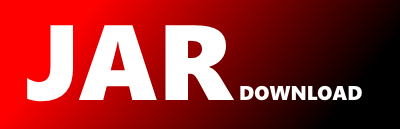
com.google.api.services.compute.model.Backend Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-08-03 17:34:38 UTC)
* on 2015-10-29 at 23:19:12 UTC
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* Message containing information of one individual backend.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Backend extends com.google.api.client.json.GenericJson {
/**
* Specifies the balancing mode for this backend. The default is UTILIZATION but available values
* are UTILIZATION and RATE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String balancingMode;
/**
* A multiplier applied to the group's maximum servicing capacity (either UTILIZATION or RATE).
* Default value is 1, which means the group will serve up to 100% of its configured CPU or RPS
* (depending on balancingMode). A setting of 0 means the group is completely drained, offering 0%
* of its available CPU or RPS. Valid range is [0.0,1.0].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float capacityScaler;
/**
* An optional textual description of the resource. Provided by the client when the resource is
* created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The fully-qualified URL of a zonal Instance Group resource. This instance group defines the
* list of instances that serve traffic. Member virtual machine instances from each instance group
* must live in the same zone as the instance group itself. No two backends in a backend service
* are allowed to use same Instance Group resource.
*
* Note that you must specify an Instance Group resource using the fully-qualified URL, rather
* than a partial URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String group;
/**
* The max RPS of the group. Can be used with either balancing mode, but required if RATE mode.
* For RATE mode, either maxRate or maxRatePerInstance must be set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxRate;
/**
* The max RPS that a single backed instance can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode, either maxRate or
* maxRatePerInstance must be set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float maxRatePerInstance;
/**
* Used when balancingMode is UTILIZATION. This ratio defines the CPU utilization target for the
* group. The default is 0.8. Valid range is [0.0, 1.0].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float maxUtilization;
/**
* Specifies the balancing mode for this backend. The default is UTILIZATION but available values
* are UTILIZATION and RATE.
* @return value or {@code null} for none
*/
public java.lang.String getBalancingMode() {
return balancingMode;
}
/**
* Specifies the balancing mode for this backend. The default is UTILIZATION but available values
* are UTILIZATION and RATE.
* @param balancingMode balancingMode or {@code null} for none
*/
public Backend setBalancingMode(java.lang.String balancingMode) {
this.balancingMode = balancingMode;
return this;
}
/**
* A multiplier applied to the group's maximum servicing capacity (either UTILIZATION or RATE).
* Default value is 1, which means the group will serve up to 100% of its configured CPU or RPS
* (depending on balancingMode). A setting of 0 means the group is completely drained, offering 0%
* of its available CPU or RPS. Valid range is [0.0,1.0].
* @return value or {@code null} for none
*/
public java.lang.Float getCapacityScaler() {
return capacityScaler;
}
/**
* A multiplier applied to the group's maximum servicing capacity (either UTILIZATION or RATE).
* Default value is 1, which means the group will serve up to 100% of its configured CPU or RPS
* (depending on balancingMode). A setting of 0 means the group is completely drained, offering 0%
* of its available CPU or RPS. Valid range is [0.0,1.0].
* @param capacityScaler capacityScaler or {@code null} for none
*/
public Backend setCapacityScaler(java.lang.Float capacityScaler) {
this.capacityScaler = capacityScaler;
return this;
}
/**
* An optional textual description of the resource. Provided by the client when the resource is
* created.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* An optional textual description of the resource. Provided by the client when the resource is
* created.
* @param description description or {@code null} for none
*/
public Backend setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The fully-qualified URL of a zonal Instance Group resource. This instance group defines the
* list of instances that serve traffic. Member virtual machine instances from each instance group
* must live in the same zone as the instance group itself. No two backends in a backend service
* are allowed to use same Instance Group resource.
*
* Note that you must specify an Instance Group resource using the fully-qualified URL, rather
* than a partial URL.
* @return value or {@code null} for none
*/
public java.lang.String getGroup() {
return group;
}
/**
* The fully-qualified URL of a zonal Instance Group resource. This instance group defines the
* list of instances that serve traffic. Member virtual machine instances from each instance group
* must live in the same zone as the instance group itself. No two backends in a backend service
* are allowed to use same Instance Group resource.
*
* Note that you must specify an Instance Group resource using the fully-qualified URL, rather
* than a partial URL.
* @param group group or {@code null} for none
*/
public Backend setGroup(java.lang.String group) {
this.group = group;
return this;
}
/**
* The max RPS of the group. Can be used with either balancing mode, but required if RATE mode.
* For RATE mode, either maxRate or maxRatePerInstance must be set.
* @return value or {@code null} for none
*/
public java.lang.Integer getMaxRate() {
return maxRate;
}
/**
* The max RPS of the group. Can be used with either balancing mode, but required if RATE mode.
* For RATE mode, either maxRate or maxRatePerInstance must be set.
* @param maxRate maxRate or {@code null} for none
*/
public Backend setMaxRate(java.lang.Integer maxRate) {
this.maxRate = maxRate;
return this;
}
/**
* The max RPS that a single backed instance can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode, either maxRate or
* maxRatePerInstance must be set.
* @return value or {@code null} for none
*/
public java.lang.Float getMaxRatePerInstance() {
return maxRatePerInstance;
}
/**
* The max RPS that a single backed instance can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode, either maxRate or
* maxRatePerInstance must be set.
* @param maxRatePerInstance maxRatePerInstance or {@code null} for none
*/
public Backend setMaxRatePerInstance(java.lang.Float maxRatePerInstance) {
this.maxRatePerInstance = maxRatePerInstance;
return this;
}
/**
* Used when balancingMode is UTILIZATION. This ratio defines the CPU utilization target for the
* group. The default is 0.8. Valid range is [0.0, 1.0].
* @return value or {@code null} for none
*/
public java.lang.Float getMaxUtilization() {
return maxUtilization;
}
/**
* Used when balancingMode is UTILIZATION. This ratio defines the CPU utilization target for the
* group. The default is 0.8. Valid range is [0.0, 1.0].
* @param maxUtilization maxUtilization or {@code null} for none
*/
public Backend setMaxUtilization(java.lang.Float maxUtilization) {
this.maxUtilization = maxUtilization;
return this;
}
@Override
public Backend set(String fieldName, Object value) {
return (Backend) super.set(fieldName, value);
}
@Override
public Backend clone() {
return (Backend) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy