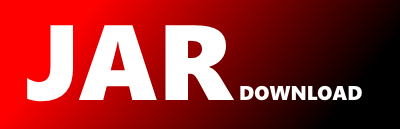
com.google.api.services.compute.model.AllocationSpecificSKUAllocationReservedInstanceProperties Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Properties of the SKU instances being reserved. Next ID: 9
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AllocationSpecificSKUAllocationReservedInstanceProperties extends com.google.api.client.json.GenericJson {
/**
* Specifies accelerator type and count.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List guestAccelerators;
static {
// hack to force ProGuard to consider AcceleratorConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AcceleratorConfig.class);
}
/**
* Specifies amount of local ssd to reserve with each instance. The type of disk is local-ssd.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List localSsds;
static {
// hack to force ProGuard to consider AllocationSpecificSKUAllocationAllocatedInstancePropertiesReservedDisk used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AllocationSpecificSKUAllocationAllocatedInstancePropertiesReservedDisk.class);
}
/**
* An opaque location hint used to place the allocation close to other resources. This field is
* for use by internal tools that use the public API.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String locationHint;
/**
* Specifies type of machine (name only) which has fixed number of vCPUs and fixed amount of
* memory. This also includes specifying custom machine type following custom-NUMBER_OF_CPUS-
* AMOUNT_OF_MEMORY pattern.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String machineType;
/**
* Specifies the number of hours after reservation creation where instances using the reservation
* won't be scheduled for maintenance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer maintenanceFreezeDurationHours;
/**
* For more information about maintenance intervals, see Setting maintenance intervals.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String maintenanceInterval;
/**
* Minimum cpu platform the reservation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String minCpuPlatform;
/**
* Specifies accelerator type and count.
* @return value or {@code null} for none
*/
public java.util.List getGuestAccelerators() {
return guestAccelerators;
}
/**
* Specifies accelerator type and count.
* @param guestAccelerators guestAccelerators or {@code null} for none
*/
public AllocationSpecificSKUAllocationReservedInstanceProperties setGuestAccelerators(java.util.List guestAccelerators) {
this.guestAccelerators = guestAccelerators;
return this;
}
/**
* Specifies amount of local ssd to reserve with each instance. The type of disk is local-ssd.
* @return value or {@code null} for none
*/
public java.util.List getLocalSsds() {
return localSsds;
}
/**
* Specifies amount of local ssd to reserve with each instance. The type of disk is local-ssd.
* @param localSsds localSsds or {@code null} for none
*/
public AllocationSpecificSKUAllocationReservedInstanceProperties setLocalSsds(java.util.List localSsds) {
this.localSsds = localSsds;
return this;
}
/**
* An opaque location hint used to place the allocation close to other resources. This field is
* for use by internal tools that use the public API.
* @return value or {@code null} for none
*/
public java.lang.String getLocationHint() {
return locationHint;
}
/**
* An opaque location hint used to place the allocation close to other resources. This field is
* for use by internal tools that use the public API.
* @param locationHint locationHint or {@code null} for none
*/
public AllocationSpecificSKUAllocationReservedInstanceProperties setLocationHint(java.lang.String locationHint) {
this.locationHint = locationHint;
return this;
}
/**
* Specifies type of machine (name only) which has fixed number of vCPUs and fixed amount of
* memory. This also includes specifying custom machine type following custom-NUMBER_OF_CPUS-
* AMOUNT_OF_MEMORY pattern.
* @return value or {@code null} for none
*/
public java.lang.String getMachineType() {
return machineType;
}
/**
* Specifies type of machine (name only) which has fixed number of vCPUs and fixed amount of
* memory. This also includes specifying custom machine type following custom-NUMBER_OF_CPUS-
* AMOUNT_OF_MEMORY pattern.
* @param machineType machineType or {@code null} for none
*/
public AllocationSpecificSKUAllocationReservedInstanceProperties setMachineType(java.lang.String machineType) {
this.machineType = machineType;
return this;
}
/**
* Specifies the number of hours after reservation creation where instances using the reservation
* won't be scheduled for maintenance.
* @return value or {@code null} for none
*/
public java.lang.Integer getMaintenanceFreezeDurationHours() {
return maintenanceFreezeDurationHours;
}
/**
* Specifies the number of hours after reservation creation where instances using the reservation
* won't be scheduled for maintenance.
* @param maintenanceFreezeDurationHours maintenanceFreezeDurationHours or {@code null} for none
*/
public AllocationSpecificSKUAllocationReservedInstanceProperties setMaintenanceFreezeDurationHours(java.lang.Integer maintenanceFreezeDurationHours) {
this.maintenanceFreezeDurationHours = maintenanceFreezeDurationHours;
return this;
}
/**
* For more information about maintenance intervals, see Setting maintenance intervals.
* @return value or {@code null} for none
*/
public java.lang.String getMaintenanceInterval() {
return maintenanceInterval;
}
/**
* For more information about maintenance intervals, see Setting maintenance intervals.
* @param maintenanceInterval maintenanceInterval or {@code null} for none
*/
public AllocationSpecificSKUAllocationReservedInstanceProperties setMaintenanceInterval(java.lang.String maintenanceInterval) {
this.maintenanceInterval = maintenanceInterval;
return this;
}
/**
* Minimum cpu platform the reservation.
* @return value or {@code null} for none
*/
public java.lang.String getMinCpuPlatform() {
return minCpuPlatform;
}
/**
* Minimum cpu platform the reservation.
* @param minCpuPlatform minCpuPlatform or {@code null} for none
*/
public AllocationSpecificSKUAllocationReservedInstanceProperties setMinCpuPlatform(java.lang.String minCpuPlatform) {
this.minCpuPlatform = minCpuPlatform;
return this;
}
@Override
public AllocationSpecificSKUAllocationReservedInstanceProperties set(String fieldName, Object value) {
return (AllocationSpecificSKUAllocationReservedInstanceProperties) super.set(fieldName, value);
}
@Override
public AllocationSpecificSKUAllocationReservedInstanceProperties clone() {
return (AllocationSpecificSKUAllocationReservedInstanceProperties) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy