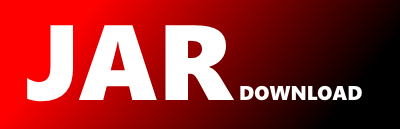
target.apidocs.com.google.api.services.compute.model.NodeTemplate.html Maven / Gradle / Ivy
NodeTemplate (Compute Engine API beta-rev20220312-1.32.1)
com.google.api.services.compute.model
Class NodeTemplate
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.NodeTemplate
-
public final class NodeTemplate
extends GenericJson
Represent a sole-tenant Node Template resource. You can use a template to define properties for
nodes in a node group. For more information, read Creating node groups and instances.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
NodeTemplate()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
NodeTemplate
clone()
List<AcceleratorConfig>
getAccelerators()
String
getCpuOvercommitType()
CPU overcommit.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional description of this resource.
List<LocalDisk>
getDisks()
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getKind()
[Output Only] The type of the resource.
String
getName()
The name of the resource, provided by the client when initially creating the resource.
Map<String,String>
getNodeAffinityLabels()
Labels to use for node affinity, which will be used in instance scheduling.
String
getNodeType()
The node type to use for nodes group that are created from this template.
NodeTemplateNodeTypeFlexibility
getNodeTypeFlexibility()
The flexible properties of the desired node type.
String
getRegion()
[Output Only] The name of the region where the node template resides, such as us-central1.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
ServerBinding
getServerBinding()
Sets the binding properties for the physical server.
String
getStatus()
[Output Only] The status of the node template.
String
getStatusMessage()
[Output Only] An optional, human-readable explanation of the status.
NodeTemplate
set(String fieldName,
Object value)
NodeTemplate
setAccelerators(List<AcceleratorConfig> accelerators)
NodeTemplate
setCpuOvercommitType(String cpuOvercommitType)
CPU overcommit.
NodeTemplate
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
NodeTemplate
setDescription(String description)
An optional description of this resource.
NodeTemplate
setDisks(List<LocalDisk> disks)
NodeTemplate
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
NodeTemplate
setKind(String kind)
[Output Only] The type of the resource.
NodeTemplate
setName(String name)
The name of the resource, provided by the client when initially creating the resource.
NodeTemplate
setNodeAffinityLabels(Map<String,String> nodeAffinityLabels)
Labels to use for node affinity, which will be used in instance scheduling.
NodeTemplate
setNodeType(String nodeType)
The node type to use for nodes group that are created from this template.
NodeTemplate
setNodeTypeFlexibility(NodeTemplateNodeTypeFlexibility nodeTypeFlexibility)
The flexible properties of the desired node type.
NodeTemplate
setRegion(String region)
[Output Only] The name of the region where the node template resides, such as us-central1.
NodeTemplate
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
NodeTemplate
setServerBinding(ServerBinding serverBinding)
Sets the binding properties for the physical server.
NodeTemplate
setStatus(String status)
[Output Only] The status of the node template.
NodeTemplate
setStatusMessage(String statusMessage)
[Output Only] An optional, human-readable explanation of the status.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAccelerators
public List<AcceleratorConfig> getAccelerators()
- Returns:
- value or
null
for none
-
setAccelerators
public NodeTemplate setAccelerators(List<AcceleratorConfig> accelerators)
- Parameters:
accelerators
- accelerators or null
for none
-
getCpuOvercommitType
public String getCpuOvercommitType()
CPU overcommit.
- Returns:
- value or
null
for none
-
setCpuOvercommitType
public NodeTemplate setCpuOvercommitType(String cpuOvercommitType)
CPU overcommit.
- Parameters:
cpuOvercommitType
- cpuOvercommitType or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public NodeTemplate setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public NodeTemplate setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
setDisks
public NodeTemplate setDisks(List<LocalDisk> disks)
- Parameters:
disks
- disks or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public NodeTemplate setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output Only] The type of the resource. Always compute#nodeTemplate for node templates.
- Returns:
- value or
null
for none
-
setKind
public NodeTemplate setKind(String kind)
[Output Only] The type of the resource. Always compute#nodeTemplate for node templates.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
The name of the resource, provided by the client when initially creating the resource. The
resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name
must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`
which means the first character must be a lowercase letter, and all following characters must
be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
- Returns:
- value or
null
for none
-
setName
public NodeTemplate setName(String name)
The name of the resource, provided by the client when initially creating the resource. The
resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name
must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`
which means the first character must be a lowercase letter, and all following characters must
be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
- Parameters:
name
- name or null
for none
-
getNodeAffinityLabels
public Map<String,String> getNodeAffinityLabels()
Labels to use for node affinity, which will be used in instance scheduling.
- Returns:
- value or
null
for none
-
setNodeAffinityLabels
public NodeTemplate setNodeAffinityLabels(Map<String,String> nodeAffinityLabels)
Labels to use for node affinity, which will be used in instance scheduling.
- Parameters:
nodeAffinityLabels
- nodeAffinityLabels or null
for none
-
getNodeType
public String getNodeType()
The node type to use for nodes group that are created from this template.
- Returns:
- value or
null
for none
-
setNodeType
public NodeTemplate setNodeType(String nodeType)
The node type to use for nodes group that are created from this template.
- Parameters:
nodeType
- nodeType or null
for none
-
getNodeTypeFlexibility
public NodeTemplateNodeTypeFlexibility getNodeTypeFlexibility()
The flexible properties of the desired node type. Node groups that use this node template will
create nodes of a type that matches these properties. This field is mutually exclusive with the
node_type property; you can only define one or the other, but not both.
- Returns:
- value or
null
for none
-
setNodeTypeFlexibility
public NodeTemplate setNodeTypeFlexibility(NodeTemplateNodeTypeFlexibility nodeTypeFlexibility)
The flexible properties of the desired node type. Node groups that use this node template will
create nodes of a type that matches these properties. This field is mutually exclusive with the
node_type property; you can only define one or the other, but not both.
- Parameters:
nodeTypeFlexibility
- nodeTypeFlexibility or null
for none
-
getRegion
public String getRegion()
[Output Only] The name of the region where the node template resides, such as us-central1.
- Returns:
- value or
null
for none
-
setRegion
public NodeTemplate setRegion(String region)
[Output Only] The name of the region where the node template resides, such as us-central1.
- Parameters:
region
- region or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public NodeTemplate setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getServerBinding
public ServerBinding getServerBinding()
Sets the binding properties for the physical server. Valid values include: - *[Default]*
RESTART_NODE_ON_ANY_SERVER: Restarts VMs on any available physical server -
RESTART_NODE_ON_MINIMAL_SERVER: Restarts VMs on the same physical server whenever possible See
Sole-tenant node options for more information.
- Returns:
- value or
null
for none
-
setServerBinding
public NodeTemplate setServerBinding(ServerBinding serverBinding)
Sets the binding properties for the physical server. Valid values include: - *[Default]*
RESTART_NODE_ON_ANY_SERVER: Restarts VMs on any available physical server -
RESTART_NODE_ON_MINIMAL_SERVER: Restarts VMs on the same physical server whenever possible See
Sole-tenant node options for more information.
- Parameters:
serverBinding
- serverBinding or null
for none
-
getStatus
public String getStatus()
[Output Only] The status of the node template. One of the following values: CREATING, READY,
and DELETING.
- Returns:
- value or
null
for none
-
setStatus
public NodeTemplate setStatus(String status)
[Output Only] The status of the node template. One of the following values: CREATING, READY,
and DELETING.
- Parameters:
status
- status or null
for none
-
getStatusMessage
public String getStatusMessage()
[Output Only] An optional, human-readable explanation of the status.
- Returns:
- value or
null
for none
-
setStatusMessage
public NodeTemplate setStatusMessage(String statusMessage)
[Output Only] An optional, human-readable explanation of the status.
- Parameters:
statusMessage
- statusMessage or null
for none
-
set
public NodeTemplate set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public NodeTemplate clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy