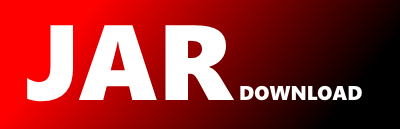
target.apidocs.com.google.api.services.compute.model.Backend.html Maven / Gradle / Ivy
Backend (Compute Engine API beta-rev20220312-1.32.1)
com.google.api.services.compute.model
Class Backend
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.Backend
-
public final class Backend
extends GenericJson
Message containing information of one individual backend.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Backend()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Backend
clone()
String
getBalancingMode()
Specifies how to determine whether the backend of a load balancer can handle additional traffic
or is fully loaded.
Float
getCapacityScaler()
A multiplier applied to the backend's target capacity of its balancing mode.
String
getDescription()
An optional description of this resource.
Boolean
getFailover()
This field designates whether this is a failover backend.
String
getGroup()
The fully-qualified URL of an instance group or network endpoint group (NEG) resource.
Integer
getMaxConnections()
Defines a target maximum number of simultaneous connections.
Integer
getMaxConnectionsPerEndpoint()
Defines a target maximum number of simultaneous connections.
Integer
getMaxConnectionsPerInstance()
Defines a target maximum number of simultaneous connections.
Integer
getMaxRate()
Defines a maximum number of HTTP requests per second (RPS).
Float
getMaxRatePerEndpoint()
Defines a maximum target for requests per second (RPS).
Float
getMaxRatePerInstance()
Defines a maximum target for requests per second (RPS).
Float
getMaxUtilization()
Optional parameter to define a target capacity for the UTILIZATIONbalancing mode.
Backend
set(String fieldName,
Object value)
Backend
setBalancingMode(String balancingMode)
Specifies how to determine whether the backend of a load balancer can handle additional traffic
or is fully loaded.
Backend
setCapacityScaler(Float capacityScaler)
A multiplier applied to the backend's target capacity of its balancing mode.
Backend
setDescription(String description)
An optional description of this resource.
Backend
setFailover(Boolean failover)
This field designates whether this is a failover backend.
Backend
setGroup(String group)
The fully-qualified URL of an instance group or network endpoint group (NEG) resource.
Backend
setMaxConnections(Integer maxConnections)
Defines a target maximum number of simultaneous connections.
Backend
setMaxConnectionsPerEndpoint(Integer maxConnectionsPerEndpoint)
Defines a target maximum number of simultaneous connections.
Backend
setMaxConnectionsPerInstance(Integer maxConnectionsPerInstance)
Defines a target maximum number of simultaneous connections.
Backend
setMaxRate(Integer maxRate)
Defines a maximum number of HTTP requests per second (RPS).
Backend
setMaxRatePerEndpoint(Float maxRatePerEndpoint)
Defines a maximum target for requests per second (RPS).
Backend
setMaxRatePerInstance(Float maxRatePerInstance)
Defines a maximum target for requests per second (RPS).
Backend
setMaxUtilization(Float maxUtilization)
Optional parameter to define a target capacity for the UTILIZATIONbalancing mode.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getBalancingMode
public String getBalancingMode()
Specifies how to determine whether the backend of a load balancer can handle additional traffic
or is fully loaded. For usage guidelines, see Connection balancing mode. Backends must use
compatible balancing modes. For more information, see Supported balancing modes and target
capacity settings and Restrictions and guidance for instance groups. Note: Currently, if you
use the API to configure incompatible balancing modes, the configuration might be accepted even
though it has no impact and is ignored. Specifically, Backend.maxUtilization is ignored when
Backend.balancingMode is RATE. In the future, this incompatible combination will be rejected.
- Returns:
- value or
null
for none
-
setBalancingMode
public Backend setBalancingMode(String balancingMode)
Specifies how to determine whether the backend of a load balancer can handle additional traffic
or is fully loaded. For usage guidelines, see Connection balancing mode. Backends must use
compatible balancing modes. For more information, see Supported balancing modes and target
capacity settings and Restrictions and guidance for instance groups. Note: Currently, if you
use the API to configure incompatible balancing modes, the configuration might be accepted even
though it has no impact and is ignored. Specifically, Backend.maxUtilization is ignored when
Backend.balancingMode is RATE. In the future, this incompatible combination will be rejected.
- Parameters:
balancingMode
- balancingMode or null
for none
-
getCapacityScaler
public Float getCapacityScaler()
A multiplier applied to the backend's target capacity of its balancing mode. The default value
is 1, which means the group serves up to 100% of its configured capacity (depending on
balancingMode). A setting of 0 means the group is completely drained, offering 0% of its
available capacity. The valid ranges are 0.0 and [0.1,1.0]. You cannot configure a setting
larger than 0 and smaller than 0.1. You cannot configure a setting of 0 when there is only one
backend attached to the backend service.
- Returns:
- value or
null
for none
-
setCapacityScaler
public Backend setCapacityScaler(Float capacityScaler)
A multiplier applied to the backend's target capacity of its balancing mode. The default value
is 1, which means the group serves up to 100% of its configured capacity (depending on
balancingMode). A setting of 0 means the group is completely drained, offering 0% of its
available capacity. The valid ranges are 0.0 and [0.1,1.0]. You cannot configure a setting
larger than 0 and smaller than 0.1. You cannot configure a setting of 0 when there is only one
backend attached to the backend service.
- Parameters:
capacityScaler
- capacityScaler or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public Backend setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getFailover
public Boolean getFailover()
This field designates whether this is a failover backend. More than one failover backend can be
configured for a given BackendService.
- Returns:
- value or
null
for none
-
setFailover
public Backend setFailover(Boolean failover)
This field designates whether this is a failover backend. More than one failover backend can be
configured for a given BackendService.
- Parameters:
failover
- failover or null
for none
-
getGroup
public String getGroup()
The fully-qualified URL of an instance group or network endpoint group (NEG) resource. To
determine what types of backends a load balancer supports, see the [Backend services
overview](https://cloud.google.com/load-balancing/docs/backend-service#backends). You must use
the *fully-qualified* URL (starting with https://www.googleapis.com/) to specify the instance
group or NEG. Partial URLs are not supported.
- Returns:
- value or
null
for none
-
setGroup
public Backend setGroup(String group)
The fully-qualified URL of an instance group or network endpoint group (NEG) resource. To
determine what types of backends a load balancer supports, see the [Backend services
overview](https://cloud.google.com/load-balancing/docs/backend-service#backends). You must use
the *fully-qualified* URL (starting with https://www.googleapis.com/) to specify the instance
group or NEG. Partial URLs are not supported.
- Parameters:
group
- group or null
for none
-
getMaxConnections
public Integer getMaxConnections()
Defines a target maximum number of simultaneous connections. For usage guidelines, see
Connection balancing mode and Utilization balancing mode. Not available if the backend's
balancingMode is RATE.
- Returns:
- value or
null
for none
-
setMaxConnections
public Backend setMaxConnections(Integer maxConnections)
Defines a target maximum number of simultaneous connections. For usage guidelines, see
Connection balancing mode and Utilization balancing mode. Not available if the backend's
balancingMode is RATE.
- Parameters:
maxConnections
- maxConnections or null
for none
-
getMaxConnectionsPerEndpoint
public Integer getMaxConnectionsPerEndpoint()
Defines a target maximum number of simultaneous connections. For usage guidelines, see
Connection balancing mode and Utilization balancing mode. Not available if the backend's
balancingMode is RATE.
- Returns:
- value or
null
for none
-
setMaxConnectionsPerEndpoint
public Backend setMaxConnectionsPerEndpoint(Integer maxConnectionsPerEndpoint)
Defines a target maximum number of simultaneous connections. For usage guidelines, see
Connection balancing mode and Utilization balancing mode. Not available if the backend's
balancingMode is RATE.
- Parameters:
maxConnectionsPerEndpoint
- maxConnectionsPerEndpoint or null
for none
-
getMaxConnectionsPerInstance
public Integer getMaxConnectionsPerInstance()
Defines a target maximum number of simultaneous connections. For usage guidelines, see
Connection balancing mode and Utilization balancing mode. Not available if the backend's
balancingMode is RATE.
- Returns:
- value or
null
for none
-
setMaxConnectionsPerInstance
public Backend setMaxConnectionsPerInstance(Integer maxConnectionsPerInstance)
Defines a target maximum number of simultaneous connections. For usage guidelines, see
Connection balancing mode and Utilization balancing mode. Not available if the backend's
balancingMode is RATE.
- Parameters:
maxConnectionsPerInstance
- maxConnectionsPerInstance or null
for none
-
getMaxRate
public Integer getMaxRate()
Defines a maximum number of HTTP requests per second (RPS). For usage guidelines, see Rate
balancing mode and Utilization balancing mode. Not available if the backend's balancingMode is
CONNECTION.
- Returns:
- value or
null
for none
-
setMaxRate
public Backend setMaxRate(Integer maxRate)
Defines a maximum number of HTTP requests per second (RPS). For usage guidelines, see Rate
balancing mode and Utilization balancing mode. Not available if the backend's balancingMode is
CONNECTION.
- Parameters:
maxRate
- maxRate or null
for none
-
getMaxRatePerEndpoint
public Float getMaxRatePerEndpoint()
Defines a maximum target for requests per second (RPS). For usage guidelines, see Rate
balancing mode and Utilization balancing mode. Not available if the backend's balancingMode is
CONNECTION.
- Returns:
- value or
null
for none
-
setMaxRatePerEndpoint
public Backend setMaxRatePerEndpoint(Float maxRatePerEndpoint)
Defines a maximum target for requests per second (RPS). For usage guidelines, see Rate
balancing mode and Utilization balancing mode. Not available if the backend's balancingMode is
CONNECTION.
- Parameters:
maxRatePerEndpoint
- maxRatePerEndpoint or null
for none
-
getMaxRatePerInstance
public Float getMaxRatePerInstance()
Defines a maximum target for requests per second (RPS). For usage guidelines, see Rate
balancing mode and Utilization balancing mode. Not available if the backend's balancingMode is
CONNECTION.
- Returns:
- value or
null
for none
-
setMaxRatePerInstance
public Backend setMaxRatePerInstance(Float maxRatePerInstance)
Defines a maximum target for requests per second (RPS). For usage guidelines, see Rate
balancing mode and Utilization balancing mode. Not available if the backend's balancingMode is
CONNECTION.
- Parameters:
maxRatePerInstance
- maxRatePerInstance or null
for none
-
getMaxUtilization
public Float getMaxUtilization()
Optional parameter to define a target capacity for the UTILIZATIONbalancing mode. The valid
range is [0.0, 1.0]. For usage guidelines, see Utilization balancing mode.
- Returns:
- value or
null
for none
-
setMaxUtilization
public Backend setMaxUtilization(Float maxUtilization)
Optional parameter to define a target capacity for the UTILIZATIONbalancing mode. The valid
range is [0.0, 1.0]. For usage guidelines, see Utilization balancing mode.
- Parameters:
maxUtilization
- maxUtilization or null
for none
-
set
public Backend set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Backend clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy