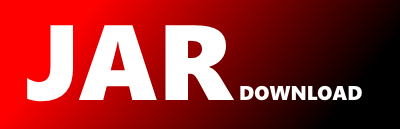
com.google.api.services.compute.model.NetworkInterface Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-08-07 at 18:49:37 UTC
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* A network interface resource attached to an instance.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class NetworkInterface extends com.google.api.client.json.GenericJson {
/**
* An array of configurations for this interface. Currently, only one access config,
* ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will
* have no external internet access.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List accessConfigs;
static {
// hack to force ProGuard to consider AccessConfig used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(AccessConfig.class);
}
/**
* An array of alias IP ranges for this network interface. Can only be specified for network
* interfaces on subnet-mode networks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List aliasIpRanges;
static {
// hack to force ProGuard to consider AliasIpRange used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(AliasIpRange.class);
}
/**
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* [Output Only] The name of the network interface, generated by the server. For network devices,
* these are eth0, eth1, etc.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* URL of the network resource for this instance. When creating an instance, if neither the
* network nor the subnetwork is specified, the default network global/networks/default is used;
* if the network is not specified but the subnetwork is specified, the network is inferred.
*
* This field is optional when creating a firewall rule. If not specified when creating a firewall
* rule, the default network global/networks/default is used.
*
* If you specify this property, you can specify the network as a full or partial URL. For
* example, the following are all valid URLs: -
* https://www.googleapis.com/compute/v1/projects/project/global/networks/network -
* projects/project/global/networks/network - global/networks/default
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String network;
/**
* An IPv4 internal network address to assign to the instance for this network interface. If not
* specified by the user, an unused internal IP is assigned by the system.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String networkIP;
/**
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy
* mode, do not provide this property. If the network is in auto subnet mode, providing the
* subnetwork is optional. If the network is in custom subnet mode, then this field should be
* specified. If you specify this property, you can specify the subnetwork as a full or partial
* URL. For example, the following are all valid URLs: -
* https://www.googleapis.com/compute/v1/projects/project/regions/region/subnetworks/subnetwork -
* regions/region/subnetworks/subnetwork
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String subnetwork;
/**
* An array of configurations for this interface. Currently, only one access config,
* ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will
* have no external internet access.
* @return value or {@code null} for none
*/
public java.util.List getAccessConfigs() {
return accessConfigs;
}
/**
* An array of configurations for this interface. Currently, only one access config,
* ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will
* have no external internet access.
* @param accessConfigs accessConfigs or {@code null} for none
*/
public NetworkInterface setAccessConfigs(java.util.List accessConfigs) {
this.accessConfigs = accessConfigs;
return this;
}
/**
* An array of alias IP ranges for this network interface. Can only be specified for network
* interfaces on subnet-mode networks.
* @return value or {@code null} for none
*/
public java.util.List getAliasIpRanges() {
return aliasIpRanges;
}
/**
* An array of alias IP ranges for this network interface. Can only be specified for network
* interfaces on subnet-mode networks.
* @param aliasIpRanges aliasIpRanges or {@code null} for none
*/
public NetworkInterface setAliasIpRanges(java.util.List aliasIpRanges) {
this.aliasIpRanges = aliasIpRanges;
return this;
}
/**
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
* @param kind kind or {@code null} for none
*/
public NetworkInterface setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* [Output Only] The name of the network interface, generated by the server. For network devices,
* these are eth0, eth1, etc.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* [Output Only] The name of the network interface, generated by the server. For network devices,
* these are eth0, eth1, etc.
* @param name name or {@code null} for none
*/
public NetworkInterface setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* URL of the network resource for this instance. When creating an instance, if neither the
* network nor the subnetwork is specified, the default network global/networks/default is used;
* if the network is not specified but the subnetwork is specified, the network is inferred.
*
* This field is optional when creating a firewall rule. If not specified when creating a firewall
* rule, the default network global/networks/default is used.
*
* If you specify this property, you can specify the network as a full or partial URL. For
* example, the following are all valid URLs: -
* https://www.googleapis.com/compute/v1/projects/project/global/networks/network -
* projects/project/global/networks/network - global/networks/default
* @return value or {@code null} for none
*/
public java.lang.String getNetwork() {
return network;
}
/**
* URL of the network resource for this instance. When creating an instance, if neither the
* network nor the subnetwork is specified, the default network global/networks/default is used;
* if the network is not specified but the subnetwork is specified, the network is inferred.
*
* This field is optional when creating a firewall rule. If not specified when creating a firewall
* rule, the default network global/networks/default is used.
*
* If you specify this property, you can specify the network as a full or partial URL. For
* example, the following are all valid URLs: -
* https://www.googleapis.com/compute/v1/projects/project/global/networks/network -
* projects/project/global/networks/network - global/networks/default
* @param network network or {@code null} for none
*/
public NetworkInterface setNetwork(java.lang.String network) {
this.network = network;
return this;
}
/**
* An IPv4 internal network address to assign to the instance for this network interface. If not
* specified by the user, an unused internal IP is assigned by the system.
* @return value or {@code null} for none
*/
public java.lang.String getNetworkIP() {
return networkIP;
}
/**
* An IPv4 internal network address to assign to the instance for this network interface. If not
* specified by the user, an unused internal IP is assigned by the system.
* @param networkIP networkIP or {@code null} for none
*/
public NetworkInterface setNetworkIP(java.lang.String networkIP) {
this.networkIP = networkIP;
return this;
}
/**
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy
* mode, do not provide this property. If the network is in auto subnet mode, providing the
* subnetwork is optional. If the network is in custom subnet mode, then this field should be
* specified. If you specify this property, you can specify the subnetwork as a full or partial
* URL. For example, the following are all valid URLs: -
* https://www.googleapis.com/compute/v1/projects/project/regions/region/subnetworks/subnetwork -
* regions/region/subnetworks/subnetwork
* @return value or {@code null} for none
*/
public java.lang.String getSubnetwork() {
return subnetwork;
}
/**
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy
* mode, do not provide this property. If the network is in auto subnet mode, providing the
* subnetwork is optional. If the network is in custom subnet mode, then this field should be
* specified. If you specify this property, you can specify the subnetwork as a full or partial
* URL. For example, the following are all valid URLs: -
* https://www.googleapis.com/compute/v1/projects/project/regions/region/subnetworks/subnetwork -
* regions/region/subnetworks/subnetwork
* @param subnetwork subnetwork or {@code null} for none
*/
public NetworkInterface setSubnetwork(java.lang.String subnetwork) {
this.subnetwork = subnetwork;
return this;
}
@Override
public NetworkInterface set(String fieldName, Object value) {
return (NetworkInterface) super.set(fieldName, value);
}
@Override
public NetworkInterface clone() {
return (NetworkInterface) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy