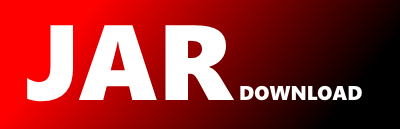
com.google.api.services.compute.model.ForwardingRule Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Represents a Forwarding Rule resource. Forwarding rule resources in Google Cloud can be either
* regional or global in scope: *
* [Global](https://cloud.google.com/compute/docs/reference/rest/beta/globalForwardingRules) *
* [Regional](https://cloud.google.com/compute/docs/reference/rest/beta/forwardingRules) A
* forwarding rule and its corresponding IP address represent the frontend configuration of a Google
* Cloud Platform load balancer. Forwarding rules can also reference target instances and Cloud VPN
* Classic gateways (targetVpnGateway). For more information, read Forwarding rule concepts and
* Using protocol forwarding.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ForwardingRule extends com.google.api.client.json.GenericJson {
/**
* IP address for which this forwarding rule accepts traffic. When a client sends traffic to this
* IP address, the forwarding rule directs the traffic to the referenced target or backendService.
* While creating a forwarding rule, specifying an IPAddress is required under the following
* circumstances: - When the target is set to targetGrpcProxy and validateForProxyless is set to
* true, the IPAddress should be set to 0.0.0.0. - When the target is a Private Service Connect
* Google APIs bundle, you must specify an IPAddress. Otherwise, you can optionally specify an IP
* address that references an existing static (reserved) IP address resource. When omitted, Google
* Cloud assigns an ephemeral IP address. Use one of the following formats to specify an IP
* address while creating a forwarding rule: * IP address number, as in `100.1.2.3` * Full
* resource URL, as in https://www.googleapis.com/compute/v1/projects/project_id/regions/region
* /addresses/address-name * Partial URL or by name, as in: -
* projects/project_id/regions/region/addresses/address-name - regions/region/addresses/address-
* name - global/addresses/address-name - address-name The forwarding rule's target or
* backendService, and in most cases, also the loadBalancingScheme, determine the type of IP
* address that you can use. For detailed information, see [IP address
* specifications](https://cloud.google.com/load-balancing/docs/forwarding-rule-
* concepts#ip_address_specifications). When reading an IPAddress, the API always returns the IP
* address number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("IPAddress")
private java.lang.String iPAddress;
/**
* The IP protocol to which this rule applies. For protocol forwarding, valid options are TCP,
* UDP, ESP, AH, SCTP, ICMP and L3_DEFAULT. The valid IP protocols are different for different
* load balancing products as described in [Load balancing features](https://cloud.google.com
* /load-balancing/docs/features#protocols_from_the_load_balancer_to_the_backends).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("IPProtocol")
private java.lang.String iPProtocol;
/**
* This field is used along with the backend_service field for Internal TCP/UDP Load Balancing or
* Network Load Balancing, or with the target field for internal and external TargetInstance. You
* can only use one of ports and port_range, or allPorts. The three are mutually exclusive. For
* TCP, UDP and SCTP traffic, packets addressed to any ports will be forwarded to the target or
* backendService.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allPorts;
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. If the field is set to TRUE, clients can access ILB
* from all regions. Otherwise only allows access from clients in the same region as the internal
* load balancer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowGlobalAccess;
/**
* Identifies the backend service to which the forwarding rule sends traffic. Required for
* Internal TCP/UDP Load Balancing and Network Load Balancing; must be omitted for all other load
* balancer types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String backendService;
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creationTimestamp;
/**
* An optional description of this resource. Provide this property when you create the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request. To see the latest fingerprint, make a get() request to retrieve a
* ForwardingRule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fingerprint;
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger id;
/**
* The IP Version that will be used by this forwarding rule. Valid options are IPV4 or IPV6.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ipVersion;
/**
* Indicates whether or not this load balancer can be used as a collector for packet mirroring. To
* prevent mirroring loops, instances behind this load balancer will not have their traffic
* mirrored even if a PacketMirroring rule applies to them. This can only be set to true for load
* balancers that have their loadBalancingScheme set to INTERNAL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isMirroringCollector;
/**
* [Output Only] Type of the resource. Always compute#forwardingRule for Forwarding Rule
* resources.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to
* retrieve a ForwardingRule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String labelFingerprint;
/**
* Labels for this resource. These can only be added or modified by the setLabels method. Each
* label key/value pair must comply with RFC1035. Label values may be empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Specifies the forwarding rule type. For more information about forwarding rules, refer to
* Forwarding rule concepts.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String loadBalancingScheme;
/**
* Opaque filter criteria used by load balancer to restrict routing configuration to a limited set
* of xDS compliant clients. In their xDS requests to load balancer, xDS clients present node
* metadata. When there is a match, the relevant configuration is made available to those proxies.
* Otherwise, all the resources (e.g. TargetHttpProxy, UrlMap) referenced by the ForwardingRule
* are not visible to those proxies. For each metadataFilter in this list, if its
* filterMatchCriteria is set to MATCH_ANY, at least one of the filterLabels must match the
* corresponding label provided in the metadata. If its filterMatchCriteria is set to MATCH_ALL,
* then all of its filterLabels must match with corresponding labels provided in the metadata. If
* multiple metadataFilters are specified, all of them need to be satisfied in order to be
* considered a match. metadataFilters specified here will be applifed before those specified in
* the UrlMap that this ForwardingRule references. metadataFilters only applies to Loadbalancers
* that have their loadBalancingScheme set to INTERNAL_SELF_MANAGED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List metadataFilters;
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash. For Private Service
* Connect forwarding rules that forward traffic to Google APIs, the forwarding rule name must be
* a 1-20 characters string with lowercase letters and numbers and must start with a letter.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* This field is not used for external load balancing. For Internal TCP/UDP Load Balancing, this
* field identifies the network that the load balanced IP should belong to for this Forwarding
* Rule. If this field is not specified, the default network will be used. For Private Service
* Connect forwarding rules that forward traffic to Google APIs, a network must be provided.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String network;
/**
* This signifies the networking tier used for configuring this load balancer and can only take
* the following values: PREMIUM, STANDARD. For regional ForwardingRule, the valid values are
* PREMIUM and STANDARD. For GlobalForwardingRule, the valid value is PREMIUM. If this field is
* not specified, it is assumed to be PREMIUM. If IPAddress is specified, this value must be equal
* to the networkTier of the Address.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String networkTier;
/**
* This is used in PSC consumer ForwardingRule to control whether it should try to auto-generate a
* DNS zone or not. Non-PSC forwarding rules do not use this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean noAutomateDnsZone;
/**
* This field can be used only if: - Load balancing scheme is one of EXTERNAL,
* INTERNAL_SELF_MANAGED or INTERNAL_MANAGED - IPProtocol is one of TCP, UDP, or SCTP. Packets
* addressed to ports in the specified range will be forwarded to target or backend_service. You
* can only use one of ports, port_range, or allPorts. The three are mutually exclusive.
* Forwarding rules with the same [IPAddress, IPProtocol] pair must have disjoint ports. Some
* types of forwarding target have constraints on the acceptable ports. For more information, see
* [Port specifications](https://cloud.google.com/load-balancing/docs/forwarding-rule-
* concepts#port_specifications). @pattern: \\d+(?:-\\d+)?
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String portRange;
/**
* The ports field is only supported when the forwarding rule references a backend_service
* directly. Only packets addressed to the [specified list of ports]((https://cloud.google.com
* /load-balancing/docs/forwarding-rule-concepts#port_specifications)) are forwarded to backends.
* You can only use one of ports and port_range, or allPorts. The three are mutually exclusive.
* You can specify a list of up to five ports, which can be non-contiguous. Forwarding rules with
* the same [IPAddress, IPProtocol] pair must have disjoint ports. @pattern: \\d+(?:-\\d+)?
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List ports;
/**
* [Output Only] The PSC connection id of the PSC Forwarding Rule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger pscConnectionId;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pscConnectionStatus;
/**
* [Output Only] URL of the region where the regional forwarding rule resides. This field is not
* applicable to global forwarding rules. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String region;
/**
* [Output Only] Server-defined URL for the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Service Directory resources to register this forwarding rule with. Currently, only supports a
* single Service Directory resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List serviceDirectoryRegistrations;
/**
* An optional prefix to the service name for this Forwarding Rule. If specified, the prefix is
* the first label of the fully qualified service name. The label must be 1-63 characters long,
* and comply with RFC1035. Specifically, the label must be 1-63 characters long and match the
* regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a
* lowercase letter, and all following characters must be a dash, lowercase letter, or digit,
* except the last character, which cannot be a dash. This field is only used for internal load
* balancing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceLabel;
/**
* [Output Only] The internal fully qualified service name for this Forwarding Rule. This field is
* only used for internal load balancing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceName;
/**
* If not empty, this Forwarding Rule will only forward the traffic when the source IP address
* matches one of the IP addresses or CIDR ranges set here. Note that a Forwarding Rule can only
* have up to 64 source IP ranges, and this field can only be used with a regional Forwarding Rule
* whose scheme is EXTERNAL. Each source_ip_range entry should be either an IP address (for
* example, 1.2.3.4) or a CIDR range (for example, 1.2.3.0/24).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sourceIpRanges;
/**
* This field identifies the subnetwork that the load balanced IP should belong to for this
* Forwarding Rule, used in internal load balancing and network load balancing with IPv6. If the
* network specified is in auto subnet mode, this field is optional. However, a subnetwork must be
* specified if the network is in custom subnet mode or when creating external forwarding rule
* with IPv6.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String subnetwork;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String target;
/**
* IP address for which this forwarding rule accepts traffic. When a client sends traffic to this
* IP address, the forwarding rule directs the traffic to the referenced target or backendService.
* While creating a forwarding rule, specifying an IPAddress is required under the following
* circumstances: - When the target is set to targetGrpcProxy and validateForProxyless is set to
* true, the IPAddress should be set to 0.0.0.0. - When the target is a Private Service Connect
* Google APIs bundle, you must specify an IPAddress. Otherwise, you can optionally specify an IP
* address that references an existing static (reserved) IP address resource. When omitted, Google
* Cloud assigns an ephemeral IP address. Use one of the following formats to specify an IP
* address while creating a forwarding rule: * IP address number, as in `100.1.2.3` * Full
* resource URL, as in https://www.googleapis.com/compute/v1/projects/project_id/regions/region
* /addresses/address-name * Partial URL or by name, as in: -
* projects/project_id/regions/region/addresses/address-name - regions/region/addresses/address-
* name - global/addresses/address-name - address-name The forwarding rule's target or
* backendService, and in most cases, also the loadBalancingScheme, determine the type of IP
* address that you can use. For detailed information, see [IP address
* specifications](https://cloud.google.com/load-balancing/docs/forwarding-rule-
* concepts#ip_address_specifications). When reading an IPAddress, the API always returns the IP
* address number.
* @return value or {@code null} for none
*/
public java.lang.String getIPAddress() {
return iPAddress;
}
/**
* IP address for which this forwarding rule accepts traffic. When a client sends traffic to this
* IP address, the forwarding rule directs the traffic to the referenced target or backendService.
* While creating a forwarding rule, specifying an IPAddress is required under the following
* circumstances: - When the target is set to targetGrpcProxy and validateForProxyless is set to
* true, the IPAddress should be set to 0.0.0.0. - When the target is a Private Service Connect
* Google APIs bundle, you must specify an IPAddress. Otherwise, you can optionally specify an IP
* address that references an existing static (reserved) IP address resource. When omitted, Google
* Cloud assigns an ephemeral IP address. Use one of the following formats to specify an IP
* address while creating a forwarding rule: * IP address number, as in `100.1.2.3` * Full
* resource URL, as in https://www.googleapis.com/compute/v1/projects/project_id/regions/region
* /addresses/address-name * Partial URL or by name, as in: -
* projects/project_id/regions/region/addresses/address-name - regions/region/addresses/address-
* name - global/addresses/address-name - address-name The forwarding rule's target or
* backendService, and in most cases, also the loadBalancingScheme, determine the type of IP
* address that you can use. For detailed information, see [IP address
* specifications](https://cloud.google.com/load-balancing/docs/forwarding-rule-
* concepts#ip_address_specifications). When reading an IPAddress, the API always returns the IP
* address number.
* @param iPAddress iPAddress or {@code null} for none
*/
public ForwardingRule setIPAddress(java.lang.String iPAddress) {
this.iPAddress = iPAddress;
return this;
}
/**
* The IP protocol to which this rule applies. For protocol forwarding, valid options are TCP,
* UDP, ESP, AH, SCTP, ICMP and L3_DEFAULT. The valid IP protocols are different for different
* load balancing products as described in [Load balancing features](https://cloud.google.com
* /load-balancing/docs/features#protocols_from_the_load_balancer_to_the_backends).
* @return value or {@code null} for none
*/
public java.lang.String getIPProtocol() {
return iPProtocol;
}
/**
* The IP protocol to which this rule applies. For protocol forwarding, valid options are TCP,
* UDP, ESP, AH, SCTP, ICMP and L3_DEFAULT. The valid IP protocols are different for different
* load balancing products as described in [Load balancing features](https://cloud.google.com
* /load-balancing/docs/features#protocols_from_the_load_balancer_to_the_backends).
* @param iPProtocol iPProtocol or {@code null} for none
*/
public ForwardingRule setIPProtocol(java.lang.String iPProtocol) {
this.iPProtocol = iPProtocol;
return this;
}
/**
* This field is used along with the backend_service field for Internal TCP/UDP Load Balancing or
* Network Load Balancing, or with the target field for internal and external TargetInstance. You
* can only use one of ports and port_range, or allPorts. The three are mutually exclusive. For
* TCP, UDP and SCTP traffic, packets addressed to any ports will be forwarded to the target or
* backendService.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllPorts() {
return allPorts;
}
/**
* This field is used along with the backend_service field for Internal TCP/UDP Load Balancing or
* Network Load Balancing, or with the target field for internal and external TargetInstance. You
* can only use one of ports and port_range, or allPorts. The three are mutually exclusive. For
* TCP, UDP and SCTP traffic, packets addressed to any ports will be forwarded to the target or
* backendService.
* @param allPorts allPorts or {@code null} for none
*/
public ForwardingRule setAllPorts(java.lang.Boolean allPorts) {
this.allPorts = allPorts;
return this;
}
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. If the field is set to TRUE, clients can access ILB
* from all regions. Otherwise only allows access from clients in the same region as the internal
* load balancer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllowGlobalAccess() {
return allowGlobalAccess;
}
/**
* This field is used along with the backend_service field for internal load balancing or with the
* target field for internal TargetInstance. If the field is set to TRUE, clients can access ILB
* from all regions. Otherwise only allows access from clients in the same region as the internal
* load balancer.
* @param allowGlobalAccess allowGlobalAccess or {@code null} for none
*/
public ForwardingRule setAllowGlobalAccess(java.lang.Boolean allowGlobalAccess) {
this.allowGlobalAccess = allowGlobalAccess;
return this;
}
/**
* Identifies the backend service to which the forwarding rule sends traffic. Required for
* Internal TCP/UDP Load Balancing and Network Load Balancing; must be omitted for all other load
* balancer types.
* @return value or {@code null} for none
*/
public java.lang.String getBackendService() {
return backendService;
}
/**
* Identifies the backend service to which the forwarding rule sends traffic. Required for
* Internal TCP/UDP Load Balancing and Network Load Balancing; must be omitted for all other load
* balancer types.
* @param backendService backendService or {@code null} for none
*/
public ForwardingRule setBackendService(java.lang.String backendService) {
this.backendService = backendService;
return this;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @return value or {@code null} for none
*/
public java.lang.String getCreationTimestamp() {
return creationTimestamp;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public ForwardingRule setCreationTimestamp(java.lang.String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
* @param description description or {@code null} for none
*/
public ForwardingRule setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request. To see the latest fingerprint, make a get() request to retrieve a
* ForwardingRule.
* @see #decodeFingerprint()
* @return value or {@code null} for none
*/
public java.lang.String getFingerprint() {
return fingerprint;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request. To see the latest fingerprint, make a get() request to retrieve a
* ForwardingRule.
* @see #getFingerprint()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeFingerprint() {
return com.google.api.client.util.Base64.decodeBase64(fingerprint);
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request. To see the latest fingerprint, make a get() request to retrieve a
* ForwardingRule.
* @see #encodeFingerprint()
* @param fingerprint fingerprint or {@code null} for none
*/
public ForwardingRule setFingerprint(java.lang.String fingerprint) {
this.fingerprint = fingerprint;
return this;
}
/**
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used
* in optimistic locking. This field will be ignored when inserting a ForwardingRule. Include the
* fingerprint in patch request to ensure that you do not overwrite changes that were applied from
* another concurrent request. To see the latest fingerprint, make a get() request to retrieve a
* ForwardingRule.
* @see #setFingerprint()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public ForwardingRule encodeFingerprint(byte[] fingerprint) {
this.fingerprint = com.google.api.client.util.Base64.encodeBase64URLSafeString(fingerprint);
return this;
}
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* @return value or {@code null} for none
*/
public java.math.BigInteger getId() {
return id;
}
/**
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
* @param id id or {@code null} for none
*/
public ForwardingRule setId(java.math.BigInteger id) {
this.id = id;
return this;
}
/**
* The IP Version that will be used by this forwarding rule. Valid options are IPV4 or IPV6.
* @return value or {@code null} for none
*/
public java.lang.String getIpVersion() {
return ipVersion;
}
/**
* The IP Version that will be used by this forwarding rule. Valid options are IPV4 or IPV6.
* @param ipVersion ipVersion or {@code null} for none
*/
public ForwardingRule setIpVersion(java.lang.String ipVersion) {
this.ipVersion = ipVersion;
return this;
}
/**
* Indicates whether or not this load balancer can be used as a collector for packet mirroring. To
* prevent mirroring loops, instances behind this load balancer will not have their traffic
* mirrored even if a PacketMirroring rule applies to them. This can only be set to true for load
* balancers that have their loadBalancingScheme set to INTERNAL.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsMirroringCollector() {
return isMirroringCollector;
}
/**
* Indicates whether or not this load balancer can be used as a collector for packet mirroring. To
* prevent mirroring loops, instances behind this load balancer will not have their traffic
* mirrored even if a PacketMirroring rule applies to them. This can only be set to true for load
* balancers that have their loadBalancingScheme set to INTERNAL.
* @param isMirroringCollector isMirroringCollector or {@code null} for none
*/
public ForwardingRule setIsMirroringCollector(java.lang.Boolean isMirroringCollector) {
this.isMirroringCollector = isMirroringCollector;
return this;
}
/**
* [Output Only] Type of the resource. Always compute#forwardingRule for Forwarding Rule
* resources.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* [Output Only] Type of the resource. Always compute#forwardingRule for Forwarding Rule
* resources.
* @param kind kind or {@code null} for none
*/
public ForwardingRule setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to
* retrieve a ForwardingRule.
* @see #decodeLabelFingerprint()
* @return value or {@code null} for none
*/
public java.lang.String getLabelFingerprint() {
return labelFingerprint;
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to
* retrieve a ForwardingRule.
* @see #getLabelFingerprint()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeLabelFingerprint() {
return com.google.api.client.util.Base64.decodeBase64(labelFingerprint);
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to
* retrieve a ForwardingRule.
* @see #encodeLabelFingerprint()
* @param labelFingerprint labelFingerprint or {@code null} for none
*/
public ForwardingRule setLabelFingerprint(java.lang.String labelFingerprint) {
this.labelFingerprint = labelFingerprint;
return this;
}
/**
* A fingerprint for the labels being applied to this resource, which is essentially a hash of the
* labels set used for optimistic locking. The fingerprint is initially generated by Compute
* Engine and changes after every request to modify or update labels. You must always provide an
* up-to-date fingerprint hash in order to update or change labels, otherwise the request will
* fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to
* retrieve a ForwardingRule.
* @see #setLabelFingerprint()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public ForwardingRule encodeLabelFingerprint(byte[] labelFingerprint) {
this.labelFingerprint = com.google.api.client.util.Base64.encodeBase64URLSafeString(labelFingerprint);
return this;
}
/**
* Labels for this resource. These can only be added or modified by the setLabels method. Each
* label key/value pair must comply with RFC1035. Label values may be empty.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Labels for this resource. These can only be added or modified by the setLabels method. Each
* label key/value pair must comply with RFC1035. Label values may be empty.
* @param labels labels or {@code null} for none
*/
public ForwardingRule setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Specifies the forwarding rule type. For more information about forwarding rules, refer to
* Forwarding rule concepts.
* @return value or {@code null} for none
*/
public java.lang.String getLoadBalancingScheme() {
return loadBalancingScheme;
}
/**
* Specifies the forwarding rule type. For more information about forwarding rules, refer to
* Forwarding rule concepts.
* @param loadBalancingScheme loadBalancingScheme or {@code null} for none
*/
public ForwardingRule setLoadBalancingScheme(java.lang.String loadBalancingScheme) {
this.loadBalancingScheme = loadBalancingScheme;
return this;
}
/**
* Opaque filter criteria used by load balancer to restrict routing configuration to a limited set
* of xDS compliant clients. In their xDS requests to load balancer, xDS clients present node
* metadata. When there is a match, the relevant configuration is made available to those proxies.
* Otherwise, all the resources (e.g. TargetHttpProxy, UrlMap) referenced by the ForwardingRule
* are not visible to those proxies. For each metadataFilter in this list, if its
* filterMatchCriteria is set to MATCH_ANY, at least one of the filterLabels must match the
* corresponding label provided in the metadata. If its filterMatchCriteria is set to MATCH_ALL,
* then all of its filterLabels must match with corresponding labels provided in the metadata. If
* multiple metadataFilters are specified, all of them need to be satisfied in order to be
* considered a match. metadataFilters specified here will be applifed before those specified in
* the UrlMap that this ForwardingRule references. metadataFilters only applies to Loadbalancers
* that have their loadBalancingScheme set to INTERNAL_SELF_MANAGED.
* @return value or {@code null} for none
*/
public java.util.List getMetadataFilters() {
return metadataFilters;
}
/**
* Opaque filter criteria used by load balancer to restrict routing configuration to a limited set
* of xDS compliant clients. In their xDS requests to load balancer, xDS clients present node
* metadata. When there is a match, the relevant configuration is made available to those proxies.
* Otherwise, all the resources (e.g. TargetHttpProxy, UrlMap) referenced by the ForwardingRule
* are not visible to those proxies. For each metadataFilter in this list, if its
* filterMatchCriteria is set to MATCH_ANY, at least one of the filterLabels must match the
* corresponding label provided in the metadata. If its filterMatchCriteria is set to MATCH_ALL,
* then all of its filterLabels must match with corresponding labels provided in the metadata. If
* multiple metadataFilters are specified, all of them need to be satisfied in order to be
* considered a match. metadataFilters specified here will be applifed before those specified in
* the UrlMap that this ForwardingRule references. metadataFilters only applies to Loadbalancers
* that have their loadBalancingScheme set to INTERNAL_SELF_MANAGED.
* @param metadataFilters metadataFilters or {@code null} for none
*/
public ForwardingRule setMetadataFilters(java.util.List metadataFilters) {
this.metadataFilters = metadataFilters;
return this;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash. For Private Service
* Connect forwarding rules that forward traffic to Google APIs, the forwarding rule name must be
* a 1-20 characters string with lowercase letters and numbers and must start with a letter.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
* long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
* character must be a lowercase letter, and all following characters must be a dash, lowercase
* letter, or digit, except the last character, which cannot be a dash. For Private Service
* Connect forwarding rules that forward traffic to Google APIs, the forwarding rule name must be
* a 1-20 characters string with lowercase letters and numbers and must start with a letter.
* @param name name or {@code null} for none
*/
public ForwardingRule setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* This field is not used for external load balancing. For Internal TCP/UDP Load Balancing, this
* field identifies the network that the load balanced IP should belong to for this Forwarding
* Rule. If this field is not specified, the default network will be used. For Private Service
* Connect forwarding rules that forward traffic to Google APIs, a network must be provided.
* @return value or {@code null} for none
*/
public java.lang.String getNetwork() {
return network;
}
/**
* This field is not used for external load balancing. For Internal TCP/UDP Load Balancing, this
* field identifies the network that the load balanced IP should belong to for this Forwarding
* Rule. If this field is not specified, the default network will be used. For Private Service
* Connect forwarding rules that forward traffic to Google APIs, a network must be provided.
* @param network network or {@code null} for none
*/
public ForwardingRule setNetwork(java.lang.String network) {
this.network = network;
return this;
}
/**
* This signifies the networking tier used for configuring this load balancer and can only take
* the following values: PREMIUM, STANDARD. For regional ForwardingRule, the valid values are
* PREMIUM and STANDARD. For GlobalForwardingRule, the valid value is PREMIUM. If this field is
* not specified, it is assumed to be PREMIUM. If IPAddress is specified, this value must be equal
* to the networkTier of the Address.
* @return value or {@code null} for none
*/
public java.lang.String getNetworkTier() {
return networkTier;
}
/**
* This signifies the networking tier used for configuring this load balancer and can only take
* the following values: PREMIUM, STANDARD. For regional ForwardingRule, the valid values are
* PREMIUM and STANDARD. For GlobalForwardingRule, the valid value is PREMIUM. If this field is
* not specified, it is assumed to be PREMIUM. If IPAddress is specified, this value must be equal
* to the networkTier of the Address.
* @param networkTier networkTier or {@code null} for none
*/
public ForwardingRule setNetworkTier(java.lang.String networkTier) {
this.networkTier = networkTier;
return this;
}
/**
* This is used in PSC consumer ForwardingRule to control whether it should try to auto-generate a
* DNS zone or not. Non-PSC forwarding rules do not use this field.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNoAutomateDnsZone() {
return noAutomateDnsZone;
}
/**
* This is used in PSC consumer ForwardingRule to control whether it should try to auto-generate a
* DNS zone or not. Non-PSC forwarding rules do not use this field.
* @param noAutomateDnsZone noAutomateDnsZone or {@code null} for none
*/
public ForwardingRule setNoAutomateDnsZone(java.lang.Boolean noAutomateDnsZone) {
this.noAutomateDnsZone = noAutomateDnsZone;
return this;
}
/**
* This field can be used only if: - Load balancing scheme is one of EXTERNAL,
* INTERNAL_SELF_MANAGED or INTERNAL_MANAGED - IPProtocol is one of TCP, UDP, or SCTP. Packets
* addressed to ports in the specified range will be forwarded to target or backend_service. You
* can only use one of ports, port_range, or allPorts. The three are mutually exclusive.
* Forwarding rules with the same [IPAddress, IPProtocol] pair must have disjoint ports. Some
* types of forwarding target have constraints on the acceptable ports. For more information, see
* [Port specifications](https://cloud.google.com/load-balancing/docs/forwarding-rule-
* concepts#port_specifications). @pattern: \\d+(?:-\\d+)?
* @return value or {@code null} for none
*/
public java.lang.String getPortRange() {
return portRange;
}
/**
* This field can be used only if: - Load balancing scheme is one of EXTERNAL,
* INTERNAL_SELF_MANAGED or INTERNAL_MANAGED - IPProtocol is one of TCP, UDP, or SCTP. Packets
* addressed to ports in the specified range will be forwarded to target or backend_service. You
* can only use one of ports, port_range, or allPorts. The three are mutually exclusive.
* Forwarding rules with the same [IPAddress, IPProtocol] pair must have disjoint ports. Some
* types of forwarding target have constraints on the acceptable ports. For more information, see
* [Port specifications](https://cloud.google.com/load-balancing/docs/forwarding-rule-
* concepts#port_specifications). @pattern: \\d+(?:-\\d+)?
* @param portRange portRange or {@code null} for none
*/
public ForwardingRule setPortRange(java.lang.String portRange) {
this.portRange = portRange;
return this;
}
/**
* The ports field is only supported when the forwarding rule references a backend_service
* directly. Only packets addressed to the [specified list of ports]((https://cloud.google.com
* /load-balancing/docs/forwarding-rule-concepts#port_specifications)) are forwarded to backends.
* You can only use one of ports and port_range, or allPorts. The three are mutually exclusive.
* You can specify a list of up to five ports, which can be non-contiguous. Forwarding rules with
* the same [IPAddress, IPProtocol] pair must have disjoint ports. @pattern: \\d+(?:-\\d+)?
* @return value or {@code null} for none
*/
public java.util.List getPorts() {
return ports;
}
/**
* The ports field is only supported when the forwarding rule references a backend_service
* directly. Only packets addressed to the [specified list of ports]((https://cloud.google.com
* /load-balancing/docs/forwarding-rule-concepts#port_specifications)) are forwarded to backends.
* You can only use one of ports and port_range, or allPorts. The three are mutually exclusive.
* You can specify a list of up to five ports, which can be non-contiguous. Forwarding rules with
* the same [IPAddress, IPProtocol] pair must have disjoint ports. @pattern: \\d+(?:-\\d+)?
* @param ports ports or {@code null} for none
*/
public ForwardingRule setPorts(java.util.List ports) {
this.ports = ports;
return this;
}
/**
* [Output Only] The PSC connection id of the PSC Forwarding Rule.
* @return value or {@code null} for none
*/
public java.math.BigInteger getPscConnectionId() {
return pscConnectionId;
}
/**
* [Output Only] The PSC connection id of the PSC Forwarding Rule.
* @param pscConnectionId pscConnectionId or {@code null} for none
*/
public ForwardingRule setPscConnectionId(java.math.BigInteger pscConnectionId) {
this.pscConnectionId = pscConnectionId;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getPscConnectionStatus() {
return pscConnectionStatus;
}
/**
* @param pscConnectionStatus pscConnectionStatus or {@code null} for none
*/
public ForwardingRule setPscConnectionStatus(java.lang.String pscConnectionStatus) {
this.pscConnectionStatus = pscConnectionStatus;
return this;
}
/**
* [Output Only] URL of the region where the regional forwarding rule resides. This field is not
* applicable to global forwarding rules. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* @return value or {@code null} for none
*/
public java.lang.String getRegion() {
return region;
}
/**
* [Output Only] URL of the region where the regional forwarding rule resides. This field is not
* applicable to global forwarding rules. You must specify this field as part of the HTTP request
* URL. It is not settable as a field in the request body.
* @param region region or {@code null} for none
*/
public ForwardingRule setRegion(java.lang.String region) {
this.region = region;
return this;
}
/**
* [Output Only] Server-defined URL for the resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* [Output Only] Server-defined URL for the resource.
* @param selfLink selfLink or {@code null} for none
*/
public ForwardingRule setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* Service Directory resources to register this forwarding rule with. Currently, only supports a
* single Service Directory resource.
* @return value or {@code null} for none
*/
public java.util.List getServiceDirectoryRegistrations() {
return serviceDirectoryRegistrations;
}
/**
* Service Directory resources to register this forwarding rule with. Currently, only supports a
* single Service Directory resource.
* @param serviceDirectoryRegistrations serviceDirectoryRegistrations or {@code null} for none
*/
public ForwardingRule setServiceDirectoryRegistrations(java.util.List serviceDirectoryRegistrations) {
this.serviceDirectoryRegistrations = serviceDirectoryRegistrations;
return this;
}
/**
* An optional prefix to the service name for this Forwarding Rule. If specified, the prefix is
* the first label of the fully qualified service name. The label must be 1-63 characters long,
* and comply with RFC1035. Specifically, the label must be 1-63 characters long and match the
* regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a
* lowercase letter, and all following characters must be a dash, lowercase letter, or digit,
* except the last character, which cannot be a dash. This field is only used for internal load
* balancing.
* @return value or {@code null} for none
*/
public java.lang.String getServiceLabel() {
return serviceLabel;
}
/**
* An optional prefix to the service name for this Forwarding Rule. If specified, the prefix is
* the first label of the fully qualified service name. The label must be 1-63 characters long,
* and comply with RFC1035. Specifically, the label must be 1-63 characters long and match the
* regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a
* lowercase letter, and all following characters must be a dash, lowercase letter, or digit,
* except the last character, which cannot be a dash. This field is only used for internal load
* balancing.
* @param serviceLabel serviceLabel or {@code null} for none
*/
public ForwardingRule setServiceLabel(java.lang.String serviceLabel) {
this.serviceLabel = serviceLabel;
return this;
}
/**
* [Output Only] The internal fully qualified service name for this Forwarding Rule. This field is
* only used for internal load balancing.
* @return value or {@code null} for none
*/
public java.lang.String getServiceName() {
return serviceName;
}
/**
* [Output Only] The internal fully qualified service name for this Forwarding Rule. This field is
* only used for internal load balancing.
* @param serviceName serviceName or {@code null} for none
*/
public ForwardingRule setServiceName(java.lang.String serviceName) {
this.serviceName = serviceName;
return this;
}
/**
* If not empty, this Forwarding Rule will only forward the traffic when the source IP address
* matches one of the IP addresses or CIDR ranges set here. Note that a Forwarding Rule can only
* have up to 64 source IP ranges, and this field can only be used with a regional Forwarding Rule
* whose scheme is EXTERNAL. Each source_ip_range entry should be either an IP address (for
* example, 1.2.3.4) or a CIDR range (for example, 1.2.3.0/24).
* @return value or {@code null} for none
*/
public java.util.List getSourceIpRanges() {
return sourceIpRanges;
}
/**
* If not empty, this Forwarding Rule will only forward the traffic when the source IP address
* matches one of the IP addresses or CIDR ranges set here. Note that a Forwarding Rule can only
* have up to 64 source IP ranges, and this field can only be used with a regional Forwarding Rule
* whose scheme is EXTERNAL. Each source_ip_range entry should be either an IP address (for
* example, 1.2.3.4) or a CIDR range (for example, 1.2.3.0/24).
* @param sourceIpRanges sourceIpRanges or {@code null} for none
*/
public ForwardingRule setSourceIpRanges(java.util.List sourceIpRanges) {
this.sourceIpRanges = sourceIpRanges;
return this;
}
/**
* This field identifies the subnetwork that the load balanced IP should belong to for this
* Forwarding Rule, used in internal load balancing and network load balancing with IPv6. If the
* network specified is in auto subnet mode, this field is optional. However, a subnetwork must be
* specified if the network is in custom subnet mode or when creating external forwarding rule
* with IPv6.
* @return value or {@code null} for none
*/
public java.lang.String getSubnetwork() {
return subnetwork;
}
/**
* This field identifies the subnetwork that the load balanced IP should belong to for this
* Forwarding Rule, used in internal load balancing and network load balancing with IPv6. If the
* network specified is in auto subnet mode, this field is optional. However, a subnetwork must be
* specified if the network is in custom subnet mode or when creating external forwarding rule
* with IPv6.
* @param subnetwork subnetwork or {@code null} for none
*/
public ForwardingRule setSubnetwork(java.lang.String subnetwork) {
this.subnetwork = subnetwork;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getTarget() {
return target;
}
/**
* @param target target or {@code null} for none
*/
public ForwardingRule setTarget(java.lang.String target) {
this.target = target;
return this;
}
@Override
public ForwardingRule set(String fieldName, Object value) {
return (ForwardingRule) super.set(fieldName, value);
}
@Override
public ForwardingRule clone() {
return (ForwardingRule) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy