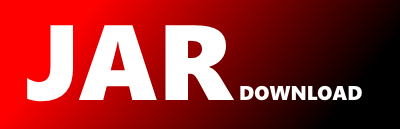
com.google.api.services.compute.model.AttachedDisk Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2013-05-14 03:07:32 UTC)
* on 2013-05-15 at 01:58:25 UTC
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* An instance-attached disk resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* http://code.google.com/p/google-api-java-client/wiki/Json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AttachedDisk extends com.google.api.client.json.GenericJson {
/**
* Indicates that this is a boot disk. VM will use the first partition of the disk for its root
* filesystem.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean boot;
/**
* Persistent disk only; must be unique within the instance when specified. This represents a
* unique device name that is reflected into the /dev/ tree of a Linux operating system running
* within the instance. If not specified, a default will be chosen by the system.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deviceName;
/**
* A zero-based index to assign to this disk, where 0 is reserved for the boot disk. If not
* specified, the server will choose an appropriate value (output only).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer index;
/**
* Type of the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The mode in which to attach this disk, either "READ_WRITE" or "READ_ONLY".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mode;
/**
* Persistent disk only; the URL of the persistent disk resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String source;
/**
* Type of the disk, either "SCRATCH" or "PERSISTENT". Note that persistent disks must be created
* before you can specify them here.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* Indicates that this is a boot disk. VM will use the first partition of the disk for its root
* filesystem.
* @return value or {@code null} for none
*/
public java.lang.Boolean getBoot() {
return boot;
}
/**
* Indicates that this is a boot disk. VM will use the first partition of the disk for its root
* filesystem.
* @param boot boot or {@code null} for none
*/
public AttachedDisk setBoot(java.lang.Boolean boot) {
this.boot = boot;
return this;
}
/**
* Persistent disk only; must be unique within the instance when specified. This represents a
* unique device name that is reflected into the /dev/ tree of a Linux operating system running
* within the instance. If not specified, a default will be chosen by the system.
* @return value or {@code null} for none
*/
public java.lang.String getDeviceName() {
return deviceName;
}
/**
* Persistent disk only; must be unique within the instance when specified. This represents a
* unique device name that is reflected into the /dev/ tree of a Linux operating system running
* within the instance. If not specified, a default will be chosen by the system.
* @param deviceName deviceName or {@code null} for none
*/
public AttachedDisk setDeviceName(java.lang.String deviceName) {
this.deviceName = deviceName;
return this;
}
/**
* A zero-based index to assign to this disk, where 0 is reserved for the boot disk. If not
* specified, the server will choose an appropriate value (output only).
* @return value or {@code null} for none
*/
public java.lang.Integer getIndex() {
return index;
}
/**
* A zero-based index to assign to this disk, where 0 is reserved for the boot disk. If not
* specified, the server will choose an appropriate value (output only).
* @param index index or {@code null} for none
*/
public AttachedDisk setIndex(java.lang.Integer index) {
this.index = index;
return this;
}
/**
* Type of the resource.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Type of the resource.
* @param kind kind or {@code null} for none
*/
public AttachedDisk setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The mode in which to attach this disk, either "READ_WRITE" or "READ_ONLY".
* @return value or {@code null} for none
*/
public java.lang.String getMode() {
return mode;
}
/**
* The mode in which to attach this disk, either "READ_WRITE" or "READ_ONLY".
* @param mode mode or {@code null} for none
*/
public AttachedDisk setMode(java.lang.String mode) {
this.mode = mode;
return this;
}
/**
* Persistent disk only; the URL of the persistent disk resource.
* @return value or {@code null} for none
*/
public java.lang.String getSource() {
return source;
}
/**
* Persistent disk only; the URL of the persistent disk resource.
* @param source source or {@code null} for none
*/
public AttachedDisk setSource(java.lang.String source) {
this.source = source;
return this;
}
/**
* Type of the disk, either "SCRATCH" or "PERSISTENT". Note that persistent disks must be created
* before you can specify them here.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Type of the disk, either "SCRATCH" or "PERSISTENT". Note that persistent disks must be created
* before you can specify them here.
* @param type type or {@code null} for none
*/
public AttachedDisk setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public AttachedDisk set(String fieldName, Object value) {
return (AttachedDisk) super.set(fieldName, value);
}
@Override
public AttachedDisk clone() {
return (AttachedDisk) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy