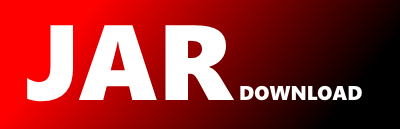
target.apidocs.com.google.api.services.compute.model.Autoscaler.html Maven / Gradle / Ivy
Autoscaler (Compute Engine API beta-rev20220726-2.0.0)
com.google.api.services.compute.model
Class Autoscaler
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.Autoscaler
-
public final class Autoscaler
extends GenericJson
Represents an Autoscaler resource. Google Compute Engine has two Autoscaler resources: *
[Zonal](/compute/docs/reference/rest/beta/autoscalers) *
[Regional](/compute/docs/reference/rest/beta/regionAutoscalers) Use autoscalers to automatically
add or delete instances from a managed instance group according to your defined autoscaling
policy. For more information, read Autoscaling Groups of Instances. For zonal managed instance
groups resource, use the autoscaler resource. For regional managed instance groups, use the
regionAutoscalers resource.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Autoscaler()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Autoscaler
clone()
AutoscalingPolicy
getAutoscalingPolicy()
The configuration parameters for the autoscaling algorithm.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional description of this resource.
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getKind()
[Output Only] Type of the resource.
String
getName()
Name of the resource.
Integer
getRecommendedSize()
[Output Only] Target recommended MIG size (number of instances) computed by autoscaler.
String
getRegion()
[Output Only] URL of the region where the instance group resides (for autoscalers living in
regional scope).
Map<String,ScalingScheduleStatus>
getScalingScheduleStatus()
[Output Only] Status information of existing scaling schedules.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
String
getStatus()
[Output Only] The status of the autoscaler configuration.
List<AutoscalerStatusDetails>
getStatusDetails()
[Output Only] Human-readable details about the current state of the autoscaler.
String
getTarget()
URL of the managed instance group that this autoscaler will scale.
String
getZone()
[Output Only] URL of the zone where the instance group resides (for autoscalers living in zonal
scope).
Autoscaler
set(String fieldName,
Object value)
Autoscaler
setAutoscalingPolicy(AutoscalingPolicy autoscalingPolicy)
The configuration parameters for the autoscaling algorithm.
Autoscaler
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
Autoscaler
setDescription(String description)
An optional description of this resource.
Autoscaler
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
Autoscaler
setKind(String kind)
[Output Only] Type of the resource.
Autoscaler
setName(String name)
Name of the resource.
Autoscaler
setRecommendedSize(Integer recommendedSize)
[Output Only] Target recommended MIG size (number of instances) computed by autoscaler.
Autoscaler
setRegion(String region)
[Output Only] URL of the region where the instance group resides (for autoscalers living in
regional scope).
Autoscaler
setScalingScheduleStatus(Map<String,ScalingScheduleStatus> scalingScheduleStatus)
[Output Only] Status information of existing scaling schedules.
Autoscaler
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
Autoscaler
setStatus(String status)
[Output Only] The status of the autoscaler configuration.
Autoscaler
setStatusDetails(List<AutoscalerStatusDetails> statusDetails)
[Output Only] Human-readable details about the current state of the autoscaler.
Autoscaler
setTarget(String target)
URL of the managed instance group that this autoscaler will scale.
Autoscaler
setZone(String zone)
[Output Only] URL of the zone where the instance group resides (for autoscalers living in zonal
scope).
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAutoscalingPolicy
public AutoscalingPolicy getAutoscalingPolicy()
The configuration parameters for the autoscaling algorithm. You can define one or more signals
for an autoscaler: cpuUtilization, customMetricUtilizations, and loadBalancingUtilization. If
none of these are specified, the default will be to autoscale based on cpuUtilization to 0.6 or
60%.
- Returns:
- value or
null
for none
-
setAutoscalingPolicy
public Autoscaler setAutoscalingPolicy(AutoscalingPolicy autoscalingPolicy)
The configuration parameters for the autoscaling algorithm. You can define one or more signals
for an autoscaler: cpuUtilization, customMetricUtilizations, and loadBalancingUtilization. If
none of these are specified, the default will be to autoscale based on cpuUtilization to 0.6 or
60%.
- Parameters:
autoscalingPolicy
- autoscalingPolicy or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public Autoscaler setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public Autoscaler setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public Autoscaler setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output Only] Type of the resource. Always compute#autoscaler for autoscalers.
- Returns:
- value or
null
for none
-
setKind
public Autoscaler setKind(String kind)
[Output Only] Type of the resource. Always compute#autoscaler for autoscalers.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Returns:
- value or
null
for none
-
setName
public Autoscaler setName(String name)
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Parameters:
name
- name or null
for none
-
getRecommendedSize
public Integer getRecommendedSize()
[Output Only] Target recommended MIG size (number of instances) computed by autoscaler.
Autoscaler calculates the recommended MIG size even when the autoscaling policy mode is
different from ON. This field is empty when autoscaler is not connected to an existing managed
instance group or autoscaler did not generate its prediction.
- Returns:
- value or
null
for none
-
setRecommendedSize
public Autoscaler setRecommendedSize(Integer recommendedSize)
[Output Only] Target recommended MIG size (number of instances) computed by autoscaler.
Autoscaler calculates the recommended MIG size even when the autoscaling policy mode is
different from ON. This field is empty when autoscaler is not connected to an existing managed
instance group or autoscaler did not generate its prediction.
- Parameters:
recommendedSize
- recommendedSize or null
for none
-
getRegion
public String getRegion()
[Output Only] URL of the region where the instance group resides (for autoscalers living in
regional scope).
- Returns:
- value or
null
for none
-
setRegion
public Autoscaler setRegion(String region)
[Output Only] URL of the region where the instance group resides (for autoscalers living in
regional scope).
- Parameters:
region
- region or null
for none
-
getScalingScheduleStatus
public Map<String,ScalingScheduleStatus> getScalingScheduleStatus()
[Output Only] Status information of existing scaling schedules.
- Returns:
- value or
null
for none
-
setScalingScheduleStatus
public Autoscaler setScalingScheduleStatus(Map<String,ScalingScheduleStatus> scalingScheduleStatus)
[Output Only] Status information of existing scaling schedules.
- Parameters:
scalingScheduleStatus
- scalingScheduleStatus or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public Autoscaler setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getStatus
public String getStatus()
[Output Only] The status of the autoscaler configuration. Current set of possible values: -
PENDING: Autoscaler backend hasn't read new/updated configuration. - DELETING: Configuration is
being deleted. - ACTIVE: Configuration is acknowledged to be effective. Some warnings might be
present in the statusDetails field. - ERROR: Configuration has errors. Actionable for users.
Details are present in the statusDetails field. New values might be added in the future.
- Returns:
- value or
null
for none
-
setStatus
public Autoscaler setStatus(String status)
[Output Only] The status of the autoscaler configuration. Current set of possible values: -
PENDING: Autoscaler backend hasn't read new/updated configuration. - DELETING: Configuration is
being deleted. - ACTIVE: Configuration is acknowledged to be effective. Some warnings might be
present in the statusDetails field. - ERROR: Configuration has errors. Actionable for users.
Details are present in the statusDetails field. New values might be added in the future.
- Parameters:
status
- status or null
for none
-
getStatusDetails
public List<AutoscalerStatusDetails> getStatusDetails()
[Output Only] Human-readable details about the current state of the autoscaler. Read the
documentation for Commonly returned status messages for examples of status messages you might
encounter.
- Returns:
- value or
null
for none
-
setStatusDetails
public Autoscaler setStatusDetails(List<AutoscalerStatusDetails> statusDetails)
[Output Only] Human-readable details about the current state of the autoscaler. Read the
documentation for Commonly returned status messages for examples of status messages you might
encounter.
- Parameters:
statusDetails
- statusDetails or null
for none
-
getTarget
public String getTarget()
URL of the managed instance group that this autoscaler will scale. This field is required when
creating an autoscaler.
- Returns:
- value or
null
for none
-
setTarget
public Autoscaler setTarget(String target)
URL of the managed instance group that this autoscaler will scale. This field is required when
creating an autoscaler.
- Parameters:
target
- target or null
for none
-
getZone
public String getZone()
[Output Only] URL of the zone where the instance group resides (for autoscalers living in zonal
scope).
- Returns:
- value or
null
for none
-
setZone
public Autoscaler setZone(String zone)
[Output Only] URL of the zone where the instance group resides (for autoscalers living in zonal
scope).
- Parameters:
zone
- zone or null
for none
-
set
public Autoscaler set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Autoscaler clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy