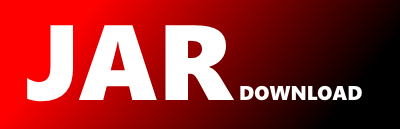
com.google.api.services.computeaccounts.model.Operation Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-08-03 17:34:38 UTC)
* on 2015-09-07 at 15:02:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.computeaccounts.model;
/**
* An Operation resource, used to manage asynchronous API requests.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Compute Accounts API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Operation extends com.google.api.client.json.GenericJson {
/**
* [Output Only] An optional identifier specified by the client when the mutation was initiated.
* Must be unique for all Operation resources in the project.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clientOperationId;
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creationTimestamp;
/**
* [Output Only] The time that this operation was completed. This is in RFC3339 text format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String endTime;
/**
* [Output Only] If errors are generated during processing of the operation, this field will be
* populated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Error error;
/**
* [Output Only] If the operation fails, this field contains the HTTP error message that was
* returned, such as NOT FOUND.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String httpErrorMessage;
/**
* [Output Only] If the operation fails, this field contains the HTTP error message that was
* returned, such as 404.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer httpErrorStatusCode;
/**
* [Output Only] Unique identifier for the resource; defined by the server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger id;
/**
* [Output Only] The time that this operation was requested. This is in RFC3339 text format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String insertTime;
/**
* [Output Only] Type of the resource. Always compute#Operation for Operation resources.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* [Output Only] Name of the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* [Output Only] Type of the operation, such as insert, update, and delete.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String operationType;
/**
* [Output Only] An optional progress indicator that ranges from 0 to 100. There is no requirement
* that this be linear or support any granularity of operations. This should not be used to guess
* at when the operation will be complete. This number should monotonically increase as the
* operation progresses.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer progress;
/**
* [Output Only] URL of the region where the operation resides. Only applicable for regional
* resources.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String region;
/**
* [Output Only] Server defined URL for the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* [Output Only] The time that this operation was started by the server. This is in RFC3339 text
* format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String startTime;
/**
* [Output Only] Status of the operation. Can be one of the following: PENDING, RUNNING, or DONE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* [Output Only] An optional textual description of the current status of the operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String statusMessage;
/**
* [Output Only] Unique target ID which identifies a particular incarnation of the target.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger targetId;
/**
* [Output Only] URL of the resource the operation is mutating.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String targetLink;
/**
* [Output Only] User who requested the operation, for example: [email protected].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String user;
/**
* [Output Only] If warning messages are generated during processing of the operation, this field
* will be populated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List warnings;
static {
// hack to force ProGuard to consider Warnings used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Warnings.class);
}
/**
* [Output Only] URL of the zone where the operation resides.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String zone;
/**
* [Output Only] An optional identifier specified by the client when the mutation was initiated.
* Must be unique for all Operation resources in the project.
* @return value or {@code null} for none
*/
public java.lang.String getClientOperationId() {
return clientOperationId;
}
/**
* [Output Only] An optional identifier specified by the client when the mutation was initiated.
* Must be unique for all Operation resources in the project.
* @param clientOperationId clientOperationId or {@code null} for none
*/
public Operation setClientOperationId(java.lang.String clientOperationId) {
this.clientOperationId = clientOperationId;
return this;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @return value or {@code null} for none
*/
public java.lang.String getCreationTimestamp() {
return creationTimestamp;
}
/**
* [Output Only] Creation timestamp in RFC3339 text format.
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public Operation setCreationTimestamp(java.lang.String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* [Output Only] The time that this operation was completed. This is in RFC3339 text format.
* @return value or {@code null} for none
*/
public java.lang.String getEndTime() {
return endTime;
}
/**
* [Output Only] The time that this operation was completed. This is in RFC3339 text format.
* @param endTime endTime or {@code null} for none
*/
public Operation setEndTime(java.lang.String endTime) {
this.endTime = endTime;
return this;
}
/**
* [Output Only] If errors are generated during processing of the operation, this field will be
* populated.
* @return value or {@code null} for none
*/
public Error getError() {
return error;
}
/**
* [Output Only] If errors are generated during processing of the operation, this field will be
* populated.
* @param error error or {@code null} for none
*/
public Operation setError(Error error) {
this.error = error;
return this;
}
/**
* [Output Only] If the operation fails, this field contains the HTTP error message that was
* returned, such as NOT FOUND.
* @return value or {@code null} for none
*/
public java.lang.String getHttpErrorMessage() {
return httpErrorMessage;
}
/**
* [Output Only] If the operation fails, this field contains the HTTP error message that was
* returned, such as NOT FOUND.
* @param httpErrorMessage httpErrorMessage or {@code null} for none
*/
public Operation setHttpErrorMessage(java.lang.String httpErrorMessage) {
this.httpErrorMessage = httpErrorMessage;
return this;
}
/**
* [Output Only] If the operation fails, this field contains the HTTP error message that was
* returned, such as 404.
* @return value or {@code null} for none
*/
public java.lang.Integer getHttpErrorStatusCode() {
return httpErrorStatusCode;
}
/**
* [Output Only] If the operation fails, this field contains the HTTP error message that was
* returned, such as 404.
* @param httpErrorStatusCode httpErrorStatusCode or {@code null} for none
*/
public Operation setHttpErrorStatusCode(java.lang.Integer httpErrorStatusCode) {
this.httpErrorStatusCode = httpErrorStatusCode;
return this;
}
/**
* [Output Only] Unique identifier for the resource; defined by the server.
* @return value or {@code null} for none
*/
public java.math.BigInteger getId() {
return id;
}
/**
* [Output Only] Unique identifier for the resource; defined by the server.
* @param id id or {@code null} for none
*/
public Operation setId(java.math.BigInteger id) {
this.id = id;
return this;
}
/**
* [Output Only] The time that this operation was requested. This is in RFC3339 text format.
* @return value or {@code null} for none
*/
public java.lang.String getInsertTime() {
return insertTime;
}
/**
* [Output Only] The time that this operation was requested. This is in RFC3339 text format.
* @param insertTime insertTime or {@code null} for none
*/
public Operation setInsertTime(java.lang.String insertTime) {
this.insertTime = insertTime;
return this;
}
/**
* [Output Only] Type of the resource. Always compute#Operation for Operation resources.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* [Output Only] Type of the resource. Always compute#Operation for Operation resources.
* @param kind kind or {@code null} for none
*/
public Operation setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* [Output Only] Name of the resource.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* [Output Only] Name of the resource.
* @param name name or {@code null} for none
*/
public Operation setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* [Output Only] Type of the operation, such as insert, update, and delete.
* @return value or {@code null} for none
*/
public java.lang.String getOperationType() {
return operationType;
}
/**
* [Output Only] Type of the operation, such as insert, update, and delete.
* @param operationType operationType or {@code null} for none
*/
public Operation setOperationType(java.lang.String operationType) {
this.operationType = operationType;
return this;
}
/**
* [Output Only] An optional progress indicator that ranges from 0 to 100. There is no requirement
* that this be linear or support any granularity of operations. This should not be used to guess
* at when the operation will be complete. This number should monotonically increase as the
* operation progresses.
* @return value or {@code null} for none
*/
public java.lang.Integer getProgress() {
return progress;
}
/**
* [Output Only] An optional progress indicator that ranges from 0 to 100. There is no requirement
* that this be linear or support any granularity of operations. This should not be used to guess
* at when the operation will be complete. This number should monotonically increase as the
* operation progresses.
* @param progress progress or {@code null} for none
*/
public Operation setProgress(java.lang.Integer progress) {
this.progress = progress;
return this;
}
/**
* [Output Only] URL of the region where the operation resides. Only applicable for regional
* resources.
* @return value or {@code null} for none
*/
public java.lang.String getRegion() {
return region;
}
/**
* [Output Only] URL of the region where the operation resides. Only applicable for regional
* resources.
* @param region region or {@code null} for none
*/
public Operation setRegion(java.lang.String region) {
this.region = region;
return this;
}
/**
* [Output Only] Server defined URL for the resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* [Output Only] Server defined URL for the resource.
* @param selfLink selfLink or {@code null} for none
*/
public Operation setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* [Output Only] The time that this operation was started by the server. This is in RFC3339 text
* format.
* @return value or {@code null} for none
*/
public java.lang.String getStartTime() {
return startTime;
}
/**
* [Output Only] The time that this operation was started by the server. This is in RFC3339 text
* format.
* @param startTime startTime or {@code null} for none
*/
public Operation setStartTime(java.lang.String startTime) {
this.startTime = startTime;
return this;
}
/**
* [Output Only] Status of the operation. Can be one of the following: PENDING, RUNNING, or DONE.
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* [Output Only] Status of the operation. Can be one of the following: PENDING, RUNNING, or DONE.
* @param status status or {@code null} for none
*/
public Operation setStatus(java.lang.String status) {
this.status = status;
return this;
}
/**
* [Output Only] An optional textual description of the current status of the operation.
* @return value or {@code null} for none
*/
public java.lang.String getStatusMessage() {
return statusMessage;
}
/**
* [Output Only] An optional textual description of the current status of the operation.
* @param statusMessage statusMessage or {@code null} for none
*/
public Operation setStatusMessage(java.lang.String statusMessage) {
this.statusMessage = statusMessage;
return this;
}
/**
* [Output Only] Unique target ID which identifies a particular incarnation of the target.
* @return value or {@code null} for none
*/
public java.math.BigInteger getTargetId() {
return targetId;
}
/**
* [Output Only] Unique target ID which identifies a particular incarnation of the target.
* @param targetId targetId or {@code null} for none
*/
public Operation setTargetId(java.math.BigInteger targetId) {
this.targetId = targetId;
return this;
}
/**
* [Output Only] URL of the resource the operation is mutating.
* @return value or {@code null} for none
*/
public java.lang.String getTargetLink() {
return targetLink;
}
/**
* [Output Only] URL of the resource the operation is mutating.
* @param targetLink targetLink or {@code null} for none
*/
public Operation setTargetLink(java.lang.String targetLink) {
this.targetLink = targetLink;
return this;
}
/**
* [Output Only] User who requested the operation, for example: [email protected].
* @return value or {@code null} for none
*/
public java.lang.String getUser() {
return user;
}
/**
* [Output Only] User who requested the operation, for example: [email protected].
* @param user user or {@code null} for none
*/
public Operation setUser(java.lang.String user) {
this.user = user;
return this;
}
/**
* [Output Only] If warning messages are generated during processing of the operation, this field
* will be populated.
* @return value or {@code null} for none
*/
public java.util.List getWarnings() {
return warnings;
}
/**
* [Output Only] If warning messages are generated during processing of the operation, this field
* will be populated.
* @param warnings warnings or {@code null} for none
*/
public Operation setWarnings(java.util.List warnings) {
this.warnings = warnings;
return this;
}
/**
* [Output Only] URL of the zone where the operation resides.
* @return value or {@code null} for none
*/
public java.lang.String getZone() {
return zone;
}
/**
* [Output Only] URL of the zone where the operation resides.
* @param zone zone or {@code null} for none
*/
public Operation setZone(java.lang.String zone) {
this.zone = zone;
return this;
}
@Override
public Operation set(String fieldName, Object value) {
return (Operation) super.set(fieldName, value);
}
@Override
public Operation clone() {
return (Operation) super.clone();
}
/**
* [Output Only] If errors are generated during processing of the operation, this field will be
* populated.
*/
public static final class Error extends com.google.api.client.json.GenericJson {
/**
* [Output Only] The array of errors encountered while processing this operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List errors;
static {
// hack to force ProGuard to consider Errors used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Errors.class);
}
/**
* [Output Only] The array of errors encountered while processing this operation.
* @return value or {@code null} for none
*/
public java.util.List getErrors() {
return errors;
}
/**
* [Output Only] The array of errors encountered while processing this operation.
* @param errors errors or {@code null} for none
*/
public Error setErrors(java.util.List errors) {
this.errors = errors;
return this;
}
@Override
public Error set(String fieldName, Object value) {
return (Error) super.set(fieldName, value);
}
@Override
public Error clone() {
return (Error) super.clone();
}
/**
* Model definition for OperationErrorErrors.
*/
public static final class Errors extends com.google.api.client.json.GenericJson {
/**
* [Output Only] The error type identifier for this error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String code;
/**
* [Output Only] Indicates the field in the request which caused the error. This property is
* optional.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String location;
/**
* [Output Only] An optional, human-readable error message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String message;
/**
* [Output Only] The error type identifier for this error.
* @return value or {@code null} for none
*/
public java.lang.String getCode() {
return code;
}
/**
* [Output Only] The error type identifier for this error.
* @param code code or {@code null} for none
*/
public Errors setCode(java.lang.String code) {
this.code = code;
return this;
}
/**
* [Output Only] Indicates the field in the request which caused the error. This property is
* optional.
* @return value or {@code null} for none
*/
public java.lang.String getLocation() {
return location;
}
/**
* [Output Only] Indicates the field in the request which caused the error. This property is
* optional.
* @param location location or {@code null} for none
*/
public Errors setLocation(java.lang.String location) {
this.location = location;
return this;
}
/**
* [Output Only] An optional, human-readable error message.
* @return value or {@code null} for none
*/
public java.lang.String getMessage() {
return message;
}
/**
* [Output Only] An optional, human-readable error message.
* @param message message or {@code null} for none
*/
public Errors setMessage(java.lang.String message) {
this.message = message;
return this;
}
@Override
public Errors set(String fieldName, Object value) {
return (Errors) super.set(fieldName, value);
}
@Override
public Errors clone() {
return (Errors) super.clone();
}
}
}
/**
* Model definition for OperationWarnings.
*/
public static final class Warnings extends com.google.api.client.json.GenericJson {
/**
* [Output Only] The warning type identifier for this warning.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String code;
/**
* [Output Only] Metadata for this warning in key: value format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List data;
static {
// hack to force ProGuard to consider Data used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Data.class);
}
/**
* [Output Only] Optional human-readable details for this warning.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String message;
/**
* [Output Only] The warning type identifier for this warning.
* @return value or {@code null} for none
*/
public java.lang.String getCode() {
return code;
}
/**
* [Output Only] The warning type identifier for this warning.
* @param code code or {@code null} for none
*/
public Warnings setCode(java.lang.String code) {
this.code = code;
return this;
}
/**
* [Output Only] Metadata for this warning in key: value format.
* @return value or {@code null} for none
*/
public java.util.List getData() {
return data;
}
/**
* [Output Only] Metadata for this warning in key: value format.
* @param data data or {@code null} for none
*/
public Warnings setData(java.util.List data) {
this.data = data;
return this;
}
/**
* [Output Only] Optional human-readable details for this warning.
* @return value or {@code null} for none
*/
public java.lang.String getMessage() {
return message;
}
/**
* [Output Only] Optional human-readable details for this warning.
* @param message message or {@code null} for none
*/
public Warnings setMessage(java.lang.String message) {
this.message = message;
return this;
}
@Override
public Warnings set(String fieldName, Object value) {
return (Warnings) super.set(fieldName, value);
}
@Override
public Warnings clone() {
return (Warnings) super.clone();
}
/**
* Model definition for OperationWarningsData.
*/
public static final class Data extends com.google.api.client.json.GenericJson {
/**
* [Output Only] A key for the warning data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String key;
/**
* [Output Only] A warning data value corresponding to the key.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* [Output Only] A key for the warning data.
* @return value or {@code null} for none
*/
public java.lang.String getKey() {
return key;
}
/**
* [Output Only] A key for the warning data.
* @param key key or {@code null} for none
*/
public Data setKey(java.lang.String key) {
this.key = key;
return this;
}
/**
* [Output Only] A warning data value corresponding to the key.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* [Output Only] A warning data value corresponding to the key.
* @param value value or {@code null} for none
*/
public Data setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public Data set(String fieldName, Object value) {
return (Data) super.set(fieldName, value);
}
@Override
public Data clone() {
return (Data) super.clone();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy