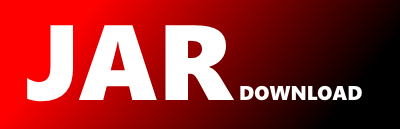
target.apidocs.com.google.api.services.compute.Compute.html Maven / Gradle / Ivy
Compute (Compute Engine API beta-rev20220726-2.0.0)
com.google.api.services.compute
Class Compute
- java.lang.Object
-
- com.google.api.client.googleapis.services.AbstractGoogleClient
-
- com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient
-
- com.google.api.services.compute.Compute
-
public class Compute
extends AbstractGoogleJsonClient
Service definition for Compute (beta).
Creates and runs virtual machines on Google Cloud Platform.
For more information about this service, see the
API Documentation
This service uses ComputeRequestInitializer
to initialize global parameters via its
Compute.Builder
.
- Since:
- 1.3
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
class
Compute.AcceleratorTypes
The "acceleratorTypes" collection of methods.
class
Compute.Addresses
The "addresses" collection of methods.
class
Compute.Autoscalers
The "autoscalers" collection of methods.
class
Compute.BackendBuckets
The "backendBuckets" collection of methods.
class
Compute.BackendServices
The "backendServices" collection of methods.
static class
Compute.Builder
Builder for Compute
.
class
Compute.Disks
The "disks" collection of methods.
class
Compute.DiskTypes
The "diskTypes" collection of methods.
class
Compute.ExternalVpnGateways
The "externalVpnGateways" collection of methods.
class
Compute.FirewallPolicies
The "firewallPolicies" collection of methods.
class
Compute.Firewalls
The "firewalls" collection of methods.
class
Compute.ForwardingRules
The "forwardingRules" collection of methods.
class
Compute.GlobalAddresses
The "globalAddresses" collection of methods.
class
Compute.GlobalForwardingRules
The "globalForwardingRules" collection of methods.
class
Compute.GlobalNetworkEndpointGroups
The "globalNetworkEndpointGroups" collection of methods.
class
Compute.GlobalOperations
The "globalOperations" collection of methods.
class
Compute.GlobalOrganizationOperations
The "globalOrganizationOperations" collection of methods.
class
Compute.GlobalPublicDelegatedPrefixes
The "globalPublicDelegatedPrefixes" collection of methods.
class
Compute.HealthChecks
The "healthChecks" collection of methods.
class
Compute.HttpHealthChecks
The "httpHealthChecks" collection of methods.
class
Compute.HttpsHealthChecks
The "httpsHealthChecks" collection of methods.
class
Compute.ImageFamilyViews
The "imageFamilyViews" collection of methods.
class
Compute.Images
The "images" collection of methods.
class
Compute.InstanceGroupManagers
The "instanceGroupManagers" collection of methods.
class
Compute.InstanceGroups
The "instanceGroups" collection of methods.
class
Compute.Instances
The "instances" collection of methods.
class
Compute.InstanceTemplates
The "instanceTemplates" collection of methods.
class
Compute.InterconnectAttachments
The "interconnectAttachments" collection of methods.
class
Compute.InterconnectLocations
The "interconnectLocations" collection of methods.
class
Compute.Interconnects
The "interconnects" collection of methods.
class
Compute.LicenseCodes
The "licenseCodes" collection of methods.
class
Compute.Licenses
The "licenses" collection of methods.
class
Compute.MachineImages
The "machineImages" collection of methods.
class
Compute.MachineTypes
The "machineTypes" collection of methods.
class
Compute.NetworkEdgeSecurityServices
The "networkEdgeSecurityServices" collection of methods.
class
Compute.NetworkEndpointGroups
The "networkEndpointGroups" collection of methods.
class
Compute.NetworkFirewallPolicies
The "networkFirewallPolicies" collection of methods.
class
Compute.Networks
The "networks" collection of methods.
class
Compute.NodeGroups
The "nodeGroups" collection of methods.
class
Compute.NodeTemplates
The "nodeTemplates" collection of methods.
class
Compute.NodeTypes
The "nodeTypes" collection of methods.
class
Compute.OrganizationSecurityPolicies
The "organizationSecurityPolicies" collection of methods.
class
Compute.PacketMirrorings
The "packetMirrorings" collection of methods.
class
Compute.Projects
The "projects" collection of methods.
class
Compute.PublicAdvertisedPrefixes
The "publicAdvertisedPrefixes" collection of methods.
class
Compute.PublicDelegatedPrefixes
The "publicDelegatedPrefixes" collection of methods.
class
Compute.RegionAutoscalers
The "regionAutoscalers" collection of methods.
class
Compute.RegionBackendServices
The "regionBackendServices" collection of methods.
class
Compute.RegionCommitments
The "regionCommitments" collection of methods.
class
Compute.RegionDisks
The "regionDisks" collection of methods.
class
Compute.RegionDiskTypes
The "regionDiskTypes" collection of methods.
class
Compute.RegionHealthChecks
The "regionHealthChecks" collection of methods.
class
Compute.RegionHealthCheckServices
The "regionHealthCheckServices" collection of methods.
class
Compute.RegionInstanceGroupManagers
The "regionInstanceGroupManagers" collection of methods.
class
Compute.RegionInstanceGroups
The "regionInstanceGroups" collection of methods.
class
Compute.RegionInstances
The "regionInstances" collection of methods.
class
Compute.RegionNetworkEndpointGroups
The "regionNetworkEndpointGroups" collection of methods.
class
Compute.RegionNetworkFirewallPolicies
The "regionNetworkFirewallPolicies" collection of methods.
class
Compute.RegionNotificationEndpoints
The "regionNotificationEndpoints" collection of methods.
class
Compute.RegionOperations
The "regionOperations" collection of methods.
class
Compute.Regions
The "regions" collection of methods.
class
Compute.RegionSecurityPolicies
The "regionSecurityPolicies" collection of methods.
class
Compute.RegionSslCertificates
The "regionSslCertificates" collection of methods.
class
Compute.RegionSslPolicies
The "regionSslPolicies" collection of methods.
class
Compute.RegionTargetHttpProxies
The "regionTargetHttpProxies" collection of methods.
class
Compute.RegionTargetHttpsProxies
The "regionTargetHttpsProxies" collection of methods.
class
Compute.RegionTargetTcpProxies
The "regionTargetTcpProxies" collection of methods.
class
Compute.RegionUrlMaps
The "regionUrlMaps" collection of methods.
class
Compute.Reservations
The "reservations" collection of methods.
class
Compute.ResourcePolicies
The "resourcePolicies" collection of methods.
class
Compute.Routers
The "routers" collection of methods.
class
Compute.Routes
The "routes" collection of methods.
class
Compute.SecurityPolicies
The "securityPolicies" collection of methods.
class
Compute.ServiceAttachments
The "serviceAttachments" collection of methods.
class
Compute.Snapshots
The "snapshots" collection of methods.
class
Compute.SslCertificates
The "sslCertificates" collection of methods.
class
Compute.SslPolicies
The "sslPolicies" collection of methods.
class
Compute.Subnetworks
The "subnetworks" collection of methods.
class
Compute.TargetGrpcProxies
The "targetGrpcProxies" collection of methods.
class
Compute.TargetHttpProxies
The "targetHttpProxies" collection of methods.
class
Compute.TargetHttpsProxies
The "targetHttpsProxies" collection of methods.
class
Compute.TargetInstances
The "targetInstances" collection of methods.
class
Compute.TargetPools
The "targetPools" collection of methods.
class
Compute.TargetSslProxies
The "targetSslProxies" collection of methods.
class
Compute.TargetTcpProxies
The "targetTcpProxies" collection of methods.
class
Compute.TargetVpnGateways
The "targetVpnGateways" collection of methods.
class
Compute.UrlMaps
The "urlMaps" collection of methods.
class
Compute.VpnGateways
The "vpnGateways" collection of methods.
class
Compute.VpnTunnels
The "vpnTunnels" collection of methods.
class
Compute.ZoneOperations
The "zoneOperations" collection of methods.
class
Compute.Zones
The "zones" collection of methods.
-
Field Summary
Fields
Modifier and Type
Field and Description
static String
DEFAULT_BASE_URL
The default encoded base URL of the service.
static String
DEFAULT_BATCH_PATH
The default encoded batch path of the service.
static String
DEFAULT_MTLS_ROOT_URL
The default encoded mTLS root URL of the service.
static String
DEFAULT_ROOT_URL
The default encoded root URL of the service.
static String
DEFAULT_SERVICE_PATH
The default encoded service path of the service.
-
Constructor Summary
Constructors
Constructor and Description
Compute(HttpTransport transport,
JsonFactory jsonFactory,
HttpRequestInitializer httpRequestInitializer)
Constructor.
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Compute.AcceleratorTypes
acceleratorTypes()
An accessor for creating requests from the AcceleratorTypes collection.
Compute.Addresses
addresses()
An accessor for creating requests from the Addresses collection.
Compute.Autoscalers
autoscalers()
An accessor for creating requests from the Autoscalers collection.
Compute.BackendBuckets
backendBuckets()
An accessor for creating requests from the BackendBuckets collection.
Compute.BackendServices
backendServices()
An accessor for creating requests from the BackendServices collection.
Compute.Disks
disks()
An accessor for creating requests from the Disks collection.
Compute.DiskTypes
diskTypes()
An accessor for creating requests from the DiskTypes collection.
Compute.ExternalVpnGateways
externalVpnGateways()
An accessor for creating requests from the ExternalVpnGateways collection.
Compute.FirewallPolicies
firewallPolicies()
An accessor for creating requests from the FirewallPolicies collection.
Compute.Firewalls
firewalls()
An accessor for creating requests from the Firewalls collection.
Compute.ForwardingRules
forwardingRules()
An accessor for creating requests from the ForwardingRules collection.
Compute.GlobalAddresses
globalAddresses()
An accessor for creating requests from the GlobalAddresses collection.
Compute.GlobalForwardingRules
globalForwardingRules()
An accessor for creating requests from the GlobalForwardingRules collection.
Compute.GlobalNetworkEndpointGroups
globalNetworkEndpointGroups()
An accessor for creating requests from the GlobalNetworkEndpointGroups collection.
Compute.GlobalOperations
globalOperations()
An accessor for creating requests from the GlobalOperations collection.
Compute.GlobalOrganizationOperations
globalOrganizationOperations()
An accessor for creating requests from the GlobalOrganizationOperations collection.
Compute.GlobalPublicDelegatedPrefixes
globalPublicDelegatedPrefixes()
An accessor for creating requests from the GlobalPublicDelegatedPrefixes collection.
Compute.HealthChecks
healthChecks()
An accessor for creating requests from the HealthChecks collection.
Compute.HttpHealthChecks
httpHealthChecks()
An accessor for creating requests from the HttpHealthChecks collection.
Compute.HttpsHealthChecks
httpsHealthChecks()
An accessor for creating requests from the HttpsHealthChecks collection.
Compute.ImageFamilyViews
imageFamilyViews()
An accessor for creating requests from the ImageFamilyViews collection.
Compute.Images
images()
An accessor for creating requests from the Images collection.
protected void
initialize(AbstractGoogleClientRequest<?> httpClientRequest)
Compute.InstanceGroupManagers
instanceGroupManagers()
An accessor for creating requests from the InstanceGroupManagers collection.
Compute.InstanceGroups
instanceGroups()
An accessor for creating requests from the InstanceGroups collection.
Compute.Instances
instances()
An accessor for creating requests from the Instances collection.
Compute.InstanceTemplates
instanceTemplates()
An accessor for creating requests from the InstanceTemplates collection.
Compute.InterconnectAttachments
interconnectAttachments()
An accessor for creating requests from the InterconnectAttachments collection.
Compute.InterconnectLocations
interconnectLocations()
An accessor for creating requests from the InterconnectLocations collection.
Compute.Interconnects
interconnects()
An accessor for creating requests from the Interconnects collection.
Compute.LicenseCodes
licenseCodes()
An accessor for creating requests from the LicenseCodes collection.
Compute.Licenses
licenses()
An accessor for creating requests from the Licenses collection.
Compute.MachineImages
machineImages()
An accessor for creating requests from the MachineImages collection.
Compute.MachineTypes
machineTypes()
An accessor for creating requests from the MachineTypes collection.
Compute.NetworkEdgeSecurityServices
networkEdgeSecurityServices()
An accessor for creating requests from the NetworkEdgeSecurityServices collection.
Compute.NetworkEndpointGroups
networkEndpointGroups()
An accessor for creating requests from the NetworkEndpointGroups collection.
Compute.NetworkFirewallPolicies
networkFirewallPolicies()
An accessor for creating requests from the NetworkFirewallPolicies collection.
Compute.Networks
networks()
An accessor for creating requests from the Networks collection.
Compute.NodeGroups
nodeGroups()
An accessor for creating requests from the NodeGroups collection.
Compute.NodeTemplates
nodeTemplates()
An accessor for creating requests from the NodeTemplates collection.
Compute.NodeTypes
nodeTypes()
An accessor for creating requests from the NodeTypes collection.
Compute.OrganizationSecurityPolicies
organizationSecurityPolicies()
An accessor for creating requests from the OrganizationSecurityPolicies collection.
Compute.PacketMirrorings
packetMirrorings()
An accessor for creating requests from the PacketMirrorings collection.
Compute.Projects
projects()
An accessor for creating requests from the Projects collection.
Compute.PublicAdvertisedPrefixes
publicAdvertisedPrefixes()
An accessor for creating requests from the PublicAdvertisedPrefixes collection.
Compute.PublicDelegatedPrefixes
publicDelegatedPrefixes()
An accessor for creating requests from the PublicDelegatedPrefixes collection.
Compute.RegionAutoscalers
regionAutoscalers()
An accessor for creating requests from the RegionAutoscalers collection.
Compute.RegionBackendServices
regionBackendServices()
An accessor for creating requests from the RegionBackendServices collection.
Compute.RegionCommitments
regionCommitments()
An accessor for creating requests from the RegionCommitments collection.
Compute.RegionDisks
regionDisks()
An accessor for creating requests from the RegionDisks collection.
Compute.RegionDiskTypes
regionDiskTypes()
An accessor for creating requests from the RegionDiskTypes collection.
Compute.RegionHealthChecks
regionHealthChecks()
An accessor for creating requests from the RegionHealthChecks collection.
Compute.RegionHealthCheckServices
regionHealthCheckServices()
An accessor for creating requests from the RegionHealthCheckServices collection.
Compute.RegionInstanceGroupManagers
regionInstanceGroupManagers()
An accessor for creating requests from the RegionInstanceGroupManagers collection.
Compute.RegionInstanceGroups
regionInstanceGroups()
An accessor for creating requests from the RegionInstanceGroups collection.
Compute.RegionInstances
regionInstances()
An accessor for creating requests from the RegionInstances collection.
Compute.RegionNetworkEndpointGroups
regionNetworkEndpointGroups()
An accessor for creating requests from the RegionNetworkEndpointGroups collection.
Compute.RegionNetworkFirewallPolicies
regionNetworkFirewallPolicies()
An accessor for creating requests from the RegionNetworkFirewallPolicies collection.
Compute.RegionNotificationEndpoints
regionNotificationEndpoints()
An accessor for creating requests from the RegionNotificationEndpoints collection.
Compute.RegionOperations
regionOperations()
An accessor for creating requests from the RegionOperations collection.
Compute.Regions
regions()
An accessor for creating requests from the Regions collection.
Compute.RegionSecurityPolicies
regionSecurityPolicies()
An accessor for creating requests from the RegionSecurityPolicies collection.
Compute.RegionSslCertificates
regionSslCertificates()
An accessor for creating requests from the RegionSslCertificates collection.
Compute.RegionSslPolicies
regionSslPolicies()
An accessor for creating requests from the RegionSslPolicies collection.
Compute.RegionTargetHttpProxies
regionTargetHttpProxies()
An accessor for creating requests from the RegionTargetHttpProxies collection.
Compute.RegionTargetHttpsProxies
regionTargetHttpsProxies()
An accessor for creating requests from the RegionTargetHttpsProxies collection.
Compute.RegionTargetTcpProxies
regionTargetTcpProxies()
An accessor for creating requests from the RegionTargetTcpProxies collection.
Compute.RegionUrlMaps
regionUrlMaps()
An accessor for creating requests from the RegionUrlMaps collection.
Compute.Reservations
reservations()
An accessor for creating requests from the Reservations collection.
Compute.ResourcePolicies
resourcePolicies()
An accessor for creating requests from the ResourcePolicies collection.
Compute.Routers
routers()
An accessor for creating requests from the Routers collection.
Compute.Routes
routes()
An accessor for creating requests from the Routes collection.
Compute.SecurityPolicies
securityPolicies()
An accessor for creating requests from the SecurityPolicies collection.
Compute.ServiceAttachments
serviceAttachments()
An accessor for creating requests from the ServiceAttachments collection.
Compute.Snapshots
snapshots()
An accessor for creating requests from the Snapshots collection.
Compute.SslCertificates
sslCertificates()
An accessor for creating requests from the SslCertificates collection.
Compute.SslPolicies
sslPolicies()
An accessor for creating requests from the SslPolicies collection.
Compute.Subnetworks
subnetworks()
An accessor for creating requests from the Subnetworks collection.
Compute.TargetGrpcProxies
targetGrpcProxies()
An accessor for creating requests from the TargetGrpcProxies collection.
Compute.TargetHttpProxies
targetHttpProxies()
An accessor for creating requests from the TargetHttpProxies collection.
Compute.TargetHttpsProxies
targetHttpsProxies()
An accessor for creating requests from the TargetHttpsProxies collection.
Compute.TargetInstances
targetInstances()
An accessor for creating requests from the TargetInstances collection.
Compute.TargetPools
targetPools()
An accessor for creating requests from the TargetPools collection.
Compute.TargetSslProxies
targetSslProxies()
An accessor for creating requests from the TargetSslProxies collection.
Compute.TargetTcpProxies
targetTcpProxies()
An accessor for creating requests from the TargetTcpProxies collection.
Compute.TargetVpnGateways
targetVpnGateways()
An accessor for creating requests from the TargetVpnGateways collection.
Compute.UrlMaps
urlMaps()
An accessor for creating requests from the UrlMaps collection.
Compute.VpnGateways
vpnGateways()
An accessor for creating requests from the VpnGateways collection.
Compute.VpnTunnels
vpnTunnels()
An accessor for creating requests from the VpnTunnels collection.
Compute.ZoneOperations
zoneOperations()
An accessor for creating requests from the ZoneOperations collection.
Compute.Zones
zones()
An accessor for creating requests from the Zones collection.
-
Methods inherited from class com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient
getJsonFactory, getObjectParser
-
Methods inherited from class com.google.api.client.googleapis.services.AbstractGoogleClient
batch, batch, getApplicationName, getBaseUrl, getGoogleClientRequestInitializer, getRequestFactory, getRootUrl, getServicePath, getSuppressPatternChecks, getSuppressRequiredParameterChecks
-
-
Field Detail
-
DEFAULT_ROOT_URL
public static final String DEFAULT_ROOT_URL
The default encoded root URL of the service. This is determined when the library is generated
and normally should not be changed.
- Since:
- 1.7
- See Also:
- Constant Field Values
-
DEFAULT_MTLS_ROOT_URL
public static final String DEFAULT_MTLS_ROOT_URL
The default encoded mTLS root URL of the service. This is determined when the library is generated
and normally should not be changed.
- Since:
- 1.31
- See Also:
- Constant Field Values
-
DEFAULT_SERVICE_PATH
public static final String DEFAULT_SERVICE_PATH
The default encoded service path of the service. This is determined when the library is
generated and normally should not be changed.
- Since:
- 1.7
- See Also:
- Constant Field Values
-
DEFAULT_BATCH_PATH
public static final String DEFAULT_BATCH_PATH
The default encoded batch path of the service. This is determined when the library is
generated and normally should not be changed.
- Since:
- 1.23
- See Also:
- Constant Field Values
-
DEFAULT_BASE_URL
public static final String DEFAULT_BASE_URL
The default encoded base URL of the service. This is determined when the library is generated
and normally should not be changed.
- See Also:
- Constant Field Values
-
Constructor Detail
-
Compute
public Compute(HttpTransport transport,
JsonFactory jsonFactory,
HttpRequestInitializer httpRequestInitializer)
Constructor.
Use Compute.Builder
if you need to specify any of the optional parameters.
- Parameters:
transport
- HTTP transport, which should normally be:
- Google App Engine:
com.google.api.client.extensions.appengine.http.UrlFetchTransport
- Android:
newCompatibleTransport
from
com.google.api.client.extensions.android.http.AndroidHttp
- Java:
GoogleNetHttpTransport
jsonFactory
- JSON factory, which may be:
- Jackson:
com.google.api.client.json.jackson2.JacksonFactory
- Google GSON:
com.google.api.client.json.gson.GsonFactory
- Android Honeycomb or higher:
com.google.api.client.extensions.android.json.AndroidJsonFactory
httpRequestInitializer
- HTTP request initializer or null
for none
- Since:
- 1.7
-
Method Detail
-
initialize
protected void initialize(AbstractGoogleClientRequest<?> httpClientRequest)
throws IOException
- Overrides:
initialize
in class AbstractGoogleClient
- Throws:
IOException
-
acceleratorTypes
public Compute.AcceleratorTypes acceleratorTypes()
An accessor for creating requests from the AcceleratorTypes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.AcceleratorTypes.List request = compute.acceleratorTypes().list(parameters ...)
- Returns:
- the resource collection
-
addresses
public Compute.Addresses addresses()
An accessor for creating requests from the Addresses collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Addresses.List request = compute.addresses().list(parameters ...)
- Returns:
- the resource collection
-
autoscalers
public Compute.Autoscalers autoscalers()
An accessor for creating requests from the Autoscalers collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Autoscalers.List request = compute.autoscalers().list(parameters ...)
- Returns:
- the resource collection
-
backendBuckets
public Compute.BackendBuckets backendBuckets()
An accessor for creating requests from the BackendBuckets collection.
The typical use is:
Compute compute = new Compute(...);
Compute.BackendBuckets.List request = compute.backendBuckets().list(parameters ...)
- Returns:
- the resource collection
-
backendServices
public Compute.BackendServices backendServices()
An accessor for creating requests from the BackendServices collection.
The typical use is:
Compute compute = new Compute(...);
Compute.BackendServices.List request = compute.backendServices().list(parameters ...)
- Returns:
- the resource collection
-
diskTypes
public Compute.DiskTypes diskTypes()
An accessor for creating requests from the DiskTypes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.DiskTypes.List request = compute.diskTypes().list(parameters ...)
- Returns:
- the resource collection
-
disks
public Compute.Disks disks()
An accessor for creating requests from the Disks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Disks.List request = compute.disks().list(parameters ...)
- Returns:
- the resource collection
-
externalVpnGateways
public Compute.ExternalVpnGateways externalVpnGateways()
An accessor for creating requests from the ExternalVpnGateways collection.
The typical use is:
Compute compute = new Compute(...);
Compute.ExternalVpnGateways.List request = compute.externalVpnGateways().list(parameters ...)
- Returns:
- the resource collection
-
firewallPolicies
public Compute.FirewallPolicies firewallPolicies()
An accessor for creating requests from the FirewallPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.FirewallPolicies.List request = compute.firewallPolicies().list(parameters ...)
- Returns:
- the resource collection
-
firewalls
public Compute.Firewalls firewalls()
An accessor for creating requests from the Firewalls collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Firewalls.List request = compute.firewalls().list(parameters ...)
- Returns:
- the resource collection
-
forwardingRules
public Compute.ForwardingRules forwardingRules()
An accessor for creating requests from the ForwardingRules collection.
The typical use is:
Compute compute = new Compute(...);
Compute.ForwardingRules.List request = compute.forwardingRules().list(parameters ...)
- Returns:
- the resource collection
-
globalAddresses
public Compute.GlobalAddresses globalAddresses()
An accessor for creating requests from the GlobalAddresses collection.
The typical use is:
Compute compute = new Compute(...);
Compute.GlobalAddresses.List request = compute.globalAddresses().list(parameters ...)
- Returns:
- the resource collection
-
globalForwardingRules
public Compute.GlobalForwardingRules globalForwardingRules()
An accessor for creating requests from the GlobalForwardingRules collection.
The typical use is:
Compute compute = new Compute(...);
Compute.GlobalForwardingRules.List request = compute.globalForwardingRules().list(parameters ...)
- Returns:
- the resource collection
-
globalNetworkEndpointGroups
public Compute.GlobalNetworkEndpointGroups globalNetworkEndpointGroups()
An accessor for creating requests from the GlobalNetworkEndpointGroups collection.
The typical use is:
Compute compute = new Compute(...);
Compute.GlobalNetworkEndpointGroups.List request = compute.globalNetworkEndpointGroups().list(parameters ...)
- Returns:
- the resource collection
-
globalOperations
public Compute.GlobalOperations globalOperations()
An accessor for creating requests from the GlobalOperations collection.
The typical use is:
Compute compute = new Compute(...);
Compute.GlobalOperations.List request = compute.globalOperations().list(parameters ...)
- Returns:
- the resource collection
-
globalOrganizationOperations
public Compute.GlobalOrganizationOperations globalOrganizationOperations()
An accessor for creating requests from the GlobalOrganizationOperations collection.
The typical use is:
Compute compute = new Compute(...);
Compute.GlobalOrganizationOperations.List request = compute.globalOrganizationOperations().list(parameters ...)
- Returns:
- the resource collection
-
globalPublicDelegatedPrefixes
public Compute.GlobalPublicDelegatedPrefixes globalPublicDelegatedPrefixes()
An accessor for creating requests from the GlobalPublicDelegatedPrefixes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.GlobalPublicDelegatedPrefixes.List request = compute.globalPublicDelegatedPrefixes().list(parameters ...)
- Returns:
- the resource collection
-
healthChecks
public Compute.HealthChecks healthChecks()
An accessor for creating requests from the HealthChecks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.HealthChecks.List request = compute.healthChecks().list(parameters ...)
- Returns:
- the resource collection
-
httpHealthChecks
public Compute.HttpHealthChecks httpHealthChecks()
An accessor for creating requests from the HttpHealthChecks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.HttpHealthChecks.List request = compute.httpHealthChecks().list(parameters ...)
- Returns:
- the resource collection
-
httpsHealthChecks
public Compute.HttpsHealthChecks httpsHealthChecks()
An accessor for creating requests from the HttpsHealthChecks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.HttpsHealthChecks.List request = compute.httpsHealthChecks().list(parameters ...)
- Returns:
- the resource collection
-
imageFamilyViews
public Compute.ImageFamilyViews imageFamilyViews()
An accessor for creating requests from the ImageFamilyViews collection.
The typical use is:
Compute compute = new Compute(...);
Compute.ImageFamilyViews.List request = compute.imageFamilyViews().list(parameters ...)
- Returns:
- the resource collection
-
images
public Compute.Images images()
An accessor for creating requests from the Images collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Images.List request = compute.images().list(parameters ...)
- Returns:
- the resource collection
-
instanceGroupManagers
public Compute.InstanceGroupManagers instanceGroupManagers()
An accessor for creating requests from the InstanceGroupManagers collection.
The typical use is:
Compute compute = new Compute(...);
Compute.InstanceGroupManagers.List request = compute.instanceGroupManagers().list(parameters ...)
- Returns:
- the resource collection
-
instanceGroups
public Compute.InstanceGroups instanceGroups()
An accessor for creating requests from the InstanceGroups collection.
The typical use is:
Compute compute = new Compute(...);
Compute.InstanceGroups.List request = compute.instanceGroups().list(parameters ...)
- Returns:
- the resource collection
-
instanceTemplates
public Compute.InstanceTemplates instanceTemplates()
An accessor for creating requests from the InstanceTemplates collection.
The typical use is:
Compute compute = new Compute(...);
Compute.InstanceTemplates.List request = compute.instanceTemplates().list(parameters ...)
- Returns:
- the resource collection
-
instances
public Compute.Instances instances()
An accessor for creating requests from the Instances collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Instances.List request = compute.instances().list(parameters ...)
- Returns:
- the resource collection
-
interconnectAttachments
public Compute.InterconnectAttachments interconnectAttachments()
An accessor for creating requests from the InterconnectAttachments collection.
The typical use is:
Compute compute = new Compute(...);
Compute.InterconnectAttachments.List request = compute.interconnectAttachments().list(parameters ...)
- Returns:
- the resource collection
-
interconnectLocations
public Compute.InterconnectLocations interconnectLocations()
An accessor for creating requests from the InterconnectLocations collection.
The typical use is:
Compute compute = new Compute(...);
Compute.InterconnectLocations.List request = compute.interconnectLocations().list(parameters ...)
- Returns:
- the resource collection
-
interconnects
public Compute.Interconnects interconnects()
An accessor for creating requests from the Interconnects collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Interconnects.List request = compute.interconnects().list(parameters ...)
- Returns:
- the resource collection
-
licenseCodes
public Compute.LicenseCodes licenseCodes()
An accessor for creating requests from the LicenseCodes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.LicenseCodes.List request = compute.licenseCodes().list(parameters ...)
- Returns:
- the resource collection
-
licenses
public Compute.Licenses licenses()
An accessor for creating requests from the Licenses collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Licenses.List request = compute.licenses().list(parameters ...)
- Returns:
- the resource collection
-
machineImages
public Compute.MachineImages machineImages()
An accessor for creating requests from the MachineImages collection.
The typical use is:
Compute compute = new Compute(...);
Compute.MachineImages.List request = compute.machineImages().list(parameters ...)
- Returns:
- the resource collection
-
machineTypes
public Compute.MachineTypes machineTypes()
An accessor for creating requests from the MachineTypes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.MachineTypes.List request = compute.machineTypes().list(parameters ...)
- Returns:
- the resource collection
-
networkEdgeSecurityServices
public Compute.NetworkEdgeSecurityServices networkEdgeSecurityServices()
An accessor for creating requests from the NetworkEdgeSecurityServices collection.
The typical use is:
Compute compute = new Compute(...);
Compute.NetworkEdgeSecurityServices.List request = compute.networkEdgeSecurityServices().list(parameters ...)
- Returns:
- the resource collection
-
networkEndpointGroups
public Compute.NetworkEndpointGroups networkEndpointGroups()
An accessor for creating requests from the NetworkEndpointGroups collection.
The typical use is:
Compute compute = new Compute(...);
Compute.NetworkEndpointGroups.List request = compute.networkEndpointGroups().list(parameters ...)
- Returns:
- the resource collection
-
networkFirewallPolicies
public Compute.NetworkFirewallPolicies networkFirewallPolicies()
An accessor for creating requests from the NetworkFirewallPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.NetworkFirewallPolicies.List request = compute.networkFirewallPolicies().list(parameters ...)
- Returns:
- the resource collection
-
networks
public Compute.Networks networks()
An accessor for creating requests from the Networks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Networks.List request = compute.networks().list(parameters ...)
- Returns:
- the resource collection
-
nodeGroups
public Compute.NodeGroups nodeGroups()
An accessor for creating requests from the NodeGroups collection.
The typical use is:
Compute compute = new Compute(...);
Compute.NodeGroups.List request = compute.nodeGroups().list(parameters ...)
- Returns:
- the resource collection
-
nodeTemplates
public Compute.NodeTemplates nodeTemplates()
An accessor for creating requests from the NodeTemplates collection.
The typical use is:
Compute compute = new Compute(...);
Compute.NodeTemplates.List request = compute.nodeTemplates().list(parameters ...)
- Returns:
- the resource collection
-
nodeTypes
public Compute.NodeTypes nodeTypes()
An accessor for creating requests from the NodeTypes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.NodeTypes.List request = compute.nodeTypes().list(parameters ...)
- Returns:
- the resource collection
-
organizationSecurityPolicies
public Compute.OrganizationSecurityPolicies organizationSecurityPolicies()
An accessor for creating requests from the OrganizationSecurityPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.OrganizationSecurityPolicies.List request = compute.organizationSecurityPolicies().list(parameters ...)
- Returns:
- the resource collection
-
packetMirrorings
public Compute.PacketMirrorings packetMirrorings()
An accessor for creating requests from the PacketMirrorings collection.
The typical use is:
Compute compute = new Compute(...);
Compute.PacketMirrorings.List request = compute.packetMirrorings().list(parameters ...)
- Returns:
- the resource collection
-
projects
public Compute.Projects projects()
An accessor for creating requests from the Projects collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Projects.List request = compute.projects().list(parameters ...)
- Returns:
- the resource collection
-
publicAdvertisedPrefixes
public Compute.PublicAdvertisedPrefixes publicAdvertisedPrefixes()
An accessor for creating requests from the PublicAdvertisedPrefixes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.PublicAdvertisedPrefixes.List request = compute.publicAdvertisedPrefixes().list(parameters ...)
- Returns:
- the resource collection
-
publicDelegatedPrefixes
public Compute.PublicDelegatedPrefixes publicDelegatedPrefixes()
An accessor for creating requests from the PublicDelegatedPrefixes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.PublicDelegatedPrefixes.List request = compute.publicDelegatedPrefixes().list(parameters ...)
- Returns:
- the resource collection
-
regionAutoscalers
public Compute.RegionAutoscalers regionAutoscalers()
An accessor for creating requests from the RegionAutoscalers collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionAutoscalers.List request = compute.regionAutoscalers().list(parameters ...)
- Returns:
- the resource collection
-
regionBackendServices
public Compute.RegionBackendServices regionBackendServices()
An accessor for creating requests from the RegionBackendServices collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionBackendServices.List request = compute.regionBackendServices().list(parameters ...)
- Returns:
- the resource collection
-
regionCommitments
public Compute.RegionCommitments regionCommitments()
An accessor for creating requests from the RegionCommitments collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionCommitments.List request = compute.regionCommitments().list(parameters ...)
- Returns:
- the resource collection
-
regionDiskTypes
public Compute.RegionDiskTypes regionDiskTypes()
An accessor for creating requests from the RegionDiskTypes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionDiskTypes.List request = compute.regionDiskTypes().list(parameters ...)
- Returns:
- the resource collection
-
regionDisks
public Compute.RegionDisks regionDisks()
An accessor for creating requests from the RegionDisks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionDisks.List request = compute.regionDisks().list(parameters ...)
- Returns:
- the resource collection
-
regionHealthCheckServices
public Compute.RegionHealthCheckServices regionHealthCheckServices()
An accessor for creating requests from the RegionHealthCheckServices collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionHealthCheckServices.List request = compute.regionHealthCheckServices().list(parameters ...)
- Returns:
- the resource collection
-
regionHealthChecks
public Compute.RegionHealthChecks regionHealthChecks()
An accessor for creating requests from the RegionHealthChecks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionHealthChecks.List request = compute.regionHealthChecks().list(parameters ...)
- Returns:
- the resource collection
-
regionInstanceGroupManagers
public Compute.RegionInstanceGroupManagers regionInstanceGroupManagers()
An accessor for creating requests from the RegionInstanceGroupManagers collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionInstanceGroupManagers.List request = compute.regionInstanceGroupManagers().list(parameters ...)
- Returns:
- the resource collection
-
regionInstanceGroups
public Compute.RegionInstanceGroups regionInstanceGroups()
An accessor for creating requests from the RegionInstanceGroups collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionInstanceGroups.List request = compute.regionInstanceGroups().list(parameters ...)
- Returns:
- the resource collection
-
regionInstances
public Compute.RegionInstances regionInstances()
An accessor for creating requests from the RegionInstances collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionInstances.List request = compute.regionInstances().list(parameters ...)
- Returns:
- the resource collection
-
regionNetworkEndpointGroups
public Compute.RegionNetworkEndpointGroups regionNetworkEndpointGroups()
An accessor for creating requests from the RegionNetworkEndpointGroups collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionNetworkEndpointGroups.List request = compute.regionNetworkEndpointGroups().list(parameters ...)
- Returns:
- the resource collection
-
regionNetworkFirewallPolicies
public Compute.RegionNetworkFirewallPolicies regionNetworkFirewallPolicies()
An accessor for creating requests from the RegionNetworkFirewallPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionNetworkFirewallPolicies.List request = compute.regionNetworkFirewallPolicies().list(parameters ...)
- Returns:
- the resource collection
-
regionNotificationEndpoints
public Compute.RegionNotificationEndpoints regionNotificationEndpoints()
An accessor for creating requests from the RegionNotificationEndpoints collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionNotificationEndpoints.List request = compute.regionNotificationEndpoints().list(parameters ...)
- Returns:
- the resource collection
-
regionOperations
public Compute.RegionOperations regionOperations()
An accessor for creating requests from the RegionOperations collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionOperations.List request = compute.regionOperations().list(parameters ...)
- Returns:
- the resource collection
-
regionSecurityPolicies
public Compute.RegionSecurityPolicies regionSecurityPolicies()
An accessor for creating requests from the RegionSecurityPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionSecurityPolicies.List request = compute.regionSecurityPolicies().list(parameters ...)
- Returns:
- the resource collection
-
regionSslCertificates
public Compute.RegionSslCertificates regionSslCertificates()
An accessor for creating requests from the RegionSslCertificates collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionSslCertificates.List request = compute.regionSslCertificates().list(parameters ...)
- Returns:
- the resource collection
-
regionSslPolicies
public Compute.RegionSslPolicies regionSslPolicies()
An accessor for creating requests from the RegionSslPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionSslPolicies.List request = compute.regionSslPolicies().list(parameters ...)
- Returns:
- the resource collection
-
regionTargetHttpProxies
public Compute.RegionTargetHttpProxies regionTargetHttpProxies()
An accessor for creating requests from the RegionTargetHttpProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionTargetHttpProxies.List request = compute.regionTargetHttpProxies().list(parameters ...)
- Returns:
- the resource collection
-
regionTargetHttpsProxies
public Compute.RegionTargetHttpsProxies regionTargetHttpsProxies()
An accessor for creating requests from the RegionTargetHttpsProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionTargetHttpsProxies.List request = compute.regionTargetHttpsProxies().list(parameters ...)
- Returns:
- the resource collection
-
regionTargetTcpProxies
public Compute.RegionTargetTcpProxies regionTargetTcpProxies()
An accessor for creating requests from the RegionTargetTcpProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionTargetTcpProxies.List request = compute.regionTargetTcpProxies().list(parameters ...)
- Returns:
- the resource collection
-
regionUrlMaps
public Compute.RegionUrlMaps regionUrlMaps()
An accessor for creating requests from the RegionUrlMaps collection.
The typical use is:
Compute compute = new Compute(...);
Compute.RegionUrlMaps.List request = compute.regionUrlMaps().list(parameters ...)
- Returns:
- the resource collection
-
regions
public Compute.Regions regions()
An accessor for creating requests from the Regions collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Regions.List request = compute.regions().list(parameters ...)
- Returns:
- the resource collection
-
reservations
public Compute.Reservations reservations()
An accessor for creating requests from the Reservations collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Reservations.List request = compute.reservations().list(parameters ...)
- Returns:
- the resource collection
-
resourcePolicies
public Compute.ResourcePolicies resourcePolicies()
An accessor for creating requests from the ResourcePolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.ResourcePolicies.List request = compute.resourcePolicies().list(parameters ...)
- Returns:
- the resource collection
-
routers
public Compute.Routers routers()
An accessor for creating requests from the Routers collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Routers.List request = compute.routers().list(parameters ...)
- Returns:
- the resource collection
-
routes
public Compute.Routes routes()
An accessor for creating requests from the Routes collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Routes.List request = compute.routes().list(parameters ...)
- Returns:
- the resource collection
-
securityPolicies
public Compute.SecurityPolicies securityPolicies()
An accessor for creating requests from the SecurityPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.SecurityPolicies.List request = compute.securityPolicies().list(parameters ...)
- Returns:
- the resource collection
-
serviceAttachments
public Compute.ServiceAttachments serviceAttachments()
An accessor for creating requests from the ServiceAttachments collection.
The typical use is:
Compute compute = new Compute(...);
Compute.ServiceAttachments.List request = compute.serviceAttachments().list(parameters ...)
- Returns:
- the resource collection
-
snapshots
public Compute.Snapshots snapshots()
An accessor for creating requests from the Snapshots collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Snapshots.List request = compute.snapshots().list(parameters ...)
- Returns:
- the resource collection
-
sslCertificates
public Compute.SslCertificates sslCertificates()
An accessor for creating requests from the SslCertificates collection.
The typical use is:
Compute compute = new Compute(...);
Compute.SslCertificates.List request = compute.sslCertificates().list(parameters ...)
- Returns:
- the resource collection
-
sslPolicies
public Compute.SslPolicies sslPolicies()
An accessor for creating requests from the SslPolicies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.SslPolicies.List request = compute.sslPolicies().list(parameters ...)
- Returns:
- the resource collection
-
subnetworks
public Compute.Subnetworks subnetworks()
An accessor for creating requests from the Subnetworks collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Subnetworks.List request = compute.subnetworks().list(parameters ...)
- Returns:
- the resource collection
-
targetGrpcProxies
public Compute.TargetGrpcProxies targetGrpcProxies()
An accessor for creating requests from the TargetGrpcProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetGrpcProxies.List request = compute.targetGrpcProxies().list(parameters ...)
- Returns:
- the resource collection
-
targetHttpProxies
public Compute.TargetHttpProxies targetHttpProxies()
An accessor for creating requests from the TargetHttpProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetHttpProxies.List request = compute.targetHttpProxies().list(parameters ...)
- Returns:
- the resource collection
-
targetHttpsProxies
public Compute.TargetHttpsProxies targetHttpsProxies()
An accessor for creating requests from the TargetHttpsProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetHttpsProxies.List request = compute.targetHttpsProxies().list(parameters ...)
- Returns:
- the resource collection
-
targetInstances
public Compute.TargetInstances targetInstances()
An accessor for creating requests from the TargetInstances collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetInstances.List request = compute.targetInstances().list(parameters ...)
- Returns:
- the resource collection
-
targetPools
public Compute.TargetPools targetPools()
An accessor for creating requests from the TargetPools collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetPools.List request = compute.targetPools().list(parameters ...)
- Returns:
- the resource collection
-
targetSslProxies
public Compute.TargetSslProxies targetSslProxies()
An accessor for creating requests from the TargetSslProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetSslProxies.List request = compute.targetSslProxies().list(parameters ...)
- Returns:
- the resource collection
-
targetTcpProxies
public Compute.TargetTcpProxies targetTcpProxies()
An accessor for creating requests from the TargetTcpProxies collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetTcpProxies.List request = compute.targetTcpProxies().list(parameters ...)
- Returns:
- the resource collection
-
targetVpnGateways
public Compute.TargetVpnGateways targetVpnGateways()
An accessor for creating requests from the TargetVpnGateways collection.
The typical use is:
Compute compute = new Compute(...);
Compute.TargetVpnGateways.List request = compute.targetVpnGateways().list(parameters ...)
- Returns:
- the resource collection
-
urlMaps
public Compute.UrlMaps urlMaps()
An accessor for creating requests from the UrlMaps collection.
The typical use is:
Compute compute = new Compute(...);
Compute.UrlMaps.List request = compute.urlMaps().list(parameters ...)
- Returns:
- the resource collection
-
vpnGateways
public Compute.VpnGateways vpnGateways()
An accessor for creating requests from the VpnGateways collection.
The typical use is:
Compute compute = new Compute(...);
Compute.VpnGateways.List request = compute.vpnGateways().list(parameters ...)
- Returns:
- the resource collection
-
vpnTunnels
public Compute.VpnTunnels vpnTunnels()
An accessor for creating requests from the VpnTunnels collection.
The typical use is:
Compute compute = new Compute(...);
Compute.VpnTunnels.List request = compute.vpnTunnels().list(parameters ...)
- Returns:
- the resource collection
-
zoneOperations
public Compute.ZoneOperations zoneOperations()
An accessor for creating requests from the ZoneOperations collection.
The typical use is:
Compute compute = new Compute(...);
Compute.ZoneOperations.List request = compute.zoneOperations().list(parameters ...)
- Returns:
- the resource collection
-
zones
public Compute.Zones zones()
An accessor for creating requests from the Zones collection.
The typical use is:
Compute compute = new Compute(...);
Compute.Zones.List request = compute.zones().list(parameters ...)
- Returns:
- the resource collection
Copyright © 2011–2022 Google. All rights reserved.