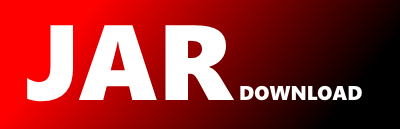
com.google.api.services.compute.model.InstanceGroupManagerActionsSummary Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-08-07 at 18:49:37 UTC
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Model definition for InstanceGroupManagerActionsSummary.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class InstanceGroupManagerActionsSummary extends com.google.api.client.json.GenericJson {
/**
* [Output Only] The total number of instances in the managed instance group that are scheduled to
* be abandoned. Abandoning an instance removes it from the managed instance group without
* deleting it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer abandoning;
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* created or are currently being created. If the group fails to create any of these instances, it
* tries again until it creates the instance successfully.
*
* If you have disabled creation retries, this field will not be populated; instead, the
* creatingWithoutRetries field will be populated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer creating;
/**
* [Output Only] The number of instances that the managed instance group will attempt to create.
* The group attempts to create each instance only once. If the group fails to create any of these
* instances, it decreases the group's targetSize value accordingly.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer creatingWithoutRetries;
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* deleted or are currently being deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer deleting;
/**
* [Output Only] The number of instances in the managed instance group that are running and have
* no scheduled actions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer none;
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* recreated or are currently being being recreated. Recreating an instance deletes the existing
* root persistent disk and creates a new disk from the image that is defined in the instance
* template.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer recreating;
/**
* [Output Only] The number of instances in the managed instance group that are being reconfigured
* with properties that do not require a restart or a recreate action. For example, setting or
* removing target pools for the instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer refreshing;
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* restarted or are currently being restarted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer restarting;
/**
* [Output Only] The number of instances in the managed instance group that are being verified.
* More details regarding verification process are covered in the documentation of
* ManagedInstance.InstanceAction.VERIFYING enum field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer verifying;
/**
* [Output Only] The total number of instances in the managed instance group that are scheduled to
* be abandoned. Abandoning an instance removes it from the managed instance group without
* deleting it.
* @return value or {@code null} for none
*/
public java.lang.Integer getAbandoning() {
return abandoning;
}
/**
* [Output Only] The total number of instances in the managed instance group that are scheduled to
* be abandoned. Abandoning an instance removes it from the managed instance group without
* deleting it.
* @param abandoning abandoning or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setAbandoning(java.lang.Integer abandoning) {
this.abandoning = abandoning;
return this;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* created or are currently being created. If the group fails to create any of these instances, it
* tries again until it creates the instance successfully.
*
* If you have disabled creation retries, this field will not be populated; instead, the
* creatingWithoutRetries field will be populated.
* @return value or {@code null} for none
*/
public java.lang.Integer getCreating() {
return creating;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* created or are currently being created. If the group fails to create any of these instances, it
* tries again until it creates the instance successfully.
*
* If you have disabled creation retries, this field will not be populated; instead, the
* creatingWithoutRetries field will be populated.
* @param creating creating or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setCreating(java.lang.Integer creating) {
this.creating = creating;
return this;
}
/**
* [Output Only] The number of instances that the managed instance group will attempt to create.
* The group attempts to create each instance only once. If the group fails to create any of these
* instances, it decreases the group's targetSize value accordingly.
* @return value or {@code null} for none
*/
public java.lang.Integer getCreatingWithoutRetries() {
return creatingWithoutRetries;
}
/**
* [Output Only] The number of instances that the managed instance group will attempt to create.
* The group attempts to create each instance only once. If the group fails to create any of these
* instances, it decreases the group's targetSize value accordingly.
* @param creatingWithoutRetries creatingWithoutRetries or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setCreatingWithoutRetries(java.lang.Integer creatingWithoutRetries) {
this.creatingWithoutRetries = creatingWithoutRetries;
return this;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* deleted or are currently being deleted.
* @return value or {@code null} for none
*/
public java.lang.Integer getDeleting() {
return deleting;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* deleted or are currently being deleted.
* @param deleting deleting or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setDeleting(java.lang.Integer deleting) {
this.deleting = deleting;
return this;
}
/**
* [Output Only] The number of instances in the managed instance group that are running and have
* no scheduled actions.
* @return value or {@code null} for none
*/
public java.lang.Integer getNone() {
return none;
}
/**
* [Output Only] The number of instances in the managed instance group that are running and have
* no scheduled actions.
* @param none none or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setNone(java.lang.Integer none) {
this.none = none;
return this;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* recreated or are currently being being recreated. Recreating an instance deletes the existing
* root persistent disk and creates a new disk from the image that is defined in the instance
* template.
* @return value or {@code null} for none
*/
public java.lang.Integer getRecreating() {
return recreating;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* recreated or are currently being being recreated. Recreating an instance deletes the existing
* root persistent disk and creates a new disk from the image that is defined in the instance
* template.
* @param recreating recreating or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setRecreating(java.lang.Integer recreating) {
this.recreating = recreating;
return this;
}
/**
* [Output Only] The number of instances in the managed instance group that are being reconfigured
* with properties that do not require a restart or a recreate action. For example, setting or
* removing target pools for the instance.
* @return value or {@code null} for none
*/
public java.lang.Integer getRefreshing() {
return refreshing;
}
/**
* [Output Only] The number of instances in the managed instance group that are being reconfigured
* with properties that do not require a restart or a recreate action. For example, setting or
* removing target pools for the instance.
* @param refreshing refreshing or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setRefreshing(java.lang.Integer refreshing) {
this.refreshing = refreshing;
return this;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* restarted or are currently being restarted.
* @return value or {@code null} for none
*/
public java.lang.Integer getRestarting() {
return restarting;
}
/**
* [Output Only] The number of instances in the managed instance group that are scheduled to be
* restarted or are currently being restarted.
* @param restarting restarting or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setRestarting(java.lang.Integer restarting) {
this.restarting = restarting;
return this;
}
/**
* [Output Only] The number of instances in the managed instance group that are being verified.
* More details regarding verification process are covered in the documentation of
* ManagedInstance.InstanceAction.VERIFYING enum field.
* @return value or {@code null} for none
*/
public java.lang.Integer getVerifying() {
return verifying;
}
/**
* [Output Only] The number of instances in the managed instance group that are being verified.
* More details regarding verification process are covered in the documentation of
* ManagedInstance.InstanceAction.VERIFYING enum field.
* @param verifying verifying or {@code null} for none
*/
public InstanceGroupManagerActionsSummary setVerifying(java.lang.Integer verifying) {
this.verifying = verifying;
return this;
}
@Override
public InstanceGroupManagerActionsSummary set(String fieldName, Object value) {
return (InstanceGroupManagerActionsSummary) super.set(fieldName, value);
}
@Override
public InstanceGroupManagerActionsSummary clone() {
return (InstanceGroupManagerActionsSummary) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy