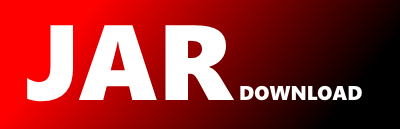
target.apidocs.com.google.api.services.compute.model.Route.html Maven / Gradle / Ivy
Route (Compute Engine API beta-rev20220312-1.32.1)
com.google.api.services.compute.model
Class Route
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.Route
-
public final class Route
extends GenericJson
Represents a Route resource. A route defines a path from VM instances in the VPC network to a
specific destination. This destination can be inside or outside the VPC network. For more
information, read the Routes overview.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
Route.Warnings
Model definition for RouteWarnings.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Route()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Route
clone()
List<RouteAsPath>
getAsPaths()
[Output Only] AS path.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional description of this resource.
String
getDestRange()
The destination range of outgoing packets that this route applies to.
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getKind()
[Output Only] Type of this resource.
String
getName()
Name of the resource.
String
getNetwork()
Fully-qualified URL of the network that this route applies to.
String
getNextHopGateway()
The URL to a gateway that should handle matching packets.
String
getNextHopIlb()
The URL to a forwarding rule of type loadBalancingScheme=INTERNAL that should handle matching
packets or the IP address of the forwarding Rule.
String
getNextHopInstance()
The URL to an instance that should handle matching packets.
String
getNextHopInterconnectAttachment()
[Output Only] The URL to an InterconnectAttachment which is the next hop for the route.
String
getNextHopIp()
The network IP address of an instance that should handle matching packets.
String
getNextHopNetwork()
The URL of the local network if it should handle matching packets.
String
getNextHopPeering()
[Output Only] The network peering name that should handle matching packets, which should
conform to RFC1035.
String
getNextHopVpnTunnel()
The URL to a VpnTunnel that should handle matching packets.
Long
getPriority()
The priority of this route.
String
getRouteStatus()
[Output only] The status of the route.
String
getRouteType()
[Output Only] The type of this route, which can be one of the following values: - 'TRANSIT' for
a transit route that this router learned from another Cloud Router and will readvertise to one
of its BGP peers - 'SUBNET' for a route from a subnet of the VPC - 'BGP' for a route learned
from a BGP peer of this router - 'STATIC' for a static route
String
getSelfLink()
[Output Only] Server-defined fully-qualified URL for this resource.
List<String>
getTags()
A list of instance tags to which this route applies.
List<Route.Warnings>
getWarnings()
[Output Only] If potential misconfigurations are detected for this route, this field will be
populated with warning messages.
Route
set(String fieldName,
Object value)
Route
setAsPaths(List<RouteAsPath> asPaths)
[Output Only] AS path.
Route
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
Route
setDescription(String description)
An optional description of this resource.
Route
setDestRange(String destRange)
The destination range of outgoing packets that this route applies to.
Route
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
Route
setKind(String kind)
[Output Only] Type of this resource.
Route
setName(String name)
Name of the resource.
Route
setNetwork(String network)
Fully-qualified URL of the network that this route applies to.
Route
setNextHopGateway(String nextHopGateway)
The URL to a gateway that should handle matching packets.
Route
setNextHopIlb(String nextHopIlb)
The URL to a forwarding rule of type loadBalancingScheme=INTERNAL that should handle matching
packets or the IP address of the forwarding Rule.
Route
setNextHopInstance(String nextHopInstance)
The URL to an instance that should handle matching packets.
Route
setNextHopInterconnectAttachment(String nextHopInterconnectAttachment)
[Output Only] The URL to an InterconnectAttachment which is the next hop for the route.
Route
setNextHopIp(String nextHopIp)
The network IP address of an instance that should handle matching packets.
Route
setNextHopNetwork(String nextHopNetwork)
The URL of the local network if it should handle matching packets.
Route
setNextHopPeering(String nextHopPeering)
[Output Only] The network peering name that should handle matching packets, which should
conform to RFC1035.
Route
setNextHopVpnTunnel(String nextHopVpnTunnel)
The URL to a VpnTunnel that should handle matching packets.
Route
setPriority(Long priority)
The priority of this route.
Route
setRouteStatus(String routeStatus)
[Output only] The status of the route.
Route
setRouteType(String routeType)
[Output Only] The type of this route, which can be one of the following values: - 'TRANSIT' for
a transit route that this router learned from another Cloud Router and will readvertise to one
of its BGP peers - 'SUBNET' for a route from a subnet of the VPC - 'BGP' for a route learned
from a BGP peer of this router - 'STATIC' for a static route
Route
setSelfLink(String selfLink)
[Output Only] Server-defined fully-qualified URL for this resource.
Route
setTags(List<String> tags)
A list of instance tags to which this route applies.
Route
setWarnings(List<Route.Warnings> warnings)
[Output Only] If potential misconfigurations are detected for this route, this field will be
populated with warning messages.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAsPaths
public List<RouteAsPath> getAsPaths()
[Output Only] AS path.
- Returns:
- value or
null
for none
-
setAsPaths
public Route setAsPaths(List<RouteAsPath> asPaths)
[Output Only] AS path.
- Parameters:
asPaths
- asPaths or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public Route setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this field when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public Route setDescription(String description)
An optional description of this resource. Provide this field when you create the resource.
- Parameters:
description
- description or null
for none
-
getDestRange
public String getDestRange()
The destination range of outgoing packets that this route applies to. Both IPv4 and IPv6 are
supported.
- Returns:
- value or
null
for none
-
setDestRange
public Route setDestRange(String destRange)
The destination range of outgoing packets that this route applies to. Both IPv4 and IPv6 are
supported.
- Parameters:
destRange
- destRange or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public Route setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output Only] Type of this resource. Always compute#routes for Route resources.
- Returns:
- value or
null
for none
-
setKind
public Route setKind(String kind)
[Output Only] Type of this resource. Always compute#routes for Route resources.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be
a lowercase letter, and all following characters (except for the last character) must be a
dash, lowercase letter, or digit. The last character must be a lowercase letter or digit.
- Returns:
- value or
null
for none
-
setName
public Route setName(String name)
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be
a lowercase letter, and all following characters (except for the last character) must be a
dash, lowercase letter, or digit. The last character must be a lowercase letter or digit.
- Parameters:
name
- name or null
for none
-
getNetwork
public String getNetwork()
Fully-qualified URL of the network that this route applies to.
- Returns:
- value or
null
for none
-
setNetwork
public Route setNetwork(String network)
Fully-qualified URL of the network that this route applies to.
- Parameters:
network
- network or null
for none
-
getNextHopGateway
public String getNextHopGateway()
The URL to a gateway that should handle matching packets. You can only specify the internet
gateway using a full or partial valid URL: projects/ project/global/gateways/default-internet-
gateway
- Returns:
- value or
null
for none
-
setNextHopGateway
public Route setNextHopGateway(String nextHopGateway)
The URL to a gateway that should handle matching packets. You can only specify the internet
gateway using a full or partial valid URL: projects/ project/global/gateways/default-internet-
gateway
- Parameters:
nextHopGateway
- nextHopGateway or null
for none
-
getNextHopIlb
public String getNextHopIlb()
The URL to a forwarding rule of type loadBalancingScheme=INTERNAL that should handle matching
packets or the IP address of the forwarding Rule. For example, the following are all valid
URLs: - 10.128.0.56 - https://www.googleapis.com/compute/v1/projects/project/regions/region
/forwardingRules/forwardingRule - regions/region/forwardingRules/forwardingRule
- Returns:
- value or
null
for none
-
setNextHopIlb
public Route setNextHopIlb(String nextHopIlb)
The URL to a forwarding rule of type loadBalancingScheme=INTERNAL that should handle matching
packets or the IP address of the forwarding Rule. For example, the following are all valid
URLs: - 10.128.0.56 - https://www.googleapis.com/compute/v1/projects/project/regions/region
/forwardingRules/forwardingRule - regions/region/forwardingRules/forwardingRule
- Parameters:
nextHopIlb
- nextHopIlb or null
for none
-
getNextHopInstance
public String getNextHopInstance()
The URL to an instance that should handle matching packets. You can specify this as a full or
partial URL. For example:
https://www.googleapis.com/compute/v1/projects/project/zones/zone/instances/
- Returns:
- value or
null
for none
-
setNextHopInstance
public Route setNextHopInstance(String nextHopInstance)
The URL to an instance that should handle matching packets. You can specify this as a full or
partial URL. For example:
https://www.googleapis.com/compute/v1/projects/project/zones/zone/instances/
- Parameters:
nextHopInstance
- nextHopInstance or null
for none
-
getNextHopInterconnectAttachment
public String getNextHopInterconnectAttachment()
[Output Only] The URL to an InterconnectAttachment which is the next hop for the route. This
field will only be populated for the dynamic routes generated by Cloud Router with a linked
interconnectAttachment.
- Returns:
- value or
null
for none
-
setNextHopInterconnectAttachment
public Route setNextHopInterconnectAttachment(String nextHopInterconnectAttachment)
[Output Only] The URL to an InterconnectAttachment which is the next hop for the route. This
field will only be populated for the dynamic routes generated by Cloud Router with a linked
interconnectAttachment.
- Parameters:
nextHopInterconnectAttachment
- nextHopInterconnectAttachment or null
for none
-
getNextHopIp
public String getNextHopIp()
The network IP address of an instance that should handle matching packets. Only IPv4 is
supported.
- Returns:
- value or
null
for none
-
setNextHopIp
public Route setNextHopIp(String nextHopIp)
The network IP address of an instance that should handle matching packets. Only IPv4 is
supported.
- Parameters:
nextHopIp
- nextHopIp or null
for none
-
getNextHopNetwork
public String getNextHopNetwork()
The URL of the local network if it should handle matching packets.
- Returns:
- value or
null
for none
-
setNextHopNetwork
public Route setNextHopNetwork(String nextHopNetwork)
The URL of the local network if it should handle matching packets.
- Parameters:
nextHopNetwork
- nextHopNetwork or null
for none
-
getNextHopPeering
public String getNextHopPeering()
[Output Only] The network peering name that should handle matching packets, which should
conform to RFC1035.
- Returns:
- value or
null
for none
-
setNextHopPeering
public Route setNextHopPeering(String nextHopPeering)
[Output Only] The network peering name that should handle matching packets, which should
conform to RFC1035.
- Parameters:
nextHopPeering
- nextHopPeering or null
for none
-
getNextHopVpnTunnel
public String getNextHopVpnTunnel()
The URL to a VpnTunnel that should handle matching packets.
- Returns:
- value or
null
for none
-
setNextHopVpnTunnel
public Route setNextHopVpnTunnel(String nextHopVpnTunnel)
The URL to a VpnTunnel that should handle matching packets.
- Parameters:
nextHopVpnTunnel
- nextHopVpnTunnel or null
for none
-
getPriority
public Long getPriority()
The priority of this route. Priority is used to break ties in cases where there is more than
one matching route of equal prefix length. In cases where multiple routes have equal prefix
length, the one with the lowest-numbered priority value wins. The default value is `1000`. The
priority value must be from `0` to `65535`, inclusive.
- Returns:
- value or
null
for none
-
setPriority
public Route setPriority(Long priority)
The priority of this route. Priority is used to break ties in cases where there is more than
one matching route of equal prefix length. In cases where multiple routes have equal prefix
length, the one with the lowest-numbered priority value wins. The default value is `1000`. The
priority value must be from `0` to `65535`, inclusive.
- Parameters:
priority
- priority or null
for none
-
getRouteStatus
public String getRouteStatus()
[Output only] The status of the route.
- Returns:
- value or
null
for none
-
setRouteStatus
public Route setRouteStatus(String routeStatus)
[Output only] The status of the route.
- Parameters:
routeStatus
- routeStatus or null
for none
-
getRouteType
public String getRouteType()
[Output Only] The type of this route, which can be one of the following values: - 'TRANSIT' for
a transit route that this router learned from another Cloud Router and will readvertise to one
of its BGP peers - 'SUBNET' for a route from a subnet of the VPC - 'BGP' for a route learned
from a BGP peer of this router - 'STATIC' for a static route
- Returns:
- value or
null
for none
-
setRouteType
public Route setRouteType(String routeType)
[Output Only] The type of this route, which can be one of the following values: - 'TRANSIT' for
a transit route that this router learned from another Cloud Router and will readvertise to one
of its BGP peers - 'SUBNET' for a route from a subnet of the VPC - 'BGP' for a route learned
from a BGP peer of this router - 'STATIC' for a static route
- Parameters:
routeType
- routeType or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined fully-qualified URL for this resource.
- Returns:
- value or
null
for none
-
setSelfLink
public Route setSelfLink(String selfLink)
[Output Only] Server-defined fully-qualified URL for this resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getTags
public List<String> getTags()
A list of instance tags to which this route applies.
- Returns:
- value or
null
for none
-
setTags
public Route setTags(List<String> tags)
A list of instance tags to which this route applies.
- Parameters:
tags
- tags or null
for none
-
getWarnings
public List<Route.Warnings> getWarnings()
[Output Only] If potential misconfigurations are detected for this route, this field will be
populated with warning messages.
- Returns:
- value or
null
for none
-
setWarnings
public Route setWarnings(List<Route.Warnings> warnings)
[Output Only] If potential misconfigurations are detected for this route, this field will be
populated with warning messages.
- Parameters:
warnings
- warnings or null
for none
-
set
public Route set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Route clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy