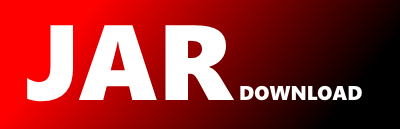
com.google.api.services.consumersurveys.model.SurveyQuestion Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2016-10-17 16:43:55 UTC)
* on 2016-11-16 at 05:24:47 UTC
* Modify at your own risk.
*/
package com.google.api.services.consumersurveys.model;
/**
* Model definition for SurveyQuestion.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Consumer Surveys API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SurveyQuestion extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String answerOrder;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List answers;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasOther;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String highValueLabel;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List images;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean lastAnswerPositionPinned;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lowValueLabel;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean mustPickSuggestion;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String numStars;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String openTextPlaceholder;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List openTextSuggestions;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String question;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sentimentText;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean singleLineResponse;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List thresholdAnswers;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String unitOfMeasurementLabel;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String videoId;
/**
* @return value or {@code null} for none
*/
public java.lang.String getAnswerOrder() {
return answerOrder;
}
/**
* @param answerOrder answerOrder or {@code null} for none
*/
public SurveyQuestion setAnswerOrder(java.lang.String answerOrder) {
this.answerOrder = answerOrder;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getAnswers() {
return answers;
}
/**
* @param answers answers or {@code null} for none
*/
public SurveyQuestion setAnswers(java.util.List answers) {
this.answers = answers;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasOther() {
return hasOther;
}
/**
* @param hasOther hasOther or {@code null} for none
*/
public SurveyQuestion setHasOther(java.lang.Boolean hasOther) {
this.hasOther = hasOther;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getHighValueLabel() {
return highValueLabel;
}
/**
* @param highValueLabel highValueLabel or {@code null} for none
*/
public SurveyQuestion setHighValueLabel(java.lang.String highValueLabel) {
this.highValueLabel = highValueLabel;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getImages() {
return images;
}
/**
* @param images images or {@code null} for none
*/
public SurveyQuestion setImages(java.util.List images) {
this.images = images;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getLastAnswerPositionPinned() {
return lastAnswerPositionPinned;
}
/**
* @param lastAnswerPositionPinned lastAnswerPositionPinned or {@code null} for none
*/
public SurveyQuestion setLastAnswerPositionPinned(java.lang.Boolean lastAnswerPositionPinned) {
this.lastAnswerPositionPinned = lastAnswerPositionPinned;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getLowValueLabel() {
return lowValueLabel;
}
/**
* @param lowValueLabel lowValueLabel or {@code null} for none
*/
public SurveyQuestion setLowValueLabel(java.lang.String lowValueLabel) {
this.lowValueLabel = lowValueLabel;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getMustPickSuggestion() {
return mustPickSuggestion;
}
/**
* @param mustPickSuggestion mustPickSuggestion or {@code null} for none
*/
public SurveyQuestion setMustPickSuggestion(java.lang.Boolean mustPickSuggestion) {
this.mustPickSuggestion = mustPickSuggestion;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getNumStars() {
return numStars;
}
/**
* @param numStars numStars or {@code null} for none
*/
public SurveyQuestion setNumStars(java.lang.String numStars) {
this.numStars = numStars;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getOpenTextPlaceholder() {
return openTextPlaceholder;
}
/**
* @param openTextPlaceholder openTextPlaceholder or {@code null} for none
*/
public SurveyQuestion setOpenTextPlaceholder(java.lang.String openTextPlaceholder) {
this.openTextPlaceholder = openTextPlaceholder;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getOpenTextSuggestions() {
return openTextSuggestions;
}
/**
* @param openTextSuggestions openTextSuggestions or {@code null} for none
*/
public SurveyQuestion setOpenTextSuggestions(java.util.List openTextSuggestions) {
this.openTextSuggestions = openTextSuggestions;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getQuestion() {
return question;
}
/**
* @param question question or {@code null} for none
*/
public SurveyQuestion setQuestion(java.lang.String question) {
this.question = question;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getSentimentText() {
return sentimentText;
}
/**
* @param sentimentText sentimentText or {@code null} for none
*/
public SurveyQuestion setSentimentText(java.lang.String sentimentText) {
this.sentimentText = sentimentText;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Boolean getSingleLineResponse() {
return singleLineResponse;
}
/**
* @param singleLineResponse singleLineResponse or {@code null} for none
*/
public SurveyQuestion setSingleLineResponse(java.lang.Boolean singleLineResponse) {
this.singleLineResponse = singleLineResponse;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getThresholdAnswers() {
return thresholdAnswers;
}
/**
* @param thresholdAnswers thresholdAnswers or {@code null} for none
*/
public SurveyQuestion setThresholdAnswers(java.util.List thresholdAnswers) {
this.thresholdAnswers = thresholdAnswers;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* @param type type or {@code null} for none
*/
public SurveyQuestion setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getUnitOfMeasurementLabel() {
return unitOfMeasurementLabel;
}
/**
* @param unitOfMeasurementLabel unitOfMeasurementLabel or {@code null} for none
*/
public SurveyQuestion setUnitOfMeasurementLabel(java.lang.String unitOfMeasurementLabel) {
this.unitOfMeasurementLabel = unitOfMeasurementLabel;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getVideoId() {
return videoId;
}
/**
* @param videoId videoId or {@code null} for none
*/
public SurveyQuestion setVideoId(java.lang.String videoId) {
this.videoId = videoId;
return this;
}
@Override
public SurveyQuestion set(String fieldName, Object value) {
return (SurveyQuestion) super.set(fieldName, value);
}
@Override
public SurveyQuestion clone() {
return (SurveyQuestion) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy