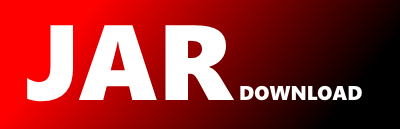
com.google.api.services.compute.model.HttpRetryPolicy Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* The retry policy associates with HttpRouteRule
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class HttpRetryPolicy extends com.google.api.client.json.GenericJson {
/**
* Specifies the allowed number retries. This number must be > 0. If not specified, defaults to 1.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long numRetries;
/**
* Specifies a non-zero timeout per retry attempt. If not specified, will use the timeout set in
* the HttpRouteAction field. If timeout in the HttpRouteAction field is not set, this field uses
* the largest timeout among all backend services associated with the route. Not supported when
* the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to
* true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Duration perTryTimeout;
/**
* Specifies one or more conditions when this retry policy applies. Valid values are: - 5xx: retry
* is attempted if the instance or endpoint responds with any 5xx response code, or if the
* instance or endpoint does not respond at all. For example, disconnects, reset, read timeout,
* connection failure, and refused streams. - gateway-error: Similar to 5xx, but only applies to
* response codes 502, 503 or 504. - connect-failure: a retry is attempted on failures connecting
* to the instance or endpoint. For example, connection timeouts. - retriable-4xx: a retry is
* attempted if the instance or endpoint responds with a 4xx response code. The only error that
* you can retry is error code 409. - refused-stream: a retry is attempted if the instance or
* endpoint resets the stream with a REFUSED_STREAM error code. This reset type indicates that it
* is safe to retry. - cancelled: a retry is attempted if the gRPC status code in the response
* header is set to cancelled. - deadline-exceeded: a retry is attempted if the gRPC status code
* in the response header is set to deadline-exceeded. - internal: a retry is attempted if the
* gRPC status code in the response header is set to internal. - resource-exhausted: a retry is
* attempted if the gRPC status code in the response header is set to resource-exhausted. -
* unavailable: a retry is attempted if the gRPC status code in the response header is set to
* unavailable. Only the following codes are supported when the URL map is bound to target gRPC
* proxy that has validateForProxyless field set to true. - cancelled - deadline-exceeded -
* internal - resource-exhausted - unavailable
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List retryConditions;
/**
* Specifies the allowed number retries. This number must be > 0. If not specified, defaults to 1.
* @return value or {@code null} for none
*/
public java.lang.Long getNumRetries() {
return numRetries;
}
/**
* Specifies the allowed number retries. This number must be > 0. If not specified, defaults to 1.
* @param numRetries numRetries or {@code null} for none
*/
public HttpRetryPolicy setNumRetries(java.lang.Long numRetries) {
this.numRetries = numRetries;
return this;
}
/**
* Specifies a non-zero timeout per retry attempt. If not specified, will use the timeout set in
* the HttpRouteAction field. If timeout in the HttpRouteAction field is not set, this field uses
* the largest timeout among all backend services associated with the route. Not supported when
* the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to
* true.
* @return value or {@code null} for none
*/
public Duration getPerTryTimeout() {
return perTryTimeout;
}
/**
* Specifies a non-zero timeout per retry attempt. If not specified, will use the timeout set in
* the HttpRouteAction field. If timeout in the HttpRouteAction field is not set, this field uses
* the largest timeout among all backend services associated with the route. Not supported when
* the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to
* true.
* @param perTryTimeout perTryTimeout or {@code null} for none
*/
public HttpRetryPolicy setPerTryTimeout(Duration perTryTimeout) {
this.perTryTimeout = perTryTimeout;
return this;
}
/**
* Specifies one or more conditions when this retry policy applies. Valid values are: - 5xx: retry
* is attempted if the instance or endpoint responds with any 5xx response code, or if the
* instance or endpoint does not respond at all. For example, disconnects, reset, read timeout,
* connection failure, and refused streams. - gateway-error: Similar to 5xx, but only applies to
* response codes 502, 503 or 504. - connect-failure: a retry is attempted on failures connecting
* to the instance or endpoint. For example, connection timeouts. - retriable-4xx: a retry is
* attempted if the instance or endpoint responds with a 4xx response code. The only error that
* you can retry is error code 409. - refused-stream: a retry is attempted if the instance or
* endpoint resets the stream with a REFUSED_STREAM error code. This reset type indicates that it
* is safe to retry. - cancelled: a retry is attempted if the gRPC status code in the response
* header is set to cancelled. - deadline-exceeded: a retry is attempted if the gRPC status code
* in the response header is set to deadline-exceeded. - internal: a retry is attempted if the
* gRPC status code in the response header is set to internal. - resource-exhausted: a retry is
* attempted if the gRPC status code in the response header is set to resource-exhausted. -
* unavailable: a retry is attempted if the gRPC status code in the response header is set to
* unavailable. Only the following codes are supported when the URL map is bound to target gRPC
* proxy that has validateForProxyless field set to true. - cancelled - deadline-exceeded -
* internal - resource-exhausted - unavailable
* @return value or {@code null} for none
*/
public java.util.List getRetryConditions() {
return retryConditions;
}
/**
* Specifies one or more conditions when this retry policy applies. Valid values are: - 5xx: retry
* is attempted if the instance or endpoint responds with any 5xx response code, or if the
* instance or endpoint does not respond at all. For example, disconnects, reset, read timeout,
* connection failure, and refused streams. - gateway-error: Similar to 5xx, but only applies to
* response codes 502, 503 or 504. - connect-failure: a retry is attempted on failures connecting
* to the instance or endpoint. For example, connection timeouts. - retriable-4xx: a retry is
* attempted if the instance or endpoint responds with a 4xx response code. The only error that
* you can retry is error code 409. - refused-stream: a retry is attempted if the instance or
* endpoint resets the stream with a REFUSED_STREAM error code. This reset type indicates that it
* is safe to retry. - cancelled: a retry is attempted if the gRPC status code in the response
* header is set to cancelled. - deadline-exceeded: a retry is attempted if the gRPC status code
* in the response header is set to deadline-exceeded. - internal: a retry is attempted if the
* gRPC status code in the response header is set to internal. - resource-exhausted: a retry is
* attempted if the gRPC status code in the response header is set to resource-exhausted. -
* unavailable: a retry is attempted if the gRPC status code in the response header is set to
* unavailable. Only the following codes are supported when the URL map is bound to target gRPC
* proxy that has validateForProxyless field set to true. - cancelled - deadline-exceeded -
* internal - resource-exhausted - unavailable
* @param retryConditions retryConditions or {@code null} for none
*/
public HttpRetryPolicy setRetryConditions(java.util.List retryConditions) {
this.retryConditions = retryConditions;
return this;
}
@Override
public HttpRetryPolicy set(String fieldName, Object value) {
return (HttpRetryPolicy) super.set(fieldName, value);
}
@Override
public HttpRetryPolicy clone() {
return (HttpRetryPolicy) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy