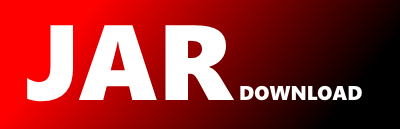
com.google.api.services.computeaccounts.ComputeAccounts Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-08-03 17:34:38 UTC)
* on 2015-09-07 at 15:02:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.computeaccounts;
/**
* Service definition for ComputeAccounts (alpha).
*
*
* API for the Google Compute Accounts service.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link ComputeAccountsRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class ComputeAccounts extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.20.0 of the Compute Accounts API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "computeaccounts/alpha/projects/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public ComputeAccounts(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
ComputeAccounts(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the GlobalAccountsOperations collection.
*
* The typical use is:
*
* {@code ComputeAccounts computeaccounts = new ComputeAccounts(...);}
* {@code ComputeAccounts.GlobalAccountsOperations.List request = computeaccounts.globalAccountsOperations().list(parameters ...)}
*
*
* @return the resource collection
*/
public GlobalAccountsOperations globalAccountsOperations() {
return new GlobalAccountsOperations();
}
/**
* The "globalAccountsOperations" collection of methods.
*/
public class GlobalAccountsOperations {
/**
* Deletes the specified operation resource.
*
* Create a request for the method "globalAccountsOperations.delete".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param operation Name of the Operations resource to delete.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String operation) throws java.io.IOException {
Delete result = new Delete(project, operation);
initialize(result);
return result;
}
public class Delete extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/operations/{operation}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern OPERATION_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Deletes the specified operation resource.
*
* Create a request for the method "globalAccountsOperations.delete".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param operation Name of the Operations resource to delete.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String operation) {
super(ComputeAccounts.this, "DELETE", REST_PATH, null, Void.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.operation = com.google.api.client.util.Preconditions.checkNotNull(operation, "Required parameter operation must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_PATTERN.matcher(operation).matches(),
"Parameter operation must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Delete setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the Operations resource to delete. */
@com.google.api.client.util.Key
private java.lang.String operation;
/** Name of the Operations resource to delete.
*/
public java.lang.String getOperation() {
return operation;
}
/** Name of the Operations resource to delete. */
public Delete setOperation(java.lang.String operation) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_PATTERN.matcher(operation).matches(),
"Parameter operation must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.operation = operation;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified operation resource.
*
* Create a request for the method "globalAccountsOperations.get".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param operation Name of the Operations resource to return.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String operation) throws java.io.IOException {
Get result = new Get(project, operation);
initialize(result);
return result;
}
public class Get extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/operations/{operation}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern OPERATION_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Retrieves the specified operation resource.
*
* Create a request for the method "globalAccountsOperations.get".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param operation Name of the Operations resource to return.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String operation) {
super(ComputeAccounts.this, "GET", REST_PATH, null, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.operation = com.google.api.client.util.Preconditions.checkNotNull(operation, "Required parameter operation must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_PATTERN.matcher(operation).matches(),
"Parameter operation must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Get setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the Operations resource to return. */
@com.google.api.client.util.Key
private java.lang.String operation;
/** Name of the Operations resource to return.
*/
public java.lang.String getOperation() {
return operation;
}
/** Name of the Operations resource to return. */
public Get setOperation(java.lang.String operation) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_PATTERN.matcher(operation).matches(),
"Parameter operation must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.operation = operation;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the list of operation resources contained within the specified project.
*
* Create a request for the method "globalAccountsOperations.list".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/operations";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Retrieves the list of operation resources contained within the specified project.
*
* Create a request for the method "globalAccountsOperations.list".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @since 1.13
*/
protected List(java.lang.String project) {
super(ComputeAccounts.this, "GET", REST_PATH, null, com.google.api.services.computeaccounts.model.OperationList.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Sets a filter expression for filtering listed resources, in the form filter={expression}. Your
{expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
- FIELD_NAME: The name of the field you want to compare. The field name must be valid for the type
of resource being filtered. Only atomic field types are supported (string, number, boolean). Array
and object fields are not currently supported. - COMPARISON_STRING: The comparison string, either
eq (equals) or ne (not equals). - LITERAL_STRING: The literal string value to filter to. The
literal value must be valid for the type of field (string, number, boolean). For string fields, the
literal value is interpreted as a regular expression using RE2 syntax. The literal value must match
the entire field. For example, you can filter by the name of a resource: filter=name ne example-
instance The above filter returns only results whose name field does not equal example-instance.
You can also enclose your literal string in single, double, or no quotes.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name.
You can also sort results in descending order based on the creation timestamp using
orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field in
reverse chronological order (newest result first). Use this to sort resources like operations so
that the newest operation is returned first.
Currently, only sorting by name or creationTimestamp desc is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** Maximum count of results to be returned. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum count of results to be returned. [default: 500] [minimum: 0] [maximum: 500]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum count of results to be returned. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Use this parameter if you want to list the next page of results. Set
pageToken to the nextPageToken returned by a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Groups collection.
*
* The typical use is:
*
* {@code ComputeAccounts computeaccounts = new ComputeAccounts(...);}
* {@code ComputeAccounts.Groups.List request = computeaccounts.groups().list(parameters ...)}
*
*
* @return the resource collection
*/
public Groups groups() {
return new Groups();
}
/**
* The "groups" collection of methods.
*/
public class Groups {
/**
* Adds users to the specified group.
*
* Create a request for the method "groups.addMember".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link AddMember#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param groupName Name of the group for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.GroupsAddMemberRequest}
* @return the request
*/
public AddMember addMember(java.lang.String project, java.lang.String groupName, com.google.api.services.computeaccounts.model.GroupsAddMemberRequest content) throws java.io.IOException {
AddMember result = new AddMember(project, groupName, content);
initialize(result);
return result;
}
public class AddMember extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/groups/{groupName}/addMember";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern GROUP_NAME_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Adds users to the specified group.
*
* Create a request for the method "groups.addMember".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link AddMember#execute()} method to invoke the remote
* operation. {@link
* AddMember#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param groupName Name of the group for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.GroupsAddMemberRequest}
* @since 1.13
*/
protected AddMember(java.lang.String project, java.lang.String groupName, com.google.api.services.computeaccounts.model.GroupsAddMemberRequest content) {
super(ComputeAccounts.this, "POST", REST_PATH, content, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.groupName = com.google.api.client.util.Preconditions.checkNotNull(groupName, "Required parameter groupName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
}
@Override
public AddMember setAlt(java.lang.String alt) {
return (AddMember) super.setAlt(alt);
}
@Override
public AddMember setFields(java.lang.String fields) {
return (AddMember) super.setFields(fields);
}
@Override
public AddMember setKey(java.lang.String key) {
return (AddMember) super.setKey(key);
}
@Override
public AddMember setOauthToken(java.lang.String oauthToken) {
return (AddMember) super.setOauthToken(oauthToken);
}
@Override
public AddMember setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddMember) super.setPrettyPrint(prettyPrint);
}
@Override
public AddMember setQuotaUser(java.lang.String quotaUser) {
return (AddMember) super.setQuotaUser(quotaUser);
}
@Override
public AddMember setUserIp(java.lang.String userIp) {
return (AddMember) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public AddMember setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the group for this request. */
@com.google.api.client.util.Key
private java.lang.String groupName;
/** Name of the group for this request.
*/
public java.lang.String getGroupName() {
return groupName;
}
/** Name of the group for this request. */
public AddMember setGroupName(java.lang.String groupName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.groupName = groupName;
return this;
}
@Override
public AddMember set(String parameterName, Object value) {
return (AddMember) super.set(parameterName, value);
}
}
/**
* Deletes the specified Group resource.
*
* Create a request for the method "groups.delete".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param groupName Name of the Group resource to delete.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String groupName) throws java.io.IOException {
Delete result = new Delete(project, groupName);
initialize(result);
return result;
}
public class Delete extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/groups/{groupName}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern GROUP_NAME_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Deletes the specified Group resource.
*
* Create a request for the method "groups.delete".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param groupName Name of the Group resource to delete.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String groupName) {
super(ComputeAccounts.this, "DELETE", REST_PATH, null, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.groupName = com.google.api.client.util.Preconditions.checkNotNull(groupName, "Required parameter groupName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Delete setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the Group resource to delete. */
@com.google.api.client.util.Key
private java.lang.String groupName;
/** Name of the Group resource to delete.
*/
public java.lang.String getGroupName() {
return groupName;
}
/** Name of the Group resource to delete. */
public Delete setGroupName(java.lang.String groupName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.groupName = groupName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns the specified Group resource.
*
* Create a request for the method "groups.get".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param groupName Name of the Group resource to return.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String groupName) throws java.io.IOException {
Get result = new Get(project, groupName);
initialize(result);
return result;
}
public class Get extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/groups/{groupName}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern GROUP_NAME_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Returns the specified Group resource.
*
* Create a request for the method "groups.get".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param groupName Name of the Group resource to return.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String groupName) {
super(ComputeAccounts.this, "GET", REST_PATH, null, com.google.api.services.computeaccounts.model.Group.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.groupName = com.google.api.client.util.Preconditions.checkNotNull(groupName, "Required parameter groupName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Get setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the Group resource to return. */
@com.google.api.client.util.Key
private java.lang.String groupName;
/** Name of the Group resource to return.
*/
public java.lang.String getGroupName() {
return groupName;
}
/** Name of the Group resource to return. */
public Get setGroupName(java.lang.String groupName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.groupName = groupName;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a Group resource in the specified project using the data included in the request.
*
* Create a request for the method "groups.insert".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.Group}
* @return the request
*/
public Insert insert(java.lang.String project, com.google.api.services.computeaccounts.model.Group content) throws java.io.IOException {
Insert result = new Insert(project, content);
initialize(result);
return result;
}
public class Insert extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/groups";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Creates a Group resource in the specified project using the data included in the request.
*
* Create a request for the method "groups.insert".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.Group}
* @since 1.13
*/
protected Insert(java.lang.String project, com.google.api.services.computeaccounts.model.Group content) {
super(ComputeAccounts.this, "POST", REST_PATH, content, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Insert setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the list of groups contained within the specified project.
*
* Create a request for the method "groups.list".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/groups";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Retrieves the list of groups contained within the specified project.
*
* Create a request for the method "groups.list".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @since 1.13
*/
protected List(java.lang.String project) {
super(ComputeAccounts.this, "GET", REST_PATH, null, com.google.api.services.computeaccounts.model.GroupList.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Sets a filter expression for filtering listed resources, in the form filter={expression}. Your
{expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
- FIELD_NAME: The name of the field you want to compare. The field name must be valid for the type
of resource being filtered. Only atomic field types are supported (string, number, boolean). Array
and object fields are not currently supported. - COMPARISON_STRING: The comparison string, either
eq (equals) or ne (not equals). - LITERAL_STRING: The literal string value to filter to. The
literal value must be valid for the type of field (string, number, boolean). For string fields, the
literal value is interpreted as a regular expression using RE2 syntax. The literal value must match
the entire field. For example, you can filter by the name of a resource: filter=name ne example-
instance The above filter returns only results whose name field does not equal example-instance.
You can also enclose your literal string in single, double, or no quotes.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name.
You can also sort results in descending order based on the creation timestamp using
orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field in
reverse chronological order (newest result first). Use this to sort resources like operations so
that the newest operation is returned first.
Currently, only sorting by name or creationTimestamp desc is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** Maximum count of results to be returned. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum count of results to be returned. [default: 500] [minimum: 0] [maximum: 500]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum count of results to be returned. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Use this parameter if you want to list the next page of results. Set
pageToken to the nextPageToken returned by a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Removes users from the specified group.
*
* Create a request for the method "groups.removeMember".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link RemoveMember#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param groupName Name of the group for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.GroupsRemoveMemberRequest}
* @return the request
*/
public RemoveMember removeMember(java.lang.String project, java.lang.String groupName, com.google.api.services.computeaccounts.model.GroupsRemoveMemberRequest content) throws java.io.IOException {
RemoveMember result = new RemoveMember(project, groupName, content);
initialize(result);
return result;
}
public class RemoveMember extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/groups/{groupName}/removeMember";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern GROUP_NAME_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Removes users from the specified group.
*
* Create a request for the method "groups.removeMember".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link RemoveMember#execute()} method to invoke the remote
* operation. {@link
* RemoveMember#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param groupName Name of the group for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.GroupsRemoveMemberRequest}
* @since 1.13
*/
protected RemoveMember(java.lang.String project, java.lang.String groupName, com.google.api.services.computeaccounts.model.GroupsRemoveMemberRequest content) {
super(ComputeAccounts.this, "POST", REST_PATH, content, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.groupName = com.google.api.client.util.Preconditions.checkNotNull(groupName, "Required parameter groupName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
}
@Override
public RemoveMember setAlt(java.lang.String alt) {
return (RemoveMember) super.setAlt(alt);
}
@Override
public RemoveMember setFields(java.lang.String fields) {
return (RemoveMember) super.setFields(fields);
}
@Override
public RemoveMember setKey(java.lang.String key) {
return (RemoveMember) super.setKey(key);
}
@Override
public RemoveMember setOauthToken(java.lang.String oauthToken) {
return (RemoveMember) super.setOauthToken(oauthToken);
}
@Override
public RemoveMember setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveMember) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveMember setQuotaUser(java.lang.String quotaUser) {
return (RemoveMember) super.setQuotaUser(quotaUser);
}
@Override
public RemoveMember setUserIp(java.lang.String userIp) {
return (RemoveMember) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public RemoveMember setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the group for this request. */
@com.google.api.client.util.Key
private java.lang.String groupName;
/** Name of the group for this request.
*/
public java.lang.String getGroupName() {
return groupName;
}
/** Name of the group for this request. */
public RemoveMember setGroupName(java.lang.String groupName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(GROUP_NAME_PATTERN.matcher(groupName).matches(),
"Parameter groupName must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.groupName = groupName;
return this;
}
@Override
public RemoveMember set(String parameterName, Object value) {
return (RemoveMember) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Linux collection.
*
* The typical use is:
*
* {@code ComputeAccounts computeaccounts = new ComputeAccounts(...);}
* {@code ComputeAccounts.Linux.List request = computeaccounts.linux().list(parameters ...)}
*
*
* @return the resource collection
*/
public Linux linux() {
return new Linux();
}
/**
* The "linux" collection of methods.
*/
public class Linux {
/**
* Returns a list of authorized public keys for a specific user account.
*
* Create a request for the method "linux.getAuthorizedKeysView".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link GetAuthorizedKeysView#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param zone Name of the zone for this request.
* @param user The user account for which you want to get a list of authorized public keys.
* @param instance The fully-qualified URL of the virtual machine requesting the view.
* @return the request
*/
public GetAuthorizedKeysView getAuthorizedKeysView(java.lang.String project, java.lang.String zone, java.lang.String user, java.lang.String instance) throws java.io.IOException {
GetAuthorizedKeysView result = new GetAuthorizedKeysView(project, zone, user, instance);
initialize(result);
return result;
}
public class GetAuthorizedKeysView extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/zones/{zone}/authorizedKeysView/{user}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern ZONE_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
private final java.util.regex.Pattern USER_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Returns a list of authorized public keys for a specific user account.
*
* Create a request for the method "linux.getAuthorizedKeysView".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link GetAuthorizedKeysView#execute()} method to invoke the
* remote operation. {@link GetAuthorizedKeysView#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Project ID for this request.
* @param zone Name of the zone for this request.
* @param user The user account for which you want to get a list of authorized public keys.
* @param instance The fully-qualified URL of the virtual machine requesting the view.
* @since 1.13
*/
protected GetAuthorizedKeysView(java.lang.String project, java.lang.String zone, java.lang.String user, java.lang.String instance) {
super(ComputeAccounts.this, "POST", REST_PATH, null, com.google.api.services.computeaccounts.model.LinuxGetAuthorizedKeysViewResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.zone = com.google.api.client.util.Preconditions.checkNotNull(zone, "Required parameter zone must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ZONE_PATTERN.matcher(zone).matches(),
"Parameter zone must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.user = com.google.api.client.util.Preconditions.checkNotNull(user, "Required parameter user must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public GetAuthorizedKeysView setAlt(java.lang.String alt) {
return (GetAuthorizedKeysView) super.setAlt(alt);
}
@Override
public GetAuthorizedKeysView setFields(java.lang.String fields) {
return (GetAuthorizedKeysView) super.setFields(fields);
}
@Override
public GetAuthorizedKeysView setKey(java.lang.String key) {
return (GetAuthorizedKeysView) super.setKey(key);
}
@Override
public GetAuthorizedKeysView setOauthToken(java.lang.String oauthToken) {
return (GetAuthorizedKeysView) super.setOauthToken(oauthToken);
}
@Override
public GetAuthorizedKeysView setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAuthorizedKeysView) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAuthorizedKeysView setQuotaUser(java.lang.String quotaUser) {
return (GetAuthorizedKeysView) super.setQuotaUser(quotaUser);
}
@Override
public GetAuthorizedKeysView setUserIp(java.lang.String userIp) {
return (GetAuthorizedKeysView) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public GetAuthorizedKeysView setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the zone for this request. */
@com.google.api.client.util.Key
private java.lang.String zone;
/** Name of the zone for this request.
*/
public java.lang.String getZone() {
return zone;
}
/** Name of the zone for this request. */
public GetAuthorizedKeysView setZone(java.lang.String zone) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ZONE_PATTERN.matcher(zone).matches(),
"Parameter zone must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.zone = zone;
return this;
}
/** The user account for which you want to get a list of authorized public keys. */
@com.google.api.client.util.Key
private java.lang.String user;
/** The user account for which you want to get a list of authorized public keys.
*/
public java.lang.String getUser() {
return user;
}
/** The user account for which you want to get a list of authorized public keys. */
public GetAuthorizedKeysView setUser(java.lang.String user) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.user = user;
return this;
}
/** The fully-qualified URL of the virtual machine requesting the view. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** The fully-qualified URL of the virtual machine requesting the view.
*/
public java.lang.String getInstance() {
return instance;
}
/** The fully-qualified URL of the virtual machine requesting the view. */
public GetAuthorizedKeysView setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
@Override
public GetAuthorizedKeysView set(String parameterName, Object value) {
return (GetAuthorizedKeysView) super.set(parameterName, value);
}
}
/**
* Retrieves a list of user accounts for an instance within a specific project.
*
* Create a request for the method "linux.getLinuxAccountViews".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link GetLinuxAccountViews#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param zone Name of the zone for this request.
* @param instance The fully-qualified URL of the virtual machine requesting the views.
* @return the request
*/
public GetLinuxAccountViews getLinuxAccountViews(java.lang.String project, java.lang.String zone, java.lang.String instance) throws java.io.IOException {
GetLinuxAccountViews result = new GetLinuxAccountViews(project, zone, instance);
initialize(result);
return result;
}
public class GetLinuxAccountViews extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/zones/{zone}/linuxAccountViews";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern ZONE_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
private final java.util.regex.Pattern USER_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Retrieves a list of user accounts for an instance within a specific project.
*
* Create a request for the method "linux.getLinuxAccountViews".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link GetLinuxAccountViews#execute()} method to invoke the
* remote operation. {@link GetLinuxAccountViews#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Project ID for this request.
* @param zone Name of the zone for this request.
* @param instance The fully-qualified URL of the virtual machine requesting the views.
* @since 1.13
*/
protected GetLinuxAccountViews(java.lang.String project, java.lang.String zone, java.lang.String instance) {
super(ComputeAccounts.this, "POST", REST_PATH, null, com.google.api.services.computeaccounts.model.LinuxGetLinuxAccountViewsResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.zone = com.google.api.client.util.Preconditions.checkNotNull(zone, "Required parameter zone must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ZONE_PATTERN.matcher(zone).matches(),
"Parameter zone must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.instance = com.google.api.client.util.Preconditions.checkNotNull(instance, "Required parameter instance must be specified.");
}
@Override
public GetLinuxAccountViews setAlt(java.lang.String alt) {
return (GetLinuxAccountViews) super.setAlt(alt);
}
@Override
public GetLinuxAccountViews setFields(java.lang.String fields) {
return (GetLinuxAccountViews) super.setFields(fields);
}
@Override
public GetLinuxAccountViews setKey(java.lang.String key) {
return (GetLinuxAccountViews) super.setKey(key);
}
@Override
public GetLinuxAccountViews setOauthToken(java.lang.String oauthToken) {
return (GetLinuxAccountViews) super.setOauthToken(oauthToken);
}
@Override
public GetLinuxAccountViews setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetLinuxAccountViews) super.setPrettyPrint(prettyPrint);
}
@Override
public GetLinuxAccountViews setQuotaUser(java.lang.String quotaUser) {
return (GetLinuxAccountViews) super.setQuotaUser(quotaUser);
}
@Override
public GetLinuxAccountViews setUserIp(java.lang.String userIp) {
return (GetLinuxAccountViews) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public GetLinuxAccountViews setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the zone for this request. */
@com.google.api.client.util.Key
private java.lang.String zone;
/** Name of the zone for this request.
*/
public java.lang.String getZone() {
return zone;
}
/** Name of the zone for this request. */
public GetLinuxAccountViews setZone(java.lang.String zone) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ZONE_PATTERN.matcher(zone).matches(),
"Parameter zone must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.zone = zone;
return this;
}
/** The fully-qualified URL of the virtual machine requesting the views. */
@com.google.api.client.util.Key
private java.lang.String instance;
/** The fully-qualified URL of the virtual machine requesting the views.
*/
public java.lang.String getInstance() {
return instance;
}
/** The fully-qualified URL of the virtual machine requesting the views. */
public GetLinuxAccountViews setInstance(java.lang.String instance) {
this.instance = instance;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name.
You can also sort results in descending order based on the creation timestamp using
orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field in
reverse chronological order (newest result first). Use this to sort resources like operations so
that the newest operation is returned first.
Currently, only sorting by name or creationTimestamp desc is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
public GetLinuxAccountViews setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Use this parameter if you want to list the next page of results. Set
pageToken to the nextPageToken returned by a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
public GetLinuxAccountViews setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum count of results to be returned. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum count of results to be returned. [default: 500] [minimum: 0] [maximum: 500]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum count of results to be returned. */
public GetLinuxAccountViews setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Sets a filter expression for filtering listed resources, in the form filter={expression}. Your
{expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
- FIELD_NAME: The name of the field you want to compare. The field name must be valid for the type
of resource being filtered. Only atomic field types are supported (string, number, boolean). Array
and object fields are not currently supported. - COMPARISON_STRING: The comparison string, either
eq (equals) or ne (not equals). - LITERAL_STRING: The literal string value to filter to. The
literal value must be valid for the type of field (string, number, boolean). For string fields, the
literal value is interpreted as a regular expression using RE2 syntax. The literal value must match
the entire field. For example, you can filter by the name of a resource: filter=name ne example-
instance The above filter returns only results whose name field does not equal example-instance.
You can also enclose your literal string in single, double, or no quotes.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
public GetLinuxAccountViews setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* If provided, the user requesting the views. If left blank, the system is requesting the
* views, instead of a particular user.
*/
@com.google.api.client.util.Key
private java.lang.String user;
/** If provided, the user requesting the views. If left blank, the system is requesting the views,
instead of a particular user.
*/
public java.lang.String getUser() {
return user;
}
/**
* If provided, the user requesting the views. If left blank, the system is requesting the
* views, instead of a particular user.
*/
public GetLinuxAccountViews setUser(java.lang.String user) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.user = user;
return this;
}
@Override
public GetLinuxAccountViews set(String parameterName, Object value) {
return (GetLinuxAccountViews) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code ComputeAccounts computeaccounts = new ComputeAccounts(...);}
* {@code ComputeAccounts.Users.List request = computeaccounts.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* Adds a public key to the specified User resource with the data included in the request.
*
* Create a request for the method "users.addPublicKey".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link AddPublicKey#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param user Name of the user for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.PublicKey}
* @return the request
*/
public AddPublicKey addPublicKey(java.lang.String project, java.lang.String user, com.google.api.services.computeaccounts.model.PublicKey content) throws java.io.IOException {
AddPublicKey result = new AddPublicKey(project, user, content);
initialize(result);
return result;
}
public class AddPublicKey extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/users/{user}/addPublicKey";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern USER_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Adds a public key to the specified User resource with the data included in the request.
*
* Create a request for the method "users.addPublicKey".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link AddPublicKey#execute()} method to invoke the remote
* operation. {@link
* AddPublicKey#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param user Name of the user for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.PublicKey}
* @since 1.13
*/
protected AddPublicKey(java.lang.String project, java.lang.String user, com.google.api.services.computeaccounts.model.PublicKey content) {
super(ComputeAccounts.this, "POST", REST_PATH, content, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.user = com.google.api.client.util.Preconditions.checkNotNull(user, "Required parameter user must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
}
@Override
public AddPublicKey setAlt(java.lang.String alt) {
return (AddPublicKey) super.setAlt(alt);
}
@Override
public AddPublicKey setFields(java.lang.String fields) {
return (AddPublicKey) super.setFields(fields);
}
@Override
public AddPublicKey setKey(java.lang.String key) {
return (AddPublicKey) super.setKey(key);
}
@Override
public AddPublicKey setOauthToken(java.lang.String oauthToken) {
return (AddPublicKey) super.setOauthToken(oauthToken);
}
@Override
public AddPublicKey setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddPublicKey) super.setPrettyPrint(prettyPrint);
}
@Override
public AddPublicKey setQuotaUser(java.lang.String quotaUser) {
return (AddPublicKey) super.setQuotaUser(quotaUser);
}
@Override
public AddPublicKey setUserIp(java.lang.String userIp) {
return (AddPublicKey) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public AddPublicKey setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the user for this request. */
@com.google.api.client.util.Key
private java.lang.String user;
/** Name of the user for this request.
*/
public java.lang.String getUser() {
return user;
}
/** Name of the user for this request. */
public AddPublicKey setUser(java.lang.String user) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.user = user;
return this;
}
@Override
public AddPublicKey set(String parameterName, Object value) {
return (AddPublicKey) super.set(parameterName, value);
}
}
/**
* Deletes the specified User resource.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param user Name of the user resource to delete.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String user) throws java.io.IOException {
Delete result = new Delete(project, user);
initialize(result);
return result;
}
public class Delete extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/users/{user}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern USER_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Deletes the specified User resource.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param user Name of the user resource to delete.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String user) {
super(ComputeAccounts.this, "DELETE", REST_PATH, null, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.user = com.google.api.client.util.Preconditions.checkNotNull(user, "Required parameter user must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Delete setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the user resource to delete. */
@com.google.api.client.util.Key
private java.lang.String user;
/** Name of the user resource to delete.
*/
public java.lang.String getUser() {
return user;
}
/** Name of the user resource to delete. */
public Delete setUser(java.lang.String user) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.user = user;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns the specified User resource.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param user Name of the user resource to return.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String user) throws java.io.IOException {
Get result = new Get(project, user);
initialize(result);
return result;
}
public class Get extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/users/{user}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern USER_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
/**
* Returns the specified User resource.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param user Name of the user resource to return.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String user) {
super(ComputeAccounts.this, "GET", REST_PATH, null, com.google.api.services.computeaccounts.model.User.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.user = com.google.api.client.util.Preconditions.checkNotNull(user, "Required parameter user must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Get setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the user resource to return. */
@com.google.api.client.util.Key
private java.lang.String user;
/** Name of the user resource to return.
*/
public java.lang.String getUser() {
return user;
}
/** Name of the user resource to return. */
public Get setUser(java.lang.String user) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.user = user;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a User resource in the specified project using the data included in the request.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.User}
* @return the request
*/
public Insert insert(java.lang.String project, com.google.api.services.computeaccounts.model.User content) throws java.io.IOException {
Insert result = new Insert(project, content);
initialize(result);
return result;
}
public class Insert extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/users";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Creates a User resource in the specified project using the data included in the request.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param content the {@link com.google.api.services.computeaccounts.model.User}
* @since 1.13
*/
protected Insert(java.lang.String project, com.google.api.services.computeaccounts.model.User content) {
super(ComputeAccounts.this, "POST", REST_PATH, content, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public Insert setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of users contained within the specified project.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project Project ID for this request.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/users";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Retrieves a list of users contained within the specified project.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @since 1.13
*/
protected List(java.lang.String project) {
super(ComputeAccounts.this, "GET", REST_PATH, null, com.google.api.services.computeaccounts.model.UserList.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Sets a filter expression for filtering listed resources, in the form filter={expression}. Your
{expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
- FIELD_NAME: The name of the field you want to compare. The field name must be valid for the type
of resource being filtered. Only atomic field types are supported (string, number, boolean). Array
and object fields are not currently supported. - COMPARISON_STRING: The comparison string, either
eq (equals) or ne (not equals). - LITERAL_STRING: The literal string value to filter to. The
literal value must be valid for the type of field (string, number, boolean). For string fields, the
literal value is interpreted as a regular expression using RE2 syntax. The literal value must match
the entire field. For example, you can filter by the name of a resource: filter=name ne example-
instance The above filter returns only results whose name field does not equal example-instance.
You can also enclose your literal string in single, double, or no quotes.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Sets a filter expression for filtering listed resources, in the form filter={expression}.
* Your {expression} must contain the following: FIELD_NAME COMPARISON_STRING LITERAL_STRING
*
* - FIELD_NAME: The name of the field you want to compare. The field name must be valid for
* the type of resource being filtered. Only atomic field types are supported (string, number,
* boolean). Array and object fields are not currently supported. - COMPARISON_STRING: The
* comparison string, either eq (equals) or ne (not equals). - LITERAL_STRING: The literal
* string value to filter to. The literal value must be valid for the type of field (string,
* number, boolean). For string fields, the literal value is interpreted as a regular
* expression using RE2 syntax. The literal value must match the entire field. For example,
* you can filter by the name of a resource: filter=name ne example-instance The above filter
* returns only results whose name field does not equal example-instance. You can also enclose
* your literal string in single, double, or no quotes.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name.
You can also sort results in descending order based on the creation timestamp using
orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field in
reverse chronological order (newest result first). Use this to sort resources like operations so
that the newest operation is returned first.
Currently, only sorting by name or creationTimestamp desc is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name.
*
* You can also sort results in descending order based on the creation timestamp using
* orderBy="creationTimestamp desc". This sorts results based on the creationTimestamp field
* in reverse chronological order (newest result first). Use this to sort resources like
* operations so that the newest operation is returned first.
*
* Currently, only sorting by name or creationTimestamp desc is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** Maximum count of results to be returned. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum count of results to be returned. [default: 500] [minimum: 0] [maximum: 500]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum count of results to be returned. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Use this parameter if you want to list the next page of results. Set
pageToken to the nextPageToken returned by a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Use this parameter if you want to list the next page of
* results. Set pageToken to the nextPageToken returned by a previous list request.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Removes the specified public key from the user.
*
* Create a request for the method "users.removePublicKey".
*
* This request holds the parameters needed by the computeaccounts server. After setting any
* optional parameters, call the {@link RemovePublicKey#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param user Name of the user for this request.
* @param fingerprint The fingerprint of the public key to delete. Public keys are identified by their fingerprint, which
* is defined by RFC4716 to be the MD5 digest of the public key.
* @return the request
*/
public RemovePublicKey removePublicKey(java.lang.String project, java.lang.String user, java.lang.String fingerprint) throws java.io.IOException {
RemovePublicKey result = new RemovePublicKey(project, user, fingerprint);
initialize(result);
return result;
}
public class RemovePublicKey extends ComputeAccountsRequest {
private static final String REST_PATH = "{project}/global/users/{user}/removePublicKey";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern USER_PATTERN =
java.util.regex.Pattern.compile("[a-z][-a-z0-9_]{0,31}");
private final java.util.regex.Pattern FINGERPRINT_PATTERN =
java.util.regex.Pattern.compile("[a-f0-9]{32}");
/**
* Removes the specified public key from the user.
*
* Create a request for the method "users.removePublicKey".
*
* This request holds the parameters needed by the the computeaccounts server. After setting any
* optional parameters, call the {@link RemovePublicKey#execute()} method to invoke the remote
* operation. {@link RemovePublicKey#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project Project ID for this request.
* @param user Name of the user for this request.
* @param fingerprint The fingerprint of the public key to delete. Public keys are identified by their fingerprint, which
* is defined by RFC4716 to be the MD5 digest of the public key.
* @since 1.13
*/
protected RemovePublicKey(java.lang.String project, java.lang.String user, java.lang.String fingerprint) {
super(ComputeAccounts.this, "POST", REST_PATH, null, com.google.api.services.computeaccounts.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.user = com.google.api.client.util.Preconditions.checkNotNull(user, "Required parameter user must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.fingerprint = com.google.api.client.util.Preconditions.checkNotNull(fingerprint, "Required parameter fingerprint must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FINGERPRINT_PATTERN.matcher(fingerprint).matches(),
"Parameter fingerprint must conform to the pattern " +
"[a-f0-9]{32}");
}
}
@Override
public RemovePublicKey setAlt(java.lang.String alt) {
return (RemovePublicKey) super.setAlt(alt);
}
@Override
public RemovePublicKey setFields(java.lang.String fields) {
return (RemovePublicKey) super.setFields(fields);
}
@Override
public RemovePublicKey setKey(java.lang.String key) {
return (RemovePublicKey) super.setKey(key);
}
@Override
public RemovePublicKey setOauthToken(java.lang.String oauthToken) {
return (RemovePublicKey) super.setOauthToken(oauthToken);
}
@Override
public RemovePublicKey setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemovePublicKey) super.setPrettyPrint(prettyPrint);
}
@Override
public RemovePublicKey setQuotaUser(java.lang.String quotaUser) {
return (RemovePublicKey) super.setQuotaUser(quotaUser);
}
@Override
public RemovePublicKey setUserIp(java.lang.String userIp) {
return (RemovePublicKey) super.setUserIp(userIp);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public RemovePublicKey setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name of the user for this request. */
@com.google.api.client.util.Key
private java.lang.String user;
/** Name of the user for this request.
*/
public java.lang.String getUser() {
return user;
}
/** Name of the user for this request. */
public RemovePublicKey setUser(java.lang.String user) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(USER_PATTERN.matcher(user).matches(),
"Parameter user must conform to the pattern " +
"[a-z][-a-z0-9_]{0,31}");
}
this.user = user;
return this;
}
/**
* The fingerprint of the public key to delete. Public keys are identified by their
* fingerprint, which is defined by RFC4716 to be the MD5 digest of the public key.
*/
@com.google.api.client.util.Key
private java.lang.String fingerprint;
/** The fingerprint of the public key to delete. Public keys are identified by their fingerprint, which
is defined by RFC4716 to be the MD5 digest of the public key.
*/
public java.lang.String getFingerprint() {
return fingerprint;
}
/**
* The fingerprint of the public key to delete. Public keys are identified by their
* fingerprint, which is defined by RFC4716 to be the MD5 digest of the public key.
*/
public RemovePublicKey setFingerprint(java.lang.String fingerprint) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FINGERPRINT_PATTERN.matcher(fingerprint).matches(),
"Parameter fingerprint must conform to the pattern " +
"[a-f0-9]{32}");
}
this.fingerprint = fingerprint;
return this;
}
@Override
public RemovePublicKey set(String parameterName, Object value) {
return (RemovePublicKey) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link ComputeAccounts}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link ComputeAccounts}. */
@Override
public ComputeAccounts build() {
return new ComputeAccounts(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link ComputeAccountsRequestInitializer}.
*
* @since 1.12
*/
public Builder setComputeAccountsRequestInitializer(
ComputeAccountsRequestInitializer computeaccountsRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(computeaccountsRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}