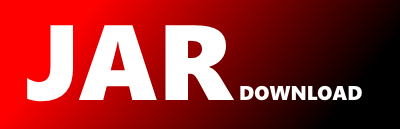
target.apidocs.com.google.api.services.compute.model.CircuitBreakers.html Maven / Gradle / Ivy
CircuitBreakers (Compute Engine API beta-rev20220726-2.0.0)
com.google.api.services.compute.model
Class CircuitBreakers
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.CircuitBreakers
-
public final class CircuitBreakers
extends GenericJson
Settings controlling the volume of requests, connections and retries to this backend service.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
CircuitBreakers()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
CircuitBreakers
clone()
Duration
getConnectTimeout()
The timeout for new network connections to hosts.
Integer
getMaxConnections()
The maximum number of connections to the backend service.
Integer
getMaxPendingRequests()
The maximum number of pending requests allowed to the backend service.
Integer
getMaxRequests()
The maximum number of parallel requests that allowed to the backend service.
Integer
getMaxRequestsPerConnection()
Maximum requests for a single connection to the backend service.
Integer
getMaxRetries()
The maximum number of parallel retries allowed to the backend cluster.
CircuitBreakers
set(String fieldName,
Object value)
CircuitBreakers
setConnectTimeout(Duration connectTimeout)
The timeout for new network connections to hosts.
CircuitBreakers
setMaxConnections(Integer maxConnections)
The maximum number of connections to the backend service.
CircuitBreakers
setMaxPendingRequests(Integer maxPendingRequests)
The maximum number of pending requests allowed to the backend service.
CircuitBreakers
setMaxRequests(Integer maxRequests)
The maximum number of parallel requests that allowed to the backend service.
CircuitBreakers
setMaxRequestsPerConnection(Integer maxRequestsPerConnection)
Maximum requests for a single connection to the backend service.
CircuitBreakers
setMaxRetries(Integer maxRetries)
The maximum number of parallel retries allowed to the backend cluster.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getConnectTimeout
public Duration getConnectTimeout()
The timeout for new network connections to hosts.
- Returns:
- value or
null
for none
-
setConnectTimeout
public CircuitBreakers setConnectTimeout(Duration connectTimeout)
The timeout for new network connections to hosts.
- Parameters:
connectTimeout
- connectTimeout or null
for none
-
getMaxConnections
public Integer getMaxConnections()
The maximum number of connections to the backend service. If not specified, there is no limit.
Not supported when the backend service is referenced by a URL map that is bound to target gRPC
proxy that has validateForProxyless field set to true.
- Returns:
- value or
null
for none
-
setMaxConnections
public CircuitBreakers setMaxConnections(Integer maxConnections)
The maximum number of connections to the backend service. If not specified, there is no limit.
Not supported when the backend service is referenced by a URL map that is bound to target gRPC
proxy that has validateForProxyless field set to true.
- Parameters:
maxConnections
- maxConnections or null
for none
-
getMaxPendingRequests
public Integer getMaxPendingRequests()
The maximum number of pending requests allowed to the backend service. If not specified, there
is no limit. Not supported when the backend service is referenced by a URL map that is bound to
target gRPC proxy that has validateForProxyless field set to true.
- Returns:
- value or
null
for none
-
setMaxPendingRequests
public CircuitBreakers setMaxPendingRequests(Integer maxPendingRequests)
The maximum number of pending requests allowed to the backend service. If not specified, there
is no limit. Not supported when the backend service is referenced by a URL map that is bound to
target gRPC proxy that has validateForProxyless field set to true.
- Parameters:
maxPendingRequests
- maxPendingRequests or null
for none
-
getMaxRequests
public Integer getMaxRequests()
The maximum number of parallel requests that allowed to the backend service. If not specified,
there is no limit.
- Returns:
- value or
null
for none
-
setMaxRequests
public CircuitBreakers setMaxRequests(Integer maxRequests)
The maximum number of parallel requests that allowed to the backend service. If not specified,
there is no limit.
- Parameters:
maxRequests
- maxRequests or null
for none
-
getMaxRequestsPerConnection
public Integer getMaxRequestsPerConnection()
Maximum requests for a single connection to the backend service. This parameter is respected by
both the HTTP/1.1 and HTTP/2 implementations. If not specified, there is no limit. Setting this
parameter to 1 will effectively disable keep alive. Not supported when the backend service is
referenced by a URL map that is bound to target gRPC proxy that has validateForProxyless field
set to true.
- Returns:
- value or
null
for none
-
setMaxRequestsPerConnection
public CircuitBreakers setMaxRequestsPerConnection(Integer maxRequestsPerConnection)
Maximum requests for a single connection to the backend service. This parameter is respected by
both the HTTP/1.1 and HTTP/2 implementations. If not specified, there is no limit. Setting this
parameter to 1 will effectively disable keep alive. Not supported when the backend service is
referenced by a URL map that is bound to target gRPC proxy that has validateForProxyless field
set to true.
- Parameters:
maxRequestsPerConnection
- maxRequestsPerConnection or null
for none
-
getMaxRetries
public Integer getMaxRetries()
The maximum number of parallel retries allowed to the backend cluster. If not specified, the
default is 1. Not supported when the backend service is referenced by a URL map that is bound
to target gRPC proxy that has validateForProxyless field set to true.
- Returns:
- value or
null
for none
-
setMaxRetries
public CircuitBreakers setMaxRetries(Integer maxRetries)
The maximum number of parallel retries allowed to the backend cluster. If not specified, the
default is 1. Not supported when the backend service is referenced by a URL map that is bound
to target gRPC proxy that has validateForProxyless field set to true.
- Parameters:
maxRetries
- maxRetries or null
for none
-
set
public CircuitBreakers set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public CircuitBreakers clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy