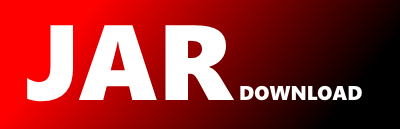
com.google.api.services.compute.model.BfdStatus Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Next free: 15
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class BfdStatus extends com.google.api.client.json.GenericJson {
/**
* The BFD session initialization mode for this BGP peer. If set to ACTIVE, the Cloud Router will
* initiate the BFD session for this BGP peer. If set to PASSIVE, the Cloud Router will wait for
* the peer router to initiate the BFD session for this BGP peer. If set to DISABLED, BFD is
* disabled for this BGP peer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String bfdSessionInitializationMode;
/**
* Unix timestamp of the most recent config update.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long configUpdateTimestampMicros;
/**
* Control packet counts for the current BFD session.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BfdStatusPacketCounts controlPacketCounts;
/**
* Inter-packet time interval statistics for control packets.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List controlPacketIntervals;
/**
* The diagnostic code specifies the local system's reason for the last change in session state.
* This allows remote systems to determine the reason that the previous session failed, for
* example. These diagnostic codes are specified in section 4.1 of RFC5880
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String localDiagnostic;
/**
* The current BFD session state as seen by the transmitting system. These states are specified in
* section 4.1 of RFC5880
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String localState;
/**
* Negotiated transmit interval for control packets.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long negotiatedLocalControlTxIntervalMs;
/**
* The most recent Rx control packet for this BFD session.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BfdPacket rxPacket;
/**
* The most recent Tx control packet for this BFD session.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BfdPacket txPacket;
/**
* Session uptime in milliseconds. Value will be 0 if session is not up.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long uptimeMs;
/**
* The BFD session initialization mode for this BGP peer. If set to ACTIVE, the Cloud Router will
* initiate the BFD session for this BGP peer. If set to PASSIVE, the Cloud Router will wait for
* the peer router to initiate the BFD session for this BGP peer. If set to DISABLED, BFD is
* disabled for this BGP peer.
* @return value or {@code null} for none
*/
public java.lang.String getBfdSessionInitializationMode() {
return bfdSessionInitializationMode;
}
/**
* The BFD session initialization mode for this BGP peer. If set to ACTIVE, the Cloud Router will
* initiate the BFD session for this BGP peer. If set to PASSIVE, the Cloud Router will wait for
* the peer router to initiate the BFD session for this BGP peer. If set to DISABLED, BFD is
* disabled for this BGP peer.
* @param bfdSessionInitializationMode bfdSessionInitializationMode or {@code null} for none
*/
public BfdStatus setBfdSessionInitializationMode(java.lang.String bfdSessionInitializationMode) {
this.bfdSessionInitializationMode = bfdSessionInitializationMode;
return this;
}
/**
* Unix timestamp of the most recent config update.
* @return value or {@code null} for none
*/
public java.lang.Long getConfigUpdateTimestampMicros() {
return configUpdateTimestampMicros;
}
/**
* Unix timestamp of the most recent config update.
* @param configUpdateTimestampMicros configUpdateTimestampMicros or {@code null} for none
*/
public BfdStatus setConfigUpdateTimestampMicros(java.lang.Long configUpdateTimestampMicros) {
this.configUpdateTimestampMicros = configUpdateTimestampMicros;
return this;
}
/**
* Control packet counts for the current BFD session.
* @return value or {@code null} for none
*/
public BfdStatusPacketCounts getControlPacketCounts() {
return controlPacketCounts;
}
/**
* Control packet counts for the current BFD session.
* @param controlPacketCounts controlPacketCounts or {@code null} for none
*/
public BfdStatus setControlPacketCounts(BfdStatusPacketCounts controlPacketCounts) {
this.controlPacketCounts = controlPacketCounts;
return this;
}
/**
* Inter-packet time interval statistics for control packets.
* @return value or {@code null} for none
*/
public java.util.List getControlPacketIntervals() {
return controlPacketIntervals;
}
/**
* Inter-packet time interval statistics for control packets.
* @param controlPacketIntervals controlPacketIntervals or {@code null} for none
*/
public BfdStatus setControlPacketIntervals(java.util.List controlPacketIntervals) {
this.controlPacketIntervals = controlPacketIntervals;
return this;
}
/**
* The diagnostic code specifies the local system's reason for the last change in session state.
* This allows remote systems to determine the reason that the previous session failed, for
* example. These diagnostic codes are specified in section 4.1 of RFC5880
* @return value or {@code null} for none
*/
public java.lang.String getLocalDiagnostic() {
return localDiagnostic;
}
/**
* The diagnostic code specifies the local system's reason for the last change in session state.
* This allows remote systems to determine the reason that the previous session failed, for
* example. These diagnostic codes are specified in section 4.1 of RFC5880
* @param localDiagnostic localDiagnostic or {@code null} for none
*/
public BfdStatus setLocalDiagnostic(java.lang.String localDiagnostic) {
this.localDiagnostic = localDiagnostic;
return this;
}
/**
* The current BFD session state as seen by the transmitting system. These states are specified in
* section 4.1 of RFC5880
* @return value or {@code null} for none
*/
public java.lang.String getLocalState() {
return localState;
}
/**
* The current BFD session state as seen by the transmitting system. These states are specified in
* section 4.1 of RFC5880
* @param localState localState or {@code null} for none
*/
public BfdStatus setLocalState(java.lang.String localState) {
this.localState = localState;
return this;
}
/**
* Negotiated transmit interval for control packets.
* @return value or {@code null} for none
*/
public java.lang.Long getNegotiatedLocalControlTxIntervalMs() {
return negotiatedLocalControlTxIntervalMs;
}
/**
* Negotiated transmit interval for control packets.
* @param negotiatedLocalControlTxIntervalMs negotiatedLocalControlTxIntervalMs or {@code null} for none
*/
public BfdStatus setNegotiatedLocalControlTxIntervalMs(java.lang.Long negotiatedLocalControlTxIntervalMs) {
this.negotiatedLocalControlTxIntervalMs = negotiatedLocalControlTxIntervalMs;
return this;
}
/**
* The most recent Rx control packet for this BFD session.
* @return value or {@code null} for none
*/
public BfdPacket getRxPacket() {
return rxPacket;
}
/**
* The most recent Rx control packet for this BFD session.
* @param rxPacket rxPacket or {@code null} for none
*/
public BfdStatus setRxPacket(BfdPacket rxPacket) {
this.rxPacket = rxPacket;
return this;
}
/**
* The most recent Tx control packet for this BFD session.
* @return value or {@code null} for none
*/
public BfdPacket getTxPacket() {
return txPacket;
}
/**
* The most recent Tx control packet for this BFD session.
* @param txPacket txPacket or {@code null} for none
*/
public BfdStatus setTxPacket(BfdPacket txPacket) {
this.txPacket = txPacket;
return this;
}
/**
* Session uptime in milliseconds. Value will be 0 if session is not up.
* @return value or {@code null} for none
*/
public java.lang.Long getUptimeMs() {
return uptimeMs;
}
/**
* Session uptime in milliseconds. Value will be 0 if session is not up.
* @param uptimeMs uptimeMs or {@code null} for none
*/
public BfdStatus setUptimeMs(java.lang.Long uptimeMs) {
this.uptimeMs = uptimeMs;
return this;
}
@Override
public BfdStatus set(String fieldName, Object value) {
return (BfdStatus) super.set(fieldName, value);
}
@Override
public BfdStatus clone() {
return (BfdStatus) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy