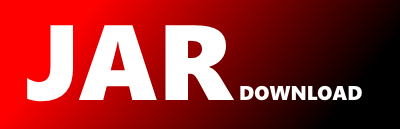
target.apidocs.com.google.api.services.compute.model.HealthCheck.html Maven / Gradle / Ivy
HealthCheck (Compute Engine API beta-rev20220726-2.0.0)
com.google.api.services.compute.model
Class HealthCheck
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.HealthCheck
-
public final class HealthCheck
extends GenericJson
Represents a Health Check resource. Google Compute Engine has two Health Check resources: *
[Global](/compute/docs/reference/rest/beta/healthChecks) *
[Regional](/compute/docs/reference/rest/beta/regionHealthChecks) Internal HTTP(S) load balancers
must use regional health checks (`compute.v1.regionHealthChecks`). Traffic Director must use
global health checks (`compute.v1.HealthChecks`). Internal TCP/UDP load balancers can use either
regional or global health checks (`compute.v1.regionHealthChecks` or `compute.v1.HealthChecks`).
External HTTP(S), TCP proxy, and SSL proxy load balancers as well as managed instance group auto-
healing must use global health checks (`compute.v1.HealthChecks`). Backend service-based network
load balancers must use regional health checks (`compute.v1.regionHealthChecks`). Target pool-
based network load balancers must use legacy HTTP health checks (`compute.v1.httpHealthChecks`).
For more information, see Health checks overview.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
HealthCheck()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
HealthCheck
clone()
Integer
getCheckIntervalSec()
How often (in seconds) to send a health check.
String
getCreationTimestamp()
[Output Only] Creation timestamp in 3339 text format.
String
getDescription()
An optional description of this resource.
GRPCHealthCheck
getGrpcHealthCheck()
Integer
getHealthyThreshold()
A so-far unhealthy instance will be marked healthy after this many consecutive successes.
HTTP2HealthCheck
getHttp2HealthCheck()
HTTPHealthCheck
getHttpHealthCheck()
HTTPSHealthCheck
getHttpsHealthCheck()
BigInteger
getId()
[Output Only] The unique identifier for the resource.
String
getKind()
Type of the resource.
HealthCheckLogConfig
getLogConfig()
Configure logging on this health check.
String
getName()
Name of the resource.
String
getRegion()
[Output Only] Region where the health check resides.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
SSLHealthCheck
getSslHealthCheck()
TCPHealthCheck
getTcpHealthCheck()
Integer
getTimeoutSec()
How long (in seconds) to wait before claiming failure.
String
getType()
Specifies the type of the healthCheck, either TCP, SSL, HTTP, HTTPS, HTTP2 or GRPC.
Integer
getUnhealthyThreshold()
A so-far healthy instance will be marked unhealthy after this many consecutive failures.
HealthCheck
set(String fieldName,
Object value)
HealthCheck
setCheckIntervalSec(Integer checkIntervalSec)
How often (in seconds) to send a health check.
HealthCheck
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in 3339 text format.
HealthCheck
setDescription(String description)
An optional description of this resource.
HealthCheck
setGrpcHealthCheck(GRPCHealthCheck grpcHealthCheck)
HealthCheck
setHealthyThreshold(Integer healthyThreshold)
A so-far unhealthy instance will be marked healthy after this many consecutive successes.
HealthCheck
setHttp2HealthCheck(HTTP2HealthCheck http2HealthCheck)
HealthCheck
setHttpHealthCheck(HTTPHealthCheck httpHealthCheck)
HealthCheck
setHttpsHealthCheck(HTTPSHealthCheck httpsHealthCheck)
HealthCheck
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
HealthCheck
setKind(String kind)
Type of the resource.
HealthCheck
setLogConfig(HealthCheckLogConfig logConfig)
Configure logging on this health check.
HealthCheck
setName(String name)
Name of the resource.
HealthCheck
setRegion(String region)
[Output Only] Region where the health check resides.
HealthCheck
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
HealthCheck
setSslHealthCheck(SSLHealthCheck sslHealthCheck)
HealthCheck
setTcpHealthCheck(TCPHealthCheck tcpHealthCheck)
HealthCheck
setTimeoutSec(Integer timeoutSec)
How long (in seconds) to wait before claiming failure.
HealthCheck
setType(String type)
Specifies the type of the healthCheck, either TCP, SSL, HTTP, HTTPS, HTTP2 or GRPC.
HealthCheck
setUnhealthyThreshold(Integer unhealthyThreshold)
A so-far healthy instance will be marked unhealthy after this many consecutive failures.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCheckIntervalSec
public Integer getCheckIntervalSec()
How often (in seconds) to send a health check. The default value is 5 seconds.
- Returns:
- value or
null
for none
-
setCheckIntervalSec
public HealthCheck setCheckIntervalSec(Integer checkIntervalSec)
How often (in seconds) to send a health check. The default value is 5 seconds.
- Parameters:
checkIntervalSec
- checkIntervalSec or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in 3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public HealthCheck setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in 3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public HealthCheck setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getGrpcHealthCheck
public GRPCHealthCheck getGrpcHealthCheck()
- Returns:
- value or
null
for none
-
setGrpcHealthCheck
public HealthCheck setGrpcHealthCheck(GRPCHealthCheck grpcHealthCheck)
- Parameters:
grpcHealthCheck
- grpcHealthCheck or null
for none
-
getHealthyThreshold
public Integer getHealthyThreshold()
A so-far unhealthy instance will be marked healthy after this many consecutive successes. The
default value is 2.
- Returns:
- value or
null
for none
-
setHealthyThreshold
public HealthCheck setHealthyThreshold(Integer healthyThreshold)
A so-far unhealthy instance will be marked healthy after this many consecutive successes. The
default value is 2.
- Parameters:
healthyThreshold
- healthyThreshold or null
for none
-
getHttp2HealthCheck
public HTTP2HealthCheck getHttp2HealthCheck()
- Returns:
- value or
null
for none
-
setHttp2HealthCheck
public HealthCheck setHttp2HealthCheck(HTTP2HealthCheck http2HealthCheck)
- Parameters:
http2HealthCheck
- http2HealthCheck or null
for none
-
getHttpHealthCheck
public HTTPHealthCheck getHttpHealthCheck()
- Returns:
- value or
null
for none
-
setHttpHealthCheck
public HealthCheck setHttpHealthCheck(HTTPHealthCheck httpHealthCheck)
- Parameters:
httpHealthCheck
- httpHealthCheck or null
for none
-
getHttpsHealthCheck
public HTTPSHealthCheck getHttpsHealthCheck()
- Returns:
- value or
null
for none
-
setHttpsHealthCheck
public HealthCheck setHttpsHealthCheck(HTTPSHealthCheck httpsHealthCheck)
- Parameters:
httpsHealthCheck
- httpsHealthCheck or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public HealthCheck setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
Type of the resource.
- Returns:
- value or
null
for none
-
setKind
public HealthCheck setKind(String kind)
Type of the resource.
- Parameters:
kind
- kind or null
for none
-
getLogConfig
public HealthCheckLogConfig getLogConfig()
Configure logging on this health check.
- Returns:
- value or
null
for none
-
setLogConfig
public HealthCheck setLogConfig(HealthCheckLogConfig logConfig)
Configure logging on this health check.
- Parameters:
logConfig
- logConfig or null
for none
-
getName
public String getName()
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. For example, a name that is 1-63 characters
long, matches the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`, and otherwise complies with
RFC1035. This regular expression describes a name where the first character is a lowercase
letter, and all following characters are a dash, lowercase letter, or digit, except the last
character, which isn't a dash.
- Returns:
- value or
null
for none
-
setName
public HealthCheck setName(String name)
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. For example, a name that is 1-63 characters
long, matches the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`, and otherwise complies with
RFC1035. This regular expression describes a name where the first character is a lowercase
letter, and all following characters are a dash, lowercase letter, or digit, except the last
character, which isn't a dash.
- Parameters:
name
- name or null
for none
-
getRegion
public String getRegion()
[Output Only] Region where the health check resides. Not applicable to global health checks.
- Returns:
- value or
null
for none
-
setRegion
public HealthCheck setRegion(String region)
[Output Only] Region where the health check resides. Not applicable to global health checks.
- Parameters:
region
- region or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public HealthCheck setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getSslHealthCheck
public SSLHealthCheck getSslHealthCheck()
- Returns:
- value or
null
for none
-
setSslHealthCheck
public HealthCheck setSslHealthCheck(SSLHealthCheck sslHealthCheck)
- Parameters:
sslHealthCheck
- sslHealthCheck or null
for none
-
getTcpHealthCheck
public TCPHealthCheck getTcpHealthCheck()
- Returns:
- value or
null
for none
-
setTcpHealthCheck
public HealthCheck setTcpHealthCheck(TCPHealthCheck tcpHealthCheck)
- Parameters:
tcpHealthCheck
- tcpHealthCheck or null
for none
-
getTimeoutSec
public Integer getTimeoutSec()
How long (in seconds) to wait before claiming failure. The default value is 5 seconds. It is
invalid for timeoutSec to have greater value than checkIntervalSec.
- Returns:
- value or
null
for none
-
setTimeoutSec
public HealthCheck setTimeoutSec(Integer timeoutSec)
How long (in seconds) to wait before claiming failure. The default value is 5 seconds. It is
invalid for timeoutSec to have greater value than checkIntervalSec.
- Parameters:
timeoutSec
- timeoutSec or null
for none
-
getType
public String getType()
Specifies the type of the healthCheck, either TCP, SSL, HTTP, HTTPS, HTTP2 or GRPC. Exactly one
of the protocol-specific health check fields must be specified, which must match type field.
- Returns:
- value or
null
for none
-
setType
public HealthCheck setType(String type)
Specifies the type of the healthCheck, either TCP, SSL, HTTP, HTTPS, HTTP2 or GRPC. Exactly one
of the protocol-specific health check fields must be specified, which must match type field.
- Parameters:
type
- type or null
for none
-
getUnhealthyThreshold
public Integer getUnhealthyThreshold()
A so-far healthy instance will be marked unhealthy after this many consecutive failures. The
default value is 2.
- Returns:
- value or
null
for none
-
setUnhealthyThreshold
public HealthCheck setUnhealthyThreshold(Integer unhealthyThreshold)
A so-far healthy instance will be marked unhealthy after this many consecutive failures. The
default value is 2.
- Parameters:
unhealthyThreshold
- unhealthyThreshold or null
for none
-
set
public HealthCheck set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public HealthCheck clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy