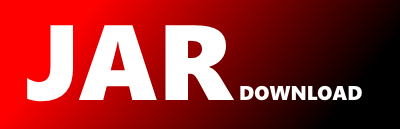
com.google.api.services.compute.model.MachineImage Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Represents a machine image resource. A machine image is a Compute Engine resource that stores all
* the configuration, metadata, permissions, and data from one or more disks required to create a
* Virtual machine (VM) instance. For more information, see Machine images.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class MachineImage extends com.google.api.client.json.GenericJson {
/**
* [Output Only] The creation timestamp for this machine image in RFC3339 text format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creationTimestamp;
/**
* An optional description of this resource. Provide this property when you create the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* [Input Only] Whether to attempt an application consistent machine image by informing the OS to
* prepare for the snapshot process.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean guestFlush;
/**
* [Output Only] A unique identifier for this machine image. The server defines this identifier.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger id;
/**
* [Output Only] Properties of source instance
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private InstanceProperties instanceProperties;
/**
* [Output Only] The resource type, which is always compute#machineImage for machine image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Encrypts the machine image using a customer-supplied encryption key. After you encrypt a
* machine image using a customer-supplied key, you must provide the same key if you use the
* machine image later. For example, you must provide the encryption key when you create an
* instance from the encrypted machine image in a future request. Customer-supplied encryption
* keys do not protect access to metadata of the machine image. If you do not provide an
* encryption key when creating the machine image, then the machine image will be encrypted using
* an automatically generated key and you do not need to provide a key to use the machine image
* later.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CustomerEncryptionKey machineImageEncryptionKey;
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* [Output Only] Reserved for future use.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean satisfiesPzs;
/**
* An array of Machine Image specific properties for disks attached to the source instance
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List savedDisks;
/**
* [Output Only] The URL for this machine image. The server defines this URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* [Input Only] The customer-supplied encryption key of the disks attached to the source instance.
* Required if the source disk is protected by a customer-supplied encryption key.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sourceDiskEncryptionKeys;
/**
* The source instance used to create the machine image. You can provide this as a partial or full
* URL to the resource. For example, the following are valid values: -
* https://www.googleapis.com/compute/v1/projects/project/zones/zone /instances/instance -
* projects/project/zones/zone/instances/instance
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sourceInstance;
/**
* [Output Only] DEPRECATED: Please use instance_properties instead for source instance related
* properties. New properties will not be added to this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SourceInstanceProperties sourceInstanceProperties;
/**
* [Output Only] The status of the machine image. One of the following values: INVALID, CREATING,
* READY, DELETING, and UPLOADING.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* The regional or multi-regional Cloud Storage bucket location where the machine image is stored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List storageLocations;
/**
* [Output Only] Total size of the storage used by the machine image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalStorageBytes;
/**
* [Output Only] The creation timestamp for this machine image in RFC3339 text format.
* @return value or {@code null} for none
*/
public java.lang.String getCreationTimestamp() {
return creationTimestamp;
}
/**
* [Output Only] The creation timestamp for this machine image in RFC3339 text format.
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public MachineImage setCreationTimestamp(java.lang.String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @param description description or {@code null} for none
*/
public MachineImage setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* [Input Only] Whether to attempt an application consistent machine image by informing the OS to
* prepare for the snapshot process.
* @return value or {@code null} for none
*/
public java.lang.Boolean getGuestFlush() {
return guestFlush;
}
/**
* [Input Only] Whether to attempt an application consistent machine image by informing the OS to
* prepare for the snapshot process.
* @param guestFlush guestFlush or {@code null} for none
*/
public MachineImage setGuestFlush(java.lang.Boolean guestFlush) {
this.guestFlush = guestFlush;
return this;
}
/**
* [Output Only] A unique identifier for this machine image. The server defines this identifier.
* @return value or {@code null} for none
*/
public java.math.BigInteger getId() {
return id;
}
/**
* [Output Only] A unique identifier for this machine image. The server defines this identifier.
* @param id id or {@code null} for none
*/
public MachineImage setId(java.math.BigInteger id) {
this.id = id;
return this;
}
/**
* [Output Only] Properties of source instance
* @return value or {@code null} for none
*/
public InstanceProperties getInstanceProperties() {
return instanceProperties;
}
/**
* [Output Only] Properties of source instance
* @param instanceProperties instanceProperties or {@code null} for none
*/
public MachineImage setInstanceProperties(InstanceProperties instanceProperties) {
this.instanceProperties = instanceProperties;
return this;
}
/**
* [Output Only] The resource type, which is always compute#machineImage for machine image.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* [Output Only] The resource type, which is always compute#machineImage for machine image.
* @param kind kind or {@code null} for none
*/
public MachineImage setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Encrypts the machine image using a customer-supplied encryption key. After you encrypt a
* machine image using a customer-supplied key, you must provide the same key if you use the
* machine image later. For example, you must provide the encryption key when you create an
* instance from the encrypted machine image in a future request. Customer-supplied encryption
* keys do not protect access to metadata of the machine image. If you do not provide an
* encryption key when creating the machine image, then the machine image will be encrypted using
* an automatically generated key and you do not need to provide a key to use the machine image
* later.
* @return value or {@code null} for none
*/
public CustomerEncryptionKey getMachineImageEncryptionKey() {
return machineImageEncryptionKey;
}
/**
* Encrypts the machine image using a customer-supplied encryption key. After you encrypt a
* machine image using a customer-supplied key, you must provide the same key if you use the
* machine image later. For example, you must provide the encryption key when you create an
* instance from the encrypted machine image in a future request. Customer-supplied encryption
* keys do not protect access to metadata of the machine image. If you do not provide an
* encryption key when creating the machine image, then the machine image will be encrypted using
* an automatically generated key and you do not need to provide a key to use the machine image
* later.
* @param machineImageEncryptionKey machineImageEncryptionKey or {@code null} for none
*/
public MachineImage setMachineImageEncryptionKey(CustomerEncryptionKey machineImageEncryptionKey) {
this.machineImageEncryptionKey = machineImageEncryptionKey;
return this;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* @param name name or {@code null} for none
*/
public MachineImage setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* [Output Only] Reserved for future use.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSatisfiesPzs() {
return satisfiesPzs;
}
/**
* [Output Only] Reserved for future use.
* @param satisfiesPzs satisfiesPzs or {@code null} for none
*/
public MachineImage setSatisfiesPzs(java.lang.Boolean satisfiesPzs) {
this.satisfiesPzs = satisfiesPzs;
return this;
}
/**
* An array of Machine Image specific properties for disks attached to the source instance
* @return value or {@code null} for none
*/
public java.util.List getSavedDisks() {
return savedDisks;
}
/**
* An array of Machine Image specific properties for disks attached to the source instance
* @param savedDisks savedDisks or {@code null} for none
*/
public MachineImage setSavedDisks(java.util.List savedDisks) {
this.savedDisks = savedDisks;
return this;
}
/**
* [Output Only] The URL for this machine image. The server defines this URL.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* [Output Only] The URL for this machine image. The server defines this URL.
* @param selfLink selfLink or {@code null} for none
*/
public MachineImage setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* [Input Only] The customer-supplied encryption key of the disks attached to the source instance.
* Required if the source disk is protected by a customer-supplied encryption key.
* @return value or {@code null} for none
*/
public java.util.List getSourceDiskEncryptionKeys() {
return sourceDiskEncryptionKeys;
}
/**
* [Input Only] The customer-supplied encryption key of the disks attached to the source instance.
* Required if the source disk is protected by a customer-supplied encryption key.
* @param sourceDiskEncryptionKeys sourceDiskEncryptionKeys or {@code null} for none
*/
public MachineImage setSourceDiskEncryptionKeys(java.util.List sourceDiskEncryptionKeys) {
this.sourceDiskEncryptionKeys = sourceDiskEncryptionKeys;
return this;
}
/**
* The source instance used to create the machine image. You can provide this as a partial or full
* URL to the resource. For example, the following are valid values: -
* https://www.googleapis.com/compute/v1/projects/project/zones/zone /instances/instance -
* projects/project/zones/zone/instances/instance
* @return value or {@code null} for none
*/
public java.lang.String getSourceInstance() {
return sourceInstance;
}
/**
* The source instance used to create the machine image. You can provide this as a partial or full
* URL to the resource. For example, the following are valid values: -
* https://www.googleapis.com/compute/v1/projects/project/zones/zone /instances/instance -
* projects/project/zones/zone/instances/instance
* @param sourceInstance sourceInstance or {@code null} for none
*/
public MachineImage setSourceInstance(java.lang.String sourceInstance) {
this.sourceInstance = sourceInstance;
return this;
}
/**
* [Output Only] DEPRECATED: Please use instance_properties instead for source instance related
* properties. New properties will not be added to this field.
* @return value or {@code null} for none
*/
public SourceInstanceProperties getSourceInstanceProperties() {
return sourceInstanceProperties;
}
/**
* [Output Only] DEPRECATED: Please use instance_properties instead for source instance related
* properties. New properties will not be added to this field.
* @param sourceInstanceProperties sourceInstanceProperties or {@code null} for none
*/
public MachineImage setSourceInstanceProperties(SourceInstanceProperties sourceInstanceProperties) {
this.sourceInstanceProperties = sourceInstanceProperties;
return this;
}
/**
* [Output Only] The status of the machine image. One of the following values: INVALID, CREATING,
* READY, DELETING, and UPLOADING.
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* [Output Only] The status of the machine image. One of the following values: INVALID, CREATING,
* READY, DELETING, and UPLOADING.
* @param status status or {@code null} for none
*/
public MachineImage setStatus(java.lang.String status) {
this.status = status;
return this;
}
/**
* The regional or multi-regional Cloud Storage bucket location where the machine image is stored.
* @return value or {@code null} for none
*/
public java.util.List getStorageLocations() {
return storageLocations;
}
/**
* The regional or multi-regional Cloud Storage bucket location where the machine image is stored.
* @param storageLocations storageLocations or {@code null} for none
*/
public MachineImage setStorageLocations(java.util.List storageLocations) {
this.storageLocations = storageLocations;
return this;
}
/**
* [Output Only] Total size of the storage used by the machine image.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalStorageBytes() {
return totalStorageBytes;
}
/**
* [Output Only] Total size of the storage used by the machine image.
* @param totalStorageBytes totalStorageBytes or {@code null} for none
*/
public MachineImage setTotalStorageBytes(java.lang.Long totalStorageBytes) {
this.totalStorageBytes = totalStorageBytes;
return this;
}
@Override
public MachineImage set(String fieldName, Object value) {
return (MachineImage) super.set(fieldName, value);
}
@Override
public MachineImage clone() {
return (MachineImage) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy