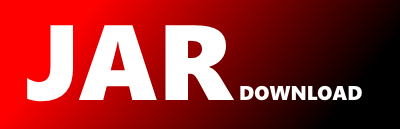
com.google.api.services.compute.model.Firewall Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2013-05-14 03:07:32 UTC)
* on 2013-05-15 at 01:58:25 UTC
* Modify at your own risk.
*/
package com.google.api.services.compute.model;
/**
* A firewall resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Engine API. For a detailed explanation see:
* http://code.google.com/p/google-api-java-client/wiki/Json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Firewall extends com.google.api.client.json.GenericJson {
/**
* The list of rules specified by this firewall. Each rule specifies a protocol and port-range
* tuple that describes a permitted connection.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List allowed;
static {
// hack to force ProGuard to consider Allowed used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Allowed.class);
}
/**
* Creation timestamp in RFC3339 text format (output only).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creationTimestamp;
/**
* An optional textual description of the resource; provided by the client when the resource is
* created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Unique identifier for the resource; defined by the server (output only).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger id;
/**
* Type of the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* URL of the network to which this firewall is applied; provided by the client when the firewall
* is created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String network;
/**
* Server defined URL for the resource (output only).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* A list of IP address blocks expressed in CIDR format which this rule applies to. One or both of
* sourceRanges and sourceTags may be set; an inbound connection is allowed if either the range or
* the tag of the source matches.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sourceRanges;
/**
* A list of instance tags which this rule applies to. One or both of sourceRanges and sourceTags
* may be set; an inbound connection is allowed if either the range or the tag of the source
* matches.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sourceTags;
/**
* A list of instance tags indicating sets of instances located on network which may make network
* connections as specified in allowed. If no targetTags are specified, the firewall rule applies
* to all instances on the specified network.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List targetTags;
/**
* The list of rules specified by this firewall. Each rule specifies a protocol and port-range
* tuple that describes a permitted connection.
* @return value or {@code null} for none
*/
public java.util.List getAllowed() {
return allowed;
}
/**
* The list of rules specified by this firewall. Each rule specifies a protocol and port-range
* tuple that describes a permitted connection.
* @param allowed allowed or {@code null} for none
*/
public Firewall setAllowed(java.util.List allowed) {
this.allowed = allowed;
return this;
}
/**
* Creation timestamp in RFC3339 text format (output only).
* @return value or {@code null} for none
*/
public java.lang.String getCreationTimestamp() {
return creationTimestamp;
}
/**
* Creation timestamp in RFC3339 text format (output only).
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public Firewall setCreationTimestamp(java.lang.String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* An optional textual description of the resource; provided by the client when the resource is
* created.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* An optional textual description of the resource; provided by the client when the resource is
* created.
* @param description description or {@code null} for none
*/
public Firewall setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Unique identifier for the resource; defined by the server (output only).
* @return value or {@code null} for none
*/
public java.math.BigInteger getId() {
return id;
}
/**
* Unique identifier for the resource; defined by the server (output only).
* @param id id or {@code null} for none
*/
public Firewall setId(java.math.BigInteger id) {
this.id = id;
return this;
}
/**
* Type of the resource.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Type of the resource.
* @param kind kind or {@code null} for none
*/
public Firewall setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the resource; provided by the client when the resource is created. The name must be
* 1-63 characters long, and comply with RFC1035.
* @param name name or {@code null} for none
*/
public Firewall setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* URL of the network to which this firewall is applied; provided by the client when the firewall
* is created.
* @return value or {@code null} for none
*/
public java.lang.String getNetwork() {
return network;
}
/**
* URL of the network to which this firewall is applied; provided by the client when the firewall
* is created.
* @param network network or {@code null} for none
*/
public Firewall setNetwork(java.lang.String network) {
this.network = network;
return this;
}
/**
* Server defined URL for the resource (output only).
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* Server defined URL for the resource (output only).
* @param selfLink selfLink or {@code null} for none
*/
public Firewall setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* A list of IP address blocks expressed in CIDR format which this rule applies to. One or both of
* sourceRanges and sourceTags may be set; an inbound connection is allowed if either the range or
* the tag of the source matches.
* @return value or {@code null} for none
*/
public java.util.List getSourceRanges() {
return sourceRanges;
}
/**
* A list of IP address blocks expressed in CIDR format which this rule applies to. One or both of
* sourceRanges and sourceTags may be set; an inbound connection is allowed if either the range or
* the tag of the source matches.
* @param sourceRanges sourceRanges or {@code null} for none
*/
public Firewall setSourceRanges(java.util.List sourceRanges) {
this.sourceRanges = sourceRanges;
return this;
}
/**
* A list of instance tags which this rule applies to. One or both of sourceRanges and sourceTags
* may be set; an inbound connection is allowed if either the range or the tag of the source
* matches.
* @return value or {@code null} for none
*/
public java.util.List getSourceTags() {
return sourceTags;
}
/**
* A list of instance tags which this rule applies to. One or both of sourceRanges and sourceTags
* may be set; an inbound connection is allowed if either the range or the tag of the source
* matches.
* @param sourceTags sourceTags or {@code null} for none
*/
public Firewall setSourceTags(java.util.List sourceTags) {
this.sourceTags = sourceTags;
return this;
}
/**
* A list of instance tags indicating sets of instances located on network which may make network
* connections as specified in allowed. If no targetTags are specified, the firewall rule applies
* to all instances on the specified network.
* @return value or {@code null} for none
*/
public java.util.List getTargetTags() {
return targetTags;
}
/**
* A list of instance tags indicating sets of instances located on network which may make network
* connections as specified in allowed. If no targetTags are specified, the firewall rule applies
* to all instances on the specified network.
* @param targetTags targetTags or {@code null} for none
*/
public Firewall setTargetTags(java.util.List targetTags) {
this.targetTags = targetTags;
return this;
}
@Override
public Firewall set(String fieldName, Object value) {
return (Firewall) super.set(fieldName, value);
}
@Override
public Firewall clone() {
return (Firewall) super.clone();
}
/**
* Model definition for FirewallAllowed.
*/
public static final class Allowed extends com.google.api.client.json.GenericJson {
/**
* Required; this is the IP protocol that is allowed for this rule. This can either be a well
* known protocol string (tcp, udp or icmp) or the IP protocol number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("IPProtocol")
private java.lang.String iPProtocol;
/**
* An optional list of ports which are allowed. It is an error to specify this for any protocol
* that isn't UDP or TCP. Each entry must be either an integer or a range. If not specified,
* connections through any port are allowed.
*
* Example inputs include: ["22"], ["80","443"] and ["12345-12349"].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List ports;
/**
* Required; this is the IP protocol that is allowed for this rule. This can either be a well
* known protocol string (tcp, udp or icmp) or the IP protocol number.
* @return value or {@code null} for none
*/
public java.lang.String getIPProtocol() {
return iPProtocol;
}
/**
* Required; this is the IP protocol that is allowed for this rule. This can either be a well
* known protocol string (tcp, udp or icmp) or the IP protocol number.
* @param iPProtocol iPProtocol or {@code null} for none
*/
public Allowed setIPProtocol(java.lang.String iPProtocol) {
this.iPProtocol = iPProtocol;
return this;
}
/**
* An optional list of ports which are allowed. It is an error to specify this for any protocol
* that isn't UDP or TCP. Each entry must be either an integer or a range. If not specified,
* connections through any port are allowed.
*
* Example inputs include: ["22"], ["80","443"] and ["12345-12349"].
* @return value or {@code null} for none
*/
public java.util.List getPorts() {
return ports;
}
/**
* An optional list of ports which are allowed. It is an error to specify this for any protocol
* that isn't UDP or TCP. Each entry must be either an integer or a range. If not specified,
* connections through any port are allowed.
*
* Example inputs include: ["22"], ["80","443"] and ["12345-12349"].
* @param ports ports or {@code null} for none
*/
public Allowed setPorts(java.util.List ports) {
this.ports = ports;
return this;
}
@Override
public Allowed set(String fieldName, Object value) {
return (Allowed) super.set(fieldName, value);
}
@Override
public Allowed clone() {
return (Allowed) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy