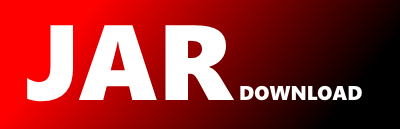
target.apidocs.com.google.api.services.compute.model.RouterNat.html Maven / Gradle / Ivy
RouterNat (Compute Engine API alpha-rev20200526-1.30.9)
com.google.api.services.compute.model
Class RouterNat
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.RouterNat
-
public final class RouterNat
extends GenericJson
Represents a Nat resource. It enables the VMs within the specified subnetworks to access Internet
without external IP addresses. It specifies a list of subnetworks (and the ranges within) that
want to use NAT. Customers can also provide the external IPs that would be used for NAT. GCP
would auto-allocate ephemeral IPs if no external IPs are provided.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
RouterNat()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
RouterNat
clone()
List<String>
getDrainNatIps()
A list of URLs of the IP resources to be drained.
Boolean
getEnableEndpointIndependentMapping()
Integer
getIcmpIdleTimeoutSec()
Timeout (in seconds) for ICMP connections.
RouterNatLogConfig
getLogConfig()
Configure logging on this NAT.
Integer
getMinPortsPerVm()
Minimum number of ports allocated to a VM from this NAT config.
String
getName()
Unique name of this Nat service.
String
getNatIpAllocateOption()
Specify the NatIpAllocateOption, which can take one of the following values: - MANUAL_ONLY:
Uses only Nat IP addresses provided by customers.
List<String>
getNatIps()
A list of URLs of the IP resources used for this Nat service.
List<RouterNatRule>
getRules()
A list of rules associated with this NAT.
String
getSourceSubnetworkIpRangesToNat()
Specify the Nat option, which can take one of the following values: -
ALL_SUBNETWORKS_ALL_IP_RANGES: All of the IP ranges in every Subnetwork are allowed to Nat.
List<RouterNatSubnetworkToNat>
getSubnetworks()
A list of Subnetwork resources whose traffic should be translated by NAT Gateway.
Integer
getTcpEstablishedIdleTimeoutSec()
Timeout (in seconds) for TCP established connections.
Integer
getTcpTimeWaitTimeoutSec()
Timeout (in seconds) for TCP connections that are in TIME_WAIT state.
Integer
getTcpTransitoryIdleTimeoutSec()
Timeout (in seconds) for TCP transitory connections.
Integer
getUdpIdleTimeoutSec()
Timeout (in seconds) for UDP connections.
RouterNat
set(String fieldName,
Object value)
RouterNat
setDrainNatIps(List<String> drainNatIps)
A list of URLs of the IP resources to be drained.
RouterNat
setEnableEndpointIndependentMapping(Boolean enableEndpointIndependentMapping)
RouterNat
setIcmpIdleTimeoutSec(Integer icmpIdleTimeoutSec)
Timeout (in seconds) for ICMP connections.
RouterNat
setLogConfig(RouterNatLogConfig logConfig)
Configure logging on this NAT.
RouterNat
setMinPortsPerVm(Integer minPortsPerVm)
Minimum number of ports allocated to a VM from this NAT config.
RouterNat
setName(String name)
Unique name of this Nat service.
RouterNat
setNatIpAllocateOption(String natIpAllocateOption)
Specify the NatIpAllocateOption, which can take one of the following values: - MANUAL_ONLY:
Uses only Nat IP addresses provided by customers.
RouterNat
setNatIps(List<String> natIps)
A list of URLs of the IP resources used for this Nat service.
RouterNat
setRules(List<RouterNatRule> rules)
A list of rules associated with this NAT.
RouterNat
setSourceSubnetworkIpRangesToNat(String sourceSubnetworkIpRangesToNat)
Specify the Nat option, which can take one of the following values: -
ALL_SUBNETWORKS_ALL_IP_RANGES: All of the IP ranges in every Subnetwork are allowed to Nat.
RouterNat
setSubnetworks(List<RouterNatSubnetworkToNat> subnetworks)
A list of Subnetwork resources whose traffic should be translated by NAT Gateway.
RouterNat
setTcpEstablishedIdleTimeoutSec(Integer tcpEstablishedIdleTimeoutSec)
Timeout (in seconds) for TCP established connections.
RouterNat
setTcpTimeWaitTimeoutSec(Integer tcpTimeWaitTimeoutSec)
Timeout (in seconds) for TCP connections that are in TIME_WAIT state.
RouterNat
setTcpTransitoryIdleTimeoutSec(Integer tcpTransitoryIdleTimeoutSec)
Timeout (in seconds) for TCP transitory connections.
RouterNat
setUdpIdleTimeoutSec(Integer udpIdleTimeoutSec)
Timeout (in seconds) for UDP connections.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getDrainNatIps
public List<String> getDrainNatIps()
A list of URLs of the IP resources to be drained. These IPs must be valid static external IPs
that have been assigned to the NAT. These IPs should be used for updating/patching a NAT only.
- Returns:
- value or
null
for none
-
setDrainNatIps
public RouterNat setDrainNatIps(List<String> drainNatIps)
A list of URLs of the IP resources to be drained. These IPs must be valid static external IPs
that have been assigned to the NAT. These IPs should be used for updating/patching a NAT only.
- Parameters:
drainNatIps
- drainNatIps or null
for none
-
getEnableEndpointIndependentMapping
public Boolean getEnableEndpointIndependentMapping()
- Returns:
- value or
null
for none
-
setEnableEndpointIndependentMapping
public RouterNat setEnableEndpointIndependentMapping(Boolean enableEndpointIndependentMapping)
- Parameters:
enableEndpointIndependentMapping
- enableEndpointIndependentMapping or null
for none
-
getIcmpIdleTimeoutSec
public Integer getIcmpIdleTimeoutSec()
Timeout (in seconds) for ICMP connections. Defaults to 30s if not set.
- Returns:
- value or
null
for none
-
setIcmpIdleTimeoutSec
public RouterNat setIcmpIdleTimeoutSec(Integer icmpIdleTimeoutSec)
Timeout (in seconds) for ICMP connections. Defaults to 30s if not set.
- Parameters:
icmpIdleTimeoutSec
- icmpIdleTimeoutSec or null
for none
-
getLogConfig
public RouterNatLogConfig getLogConfig()
Configure logging on this NAT.
- Returns:
- value or
null
for none
-
setLogConfig
public RouterNat setLogConfig(RouterNatLogConfig logConfig)
Configure logging on this NAT.
- Parameters:
logConfig
- logConfig or null
for none
-
getMinPortsPerVm
public Integer getMinPortsPerVm()
Minimum number of ports allocated to a VM from this NAT config. If not set, a default number of
ports is allocated to a VM. This is rounded up to the nearest power of 2. For example, if the
value of this field is 50, at least 64 ports are allocated to a VM.
- Returns:
- value or
null
for none
-
setMinPortsPerVm
public RouterNat setMinPortsPerVm(Integer minPortsPerVm)
Minimum number of ports allocated to a VM from this NAT config. If not set, a default number of
ports is allocated to a VM. This is rounded up to the nearest power of 2. For example, if the
value of this field is 50, at least 64 ports are allocated to a VM.
- Parameters:
minPortsPerVm
- minPortsPerVm or null
for none
-
getName
public String getName()
Unique name of this Nat service. The name must be 1-63 characters long and comply with RFC1035.
- Returns:
- value or
null
for none
-
setName
public RouterNat setName(String name)
Unique name of this Nat service. The name must be 1-63 characters long and comply with RFC1035.
- Parameters:
name
- name or null
for none
-
getNatIpAllocateOption
public String getNatIpAllocateOption()
Specify the NatIpAllocateOption, which can take one of the following values: - MANUAL_ONLY:
Uses only Nat IP addresses provided by customers. When there are not enough specified Nat IPs,
the Nat service fails for new VMs. - AUTO_ONLY: Nat IPs are allocated by Google Cloud
Platform; customers can't specify any Nat IPs. When choosing AUTO_ONLY, then nat_ip should be
empty.
- Returns:
- value or
null
for none
-
setNatIpAllocateOption
public RouterNat setNatIpAllocateOption(String natIpAllocateOption)
Specify the NatIpAllocateOption, which can take one of the following values: - MANUAL_ONLY:
Uses only Nat IP addresses provided by customers. When there are not enough specified Nat IPs,
the Nat service fails for new VMs. - AUTO_ONLY: Nat IPs are allocated by Google Cloud
Platform; customers can't specify any Nat IPs. When choosing AUTO_ONLY, then nat_ip should be
empty.
- Parameters:
natIpAllocateOption
- natIpAllocateOption or null
for none
-
getNatIps
public List<String> getNatIps()
A list of URLs of the IP resources used for this Nat service. These IP addresses must be valid
static external IP addresses assigned to the project.
- Returns:
- value or
null
for none
-
setNatIps
public RouterNat setNatIps(List<String> natIps)
A list of URLs of the IP resources used for this Nat service. These IP addresses must be valid
static external IP addresses assigned to the project.
- Parameters:
natIps
- natIps or null
for none
-
getRules
public List<RouterNatRule> getRules()
A list of rules associated with this NAT.
- Returns:
- value or
null
for none
-
setRules
public RouterNat setRules(List<RouterNatRule> rules)
A list of rules associated with this NAT.
- Parameters:
rules
- rules or null
for none
-
getSourceSubnetworkIpRangesToNat
public String getSourceSubnetworkIpRangesToNat()
Specify the Nat option, which can take one of the following values: -
ALL_SUBNETWORKS_ALL_IP_RANGES: All of the IP ranges in every Subnetwork are allowed to Nat. -
ALL_SUBNETWORKS_ALL_PRIMARY_IP_RANGES: All of the primary IP ranges in every Subnetwork are
allowed to Nat. - LIST_OF_SUBNETWORKS: A list of Subnetworks are allowed to Nat (specified in
the field subnetwork below) The default is SUBNETWORK_IP_RANGE_TO_NAT_OPTION_UNSPECIFIED. Note
that if this field contains ALL_SUBNETWORKS_ALL_IP_RANGES or
ALL_SUBNETWORKS_ALL_PRIMARY_IP_RANGES, then there should not be any other Router.Nat section in
any Router for this network in this region.
- Returns:
- value or
null
for none
-
setSourceSubnetworkIpRangesToNat
public RouterNat setSourceSubnetworkIpRangesToNat(String sourceSubnetworkIpRangesToNat)
Specify the Nat option, which can take one of the following values: -
ALL_SUBNETWORKS_ALL_IP_RANGES: All of the IP ranges in every Subnetwork are allowed to Nat. -
ALL_SUBNETWORKS_ALL_PRIMARY_IP_RANGES: All of the primary IP ranges in every Subnetwork are
allowed to Nat. - LIST_OF_SUBNETWORKS: A list of Subnetworks are allowed to Nat (specified in
the field subnetwork below) The default is SUBNETWORK_IP_RANGE_TO_NAT_OPTION_UNSPECIFIED. Note
that if this field contains ALL_SUBNETWORKS_ALL_IP_RANGES or
ALL_SUBNETWORKS_ALL_PRIMARY_IP_RANGES, then there should not be any other Router.Nat section in
any Router for this network in this region.
- Parameters:
sourceSubnetworkIpRangesToNat
- sourceSubnetworkIpRangesToNat or null
for none
-
getSubnetworks
public List<RouterNatSubnetworkToNat> getSubnetworks()
A list of Subnetwork resources whose traffic should be translated by NAT Gateway. It is used
only when LIST_OF_SUBNETWORKS is selected for the SubnetworkIpRangeToNatOption above.
- Returns:
- value or
null
for none
-
setSubnetworks
public RouterNat setSubnetworks(List<RouterNatSubnetworkToNat> subnetworks)
A list of Subnetwork resources whose traffic should be translated by NAT Gateway. It is used
only when LIST_OF_SUBNETWORKS is selected for the SubnetworkIpRangeToNatOption above.
- Parameters:
subnetworks
- subnetworks or null
for none
-
getTcpEstablishedIdleTimeoutSec
public Integer getTcpEstablishedIdleTimeoutSec()
Timeout (in seconds) for TCP established connections. Defaults to 1200s if not set.
- Returns:
- value or
null
for none
-
setTcpEstablishedIdleTimeoutSec
public RouterNat setTcpEstablishedIdleTimeoutSec(Integer tcpEstablishedIdleTimeoutSec)
Timeout (in seconds) for TCP established connections. Defaults to 1200s if not set.
- Parameters:
tcpEstablishedIdleTimeoutSec
- tcpEstablishedIdleTimeoutSec or null
for none
-
getTcpTimeWaitTimeoutSec
public Integer getTcpTimeWaitTimeoutSec()
Timeout (in seconds) for TCP connections that are in TIME_WAIT state. Defaults to 120s if not
set.
- Returns:
- value or
null
for none
-
setTcpTimeWaitTimeoutSec
public RouterNat setTcpTimeWaitTimeoutSec(Integer tcpTimeWaitTimeoutSec)
Timeout (in seconds) for TCP connections that are in TIME_WAIT state. Defaults to 120s if not
set.
- Parameters:
tcpTimeWaitTimeoutSec
- tcpTimeWaitTimeoutSec or null
for none
-
getTcpTransitoryIdleTimeoutSec
public Integer getTcpTransitoryIdleTimeoutSec()
Timeout (in seconds) for TCP transitory connections. Defaults to 30s if not set.
- Returns:
- value or
null
for none
-
setTcpTransitoryIdleTimeoutSec
public RouterNat setTcpTransitoryIdleTimeoutSec(Integer tcpTransitoryIdleTimeoutSec)
Timeout (in seconds) for TCP transitory connections. Defaults to 30s if not set.
- Parameters:
tcpTransitoryIdleTimeoutSec
- tcpTransitoryIdleTimeoutSec or null
for none
-
getUdpIdleTimeoutSec
public Integer getUdpIdleTimeoutSec()
Timeout (in seconds) for UDP connections. Defaults to 30s if not set.
- Returns:
- value or
null
for none
-
setUdpIdleTimeoutSec
public RouterNat setUdpIdleTimeoutSec(Integer udpIdleTimeoutSec)
Timeout (in seconds) for UDP connections. Defaults to 30s if not set.
- Parameters:
udpIdleTimeoutSec
- udpIdleTimeoutSec or null
for none
-
set
public RouterNat set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public RouterNat clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy