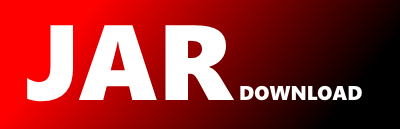
com.google.api.services.contactcenterinsights.v1.model.GoogleCloudContactcenterinsightsV1CalculateStatsResponse Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.contactcenterinsights.v1.model;
/**
* The response for calculating conversation statistics.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Contact Center AI Insights API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudContactcenterinsightsV1CalculateStatsResponse extends com.google.api.client.json.GenericJson {
/**
* The average duration of all conversations. The average is calculated using only conversations
* that have a time duration.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String averageDuration;
/**
* The average number of turns per conversation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer averageTurnCount;
/**
* The total number of conversations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer conversationCount;
/**
* A time series representing the count of conversations created over time that match that
* requested filter criteria.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1CalculateStatsResponseTimeSeries conversationCountTimeSeries;
/**
* A map associating each custom highlighter resource name with its respective number of matches
* in the set of conversations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map customHighlighterMatches;
/**
* A map associating each issue resource name with its respective number of matches in the set of
* conversations. Key has the format: `projects//locations//issueModels//issues/` Deprecated, use
* `issue_matches_stats` field instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map issueMatches;
/**
* A map associating each issue resource name with its respective number of matches in the set of
* conversations. Key has the format: `projects//locations//issueModels//issues/`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map issueMatchesStats;
/**
* A map associating each smart highlighter display name with its respective number of matches in
* the set of conversations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map smartHighlighterMatches;
/**
* The average duration of all conversations. The average is calculated using only conversations
* that have a time duration.
* @return value or {@code null} for none
*/
public String getAverageDuration() {
return averageDuration;
}
/**
* The average duration of all conversations. The average is calculated using only conversations
* that have a time duration.
* @param averageDuration averageDuration or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setAverageDuration(String averageDuration) {
this.averageDuration = averageDuration;
return this;
}
/**
* The average number of turns per conversation.
* @return value or {@code null} for none
*/
public java.lang.Integer getAverageTurnCount() {
return averageTurnCount;
}
/**
* The average number of turns per conversation.
* @param averageTurnCount averageTurnCount or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setAverageTurnCount(java.lang.Integer averageTurnCount) {
this.averageTurnCount = averageTurnCount;
return this;
}
/**
* The total number of conversations.
* @return value or {@code null} for none
*/
public java.lang.Integer getConversationCount() {
return conversationCount;
}
/**
* The total number of conversations.
* @param conversationCount conversationCount or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setConversationCount(java.lang.Integer conversationCount) {
this.conversationCount = conversationCount;
return this;
}
/**
* A time series representing the count of conversations created over time that match that
* requested filter criteria.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponseTimeSeries getConversationCountTimeSeries() {
return conversationCountTimeSeries;
}
/**
* A time series representing the count of conversations created over time that match that
* requested filter criteria.
* @param conversationCountTimeSeries conversationCountTimeSeries or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setConversationCountTimeSeries(GoogleCloudContactcenterinsightsV1CalculateStatsResponseTimeSeries conversationCountTimeSeries) {
this.conversationCountTimeSeries = conversationCountTimeSeries;
return this;
}
/**
* A map associating each custom highlighter resource name with its respective number of matches
* in the set of conversations.
* @return value or {@code null} for none
*/
public java.util.Map getCustomHighlighterMatches() {
return customHighlighterMatches;
}
/**
* A map associating each custom highlighter resource name with its respective number of matches
* in the set of conversations.
* @param customHighlighterMatches customHighlighterMatches or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setCustomHighlighterMatches(java.util.Map customHighlighterMatches) {
this.customHighlighterMatches = customHighlighterMatches;
return this;
}
/**
* A map associating each issue resource name with its respective number of matches in the set of
* conversations. Key has the format: `projects//locations//issueModels//issues/` Deprecated, use
* `issue_matches_stats` field instead.
* @return value or {@code null} for none
*/
public java.util.Map getIssueMatches() {
return issueMatches;
}
/**
* A map associating each issue resource name with its respective number of matches in the set of
* conversations. Key has the format: `projects//locations//issueModels//issues/` Deprecated, use
* `issue_matches_stats` field instead.
* @param issueMatches issueMatches or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setIssueMatches(java.util.Map issueMatches) {
this.issueMatches = issueMatches;
return this;
}
/**
* A map associating each issue resource name with its respective number of matches in the set of
* conversations. Key has the format: `projects//locations//issueModels//issues/`
* @return value or {@code null} for none
*/
public java.util.Map getIssueMatchesStats() {
return issueMatchesStats;
}
/**
* A map associating each issue resource name with its respective number of matches in the set of
* conversations. Key has the format: `projects//locations//issueModels//issues/`
* @param issueMatchesStats issueMatchesStats or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setIssueMatchesStats(java.util.Map issueMatchesStats) {
this.issueMatchesStats = issueMatchesStats;
return this;
}
/**
* A map associating each smart highlighter display name with its respective number of matches in
* the set of conversations.
* @return value or {@code null} for none
*/
public java.util.Map getSmartHighlighterMatches() {
return smartHighlighterMatches;
}
/**
* A map associating each smart highlighter display name with its respective number of matches in
* the set of conversations.
* @param smartHighlighterMatches smartHighlighterMatches or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse setSmartHighlighterMatches(java.util.Map smartHighlighterMatches) {
this.smartHighlighterMatches = smartHighlighterMatches;
return this;
}
@Override
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse set(String fieldName, Object value) {
return (GoogleCloudContactcenterinsightsV1CalculateStatsResponse) super.set(fieldName, value);
}
@Override
public GoogleCloudContactcenterinsightsV1CalculateStatsResponse clone() {
return (GoogleCloudContactcenterinsightsV1CalculateStatsResponse) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy