it up
slowly. Defaults to 100.
- Parameters:
enforcingSuccessRate
- enforcingSuccessRate or null
for none
-
getInterval
public Duration getInterval()
Time interval between ejection analysis sweeps. This can result in both new ejections as well
as hosts being returned to service. Defaults to 1 second.
- Returns:
- value or
null
for none
-
setInterval
public OutlierDetection setInterval(Duration interval)
Time interval between ejection analysis sweeps. This can result in both new ejections as well
as hosts being returned to service. Defaults to 1 second.
- Parameters:
interval
- interval or null
for none
-
getMaxEjectionPercent
public Integer getMaxEjectionPercent()
Maximum percentage of hosts in the load balancing pool for the backend service that can be
ejected. Defaults to 50%.
- Returns:
- value or
null
for none
-
setMaxEjectionPercent
public OutlierDetection setMaxEjectionPercent(Integer maxEjectionPercent)
Maximum percentage of hosts in the load balancing pool for the backend service that can be
ejected. Defaults to 50%.
- Parameters:
maxEjectionPercent
- maxEjectionPercent or null
for none
-
getSuccessRateMinimumHosts
public Integer getSuccessRateMinimumHosts()
The number of hosts in a cluster that must have enough request volume to detect success rate
outliers. If the number of hosts is less than this setting, outlier detection via success rate
statistics is not performed for any host in the cluster. Defaults to 5.
- Returns:
- value or
null
for none
-
setSuccessRateMinimumHosts
public OutlierDetection setSuccessRateMinimumHosts(Integer successRateMinimumHosts)
The number of hosts in a cluster that must have enough request volume to detect success rate
outliers. If the number of hosts is less than this setting, outlier detection via success rate
statistics is not performed for any host in the cluster. Defaults to 5.
- Parameters:
successRateMinimumHosts
- successRateMinimumHosts or null
for none
-
getSuccessRateRequestVolume
public Integer getSuccessRateRequestVolume()
The minimum number of total requests that must be collected in one interval (as defined by the
interval duration above) to include this host in success rate based outlier detection. If the
volume is lower than this setting, outlier detection via success rate statistics is not
performed for that host. Defaults to 100.
- Returns:
- value or
null
for none
-
setSuccessRateRequestVolume
public OutlierDetection setSuccessRateRequestVolume(Integer successRateRequestVolume)
The minimum number of total requests that must be collected in one interval (as defined by the
interval duration above) to include this host in success rate based outlier detection. If the
volume is lower than this setting, outlier detection via success rate statistics is not
performed for that host. Defaults to 100.
- Parameters:
successRateRequestVolume
- successRateRequestVolume or null
for none
-
getSuccessRateStdevFactor
public Integer getSuccessRateStdevFactor()
This factor is used to determine the ejection threshold for success rate outlier ejection. The
ejection threshold is the difference between the mean success rate, and the product of this
factor and the standard deviation of the mean success rate: mean - (stdev *
success_rate_stdev_factor). This factor is divided by a thousand to get a double. That is, if
the desired factor is 1.9, the runtime value should be 1900. Defaults to 1900.
- Returns:
- value or
null
for none
-
setSuccessRateStdevFactor
public OutlierDetection setSuccessRateStdevFactor(Integer successRateStdevFactor)
This factor is used to determine the ejection threshold for success rate outlier ejection. The
ejection threshold is the difference between the mean success rate, and the product of this
factor and the standard deviation of the mean success rate: mean - (stdev *
success_rate_stdev_factor). This factor is divided by a thousand to get a double. That is, if
the desired factor is 1.9, the runtime value should be 1900. Defaults to 1900.
- Parameters:
successRateStdevFactor
- successRateStdevFactor or null
for none
-
set
public OutlierDetection set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public OutlierDetection clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy