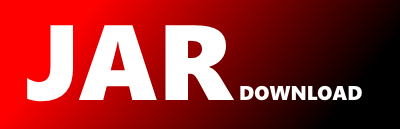
com.google.api.services.compute.model.BackendService Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Represents a Backend Service resource. A backend service defines how Google Cloud load balancers
* distribute traffic. The backend service configuration contains a set of values, such as the
* protocol used to connect to backends, various distribution and session settings, health checks,
* and timeouts. These settings provide fine-grained control over how your load balancer behaves.
* Most of the settings have default values that allow for easy configuration if you need to get
* started quickly. Backend services in Google Compute Engine can be either regionally or globally
* scoped. * [Global](https://cloud.google.com/compute/docs/reference/rest/beta/backendServices) *
* [Regional](https://cloud.google.com/compute/docs/reference/rest/beta/regionBackendServices) For
* more information, see Backend Services.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class BackendService extends com.google.api.client.json.GenericJson {
/**
* Lifetime of cookies in seconds. This setting is applicable to external and internal HTTP(S)
* load balancers and Traffic Director and requires GENERATED_COOKIE or HTTP_COOKIE session
* affinity. If set to 0, the cookie is non-persistent and lasts only until the end of the browser
* session (or equivalent). The maximum allowed value is two weeks (1,209,600). Not supported when
* the backend service is referenced by a URL map that is bound to target gRPC proxy that has
* validateForProxyless field set to true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer affinityCookieTtlSec;
/**
* The list of backends that serve this BackendService.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List backends;
static {
// hack to force ProGuard to consider Backend used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Backend.class);
}
/**
* Cloud CDN configuration for this BackendService. Only available for specified load balancer
* types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackendServiceCdnPolicy cdnPolicy;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CircuitBreakers circuitBreakers;
/**
* Compress text responses using Brotli or gzip compression, based on the client's Accept-Encoding
* header.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String compressionMode;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ConnectionDraining connectionDraining;
/**
* Connection Tracking configuration for this BackendService. Connection tracking policy settings
* are only available for Network Load Balancing and Internal TCP/UDP Load Balancing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackendServiceConnectionTrackingPolicy connectionTrackingPolicy;
/**
* Consistent Hash-based load balancing can be used to provide soft session affinity based on HTTP
* headers, cookies or other properties. This load balancing policy is applicable only for HTTP
* connections. The affinity to a particular destination host will be lost when one or more hosts
* are added/removed from the destination service. This field specifies parameters that control
* consistent hashing. This field is only applicable when localityLbPolicy is set to MAGLEV or
* RING_HASH. This field is applicable to either: - A regional backend service with the
* service_protocol set to HTTP, HTTPS, or HTTP2, and load_balancing_scheme set to
* INTERNAL_MANAGED. - A global backend service with the load_balancing_scheme set to
* INTERNAL_SELF_MANAGED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ConsistentHashLoadBalancerSettings consistentHash;
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creationTimestamp;
/**
* Headers that the load balancer adds to proxied requests. See [Creating custom
* headers](https://cloud.google.com/load-balancing/docs/custom-headers).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List customRequestHeaders;
/**
* Headers that the load balancer adds to proxied responses. See [Creating custom
* headers](https://cloud.google.com/load-balancing/docs/custom-headers).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List customResponseHeaders;
/**
* An optional description of this resource. Provide this property when you create the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* [Output Only] The resource URL for the edge security policy associated with this backend
* service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String edgeSecurityPolicy;
/**
* If true, enables Cloud CDN for the backend service of an external HTTP(S) load balancer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableCDN;
/**
* Requires at least one backend instance group to be defined as a backup (failover) backend. For
* load balancers that have configurable failover: [Internal TCP/UDP Load
* Balancing](https://cloud.google.com/load-balancing/docs/internal/failover-overview) and
* [external TCP/UDP Load Balancing](https://cloud.google.com/load-balancing/docs/network
* /networklb-failover-overview).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackendServiceFailoverPolicy failoverPolicy;
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a BackendService. An up-to-
* date fingerprint must be provided in order to update the BackendService, otherwise the request
* will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request
* to retrieve a BackendService.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fingerprint;
/**
* The list of URLs to the healthChecks, httpHealthChecks (legacy), or httpsHealthChecks (legacy)
* resource for health checking this backend service. Not all backend services support legacy
* health checks. See Load balancer guide. Currently, at most one health check can be specified
* for each backend service. Backend services with instance group or zonal NEG backends must have
* a health check. Backend services with internet or serverless NEG backends must not have a
* health check.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List healthChecks;
/**
* The configurations for Identity-Aware Proxy on this resource. Not available for Internal
* TCP/UDP Load Balancing and Network Load Balancing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackendServiceIAP iap;
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger id;
/**
* [Output Only] Type of resource. Always compute#backendService for backend services.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Specifies the load balancer type. A backend service created for one type of load balancer
* cannot be used with another. For more information, refer to Choosing a load balancer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String loadBalancingScheme;
/**
* A list of locality load balancing policies to be used in order of preference. Either the policy
* or the customPolicy field should be set. Overrides any value set in the localityLbPolicy field.
* localityLbPolicies is only supported when the BackendService is referenced by a URL Map that is
* referenced by a target gRPC proxy that has the validateForProxyless field set to true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List localityLbPolicies;
/**
* The load balancing algorithm used within the scope of the locality. The possible values are: -
* ROUND_ROBIN: This is a simple policy in which each healthy backend is selected in round robin
* order. This is the default. - LEAST_REQUEST: An O(1) algorithm which selects two random healthy
* hosts and picks the host which has fewer active requests. - RING_HASH: The ring/modulo hash
* load balancer implements consistent hashing to backends. The algorithm has the property that
* the addition/removal of a host from a set of N hosts only affects 1/N of the requests. -
* RANDOM: The load balancer selects a random healthy host. - ORIGINAL_DESTINATION: Backend host
* is selected based on the client connection metadata, i.e., connections are opened to the same
* address as the destination address of the incoming connection before the connection was
* redirected to the load balancer. - MAGLEV: used as a drop in replacement for the ring hash load
* balancer. Maglev is not as stable as ring hash but has faster table lookup build times and host
* selection times. For more information about Maglev, see
* https://ai.google/research/pubs/pub44824 This field is applicable to either: - A regional
* backend service with the service_protocol set to HTTP, HTTPS, or HTTP2, and
* load_balancing_scheme set to INTERNAL_MANAGED. - A global backend service with the
* load_balancing_scheme set to INTERNAL_SELF_MANAGED. If sessionAffinity is not NONE, and this
* field is not set to MAGLEV or RING_HASH, session affinity settings will not take effect. Only
* ROUND_ROBIN and RING_HASH are supported when the backend service is referenced by a URL map
* that is bound to target gRPC proxy that has validateForProxyless field set to true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String localityLbPolicy;
/**
* This field denotes the logging options for the load balancer traffic served by this backend
* service. If logging is enabled, logs will be exported to Stackdriver.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackendServiceLogConfig logConfig;
/**
* Specifies the default maximum duration (timeout) for streams to this service. Duration is
* computed from the beginning of the stream until the response has been completely processed,
* including all retries. A stream that does not complete in this duration is closed. If not
* specified, there will be no timeout limit, i.e. the maximum duration is infinite. This value
* can be overridden in the PathMatcher configuration of the UrlMap that references this backend
* service. This field is only allowed when the loadBalancingScheme of the backend service is
* INTERNAL_SELF_MANAGED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Duration maxStreamDuration;
/**
* Name of the resource. Provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The URL of the network to which this backend service belongs. This field can only be specified
* when the load balancing scheme is set to INTERNAL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String network;
/**
* Settings controlling the eviction of unhealthy hosts from the load balancing pool for the
* backend service. If not set, this feature is considered disabled. This field is applicable to
* either: - A regional backend service with the service_protocol set to HTTP, HTTPS, or HTTP2,
* and load_balancing_scheme set to INTERNAL_MANAGED. - A global backend service with the
* load_balancing_scheme set to INTERNAL_SELF_MANAGED. Not supported when the backend service is
* referenced by a URL map that is bound to target gRPC proxy that has validateForProxyless field
* set to true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private OutlierDetection outlierDetection;
/**
* Deprecated in favor of portName. The TCP port to connect on the backend. The default value is
* 80. For Internal TCP/UDP Load Balancing and Network Load Balancing, omit port.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer port;
/**
* A named port on a backend instance group representing the port for communication to the backend
* VMs in that group. The named port must be [defined on each backend instance
* group](https://cloud.google.com/load-balancing/docs/backend-service#named_ports). This
* parameter has no meaning if the backends are NEGs. For Internal TCP/UDP Load Balancing and
* Network Load Balancing, omit port_name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String portName;
/**
* The protocol this BackendService uses to communicate with backends. Possible values are HTTP,
* HTTPS, HTTP2, TCP, SSL, UDP or GRPC. depending on the chosen load balancer or Traffic Director
* configuration. Refer to the documentation for the load balancers or for Traffic Director for
* more information. Must be set to GRPC when the backend service is referenced by a URL map that
* is bound to target gRPC proxy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String protocol;
/**
* [Output Only] URL of the region where the regional backend service resides. This field is not
* applicable to global backend services. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String region;
/**
* [Output Only] The resource URL for the security policy associated with this backend service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String securityPolicy;
/**
* This field specifies the security settings that apply to this backend service. This field is
* applicable to a global backend service with the load_balancing_scheme set to
* INTERNAL_SELF_MANAGED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SecuritySettings securitySettings;
/**
* [Output Only] Server-defined URL for the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* URLs of networkservices.ServiceBinding resources. Can only be set if load balancing scheme is
* INTERNAL_SELF_MANAGED. If set, lists of backends and health checks must be both empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List serviceBindings;
/**
* Type of session affinity to use. The default is NONE. Only NONE and HEADER_FIELD are supported
* when the backend service is referenced by a URL map that is bound to target gRPC proxy that has
* validateForProxyless field set to true. For more details, see: [Session
* Affinity](https://cloud.google.com/load-balancing/docs/backend-service#session_affinity).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sessionAffinity;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Subsetting subsetting;
/**
* The backend service timeout has a different meaning depending on the type of load balancer. For
* more information see, Backend service settings. The default is 30 seconds. The full range of
* timeout values allowed goes from 1 through 2,147,483,647 seconds. This value can be overridden
* in the PathMatcher configuration of the UrlMap that references this backend service. Not
* supported when the backend service is referenced by a URL map that is bound to target gRPC
* proxy that has validateForProxyless field set to true. Instead, use maxStreamDuration.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer timeoutSec;
/**
* Lifetime of cookies in seconds. This setting is applicable to external and internal HTTP(S)
* load balancers and Traffic Director and requires GENERATED_COOKIE or HTTP_COOKIE session
* affinity. If set to 0, the cookie is non-persistent and lasts only until the end of the browser
* session (or equivalent). The maximum allowed value is two weeks (1,209,600). Not supported when
* the backend service is referenced by a URL map that is bound to target gRPC proxy that has
* validateForProxyless field set to true.
* @return value or {@code null} for none
*/
public java.lang.Integer getAffinityCookieTtlSec() {
return affinityCookieTtlSec;
}
/**
* Lifetime of cookies in seconds. This setting is applicable to external and internal HTTP(S)
* load balancers and Traffic Director and requires GENERATED_COOKIE or HTTP_COOKIE session
* affinity. If set to 0, the cookie is non-persistent and lasts only until the end of the browser
* session (or equivalent). The maximum allowed value is two weeks (1,209,600). Not supported when
* the backend service is referenced by a URL map that is bound to target gRPC proxy that has
* validateForProxyless field set to true.
* @param affinityCookieTtlSec affinityCookieTtlSec or {@code null} for none
*/
public BackendService setAffinityCookieTtlSec(java.lang.Integer affinityCookieTtlSec) {
this.affinityCookieTtlSec = affinityCookieTtlSec;
return this;
}
/**
* The list of backends that serve this BackendService.
* @return value or {@code null} for none
*/
public java.util.List getBackends() {
return backends;
}
/**
* The list of backends that serve this BackendService.
* @param backends backends or {@code null} for none
*/
public BackendService setBackends(java.util.List backends) {
this.backends = backends;
return this;
}
/**
* Cloud CDN configuration for this BackendService. Only available for specified load balancer
* types.
* @return value or {@code null} for none
*/
public BackendServiceCdnPolicy getCdnPolicy() {
return cdnPolicy;
}
/**
* Cloud CDN configuration for this BackendService. Only available for specified load balancer
* types.
* @param cdnPolicy cdnPolicy or {@code null} for none
*/
public BackendService setCdnPolicy(BackendServiceCdnPolicy cdnPolicy) {
this.cdnPolicy = cdnPolicy;
return this;
}
/**
* @return value or {@code null} for none
*/
public CircuitBreakers getCircuitBreakers() {
return circuitBreakers;
}
/**
* @param circuitBreakers circuitBreakers or {@code null} for none
*/
public BackendService setCircuitBreakers(CircuitBreakers circuitBreakers) {
this.circuitBreakers = circuitBreakers;
return this;
}
/**
* Compress text responses using Brotli or gzip compression, based on the client's Accept-Encoding
* header.
* @return value or {@code null} for none
*/
public java.lang.String getCompressionMode() {
return compressionMode;
}
/**
* Compress text responses using Brotli or gzip compression, based on the client's Accept-Encoding
* header.
* @param compressionMode compressionMode or {@code null} for none
*/
public BackendService setCompressionMode(java.lang.String compressionMode) {
this.compressionMode = compressionMode;
return this;
}
/**
* @return value or {@code null} for none
*/
public ConnectionDraining getConnectionDraining() {
return connectionDraining;
}
/**
* @param connectionDraining connectionDraining or {@code null} for none
*/
public BackendService setConnectionDraining(ConnectionDraining connectionDraining) {
this.connectionDraining = connectionDraining;
return this;
}
/**
* Connection Tracking configuration for this BackendService. Connection tracking policy settings
* are only available for Network Load Balancing and Internal TCP/UDP Load Balancing.
* @return value or {@code null} for none
*/
public BackendServiceConnectionTrackingPolicy getConnectionTrackingPolicy() {
return connectionTrackingPolicy;
}
/**
* Connection Tracking configuration for this BackendService. Connection tracking policy settings
* are only available for Network Load Balancing and Internal TCP/UDP Load Balancing.
* @param connectionTrackingPolicy connectionTrackingPolicy or {@code null} for none
*/
public BackendService setConnectionTrackingPolicy(BackendServiceConnectionTrackingPolicy connectionTrackingPolicy) {
this.connectionTrackingPolicy = connectionTrackingPolicy;
return this;
}
/**
* Consistent Hash-based load balancing can be used to provide soft session affinity based on HTTP
* headers, cookies or other properties. This load balancing policy is applicable only for HTTP
* connections. The affinity to a particular destination host will be lost when one or more hosts
* are added/removed from the destination service. This field specifies parameters that control
* consistent hashing. This field is only applicable when localityLbPolicy is set to MAGLEV or
* RING_HASH. This field is applicable to either: - A regional backend service with the
* service_protocol set to HTTP, HTTPS, or HTTP2, and load_balancing_scheme set to
* INTERNAL_MANAGED. - A global backend service with the load_balancing_scheme set to
* INTERNAL_SELF_MANAGED.
* @return value or {@code null} for none
*/
public ConsistentHashLoadBalancerSettings getConsistentHash() {
return consistentHash;
}
/**
* Consistent Hash-based load balancing can be used to provide soft session affinity based on HTTP
* headers, cookies or other properties. This load balancing policy is applicable only for HTTP
* connections. The affinity to a particular destination host will be lost when one or more hosts
* are added/removed from the destination service. This field specifies parameters that control
* consistent hashing. This field is only applicable when localityLbPolicy is set to MAGLEV or
* RING_HASH. This field is applicable to either: - A regional backend service with the
* service_protocol set to HTTP, HTTPS, or HTTP2, and load_balancing_scheme set to
* INTERNAL_MANAGED. - A global backend service with the load_balancing_scheme set to
* INTERNAL_SELF_MANAGED.
* @param consistentHash consistentHash or {@code null} for none
*/
public BackendService setConsistentHash(ConsistentHashLoadBalancerSettings consistentHash) {
this.consistentHash = consistentHash;
return this;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @return value or {@code null} for none
*/
public java.lang.String getCreationTimestamp() {
return creationTimestamp;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public BackendService setCreationTimestamp(java.lang.String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* Headers that the load balancer adds to proxied requests. See [Creating custom
* headers](https://cloud.google.com/load-balancing/docs/custom-headers).
* @return value or {@code null} for none
*/
public java.util.List getCustomRequestHeaders() {
return customRequestHeaders;
}
/**
* Headers that the load balancer adds to proxied requests. See [Creating custom
* headers](https://cloud.google.com/load-balancing/docs/custom-headers).
* @param customRequestHeaders customRequestHeaders or {@code null} for none
*/
public BackendService setCustomRequestHeaders(java.util.List customRequestHeaders) {
this.customRequestHeaders = customRequestHeaders;
return this;
}
/**
* Headers that the load balancer adds to proxied responses. See [Creating custom
* headers](https://cloud.google.com/load-balancing/docs/custom-headers).
* @return value or {@code null} for none
*/
public java.util.List getCustomResponseHeaders() {
return customResponseHeaders;
}
/**
* Headers that the load balancer adds to proxied responses. See [Creating custom
* headers](https://cloud.google.com/load-balancing/docs/custom-headers).
* @param customResponseHeaders customResponseHeaders or {@code null} for none
*/
public BackendService setCustomResponseHeaders(java.util.List customResponseHeaders) {
this.customResponseHeaders = customResponseHeaders;
return this;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @param description description or {@code null} for none
*/
public BackendService setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* [Output Only] The resource URL for the edge security policy associated with this backend
* service.
* @return value or {@code null} for none
*/
public java.lang.String getEdgeSecurityPolicy() {
return edgeSecurityPolicy;
}
/**
* [Output Only] The resource URL for the edge security policy associated with this backend
* service.
* @param edgeSecurityPolicy edgeSecurityPolicy or {@code null} for none
*/
public BackendService setEdgeSecurityPolicy(java.lang.String edgeSecurityPolicy) {
this.edgeSecurityPolicy = edgeSecurityPolicy;
return this;
}
/**
* If true, enables Cloud CDN for the backend service of an external HTTP(S) load balancer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableCDN() {
return enableCDN;
}
/**
* If true, enables Cloud CDN for the backend service of an external HTTP(S) load balancer.
* @param enableCDN enableCDN or {@code null} for none
*/
public BackendService setEnableCDN(java.lang.Boolean enableCDN) {
this.enableCDN = enableCDN;
return this;
}
/**
* Requires at least one backend instance group to be defined as a backup (failover) backend. For
* load balancers that have configurable failover: [Internal TCP/UDP Load
* Balancing](https://cloud.google.com/load-balancing/docs/internal/failover-overview) and
* [external TCP/UDP Load Balancing](https://cloud.google.com/load-balancing/docs/network
* /networklb-failover-overview).
* @return value or {@code null} for none
*/
public BackendServiceFailoverPolicy getFailoverPolicy() {
return failoverPolicy;
}
/**
* Requires at least one backend instance group to be defined as a backup (failover) backend. For
* load balancers that have configurable failover: [Internal TCP/UDP Load
* Balancing](https://cloud.google.com/load-balancing/docs/internal/failover-overview) and
* [external TCP/UDP Load Balancing](https://cloud.google.com/load-balancing/docs/network
* /networklb-failover-overview).
* @param failoverPolicy failoverPolicy or {@code null} for none
*/
public BackendService setFailoverPolicy(BackendServiceFailoverPolicy failoverPolicy) {
this.failoverPolicy = failoverPolicy;
return this;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a BackendService. An up-to-
* date fingerprint must be provided in order to update the BackendService, otherwise the request
* will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request
* to retrieve a BackendService.
* @see #decodeFingerprint()
* @return value or {@code null} for none
*/
public java.lang.String getFingerprint() {
return fingerprint;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a BackendService. An up-to-
* date fingerprint must be provided in order to update the BackendService, otherwise the request
* will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request
* to retrieve a BackendService.
* @see #getFingerprint()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeFingerprint() {
return com.google.api.client.util.Base64.decodeBase64(fingerprint);
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a BackendService. An up-to-
* date fingerprint must be provided in order to update the BackendService, otherwise the request
* will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request
* to retrieve a BackendService.
* @see #encodeFingerprint()
* @param fingerprint fingerprint or {@code null} for none
*/
public BackendService setFingerprint(java.lang.String fingerprint) {
this.fingerprint = fingerprint;
return this;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a BackendService. An up-to-
* date fingerprint must be provided in order to update the BackendService, otherwise the request
* will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request
* to retrieve a BackendService.
* @see #setFingerprint()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public BackendService encodeFingerprint(byte[] fingerprint) {
this.fingerprint = com.google.api.client.util.Base64.encodeBase64URLSafeString(fingerprint);
return this;
}
/**
* The list of URLs to the healthChecks, httpHealthChecks (legacy), or httpsHealthChecks (legacy)
* resource for health checking this backend service. Not all backend services support legacy
* health checks. See Load balancer guide. Currently, at most one health check can be specified
* for each backend service. Backend services with instance group or zonal NEG backends must have
* a health check. Backend services with internet or serverless NEG backends must not have a
* health check.
* @return value or {@code null} for none
*/
public java.util.List getHealthChecks() {
return healthChecks;
}
/**
* The list of URLs to the healthChecks, httpHealthChecks (legacy), or httpsHealthChecks (legacy)
* resource for health checking this backend service. Not all backend services support legacy
* health checks. See Load balancer guide. Currently, at most one health check can be specified
* for each backend service. Backend services with instance group or zonal NEG backends must have
* a health check. Backend services with internet or serverless NEG backends must not have a
* health check.
* @param healthChecks healthChecks or {@code null} for none
*/
public BackendService setHealthChecks(java.util.List healthChecks) {
this.healthChecks = healthChecks;
return this;
}
/**
* The configurations for Identity-Aware Proxy on this resource. Not available for Internal
* TCP/UDP Load Balancing and Network Load Balancing.
* @return value or {@code null} for none
*/
public BackendServiceIAP getIap() {
return iap;
}
/**
* The configurations for Identity-Aware Proxy on this resource. Not available for Internal
* TCP/UDP Load Balancing and Network Load Balancing.
* @param iap iap or {@code null} for none
*/
public BackendService setIap(BackendServiceIAP iap) {
this.iap = iap;
return this;
}
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* @return value or {@code null} for none
*/
public java.math.BigInteger getId() {
return id;
}
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* @param id id or {@code null} for none
*/
public BackendService setId(java.math.BigInteger id) {
this.id = id;
return this;
}
/**
* [Output Only] Type of resource. Always compute#backendService for backend services.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* [Output Only] Type of resource. Always compute#backendService for backend services.
* @param kind kind or {@code null} for none
*/
public BackendService setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Specifies the load balancer type. A backend service created for one type of load balancer
* cannot be used with another. For more information, refer to Choosing a load balancer.
* @return value or {@code null} for none
*/
public java.lang.String getLoadBalancingScheme() {
return loadBalancingScheme;
}
/**
* Specifies the load balancer type. A backend service created for one type of load balancer
* cannot be used with another. For more information, refer to Choosing a load balancer.
* @param loadBalancingScheme loadBalancingScheme or {@code null} for none
*/
public BackendService setLoadBalancingScheme(java.lang.String loadBalancingScheme) {
this.loadBalancingScheme = loadBalancingScheme;
return this;
}
/**
* A list of locality load balancing policies to be used in order of preference. Either the policy
* or the customPolicy field should be set. Overrides any value set in the localityLbPolicy field.
* localityLbPolicies is only supported when the BackendService is referenced by a URL Map that is
* referenced by a target gRPC proxy that has the validateForProxyless field set to true.
* @return value or {@code null} for none
*/
public java.util.List getLocalityLbPolicies() {
return localityLbPolicies;
}
/**
* A list of locality load balancing policies to be used in order of preference. Either the policy
* or the customPolicy field should be set. Overrides any value set in the localityLbPolicy field.
* localityLbPolicies is only supported when the BackendService is referenced by a URL Map that is
* referenced by a target gRPC proxy that has the validateForProxyless field set to true.
* @param localityLbPolicies localityLbPolicies or {@code null} for none
*/
public BackendService setLocalityLbPolicies(java.util.List localityLbPolicies) {
this.localityLbPolicies = localityLbPolicies;
return this;
}
/**
* The load balancing algorithm used within the scope of the locality. The possible values are: -
* ROUND_ROBIN: This is a simple policy in which each healthy backend is selected in round robin
* order. This is the default. - LEAST_REQUEST: An O(1) algorithm which selects two random healthy
* hosts and picks the host which has fewer active requests. - RING_HASH: The ring/modulo hash
* load balancer implements consistent hashing to backends. The algorithm has the property that
* the addition/removal of a host from a set of N hosts only affects 1/N of the requests. -
* RANDOM: The load balancer selects a random healthy host. - ORIGINAL_DESTINATION: Backend host
* is selected based on the client connection metadata, i.e., connections are opened to the same
* address as the destination address of the incoming connection before the connection was
* redirected to the load balancer. - MAGLEV: used as a drop in replacement for the ring hash load
* balancer. Maglev is not as stable as ring hash but has faster table lookup build times and host
* selection times. For more information about Maglev, see
* https://ai.google/research/pubs/pub44824 This field is applicable to either: - A regional
* backend service with the service_protocol set to HTTP, HTTPS, or HTTP2, and
* load_balancing_scheme set to INTERNAL_MANAGED. - A global backend service with the
* load_balancing_scheme set to INTERNAL_SELF_MANAGED. If sessionAffinity is not NONE, and this
* field is not set to MAGLEV or RING_HASH, session affinity settings will not take effect. Only
* ROUND_ROBIN and RING_HASH are supported when the backend service is referenced by a URL map
* that is bound to target gRPC proxy that has validateForProxyless field set to true.
* @return value or {@code null} for none
*/
public java.lang.String getLocalityLbPolicy() {
return localityLbPolicy;
}
/**
* The load balancing algorithm used within the scope of the locality. The possible values are: -
* ROUND_ROBIN: This is a simple policy in which each healthy backend is selected in round robin
* order. This is the default. - LEAST_REQUEST: An O(1) algorithm which selects two random healthy
* hosts and picks the host which has fewer active requests. - RING_HASH: The ring/modulo hash
* load balancer implements consistent hashing to backends. The algorithm has the property that
* the addition/removal of a host from a set of N hosts only affects 1/N of the requests. -
* RANDOM: The load balancer selects a random healthy host. - ORIGINAL_DESTINATION: Backend host
* is selected based on the client connection metadata, i.e., connections are opened to the same
* address as the destination address of the incoming connection before the connection was
* redirected to the load balancer. - MAGLEV: used as a drop in replacement for the ring hash load
* balancer. Maglev is not as stable as ring hash but has faster table lookup build times and host
* selection times. For more information about Maglev, see
* https://ai.google/research/pubs/pub44824 This field is applicable to either: - A regional
* backend service with the service_protocol set to HTTP, HTTPS, or HTTP2, and
* load_balancing_scheme set to INTERNAL_MANAGED. - A global backend service with the
* load_balancing_scheme set to INTERNAL_SELF_MANAGED. If sessionAffinity is not NONE, and this
* field is not set to MAGLEV or RING_HASH, session affinity settings will not take effect. Only
* ROUND_ROBIN and RING_HASH are supported when the backend service is referenced by a URL map
* that is bound to target gRPC proxy that has validateForProxyless field set to true.
* @param localityLbPolicy localityLbPolicy or {@code null} for none
*/
public BackendService setLocalityLbPolicy(java.lang.String localityLbPolicy) {
this.localityLbPolicy = localityLbPolicy;
return this;
}
/**
* This field denotes the logging options for the load balancer traffic served by this backend
* service. If logging is enabled, logs will be exported to Stackdriver.
* @return value or {@code null} for none
*/
public BackendServiceLogConfig getLogConfig() {
return logConfig;
}
/**
* This field denotes the logging options for the load balancer traffic served by this backend
* service. If logging is enabled, logs will be exported to Stackdriver.
* @param logConfig logConfig or {@code null} for none
*/
public BackendService setLogConfig(BackendServiceLogConfig logConfig) {
this.logConfig = logConfig;
return this;
}
/**
* Specifies the default maximum duration (timeout) for streams to this service. Duration is
* computed from the beginning of the stream until the response has been completely processed,
* including all retries. A stream that does not complete in this duration is closed. If not
* specified, there will be no timeout limit, i.e. the maximum duration is infinite. This value
* can be overridden in the PathMatcher configuration of the UrlMap that references this backend
* service. This field is only allowed when the loadBalancingScheme of the backend service is
* INTERNAL_SELF_MANAGED.
* @return value or {@code null} for none
*/
public Duration getMaxStreamDuration() {
return maxStreamDuration;
}
/**
* Specifies the default maximum duration (timeout) for streams to this service. Duration is
* computed from the beginning of the stream until the response has been completely processed,
* including all retries. A stream that does not complete in this duration is closed. If not
* specified, there will be no timeout limit, i.e. the maximum duration is infinite. This value
* can be overridden in the PathMatcher configuration of the UrlMap that references this backend
* service. This field is only allowed when the loadBalancingScheme of the backend service is
* INTERNAL_SELF_MANAGED.
* @param maxStreamDuration maxStreamDuration or {@code null} for none
*/
public BackendService setMaxStreamDuration(Duration maxStreamDuration) {
this.maxStreamDuration = maxStreamDuration;
return this;
}
/**
* Name of the resource. Provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the resource. Provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash.
* @param name name or {@code null} for none
*/
public BackendService setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The URL of the network to which this backend service belongs. This field can only be specified
* when the load balancing scheme is set to INTERNAL.
* @return value or {@code null} for none
*/
public java.lang.String getNetwork() {
return network;
}
/**
* The URL of the network to which this backend service belongs. This field can only be specified
* when the load balancing scheme is set to INTERNAL.
* @param network network or {@code null} for none
*/
public BackendService setNetwork(java.lang.String network) {
this.network = network;
return this;
}
/**
* Settings controlling the eviction of unhealthy hosts from the load balancing pool for the
* backend service. If not set, this feature is considered disabled. This field is applicable to
* either: - A regional backend service with the service_protocol set to HTTP, HTTPS, or HTTP2,
* and load_balancing_scheme set to INTERNAL_MANAGED. - A global backend service with the
* load_balancing_scheme set to INTERNAL_SELF_MANAGED. Not supported when the backend service is
* referenced by a URL map that is bound to target gRPC proxy that has validateForProxyless field
* set to true.
* @return value or {@code null} for none
*/
public OutlierDetection getOutlierDetection() {
return outlierDetection;
}
/**
* Settings controlling the eviction of unhealthy hosts from the load balancing pool for the
* backend service. If not set, this feature is considered disabled. This field is applicable to
* either: - A regional backend service with the service_protocol set to HTTP, HTTPS, or HTTP2,
* and load_balancing_scheme set to INTERNAL_MANAGED. - A global backend service with the
* load_balancing_scheme set to INTERNAL_SELF_MANAGED. Not supported when the backend service is
* referenced by a URL map that is bound to target gRPC proxy that has validateForProxyless field
* set to true.
* @param outlierDetection outlierDetection or {@code null} for none
*/
public BackendService setOutlierDetection(OutlierDetection outlierDetection) {
this.outlierDetection = outlierDetection;
return this;
}
/**
* Deprecated in favor of portName. The TCP port to connect on the backend. The default value is
* 80. For Internal TCP/UDP Load Balancing and Network Load Balancing, omit port.
* @return value or {@code null} for none
*/
public java.lang.Integer getPort() {
return port;
}
/**
* Deprecated in favor of portName. The TCP port to connect on the backend. The default value is
* 80. For Internal TCP/UDP Load Balancing and Network Load Balancing, omit port.
* @param port port or {@code null} for none
*/
public BackendService setPort(java.lang.Integer port) {
this.port = port;
return this;
}
/**
* A named port on a backend instance group representing the port for communication to the backend
* VMs in that group. The named port must be [defined on each backend instance
* group](https://cloud.google.com/load-balancing/docs/backend-service#named_ports). This
* parameter has no meaning if the backends are NEGs. For Internal TCP/UDP Load Balancing and
* Network Load Balancing, omit port_name.
* @return value or {@code null} for none
*/
public java.lang.String getPortName() {
return portName;
}
/**
* A named port on a backend instance group representing the port for communication to the backend
* VMs in that group. The named port must be [defined on each backend instance
* group](https://cloud.google.com/load-balancing/docs/backend-service#named_ports). This
* parameter has no meaning if the backends are NEGs. For Internal TCP/UDP Load Balancing and
* Network Load Balancing, omit port_name.
* @param portName portName or {@code null} for none
*/
public BackendService setPortName(java.lang.String portName) {
this.portName = portName;
return this;
}
/**
* The protocol this BackendService uses to communicate with backends. Possible values are HTTP,
* HTTPS, HTTP2, TCP, SSL, UDP or GRPC. depending on the chosen load balancer or Traffic Director
* configuration. Refer to the documentation for the load balancers or for Traffic Director for
* more information. Must be set to GRPC when the backend service is referenced by a URL map that
* is bound to target gRPC proxy.
* @return value or {@code null} for none
*/
public java.lang.String getProtocol() {
return protocol;
}
/**
* The protocol this BackendService uses to communicate with backends. Possible values are HTTP,
* HTTPS, HTTP2, TCP, SSL, UDP or GRPC. depending on the chosen load balancer or Traffic Director
* configuration. Refer to the documentation for the load balancers or for Traffic Director for
* more information. Must be set to GRPC when the backend service is referenced by a URL map that
* is bound to target gRPC proxy.
* @param protocol protocol or {@code null} for none
*/
public BackendService setProtocol(java.lang.String protocol) {
this.protocol = protocol;
return this;
}
/**
* [Output Only] URL of the region where the regional backend service resides. This field is not
* applicable to global backend services. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* @return value or {@code null} for none
*/
public java.lang.String getRegion() {
return region;
}
/**
* [Output Only] URL of the region where the regional backend service resides. This field is not
* applicable to global backend services. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* @param region region or {@code null} for none
*/
public BackendService setRegion(java.lang.String region) {
this.region = region;
return this;
}
/**
* [Output Only] The resource URL for the security policy associated with this backend service.
* @return value or {@code null} for none
*/
public java.lang.String getSecurityPolicy() {
return securityPolicy;
}
/**
* [Output Only] The resource URL for the security policy associated with this backend service.
* @param securityPolicy securityPolicy or {@code null} for none
*/
public BackendService setSecurityPolicy(java.lang.String securityPolicy) {
this.securityPolicy = securityPolicy;
return this;
}
/**
* This field specifies the security settings that apply to this backend service. This field is
* applicable to a global backend service with the load_balancing_scheme set to
* INTERNAL_SELF_MANAGED.
* @return value or {@code null} for none
*/
public SecuritySettings getSecuritySettings() {
return securitySettings;
}
/**
* This field specifies the security settings that apply to this backend service. This field is
* applicable to a global backend service with the load_balancing_scheme set to
* INTERNAL_SELF_MANAGED.
* @param securitySettings securitySettings or {@code null} for none
*/
public BackendService setSecuritySettings(SecuritySettings securitySettings) {
this.securitySettings = securitySettings;
return this;
}
/**
* [Output Only] Server-defined URL for the resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* [Output Only] Server-defined URL for the resource.
* @param selfLink selfLink or {@code null} for none
*/
public BackendService setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* URLs of networkservices.ServiceBinding resources. Can only be set if load balancing scheme is
* INTERNAL_SELF_MANAGED. If set, lists of backends and health checks must be both empty.
* @return value or {@code null} for none
*/
public java.util.List getServiceBindings() {
return serviceBindings;
}
/**
* URLs of networkservices.ServiceBinding resources. Can only be set if load balancing scheme is
* INTERNAL_SELF_MANAGED. If set, lists of backends and health checks must be both empty.
* @param serviceBindings serviceBindings or {@code null} for none
*/
public BackendService setServiceBindings(java.util.List serviceBindings) {
this.serviceBindings = serviceBindings;
return this;
}
/**
* Type of session affinity to use. The default is NONE. Only NONE and HEADER_FIELD are supported
* when the backend service is referenced by a URL map that is bound to target gRPC proxy that has
* validateForProxyless field set to true. For more details, see: [Session
* Affinity](https://cloud.google.com/load-balancing/docs/backend-service#session_affinity).
* @return value or {@code null} for none
*/
public java.lang.String getSessionAffinity() {
return sessionAffinity;
}
/**
* Type of session affinity to use. The default is NONE. Only NONE and HEADER_FIELD are supported
* when the backend service is referenced by a URL map that is bound to target gRPC proxy that has
* validateForProxyless field set to true. For more details, see: [Session
* Affinity](https://cloud.google.com/load-balancing/docs/backend-service#session_affinity).
* @param sessionAffinity sessionAffinity or {@code null} for none
*/
public BackendService setSessionAffinity(java.lang.String sessionAffinity) {
this.sessionAffinity = sessionAffinity;
return this;
}
/**
* @return value or {@code null} for none
*/
public Subsetting getSubsetting() {
return subsetting;
}
/**
* @param subsetting subsetting or {@code null} for none
*/
public BackendService setSubsetting(Subsetting subsetting) {
this.subsetting = subsetting;
return this;
}
/**
* The backend service timeout has a different meaning depending on the type of load balancer. For
* more information see, Backend service settings. The default is 30 seconds. The full range of
* timeout values allowed goes from 1 through 2,147,483,647 seconds. This value can be overridden
* in the PathMatcher configuration of the UrlMap that references this backend service. Not
* supported when the backend service is referenced by a URL map that is bound to target gRPC
* proxy that has validateForProxyless field set to true. Instead, use maxStreamDuration.
* @return value or {@code null} for none
*/
public java.lang.Integer getTimeoutSec() {
return timeoutSec;
}
/**
* The backend service timeout has a different meaning depending on the type of load balancer. For
* more information see, Backend service settings. The default is 30 seconds. The full range of
* timeout values allowed goes from 1 through 2,147,483,647 seconds. This value can be overridden
* in the PathMatcher configuration of the UrlMap that references this backend service. Not
* supported when the backend service is referenced by a URL map that is bound to target gRPC
* proxy that has validateForProxyless field set to true. Instead, use maxStreamDuration.
* @param timeoutSec timeoutSec or {@code null} for none
*/
public BackendService setTimeoutSec(java.lang.Integer timeoutSec) {
this.timeoutSec = timeoutSec;
return this;
}
@Override
public BackendService set(String fieldName, Object value) {
return (BackendService) super.set(fieldName, value);
}
@Override
public BackendService clone() {
return (BackendService) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy