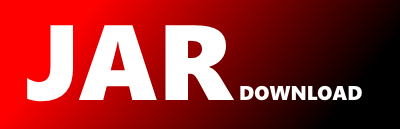
com.google.api.services.contactcenterinsights.v1.model.GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.contactcenterinsights.v1.model;
/**
* A segment of a full transcript.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Contact Center AI Insights API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment extends com.google.api.client.json.GenericJson {
/**
* For conversations derived from multi-channel audio, this is the channel number corresponding to
* the audio from that channel. For audioChannelCount = N, its output values can range from '1' to
* 'N'. A channel tag of 0 indicates that the audio is mono.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer channelTag;
/**
* A confidence estimate between 0.0 and 1.0 of the fidelity of this segment. A default value of
* 0.0 indicates that the value is unset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float confidence;
/**
* CCAI metadata relating to the current transcript segment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegmentDialogflowSegmentMetadata dialogflowSegmentMetadata;
/**
* The language code of this segment as a [BCP-47](https://www.rfc-editor.org/rfc/bcp/bcp47.txt)
* language tag. Example: "en-US".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/**
* The time that the message occurred, if provided.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String messageTime;
/**
* The participant of this segment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationParticipant segmentParticipant;
/**
* The sentiment for this transcript segment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1SentimentData sentiment;
/**
* The text of this segment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String text;
/**
* A list of the word-specific information for each word in the segment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List words;
/**
* For conversations derived from multi-channel audio, this is the channel number corresponding to
* the audio from that channel. For audioChannelCount = N, its output values can range from '1' to
* 'N'. A channel tag of 0 indicates that the audio is mono.
* @return value or {@code null} for none
*/
public java.lang.Integer getChannelTag() {
return channelTag;
}
/**
* For conversations derived from multi-channel audio, this is the channel number corresponding to
* the audio from that channel. For audioChannelCount = N, its output values can range from '1' to
* 'N'. A channel tag of 0 indicates that the audio is mono.
* @param channelTag channelTag or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setChannelTag(java.lang.Integer channelTag) {
this.channelTag = channelTag;
return this;
}
/**
* A confidence estimate between 0.0 and 1.0 of the fidelity of this segment. A default value of
* 0.0 indicates that the value is unset.
* @return value or {@code null} for none
*/
public java.lang.Float getConfidence() {
return confidence;
}
/**
* A confidence estimate between 0.0 and 1.0 of the fidelity of this segment. A default value of
* 0.0 indicates that the value is unset.
* @param confidence confidence or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setConfidence(java.lang.Float confidence) {
this.confidence = confidence;
return this;
}
/**
* CCAI metadata relating to the current transcript segment.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegmentDialogflowSegmentMetadata getDialogflowSegmentMetadata() {
return dialogflowSegmentMetadata;
}
/**
* CCAI metadata relating to the current transcript segment.
* @param dialogflowSegmentMetadata dialogflowSegmentMetadata or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setDialogflowSegmentMetadata(GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegmentDialogflowSegmentMetadata dialogflowSegmentMetadata) {
this.dialogflowSegmentMetadata = dialogflowSegmentMetadata;
return this;
}
/**
* The language code of this segment as a [BCP-47](https://www.rfc-editor.org/rfc/bcp/bcp47.txt)
* language tag. Example: "en-US".
* @return value or {@code null} for none
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language code of this segment as a [BCP-47](https://www.rfc-editor.org/rfc/bcp/bcp47.txt)
* language tag. Example: "en-US".
* @param languageCode languageCode or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The time that the message occurred, if provided.
* @return value or {@code null} for none
*/
public String getMessageTime() {
return messageTime;
}
/**
* The time that the message occurred, if provided.
* @param messageTime messageTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setMessageTime(String messageTime) {
this.messageTime = messageTime;
return this;
}
/**
* The participant of this segment.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationParticipant getSegmentParticipant() {
return segmentParticipant;
}
/**
* The participant of this segment.
* @param segmentParticipant segmentParticipant or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setSegmentParticipant(GoogleCloudContactcenterinsightsV1ConversationParticipant segmentParticipant) {
this.segmentParticipant = segmentParticipant;
return this;
}
/**
* The sentiment for this transcript segment.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1SentimentData getSentiment() {
return sentiment;
}
/**
* The sentiment for this transcript segment.
* @param sentiment sentiment or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setSentiment(GoogleCloudContactcenterinsightsV1SentimentData sentiment) {
this.sentiment = sentiment;
return this;
}
/**
* The text of this segment.
* @return value or {@code null} for none
*/
public java.lang.String getText() {
return text;
}
/**
* The text of this segment.
* @param text text or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setText(java.lang.String text) {
this.text = text;
return this;
}
/**
* A list of the word-specific information for each word in the segment.
* @return value or {@code null} for none
*/
public java.util.List getWords() {
return words;
}
/**
* A list of the word-specific information for each word in the segment.
* @param words words or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment setWords(java.util.List words) {
this.words = words;
return this;
}
@Override
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment set(String fieldName, Object value) {
return (GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment) super.set(fieldName, value);
}
@Override
public GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment clone() {
return (GoogleCloudContactcenterinsightsV1ConversationTranscriptTranscriptSegment) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy