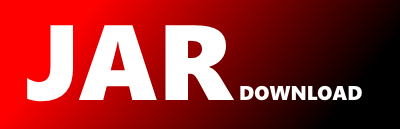
com.google.api.services.contactcenterinsights.v1.model.GoogleCloudContactcenterinsightsV1Conversation Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.contactcenterinsights.v1.model;
/**
* The conversation resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Contact Center AI Insights API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudContactcenterinsightsV1Conversation extends com.google.api.client.json.GenericJson {
/**
* An opaque, user-specified string representing the human agent who handled the conversation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String agentId;
/**
* Call-specific metadata.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationCallMetadata callMetadata;
/**
* Output only. The time at which the conversation was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* The source of the audio and transcription for the conversation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationDataSource dataSource;
/**
* Output only. All the matched Dialogflow intents in the call. The key corresponds to a
* Dialogflow intent, format: projects/{project}/agent/{agent}/intents/{intent}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map dialogflowIntents;
/**
* Output only. The duration of the conversation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String duration;
/**
* The time at which this conversation should expire. After this time, the conversation data and
* any associated analyses will be deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String expireTime;
/**
* A map for the user to specify any custom fields. A maximum of 20 labels per conversation is
* allowed, with a maximum of 256 characters per entry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* A user-specified language code for the conversation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/**
* Output only. The conversation's latest analysis, if one exists.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1Analysis latestAnalysis;
/**
* Output only. Latest summary of the conversation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationSummarizationSuggestionData latestSummary;
/**
* Immutable. The conversation medium, if unspecified will default to PHONE_CALL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String medium;
/**
* Immutable. The resource name of the conversation. Format:
* projects/{project}/locations/{location}/conversations/{conversation}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Obfuscated user ID which the customer sent to us.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String obfuscatedUserId;
/**
* Conversation metadata related to quality management.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationQualityMetadata qualityMetadata;
/**
* Output only. The annotations that were generated during the customer and agent interaction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List runtimeAnnotations;
/**
* The time at which the conversation started.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* Output only. The conversation transcript.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationTranscript transcript;
/**
* Input only. The TTL for this resource. If specified, then this TTL will be used to calculate
* the expire time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String ttl;
/**
* Output only. The number of turns in the conversation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer turnCount;
/**
* Output only. The most recent time at which the conversation was updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* An opaque, user-specified string representing the human agent who handled the conversation.
* @return value or {@code null} for none
*/
public java.lang.String getAgentId() {
return agentId;
}
/**
* An opaque, user-specified string representing the human agent who handled the conversation.
* @param agentId agentId or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setAgentId(java.lang.String agentId) {
this.agentId = agentId;
return this;
}
/**
* Call-specific metadata.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationCallMetadata getCallMetadata() {
return callMetadata;
}
/**
* Call-specific metadata.
* @param callMetadata callMetadata or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setCallMetadata(GoogleCloudContactcenterinsightsV1ConversationCallMetadata callMetadata) {
this.callMetadata = callMetadata;
return this;
}
/**
* Output only. The time at which the conversation was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The time at which the conversation was created.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* The source of the audio and transcription for the conversation.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationDataSource getDataSource() {
return dataSource;
}
/**
* The source of the audio and transcription for the conversation.
* @param dataSource dataSource or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setDataSource(GoogleCloudContactcenterinsightsV1ConversationDataSource dataSource) {
this.dataSource = dataSource;
return this;
}
/**
* Output only. All the matched Dialogflow intents in the call. The key corresponds to a
* Dialogflow intent, format: projects/{project}/agent/{agent}/intents/{intent}
* @return value or {@code null} for none
*/
public java.util.Map getDialogflowIntents() {
return dialogflowIntents;
}
/**
* Output only. All the matched Dialogflow intents in the call. The key corresponds to a
* Dialogflow intent, format: projects/{project}/agent/{agent}/intents/{intent}
* @param dialogflowIntents dialogflowIntents or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setDialogflowIntents(java.util.Map dialogflowIntents) {
this.dialogflowIntents = dialogflowIntents;
return this;
}
/**
* Output only. The duration of the conversation.
* @return value or {@code null} for none
*/
public String getDuration() {
return duration;
}
/**
* Output only. The duration of the conversation.
* @param duration duration or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setDuration(String duration) {
this.duration = duration;
return this;
}
/**
* The time at which this conversation should expire. After this time, the conversation data and
* any associated analyses will be deleted.
* @return value or {@code null} for none
*/
public String getExpireTime() {
return expireTime;
}
/**
* The time at which this conversation should expire. After this time, the conversation data and
* any associated analyses will be deleted.
* @param expireTime expireTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setExpireTime(String expireTime) {
this.expireTime = expireTime;
return this;
}
/**
* A map for the user to specify any custom fields. A maximum of 20 labels per conversation is
* allowed, with a maximum of 256 characters per entry.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* A map for the user to specify any custom fields. A maximum of 20 labels per conversation is
* allowed, with a maximum of 256 characters per entry.
* @param labels labels or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* A user-specified language code for the conversation.
* @return value or {@code null} for none
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* A user-specified language code for the conversation.
* @param languageCode languageCode or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Output only. The conversation's latest analysis, if one exists.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Analysis getLatestAnalysis() {
return latestAnalysis;
}
/**
* Output only. The conversation's latest analysis, if one exists.
* @param latestAnalysis latestAnalysis or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setLatestAnalysis(GoogleCloudContactcenterinsightsV1Analysis latestAnalysis) {
this.latestAnalysis = latestAnalysis;
return this;
}
/**
* Output only. Latest summary of the conversation.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationSummarizationSuggestionData getLatestSummary() {
return latestSummary;
}
/**
* Output only. Latest summary of the conversation.
* @param latestSummary latestSummary or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setLatestSummary(GoogleCloudContactcenterinsightsV1ConversationSummarizationSuggestionData latestSummary) {
this.latestSummary = latestSummary;
return this;
}
/**
* Immutable. The conversation medium, if unspecified will default to PHONE_CALL.
* @return value or {@code null} for none
*/
public java.lang.String getMedium() {
return medium;
}
/**
* Immutable. The conversation medium, if unspecified will default to PHONE_CALL.
* @param medium medium or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setMedium(java.lang.String medium) {
this.medium = medium;
return this;
}
/**
* Immutable. The resource name of the conversation. Format:
* projects/{project}/locations/{location}/conversations/{conversation}
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Immutable. The resource name of the conversation. Format:
* projects/{project}/locations/{location}/conversations/{conversation}
* @param name name or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Obfuscated user ID which the customer sent to us.
* @return value or {@code null} for none
*/
public java.lang.String getObfuscatedUserId() {
return obfuscatedUserId;
}
/**
* Obfuscated user ID which the customer sent to us.
* @param obfuscatedUserId obfuscatedUserId or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setObfuscatedUserId(java.lang.String obfuscatedUserId) {
this.obfuscatedUserId = obfuscatedUserId;
return this;
}
/**
* Conversation metadata related to quality management.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationQualityMetadata getQualityMetadata() {
return qualityMetadata;
}
/**
* Conversation metadata related to quality management.
* @param qualityMetadata qualityMetadata or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setQualityMetadata(GoogleCloudContactcenterinsightsV1ConversationQualityMetadata qualityMetadata) {
this.qualityMetadata = qualityMetadata;
return this;
}
/**
* Output only. The annotations that were generated during the customer and agent interaction.
* @return value or {@code null} for none
*/
public java.util.List getRuntimeAnnotations() {
return runtimeAnnotations;
}
/**
* Output only. The annotations that were generated during the customer and agent interaction.
* @param runtimeAnnotations runtimeAnnotations or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setRuntimeAnnotations(java.util.List runtimeAnnotations) {
this.runtimeAnnotations = runtimeAnnotations;
return this;
}
/**
* The time at which the conversation started.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* The time at which the conversation started.
* @param startTime startTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* Output only. The conversation transcript.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscript getTranscript() {
return transcript;
}
/**
* Output only. The conversation transcript.
* @param transcript transcript or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setTranscript(GoogleCloudContactcenterinsightsV1ConversationTranscript transcript) {
this.transcript = transcript;
return this;
}
/**
* Input only. The TTL for this resource. If specified, then this TTL will be used to calculate
* the expire time.
* @return value or {@code null} for none
*/
public String getTtl() {
return ttl;
}
/**
* Input only. The TTL for this resource. If specified, then this TTL will be used to calculate
* the expire time.
* @param ttl ttl or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setTtl(String ttl) {
this.ttl = ttl;
return this;
}
/**
* Output only. The number of turns in the conversation.
* @return value or {@code null} for none
*/
public java.lang.Integer getTurnCount() {
return turnCount;
}
/**
* Output only. The number of turns in the conversation.
* @param turnCount turnCount or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setTurnCount(java.lang.Integer turnCount) {
this.turnCount = turnCount;
return this;
}
/**
* Output only. The most recent time at which the conversation was updated.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The most recent time at which the conversation was updated.
* @param updateTime updateTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Conversation setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public GoogleCloudContactcenterinsightsV1Conversation set(String fieldName, Object value) {
return (GoogleCloudContactcenterinsightsV1Conversation) super.set(fieldName, value);
}
@Override
public GoogleCloudContactcenterinsightsV1Conversation clone() {
return (GoogleCloudContactcenterinsightsV1Conversation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy