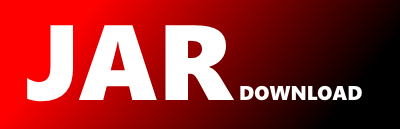
target.apidocs.com.google.api.services.compute.model.TargetPool.html Maven / Gradle / Ivy
TargetPool (Compute Engine API beta-rev20220726-2.0.0)
com.google.api.services.compute.model
Class TargetPool
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.compute.model.TargetPool
-
public final class TargetPool
extends GenericJson
Represents a Target Pool resource. Target pools are used for network TCP/UDP load balancing. A
target pool references member instances, an associated legacy HttpHealthCheck resource, and,
optionally, a backup target pool. For more information, read Using target pools.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
TargetPool()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
TargetPool
clone()
String
getBackupPool()
The server-defined URL for the resource.
String
getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
String
getDescription()
An optional description of this resource.
Float
getFailoverRatio()
This field is applicable only when the containing target pool is serving a forwarding rule as
the primary pool (i.e., not as a backup pool to some other target pool).
List<String>
getHealthChecks()
The URL of the HttpHealthCheck resource.
BigInteger
getId()
[Output Only] The unique identifier for the resource.
List<String>
getInstances()
A list of resource URLs to the virtual machine instances serving this pool.
String
getKind()
[Output Only] Type of the resource.
String
getName()
Name of the resource.
String
getRegion()
[Output Only] URL of the region where the target pool resides.
String
getSelfLink()
[Output Only] Server-defined URL for the resource.
String
getSessionAffinity()
Session affinity option, must be one of the following values: NONE: Connections from the same
client IP may go to any instance in the pool.
TargetPool
set(String fieldName,
Object value)
TargetPool
setBackupPool(String backupPool)
The server-defined URL for the resource.
TargetPool
setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
TargetPool
setDescription(String description)
An optional description of this resource.
TargetPool
setFailoverRatio(Float failoverRatio)
This field is applicable only when the containing target pool is serving a forwarding rule as
the primary pool (i.e., not as a backup pool to some other target pool).
TargetPool
setHealthChecks(List<String> healthChecks)
The URL of the HttpHealthCheck resource.
TargetPool
setId(BigInteger id)
[Output Only] The unique identifier for the resource.
TargetPool
setInstances(List<String> instances)
A list of resource URLs to the virtual machine instances serving this pool.
TargetPool
setKind(String kind)
[Output Only] Type of the resource.
TargetPool
setName(String name)
Name of the resource.
TargetPool
setRegion(String region)
[Output Only] URL of the region where the target pool resides.
TargetPool
setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
TargetPool
setSessionAffinity(String sessionAffinity)
Session affinity option, must be one of the following values: NONE: Connections from the same
client IP may go to any instance in the pool.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getBackupPool
public String getBackupPool()
The server-defined URL for the resource. This field is applicable only when the containing
target pool is serving a forwarding rule as the primary pool, and its failoverRatio field is
properly set to a value between [0, 1]. backupPool and failoverRatio together define the
fallback behavior of the primary target pool: if the ratio of the healthy instances in the
primary pool is at or below failoverRatio, traffic arriving at the load-balanced IP will be
directed to the backup pool. In case where failoverRatio and backupPool are not set, or all the
instances in the backup pool are unhealthy, the traffic will be directed back to the primary
pool in the "force" mode, where traffic will be spread to the healthy instances with the best
effort, or to all instances when no instance is healthy.
- Returns:
- value or
null
for none
-
setBackupPool
public TargetPool setBackupPool(String backupPool)
The server-defined URL for the resource. This field is applicable only when the containing
target pool is serving a forwarding rule as the primary pool, and its failoverRatio field is
properly set to a value between [0, 1]. backupPool and failoverRatio together define the
fallback behavior of the primary target pool: if the ratio of the healthy instances in the
primary pool is at or below failoverRatio, traffic arriving at the load-balanced IP will be
directed to the backup pool. In case where failoverRatio and backupPool are not set, or all the
instances in the backup pool are unhealthy, the traffic will be directed back to the primary
pool in the "force" mode, where traffic will be spread to the healthy instances with the best
effort, or to all instances when no instance is healthy.
- Parameters:
backupPool
- backupPool or null
for none
-
getCreationTimestamp
public String getCreationTimestamp()
[Output Only] Creation timestamp in RFC3339 text format.
- Returns:
- value or
null
for none
-
setCreationTimestamp
public TargetPool setCreationTimestamp(String creationTimestamp)
[Output Only] Creation timestamp in RFC3339 text format.
- Parameters:
creationTimestamp
- creationTimestamp or null
for none
-
getDescription
public String getDescription()
An optional description of this resource. Provide this property when you create the resource.
- Returns:
- value or
null
for none
-
setDescription
public TargetPool setDescription(String description)
An optional description of this resource. Provide this property when you create the resource.
- Parameters:
description
- description or null
for none
-
getFailoverRatio
public Float getFailoverRatio()
This field is applicable only when the containing target pool is serving a forwarding rule as
the primary pool (i.e., not as a backup pool to some other target pool). The value of the field
must be in [0, 1]. If set, backupPool must also be set. They together define the fallback
behavior of the primary target pool: if the ratio of the healthy instances in the primary pool
is at or below this number, traffic arriving at the load-balanced IP will be directed to the
backup pool. In case where failoverRatio is not set or all the instances in the backup pool are
unhealthy, the traffic will be directed back to the primary pool in the "force" mode, where
traffic will be spread to the healthy instances with the best effort, or to all instances when
no instance is healthy.
- Returns:
- value or
null
for none
-
setFailoverRatio
public TargetPool setFailoverRatio(Float failoverRatio)
This field is applicable only when the containing target pool is serving a forwarding rule as
the primary pool (i.e., not as a backup pool to some other target pool). The value of the field
must be in [0, 1]. If set, backupPool must also be set. They together define the fallback
behavior of the primary target pool: if the ratio of the healthy instances in the primary pool
is at or below this number, traffic arriving at the load-balanced IP will be directed to the
backup pool. In case where failoverRatio is not set or all the instances in the backup pool are
unhealthy, the traffic will be directed back to the primary pool in the "force" mode, where
traffic will be spread to the healthy instances with the best effort, or to all instances when
no instance is healthy.
- Parameters:
failoverRatio
- failoverRatio or null
for none
-
getHealthChecks
public List<String> getHealthChecks()
The URL of the HttpHealthCheck resource. A member instance in this pool is considered healthy
if and only if the health checks pass. Only legacy HttpHealthChecks are supported. Only one
health check may be specified.
- Returns:
- value or
null
for none
-
setHealthChecks
public TargetPool setHealthChecks(List<String> healthChecks)
The URL of the HttpHealthCheck resource. A member instance in this pool is considered healthy
if and only if the health checks pass. Only legacy HttpHealthChecks are supported. Only one
health check may be specified.
- Parameters:
healthChecks
- healthChecks or null
for none
-
getId
public BigInteger getId()
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Returns:
- value or
null
for none
-
setId
public TargetPool setId(BigInteger id)
[Output Only] The unique identifier for the resource. This identifier is defined by the server.
- Parameters:
id
- id or null
for none
-
getInstances
public List<String> getInstances()
A list of resource URLs to the virtual machine instances serving this pool. They must live in
zones contained in the same region as this pool.
- Returns:
- value or
null
for none
-
setInstances
public TargetPool setInstances(List<String> instances)
A list of resource URLs to the virtual machine instances serving this pool. They must live in
zones contained in the same region as this pool.
- Parameters:
instances
- instances or null
for none
-
getKind
public String getKind()
[Output Only] Type of the resource. Always compute#targetPool for target pools.
- Returns:
- value or
null
for none
-
setKind
public TargetPool setKind(String kind)
[Output Only] Type of the resource. Always compute#targetPool for target pools.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Returns:
- value or
null
for none
-
setName
public TargetPool setName(String name)
Name of the resource. Provided by the client when the resource is created. The name must be
1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters
long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first
character must be a lowercase letter, and all following characters must be a dash, lowercase
letter, or digit, except the last character, which cannot be a dash.
- Parameters:
name
- name or null
for none
-
getRegion
public String getRegion()
[Output Only] URL of the region where the target pool resides.
- Returns:
- value or
null
for none
-
setRegion
public TargetPool setRegion(String region)
[Output Only] URL of the region where the target pool resides.
- Parameters:
region
- region or null
for none
-
getSelfLink
public String getSelfLink()
[Output Only] Server-defined URL for the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public TargetPool setSelfLink(String selfLink)
[Output Only] Server-defined URL for the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
getSessionAffinity
public String getSessionAffinity()
Session affinity option, must be one of the following values: NONE: Connections from the same
client IP may go to any instance in the pool. CLIENT_IP: Connections from the same client IP
will go to the same instance in the pool while that instance remains healthy. CLIENT_IP_PROTO:
Connections from the same client IP with the same IP protocol will go to the same instance in
the pool while that instance remains healthy.
- Returns:
- value or
null
for none
-
setSessionAffinity
public TargetPool setSessionAffinity(String sessionAffinity)
Session affinity option, must be one of the following values: NONE: Connections from the same
client IP may go to any instance in the pool. CLIENT_IP: Connections from the same client IP
will go to the same instance in the pool while that instance remains healthy. CLIENT_IP_PROTO:
Connections from the same client IP with the same IP protocol will go to the same instance in
the pool while that instance remains healthy.
- Parameters:
sessionAffinity
- sessionAffinity or null
for none
-
set
public TargetPool set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public TargetPool clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy