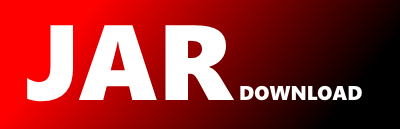
target.apidocs.com.google.api.services.compute.model.UrlMap.html Maven / Gradle / Ivy
UrlMap (Compute Engine API alpha-rev20200526-1.30.9)
com.google.api.services.compute.model
Class UrlMap
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.UrlMap
-
public final class UrlMap
extends GenericJson
Represents a URL Map resource.
Google Compute Engine has two URL Map resources:
* [Global](/compute/docs/reference/rest/{$api_version}/urlMaps) *
[Regional](/compute/docs/reference/rest/{$api_version}/regionUrlMaps)
A URL map resource is a component of certain types of GCP load balancers and Traffic Director.
* urlMaps are used by external HTTP(S) load balancers and Traffic Director. * regionUrlMaps are
used by internal HTTP(S) load balancers.
This resource defines mappings from host names and URL paths to either a backend service or a
backend bucket.
To use the global urlMaps resource, the backend service must have a loadBalancingScheme of either
EXTERNAL or INTERNAL_SELF_MANAGED. To use the regionUrlMaps resource, the backend service must
have a loadBalancingScheme of INTERNAL_MANAGED. For more information, read URL Map Concepts.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
UrlMap()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
UrlMap
clone()
byte[]
decodeFingerprint()
Fingerprint of this resource.
UrlMap
encodeFingerprint(byte[] fingerprint)
Fingerprint of this resource.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
HttpRouteAction
getDefaultRouteAction()
defaultRouteAction takes effect when none of the hostRules match.
String
getDefaultService()
The full or partial URL of the defaultService resource to which traffic is directed if none of
the hostRules match.
HttpRedirectAction
getDefaultUrlRedirect()
When none of the specified hostRules match, the request is redirected to a URL specified by
defaultUrlRedirect.
String
getDescription()
An optional description of this resource.
String
getFingerprint()
Fingerprint of this resource.
HttpHeaderAction
getHeaderAction()
Specifies changes to request and response headers that need to take effect for the selected
backendService.
List<HostRule>
getHostRules()
The list of HostRules to use against the URL.
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getKind()
[Output Only] Type of the resource.
String
getName()
Name of the resource.
List<PathMatcher>
getPathMatchers()
The list of named PathMatchers to use against the URL.
String
getRegion()
[Output Only] URL of the region where the regional URL map resides.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
List<UrlMapTest>
getTests()
The list of expected URL mapping tests.
UrlMap
set(String fieldName,
Object value)
UrlMap
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
UrlMap
setDefaultRouteAction(HttpRouteAction defaultRouteAction)
defaultRouteAction takes effect when none of the hostRules match.
UrlMap
setDefaultService(String defaultService)
The full or partial URL of the defaultService resource to which traffic is directed if none of
the hostRules match.
UrlMap
setDefaultUrlRedirect(HttpRedirectAction defaultUrlRedirect)
When none of the specified hostRules match, the request is redirected to a URL specified by
defaultUrlRedirect.
UrlMap
setDescription(String description)
An optional description of this resource.
UrlMap
setFingerprint(String fingerprint)
Fingerprint of this resource.
UrlMap
setHeaderAction(HttpHeaderAction headerAction)
Specifies changes to request and response headers that need to take effect for the selected
backendService.
UrlMap
setHostRules(List<HostRule> hostRules)
The list of HostRules to use against the URL.
UrlMap
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
UrlMap
setKind(String kind)
[Output Only] Type of the resource.
UrlMap
setName(String name)
Name of the resource.
UrlMap
setPathMatchers(List<PathMatcher> pathMatchers)
The list of named PathMatchers to use against the URL.
UrlMap
setRegion(String region)
[Output Only] URL of the region where the regional URL map resides.
UrlMap
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
UrlMap
setTests(List<UrlMapTest> tests)
The list of expected URL mapping tests.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public UrlMap setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDefaultRouteAction
public HttpRouteAction getDefaultRouteAction()
defaultRouteAction takes effect when none of the hostRules match. The load balancer performs
advanced routing actions like URL rewrites, header transformations, etc. prior to forwarding
the request to the selected backend. If defaultRouteAction specifies any
weightedBackendServices, defaultService must not be set. Conversely if defaultService is set,
defaultRouteAction cannot contain any weightedBackendServices. Only one of defaultRouteAction
or defaultUrlRedirect must be set.
- Returns:
- value or
null
for none
-
setDefaultRouteAction
public UrlMap setDefaultRouteAction(HttpRouteAction defaultRouteAction)
defaultRouteAction takes effect when none of the hostRules match. The load balancer performs
advanced routing actions like URL rewrites, header transformations, etc. prior to forwarding
the request to the selected backend. If defaultRouteAction specifies any
weightedBackendServices, defaultService must not be set. Conversely if defaultService is set,
defaultRouteAction cannot contain any weightedBackendServices. Only one of defaultRouteAction
or defaultUrlRedirect must be set.
- Parameters:
defaultRouteAction
- defaultRouteAction or null
for none
-
getDefaultService
public String getDefaultService()
The full or partial URL of the defaultService resource to which traffic is directed if none of
the hostRules match. If defaultRouteAction is additionally specified, advanced routing actions
like URL Rewrites, etc. take effect prior to sending the request to the backend. However, if
defaultService is specified, defaultRouteAction cannot contain any weightedBackendServices.
Conversely, if routeAction specifies any weightedBackendServices, service must not be
specified. Only one of defaultService, defaultUrlRedirect or
defaultRouteAction.weightedBackendService must be set.
- Returns:
- value or
null
for none
-
setDefaultService
public UrlMap setDefaultService(String defaultService)
The full or partial URL of the defaultService resource to which traffic is directed if none of
the hostRules match. If defaultRouteAction is additionally specified, advanced routing actions
like URL Rewrites, etc. take effect prior to sending the request to the backend. However, if
defaultService is specified, defaultRouteAction cannot contain any weightedBackendServices.
Conversely, if routeAction specifies any weightedBackendServices, service must not be
specified. Only one of defaultService, defaultUrlRedirect or
defaultRouteAction.weightedBackendService must be set.
- Parameters:
defaultService
- defaultService or null
for none
-
getDefaultUrlRedirect
public HttpRedirectAction getDefaultUrlRedirect()
When none of the specified hostRules match, the request is redirected to a URL specified by
defaultUrlRedirect. If defaultUrlRedirect is specified, defaultService or defaultRouteAction
must not be set.
- Returns:
- value or
null
for none
-
setDefaultUrlRedirect
public UrlMap setDefaultUrlRedirect(HttpRedirectAction defaultUrlRedirect)
When none of the specified hostRules match, the request is redirected to a URL specified by
defaultUrlRedirect. If defaultUrlRedirect is specified, defaultService or defaultRouteAction
must not be set.
- Parameters:
defaultUrlRedirect
- defaultUrlRedirect or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public UrlMap setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getFingerprint
public String getFingerprint()
Fingerprint of this resource. A hash of the contents stored in this object. This field is used
in optimistic locking. This field will be ignored when inserting a UrlMap. An up-to-date
fingerprint must be provided in order to update the UrlMap, otherwise the request will fail
with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve a UrlMap.
- Returns:
- value or
null
for none
- See Also:
decodeFingerprint()
-
decodeFingerprint
public byte[] decodeFingerprint()
Fingerprint of this resource. A hash of the contents stored in this object. This field is used
in optimistic locking. This field will be ignored when inserting a UrlMap. An up-to-date
fingerprint must be provided in order to update the UrlMap, otherwise the request will fail
with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve a UrlMap.
- Returns:
- Base64 decoded value or
null
for none
- Since:
- 1.14
- See Also:
getFingerprint()
-
setFingerprint
public UrlMap setFingerprint(String fingerprint)
Fingerprint of this resource. A hash of the contents stored in this object. This field is used
in optimistic locking. This field will be ignored when inserting a UrlMap. An up-to-date
fingerprint must be provided in order to update the UrlMap, otherwise the request will fail
with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve a UrlMap.
- Parameters:
fingerprint
- fingerprint or null
for none
- See Also:
#encodeFingerprint()
-
encodeFingerprint
public UrlMap encodeFingerprint(byte[] fingerprint)
Fingerprint of this resource. A hash of the contents stored in this object. This field is used
in optimistic locking. This field will be ignored when inserting a UrlMap. An up-to-date
fingerprint must be provided in order to update the UrlMap, otherwise the request will fail
with error 412 conditionNotMet.
To see the latest fingerprint, make a get() request to retrieve a UrlMap.
- Since:
- 1.14
- See Also:
The value is encoded Base64 or {@code null} for none.
-
getHeaderAction
public HttpHeaderAction getHeaderAction()
Specifies changes to request and response headers that need to take effect for the selected
backendService. The headerAction specified here take effect after headerAction specified under
pathMatcher.
- Returns:
- value or
null
for none
-
setHeaderAction
public UrlMap setHeaderAction(HttpHeaderAction headerAction)
Specifies changes to request and response headers that need to take effect for the selected
backendService. The headerAction specified here take effect after headerAction specified under
pathMatcher.
- Parameters:
headerAction
- headerAction or null
for none
-
getHostRules
public List<HostRule> getHostRules()
The list of HostRules to use against the URL.
- Returns:
- value or
null
for none
-
setHostRules
public UrlMap setHostRules(List<HostRule> hostRules)
The list of HostRules to use against the URL.
- Parameters:
hostRules
- hostRules or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public UrlMap setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output Only] Type of the resource. Always compute#urlMaps for url maps.
- Returns:
- value or
null
for none
-
setKind
public UrlMap setKind(String kind)
[Output Only] Type of the resource. Always compute#urlMaps for url maps.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Returns:
- value or
null
for none
-
setName
public UrlMap setName(String name)
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Parameters:
name
- name or null
for none
-
getPathMatchers
public List<PathMatcher> getPathMatchers()
The list of named PathMatchers to use against the URL.
- Returns:
- value or
null
for none
-
setPathMatchers
public UrlMap setPathMatchers(List<PathMatcher> pathMatchers)
The list of named PathMatchers to use against the URL.
- Parameters:
pathMatchers
- pathMatchers or null
for none
-
getRegion
public String getRegion()
[Output Only] URL of the region where the regional URL map resides. This field is not
applicable to global URL maps. You must specify this field as part of the HTTP request URL. It
is not settable as a field in the request body.
- Returns:
- value or
null
for none
-
setRegion
public UrlMap setRegion(String region)
[Output Only] URL of the region where the regional URL map resides. This field is not
applicable to global URL maps. You must specify this field as part of the HTTP request URL. It
is not settable as a field in the request body.
- Parameters:
region
- region or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public UrlMap setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getTests
public List<UrlMapTest> getTests()
The list of expected URL mapping tests. Request to update this UrlMap will succeed only if all
of the test cases pass. You can specify a maximum of 100 tests per UrlMap.
- Returns:
- value or
null
for none
-
setTests
public UrlMap setTests(List<UrlMapTest> tests)
The list of expected URL mapping tests. Request to update this UrlMap will succeed only if all
of the test cases pass. You can specify a maximum of 100 tests per UrlMap.
- Parameters:
tests
- tests or null
for none
-
set
public UrlMap set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public UrlMap clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2020 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy