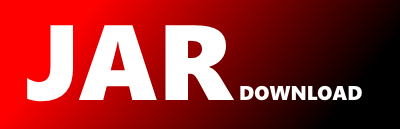
target.apidocs.com.google.api.services.compute.model.Operation.html Maven / Gradle / Ivy
Operation (Compute Engine API beta-rev20220312-1.32.1)
com.google.api.services.compute.model
Class Operation
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.Operation
-
public final class Operation
extends GenericJson
Represents an Operation resource. Google Compute Engine has three Operation resources: *
[Global](/compute/docs/reference/rest/beta/globalOperations) *
[Regional](/compute/docs/reference/rest/beta/regionOperations) *
[Zonal](/compute/docs/reference/rest/beta/zoneOperations) You can use an operation resource to
manage asynchronous API requests. For more information, read Handling API responses. Operations
can be global, regional or zonal. - For global operations, use the `globalOperations` resource. -
For regional operations, use the `regionOperations` resource. - For zonal operations, use the
`zonalOperations` resource. For more information, read Global, Regional, and Zonal Resources.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
Operation.Error
[Output Only] If errors are generated during processing of the operation, this field will be
populated.
static class
Operation.Warnings
Model definition for OperationWarnings.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Operation()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Operation
clone()
String
getClientOperationId()
[Output Only] The value of `requestId` if you provided it in the request.
String
getCreationTimestamp()
[Deprecated] This field is deprecated.
String
getDescription()
[Output Only] A textual description of the operation, which is set when the operation is
created.
String
getEndTime()
[Output Only] The time that this operation was completed.
Operation.Error
getError()
[Output Only] If errors are generated during processing of the operation, this field will be
populated.
String
getHttpErrorMessage()
[Output Only] If the operation fails, this field contains the HTTP error message that was
returned, such as `NOT FOUND`.
Integer
getHttpErrorStatusCode()
[Output Only] If the operation fails, this field contains the HTTP error status code that was
returned.
BigInteger
getId()
[Output Only] The unique identifier for the operation.
String
getInsertTime()
[Output Only] The time that this operation was requested.
String
getKind()
[Output Only] Type of the resource.
String
getName()
[Output Only] Name of the operation.
String
getOperationGroupId()
[Output Only] An ID that represents a group of operations, such as when a group of operations
results from a `bulkInsert` API request.
String
getOperationType()
[Output Only] The type of operation, such as `insert`, `update`, or `delete`, and so on.
Integer
getProgress()
[Output Only] An optional progress indicator that ranges from 0 to 100.
String
getRegion()
[Output Only] The URL of the region where the operation resides.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
String
getStartTime()
[Output Only] The time that this operation was started by the server.
String
getStatus()
[Output Only] The status of the operation, which can be one of the following: `PENDING`,
`RUNNING`, or `DONE`.
String
getStatusMessage()
[Output Only] An optional textual description of the current status of the operation.
BigInteger
getTargetId()
[Output Only] The unique target ID, which identifies a specific incarnation of the target
resource.
String
getTargetLink()
[Output Only] The URL of the resource that the operation modifies.
String
getUser()
[Output Only] User who requested the operation, for example: `[email protected]`.
List<Operation.Warnings>
getWarnings()
[Output Only] If warning messages are generated during processing of the operation, this field
will be populated.
String
getZone()
[Output Only] The URL of the zone where the operation resides.
Operation
set(String fieldName,
Object value)
Operation
setClientOperationId(String clientOperationId)
[Output Only] The value of `requestId` if you provided it in the request.
Operation
setCreationTimestamp(String creationTimestamp)
[Deprecated] This field is deprecated.
Operation
setDescription(String description)
[Output Only] A textual description of the operation, which is set when the operation is
created.
Operation
setEndTime(String endTime)
[Output Only] The time that this operation was completed.
Operation
setError(Operation.Error error)
[Output Only] If errors are generated during processing of the operation, this field will be
populated.
Operation
setHttpErrorMessage(String httpErrorMessage)
[Output Only] If the operation fails, this field contains the HTTP error message that was
returned, such as `NOT FOUND`.
Operation
setHttpErrorStatusCode(Integer httpErrorStatusCode)
[Output Only] If the operation fails, this field contains the HTTP error status code that was
returned.
Operation
setId(BigInteger id)
[Output Only] The unique identifier for the operation.
Operation
setInsertTime(String insertTime)
[Output Only] The time that this operation was requested.
Operation
setKind(String kind)
[Output Only] Type of the resource.
Operation
setName(String name)
[Output Only] Name of the operation.
Operation
setOperationGroupId(String operationGroupId)
[Output Only] An ID that represents a group of operations, such as when a group of operations
results from a `bulkInsert` API request.
Operation
setOperationType(String operationType)
[Output Only] The type of operation, such as `insert`, `update`, or `delete`, and so on.
Operation
setProgress(Integer progress)
[Output Only] An optional progress indicator that ranges from 0 to 100.
Operation
setRegion(String region)
[Output Only] The URL of the region where the operation resides.
Operation
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
Operation
setStartTime(String startTime)
[Output Only] The time that this operation was started by the server.
Operation
setStatus(String status)
[Output Only] The status of the operation, which can be one of the following: `PENDING`,
`RUNNING`, or `DONE`.
Operation
setStatusMessage(String statusMessage)
[Output Only] An optional textual description of the current status of the operation.
Operation
setTargetId(BigInteger targetId)
[Output Only] The unique target ID, which identifies a specific incarnation of the target
resource.
Operation
setTargetLink(String targetLink)
[Output Only] The URL of the resource that the operation modifies.
Operation
setUser(String user)
[Output Only] User who requested the operation, for example: `[email protected]`.
Operation
setWarnings(List<Operation.Warnings> warnings)
[Output Only] If warning messages are generated during processing of the operation, this field
will be populated.
Operation
setZone(String zone)
[Output Only] The URL of the zone where the operation resides.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getClientOperationId
public String getClientOperationId()
[Output Only] The value of `requestId` if you provided it in the request. Not present
otherwise.
- Returns:
- value or
null
for none
-
setClientOperationId
public Operation setClientOperationId(String clientOperationId)
[Output Only] The value of `requestId` if you provided it in the request. Not present
otherwise.
- Parameters:
clientOperationId
- clientOperationId or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Deprecated] This field is deprecated.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public Operation setCreationTimestamp(String creationTimestamp)
[Deprecated] This field is deprecated.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
[Output Only] A textual description of the operation, which is set when the operation is
created.
- Returns:
- value or
null
for none
-
setDescription
public Operation setDescription(String description)
[Output Only] A textual description of the operation, which is set when the operation is
created.
- Parameters:
description
- description or null
for none
-
getEndTime
public String getEndTime()
[Output Only] The time that this operation was completed. This value is in RFC3339 text format.
- Returns:
- value or
null
for none
-
setEndTime
public Operation setEndTime(String endTime)
[Output Only] The time that this operation was completed. This value is in RFC3339 text format.
- Parameters:
endTime
- endTime or null
for none
-
getError
public Operation.Error getError()
[Output Only] If errors are generated during processing of the operation, this field will be
populated.
- Returns:
- value or
null
for none
-
setError
public Operation setError(Operation.Error error)
[Output Only] If errors are generated during processing of the operation, this field will be
populated.
- Parameters:
error
- error or null
for none
-
getHttpErrorMessage
public String getHttpErrorMessage()
[Output Only] If the operation fails, this field contains the HTTP error message that was
returned, such as `NOT FOUND`.
- Returns:
- value or
null
for none
-
setHttpErrorMessage
public Operation setHttpErrorMessage(String httpErrorMessage)
[Output Only] If the operation fails, this field contains the HTTP error message that was
returned, such as `NOT FOUND`.
- Parameters:
httpErrorMessage
- httpErrorMessage or null
for none
-
getHttpErrorStatusCode
public Integer getHttpErrorStatusCode()
[Output Only] If the operation fails, this field contains the HTTP error status code that was
returned. For example, a `404` means the resource was not found.
- Returns:
- value or
null
for none
-
setHttpErrorStatusCode
public Operation setHttpErrorStatusCode(Integer httpErrorStatusCode)
[Output Only] If the operation fails, this field contains the HTTP error status code that was
returned. For example, a `404` means the resource was not found.
- Parameters:
httpErrorStatusCode
- httpErrorStatusCode or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the operation. This identifier is defined by the
server.
- Returns:
- value or
null
for none
-
setId
public Operation setId(BigInteger id)
[Output Only] The unique identifier for the operation. This identifier is defined by the
server.
- Parameters:
id
- id or null
for none
-
getInsertTime
public String getInsertTime()
[Output Only] The time that this operation was requested. This value is in RFC3339 text format.
- Returns:
- value or
null
for none
-
setInsertTime
public Operation setInsertTime(String insertTime)
[Output Only] The time that this operation was requested. This value is in RFC3339 text format.
- Parameters:
insertTime
- insertTime or null
for none
-
getKind
public String getKind()
[Output Only] Type of the resource. Always `compute#operation` for Operation resources.
- Returns:
- value or
null
for none
-
setKind
public Operation setKind(String kind)
[Output Only] Type of the resource. Always `compute#operation` for Operation resources.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
[Output Only] Name of the operation.
- Returns:
- value or
null
for none
-
setName
public Operation setName(String name)
[Output Only] Name of the operation.
- Parameters:
name
- name or null
for none
-
getOperationGroupId
public String getOperationGroupId()
[Output Only] An ID that represents a group of operations, such as when a group of operations
results from a `bulkInsert` API request.
- Returns:
- value or
null
for none
-
setOperationGroupId
public Operation setOperationGroupId(String operationGroupId)
[Output Only] An ID that represents a group of operations, such as when a group of operations
results from a `bulkInsert` API request.
- Parameters:
operationGroupId
- operationGroupId or null
for none
-
getOperationType
public String getOperationType()
[Output Only] The type of operation, such as `insert`, `update`, or `delete`, and so on.
- Returns:
- value or
null
for none
-
setOperationType
public Operation setOperationType(String operationType)
[Output Only] The type of operation, such as `insert`, `update`, or `delete`, and so on.
- Parameters:
operationType
- operationType or null
for none
-
getProgress
public Integer getProgress()
[Output Only] An optional progress indicator that ranges from 0 to 100. There is no requirement
that this be linear or support any granularity of operations. This should not be used to guess
when the operation will be complete. This number should monotonically increase as the operation
progresses.
- Returns:
- value or
null
for none
-
setProgress
public Operation setProgress(Integer progress)
[Output Only] An optional progress indicator that ranges from 0 to 100. There is no requirement
that this be linear or support any granularity of operations. This should not be used to guess
when the operation will be complete. This number should monotonically increase as the operation
progresses.
- Parameters:
progress
- progress or null
for none
-
getRegion
public String getRegion()
[Output Only] The URL of the region where the operation resides. Only applicable when
performing regional operations.
- Returns:
- value or
null
for none
-
setRegion
public Operation setRegion(String region)
[Output Only] The URL of the region where the operation resides. Only applicable when
performing regional operations.
- Parameters:
region
- region or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public Operation setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getStartTime
public String getStartTime()
[Output Only] The time that this operation was started by the server. This value is in RFC3339
text format.
- Returns:
- value or
null
for none
-
setStartTime
public Operation setStartTime(String startTime)
[Output Only] The time that this operation was started by the server. This value is in RFC3339
text format.
- Parameters:
startTime
- startTime or null
for none
-
getStatus
public String getStatus()
[Output Only] The status of the operation, which can be one of the following: `PENDING`,
`RUNNING`, or `DONE`.
- Returns:
- value or
null
for none
-
setStatus
public Operation setStatus(String status)
[Output Only] The status of the operation, which can be one of the following: `PENDING`,
`RUNNING`, or `DONE`.
- Parameters:
status
- status or null
for none
-
getStatusMessage
public String getStatusMessage()
[Output Only] An optional textual description of the current status of the operation.
- Returns:
- value or
null
for none
-
setStatusMessage
public Operation setStatusMessage(String statusMessage)
[Output Only] An optional textual description of the current status of the operation.
- Parameters:
statusMessage
- statusMessage or null
for none
-
getTargetId
public BigInteger getTargetId()
[Output Only] The unique target ID, which identifies a specific incarnation of the target
resource.
- Returns:
- value or
null
for none
-
setTargetId
public Operation setTargetId(BigInteger targetId)
[Output Only] The unique target ID, which identifies a specific incarnation of the target
resource.
- Parameters:
targetId
- targetId or null
for none
-
getTargetLink
public String getTargetLink()
[Output Only] The URL of the resource that the operation modifies. For operations related to
creating a snapshot, this points to the persistent disk that the snapshot was created from.
- Returns:
- value or
null
for none
-
setTargetLink
public Operation setTargetLink(String targetLink)
[Output Only] The URL of the resource that the operation modifies. For operations related to
creating a snapshot, this points to the persistent disk that the snapshot was created from.
- Parameters:
targetLink
- targetLink or null
for none
-
getUser
public String getUser()
[Output Only] User who requested the operation, for example: `[email protected]`.
- Returns:
- value or
null
for none
-
setUser
public Operation setUser(String user)
[Output Only] User who requested the operation, for example: `[email protected]`.
- Parameters:
user
- user or null
for none
-
getWarnings
public List<Operation.Warnings> getWarnings()
[Output Only] If warning messages are generated during processing of the operation, this field
will be populated.
- Returns:
- value or
null
for none
-
setWarnings
public Operation setWarnings(List<Operation.Warnings> warnings)
[Output Only] If warning messages are generated during processing of the operation, this field
will be populated.
- Parameters:
warnings
- warnings or null
for none
-
getZone
public String getZone()
[Output Only] The URL of the zone where the operation resides. Only applicable when performing
per-zone operations.
- Returns:
- value or
null
for none
-
setZone
public Operation setZone(String zone)
[Output Only] The URL of the zone where the operation resides. Only applicable when performing
per-zone operations.
- Parameters:
zone
- zone or null
for none
-
set
public Operation set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Operation clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy