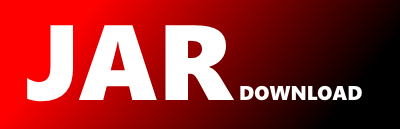
com.google.api.services.compute.model.AutoscalingPolicyCustomMetricUtilization Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Custom utilization metric policy.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AutoscalingPolicyCustomMetricUtilization extends com.google.api.client.json.GenericJson {
/**
* A filter string, compatible with a Stackdriver Monitoring filter string for TimeSeries.list API
* call. This filter is used to select a specific TimeSeries for the purpose of autoscaling and to
* determine whether the metric is exporting per-instance or per-group data. For the filter to be
* valid for autoscaling purposes, the following rules apply: - You can only use the AND operator
* for joining selectors. - You can only use direct equality comparison operator (=) without any
* functions for each selector. - You can specify the metric in both the filter string and in the
* metric field. However, if specified in both places, the metric must be identical. - The
* monitored resource type determines what kind of values are expected for the metric. If it is a
* gce_instance, the autoscaler expects the metric to include a separate TimeSeries for each
* instance in a group. In such a case, you cannot filter on resource labels. If the resource type
* is any other value, the autoscaler expects this metric to contain values that apply to the
* entire autoscaled instance group and resource label filtering can be performed to point
* autoscaler at the correct TimeSeries to scale upon. This is called a *per-group metric* for the
* purpose of autoscaling. If not specified, the type defaults to gce_instance. Try to provide a
* filter that is selective enough to pick just one TimeSeries for the autoscaled group or for
* each of the instances (if you are using gce_instance resource type). If multiple TimeSeries are
* returned upon the query execution, the autoscaler will sum their respective values to obtain
* its scaling value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**
* The identifier (type) of the Stackdriver Monitoring metric. The metric cannot have negative
* values. The metric must have a value type of INT64 or DOUBLE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String metric;
/**
* If scaling is based on a per-group metric value that represents the total amount of work to be
* done or resource usage, set this value to an amount assigned for a single instance of the
* scaled group. Autoscaler keeps the number of instances proportional to the value of this
* metric. The metric itself does not change value due to group resizing. A good metric to use
* with the target is for example pubsub.googleapis.com/subscription/num_undelivered_messages or a
* custom metric exporting the total number of requests coming to your instances. A bad example
* would be a metric exporting an average or median latency, since this value can't include a
* chunk assignable to a single instance, it could be better used with utilization_target instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double singleInstanceAssignment;
/**
* The target value of the metric that autoscaler maintains. This must be a positive value. A
* utilization metric scales number of virtual machines handling requests to increase or decrease
* proportionally to the metric. For example, a good metric to use as a utilization_target is
* https://www.googleapis.com/compute/v1/instance/network/received_bytes_count. The autoscaler
* works to keep this value constant for each of the instances.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double utilizationTarget;
/**
* Defines how target utilization value is expressed for a Stackdriver Monitoring metric. Either
* GAUGE, DELTA_PER_SECOND, or DELTA_PER_MINUTE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String utilizationTargetType;
/**
* A filter string, compatible with a Stackdriver Monitoring filter string for TimeSeries.list API
* call. This filter is used to select a specific TimeSeries for the purpose of autoscaling and to
* determine whether the metric is exporting per-instance or per-group data. For the filter to be
* valid for autoscaling purposes, the following rules apply: - You can only use the AND operator
* for joining selectors. - You can only use direct equality comparison operator (=) without any
* functions for each selector. - You can specify the metric in both the filter string and in the
* metric field. However, if specified in both places, the metric must be identical. - The
* monitored resource type determines what kind of values are expected for the metric. If it is a
* gce_instance, the autoscaler expects the metric to include a separate TimeSeries for each
* instance in a group. In such a case, you cannot filter on resource labels. If the resource type
* is any other value, the autoscaler expects this metric to contain values that apply to the
* entire autoscaled instance group and resource label filtering can be performed to point
* autoscaler at the correct TimeSeries to scale upon. This is called a *per-group metric* for the
* purpose of autoscaling. If not specified, the type defaults to gce_instance. Try to provide a
* filter that is selective enough to pick just one TimeSeries for the autoscaled group or for
* each of the instances (if you are using gce_instance resource type). If multiple TimeSeries are
* returned upon the query execution, the autoscaler will sum their respective values to obtain
* its scaling value.
* @return value or {@code null} for none
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter string, compatible with a Stackdriver Monitoring filter string for TimeSeries.list API
* call. This filter is used to select a specific TimeSeries for the purpose of autoscaling and to
* determine whether the metric is exporting per-instance or per-group data. For the filter to be
* valid for autoscaling purposes, the following rules apply: - You can only use the AND operator
* for joining selectors. - You can only use direct equality comparison operator (=) without any
* functions for each selector. - You can specify the metric in both the filter string and in the
* metric field. However, if specified in both places, the metric must be identical. - The
* monitored resource type determines what kind of values are expected for the metric. If it is a
* gce_instance, the autoscaler expects the metric to include a separate TimeSeries for each
* instance in a group. In such a case, you cannot filter on resource labels. If the resource type
* is any other value, the autoscaler expects this metric to contain values that apply to the
* entire autoscaled instance group and resource label filtering can be performed to point
* autoscaler at the correct TimeSeries to scale upon. This is called a *per-group metric* for the
* purpose of autoscaling. If not specified, the type defaults to gce_instance. Try to provide a
* filter that is selective enough to pick just one TimeSeries for the autoscaled group or for
* each of the instances (if you are using gce_instance resource type). If multiple TimeSeries are
* returned upon the query execution, the autoscaler will sum their respective values to obtain
* its scaling value.
* @param filter filter or {@code null} for none
*/
public AutoscalingPolicyCustomMetricUtilization setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The identifier (type) of the Stackdriver Monitoring metric. The metric cannot have negative
* values. The metric must have a value type of INT64 or DOUBLE.
* @return value or {@code null} for none
*/
public java.lang.String getMetric() {
return metric;
}
/**
* The identifier (type) of the Stackdriver Monitoring metric. The metric cannot have negative
* values. The metric must have a value type of INT64 or DOUBLE.
* @param metric metric or {@code null} for none
*/
public AutoscalingPolicyCustomMetricUtilization setMetric(java.lang.String metric) {
this.metric = metric;
return this;
}
/**
* If scaling is based on a per-group metric value that represents the total amount of work to be
* done or resource usage, set this value to an amount assigned for a single instance of the
* scaled group. Autoscaler keeps the number of instances proportional to the value of this
* metric. The metric itself does not change value due to group resizing. A good metric to use
* with the target is for example pubsub.googleapis.com/subscription/num_undelivered_messages or a
* custom metric exporting the total number of requests coming to your instances. A bad example
* would be a metric exporting an average or median latency, since this value can't include a
* chunk assignable to a single instance, it could be better used with utilization_target instead.
* @return value or {@code null} for none
*/
public java.lang.Double getSingleInstanceAssignment() {
return singleInstanceAssignment;
}
/**
* If scaling is based on a per-group metric value that represents the total amount of work to be
* done or resource usage, set this value to an amount assigned for a single instance of the
* scaled group. Autoscaler keeps the number of instances proportional to the value of this
* metric. The metric itself does not change value due to group resizing. A good metric to use
* with the target is for example pubsub.googleapis.com/subscription/num_undelivered_messages or a
* custom metric exporting the total number of requests coming to your instances. A bad example
* would be a metric exporting an average or median latency, since this value can't include a
* chunk assignable to a single instance, it could be better used with utilization_target instead.
* @param singleInstanceAssignment singleInstanceAssignment or {@code null} for none
*/
public AutoscalingPolicyCustomMetricUtilization setSingleInstanceAssignment(java.lang.Double singleInstanceAssignment) {
this.singleInstanceAssignment = singleInstanceAssignment;
return this;
}
/**
* The target value of the metric that autoscaler maintains. This must be a positive value. A
* utilization metric scales number of virtual machines handling requests to increase or decrease
* proportionally to the metric. For example, a good metric to use as a utilization_target is
* https://www.googleapis.com/compute/v1/instance/network/received_bytes_count. The autoscaler
* works to keep this value constant for each of the instances.
* @return value or {@code null} for none
*/
public java.lang.Double getUtilizationTarget() {
return utilizationTarget;
}
/**
* The target value of the metric that autoscaler maintains. This must be a positive value. A
* utilization metric scales number of virtual machines handling requests to increase or decrease
* proportionally to the metric. For example, a good metric to use as a utilization_target is
* https://www.googleapis.com/compute/v1/instance/network/received_bytes_count. The autoscaler
* works to keep this value constant for each of the instances.
* @param utilizationTarget utilizationTarget or {@code null} for none
*/
public AutoscalingPolicyCustomMetricUtilization setUtilizationTarget(java.lang.Double utilizationTarget) {
this.utilizationTarget = utilizationTarget;
return this;
}
/**
* Defines how target utilization value is expressed for a Stackdriver Monitoring metric. Either
* GAUGE, DELTA_PER_SECOND, or DELTA_PER_MINUTE.
* @return value or {@code null} for none
*/
public java.lang.String getUtilizationTargetType() {
return utilizationTargetType;
}
/**
* Defines how target utilization value is expressed for a Stackdriver Monitoring metric. Either
* GAUGE, DELTA_PER_SECOND, or DELTA_PER_MINUTE.
* @param utilizationTargetType utilizationTargetType or {@code null} for none
*/
public AutoscalingPolicyCustomMetricUtilization setUtilizationTargetType(java.lang.String utilizationTargetType) {
this.utilizationTargetType = utilizationTargetType;
return this;
}
@Override
public AutoscalingPolicyCustomMetricUtilization set(String fieldName, Object value) {
return (AutoscalingPolicyCustomMetricUtilization) super.set(fieldName, value);
}
@Override
public AutoscalingPolicyCustomMetricUtilization clone() {
return (AutoscalingPolicyCustomMetricUtilization) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy