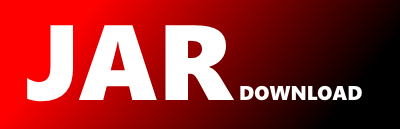
target.apidocs.com.google.api.services.compute.model.NodeGroup.html Maven / Gradle / Ivy
NodeGroup (Compute Engine API beta-rev20220312-1.32.1)
com.google.api.services.compute.model
Class NodeGroup
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.NodeGroup
-
public final class NodeGroup
extends GenericJson
Represents a sole-tenant Node Group resource. A sole-tenant node is a physical server that is
dedicated to hosting VM instances only for your specific project. Use sole-tenant nodes to keep
your instances physically separated from instances in other projects, or to group your instances
together on the same host hardware. For more information, read Sole-tenant nodes.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
NodeGroup()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
NodeGroup
clone()
byte[]
decodeFingerprint()
NodeGroup
encodeFingerprint(byte[] fingerprint)
NodeGroupAutoscalingPolicy
getAutoscalingPolicy()
Specifies how autoscaling should behave.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional description of this resource.
String
getFingerprint()
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getKind()
[Output Only] The type of the resource.
String
getLocationHint()
An opaque location hint used to place the Node close to other resources.
String
getMaintenancePolicy()
Specifies how to handle instances when a node in the group undergoes maintenance.
NodeGroupMaintenanceWindow
getMaintenanceWindow()
String
getName()
The name of the resource, provided by the client when initially creating the resource.
String
getNodeTemplate()
URL of the node template to create the node group from.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
ShareSettings
getShareSettings()
Share-settings for the node group
Integer
getSize()
[Output Only] The total number of nodes in the node group.
String
getStatus()
String
getZone()
[Output Only] The name of the zone where the node group resides, such as us-central1-a.
NodeGroup
set(String fieldName,
Object value)
NodeGroup
setAutoscalingPolicy(NodeGroupAutoscalingPolicy autoscalingPolicy)
Specifies how autoscaling should behave.
NodeGroup
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
NodeGroup
setDescription(String description)
An optional description of this resource.
NodeGroup
setFingerprint(String fingerprint)
NodeGroup
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
NodeGroup
setKind(String kind)
[Output Only] The type of the resource.
NodeGroup
setLocationHint(String locationHint)
An opaque location hint used to place the Node close to other resources.
NodeGroup
setMaintenancePolicy(String maintenancePolicy)
Specifies how to handle instances when a node in the group undergoes maintenance.
NodeGroup
setMaintenanceWindow(NodeGroupMaintenanceWindow maintenanceWindow)
NodeGroup
setName(String name)
The name of the resource, provided by the client when initially creating the resource.
NodeGroup
setNodeTemplate(String nodeTemplate)
URL of the node template to create the node group from.
NodeGroup
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
NodeGroup
setShareSettings(ShareSettings shareSettings)
Share-settings for the node group
NodeGroup
setSize(Integer size)
[Output Only] The total number of nodes in the node group.
NodeGroup
setStatus(String status)
NodeGroup
setZone(String zone)
[Output Only] The name of the zone where the node group resides, such as us-central1-a.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAutoscalingPolicy
public NodeGroupAutoscalingPolicy getAutoscalingPolicy()
Specifies how autoscaling should behave.
- Returns:
- value or
null
for none
-
setAutoscalingPolicy
public NodeGroup setAutoscalingPolicy(NodeGroupAutoscalingPolicy autoscalingPolicy)
Specifies how autoscaling should behave.
- Parameters:
autoscalingPolicy
- autoscalingPolicy or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public NodeGroup setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public NodeGroup setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getFingerprint
public String getFingerprint()
- Returns:
- value or
null
for none
- See Also:
decodeFingerprint()
-
decodeFingerprint
public byte[] decodeFingerprint()
- Returns:
- Base64 decoded value or
null
for none
- Since:
- 1.14
- See Also:
getFingerprint()
-
setFingerprint
public NodeGroup setFingerprint(String fingerprint)
- Parameters:
fingerprint
- fingerprint or null
for none
- See Also:
#encodeFingerprint()
-
encodeFingerprint
public NodeGroup encodeFingerprint(byte[] fingerprint)
- Since:
- 1.14
- See Also:
The value is encoded Base64 or {@code null} for none.
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public NodeGroup setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output Only] The type of the resource. Always compute#nodeGroup for node group.
- Returns:
- value or
null
for none
-
setKind
public NodeGroup setKind(String kind)
[Output Only] The type of the resource. Always compute#nodeGroup for node group.
- Parameters:
kind
- kind or null
for none
-
getLocationHint
public String getLocationHint()
An opaque location hint used to place the Node close to other resources. This field is for use
by internal tools that use the public API. The location hint here on the NodeGroup overrides
any location_hint present in the NodeTemplate.
- Returns:
- value or
null
for none
-
setLocationHint
public NodeGroup setLocationHint(String locationHint)
An opaque location hint used to place the Node close to other resources. This field is for use
by internal tools that use the public API. The location hint here on the NodeGroup overrides
any location_hint present in the NodeTemplate.
- Parameters:
locationHint
- locationHint or null
for none
-
getMaintenancePolicy
public String getMaintenancePolicy()
Specifies how to handle instances when a node in the group undergoes maintenance. Set to one
of: DEFAULT, RESTART_IN_PLACE, or MIGRATE_WITHIN_NODE_GROUP. The default value is DEFAULT. For
more information, see Maintenance policies.
- Returns:
- value or
null
for none
-
setMaintenancePolicy
public NodeGroup setMaintenancePolicy(String maintenancePolicy)
Specifies how to handle instances when a node in the group undergoes maintenance. Set to one
of: DEFAULT, RESTART_IN_PLACE, or MIGRATE_WITHIN_NODE_GROUP. The default value is DEFAULT. For
more information, see Maintenance policies.
- Parameters:
maintenancePolicy
- maintenancePolicy or null
for none
-
getMaintenanceWindow
public NodeGroupMaintenanceWindow getMaintenanceWindow()
- Returns:
- value or
null
for none
-
setMaintenanceWindow
public NodeGroup setMaintenanceWindow(NodeGroupMaintenanceWindow maintenanceWindow)
- Parameters:
maintenanceWindow
- maintenanceWindow or null
for none
-
getName
public String getName()
The name of the resource, provided by the client when initially creating the resource. The
resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name
must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`
which means the first character must be a lowercase letter, and all following characters must
be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
- Returns:
- value or
null
for none
-
setName
public NodeGroup setName(String name)
The name of the resource, provided by the client when initially creating the resource. The
resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name
must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`
which means the first character must be a lowercase letter, and all following characters must
be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
- Parameters:
name
- name or null
for none
-
getNodeTemplate
public String getNodeTemplate()
URL of the node template to create the node group from.
- Returns:
- value or
null
for none
-
setNodeTemplate
public NodeGroup setNodeTemplate(String nodeTemplate)
URL of the node template to create the node group from.
- Parameters:
nodeTemplate
- nodeTemplate or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public NodeGroup setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getShareSettings
public ShareSettings getShareSettings()
Share-settings for the node group
- Returns:
- value or
null
for none
-
setShareSettings
public NodeGroup setShareSettings(ShareSettings shareSettings)
Share-settings for the node group
- Parameters:
shareSettings
- shareSettings or null
for none
-
getSize
public Integer getSize()
[Output Only] The total number of nodes in the node group.
- Returns:
- value or
null
for none
-
setSize
public NodeGroup setSize(Integer size)
[Output Only] The total number of nodes in the node group.
- Parameters:
size
- size or null
for none
-
getStatus
public String getStatus()
- Returns:
- value or
null
for none
-
getZone
public String getZone()
[Output Only] The name of the zone where the node group resides, such as us-central1-a.
- Returns:
- value or
null
for none
-
setZone
public NodeGroup setZone(String zone)
[Output Only] The name of the zone where the node group resides, such as us-central1-a.
- Parameters:
zone
- zone or null
for none
-
set
public NodeGroup set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public NodeGroup clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy