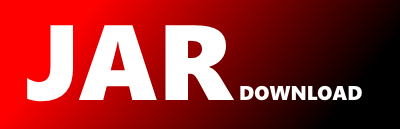
com.google.api.services.contactcenterinsights.v1.model.GoogleCloudContactcenterinsightsV1RuntimeAnnotation Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.contactcenterinsights.v1.model;
/**
* An annotation that was generated during the customer and agent interaction.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Contact Center AI Insights API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudContactcenterinsightsV1RuntimeAnnotation extends com.google.api.client.json.GenericJson {
/**
* The unique identifier of the annotation. Format: projects/{project}/locations/{location}/conver
* sationDatasets/{dataset}/conversationDataItems/{data_item}/conversationAnnotations/{annotation}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String annotationId;
/**
* The feedback that the customer has about the answer in `data`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1AnswerFeedback answerFeedback;
/**
* Agent Assist Article Suggestion data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ArticleSuggestionData articleSuggestion;
/**
* Conversation summarization suggestion data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1ConversationSummarizationSuggestionData conversationSummarizationSuggestion;
/**
* The time at which this annotation was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Dialogflow interaction data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1DialogflowInteractionData dialogflowInteraction;
/**
* The boundary in the conversation where the annotation ends, inclusive.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1AnnotationBoundary endBoundary;
/**
* Agent Assist FAQ answer data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1FaqAnswerData faqAnswer;
/**
* The generator suggestion result.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1GetGeneratorSuggestionResponse generatorSuggestionResult;
/**
* The Knowledge Assist result.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1GetKnowledgeAssistResponse knowledgeAssistResult;
/**
* The Knowledge Search result.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1SearchKnowledgeAnswer knowledgeSearchResult;
/**
* Agent Assist Smart Compose suggestion data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1SmartComposeSuggestionData smartComposeSuggestion;
/**
* Agent Assist Smart Reply data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1SmartReplyData smartReply;
/**
* The boundary in the conversation where the annotation starts, inclusive.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1AnnotationBoundary startBoundary;
/**
* The unique identifier of the annotation. Format: projects/{project}/locations/{location}/conver
* sationDatasets/{dataset}/conversationDataItems/{data_item}/conversationAnnotations/{annotation}
* @return value or {@code null} for none
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/**
* The unique identifier of the annotation. Format: projects/{project}/locations/{location}/conver
* sationDatasets/{dataset}/conversationDataItems/{data_item}/conversationAnnotations/{annotation}
* @param annotationId annotationId or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
/**
* The feedback that the customer has about the answer in `data`.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1AnswerFeedback getAnswerFeedback() {
return answerFeedback;
}
/**
* The feedback that the customer has about the answer in `data`.
* @param answerFeedback answerFeedback or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setAnswerFeedback(GoogleCloudContactcenterinsightsV1AnswerFeedback answerFeedback) {
this.answerFeedback = answerFeedback;
return this;
}
/**
* Agent Assist Article Suggestion data.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ArticleSuggestionData getArticleSuggestion() {
return articleSuggestion;
}
/**
* Agent Assist Article Suggestion data.
* @param articleSuggestion articleSuggestion or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setArticleSuggestion(GoogleCloudContactcenterinsightsV1ArticleSuggestionData articleSuggestion) {
this.articleSuggestion = articleSuggestion;
return this;
}
/**
* Conversation summarization suggestion data.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1ConversationSummarizationSuggestionData getConversationSummarizationSuggestion() {
return conversationSummarizationSuggestion;
}
/**
* Conversation summarization suggestion data.
* @param conversationSummarizationSuggestion conversationSummarizationSuggestion or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setConversationSummarizationSuggestion(GoogleCloudContactcenterinsightsV1ConversationSummarizationSuggestionData conversationSummarizationSuggestion) {
this.conversationSummarizationSuggestion = conversationSummarizationSuggestion;
return this;
}
/**
* The time at which this annotation was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* The time at which this annotation was created.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Dialogflow interaction data.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1DialogflowInteractionData getDialogflowInteraction() {
return dialogflowInteraction;
}
/**
* Dialogflow interaction data.
* @param dialogflowInteraction dialogflowInteraction or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setDialogflowInteraction(GoogleCloudContactcenterinsightsV1DialogflowInteractionData dialogflowInteraction) {
this.dialogflowInteraction = dialogflowInteraction;
return this;
}
/**
* The boundary in the conversation where the annotation ends, inclusive.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1AnnotationBoundary getEndBoundary() {
return endBoundary;
}
/**
* The boundary in the conversation where the annotation ends, inclusive.
* @param endBoundary endBoundary or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setEndBoundary(GoogleCloudContactcenterinsightsV1AnnotationBoundary endBoundary) {
this.endBoundary = endBoundary;
return this;
}
/**
* Agent Assist FAQ answer data.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1FaqAnswerData getFaqAnswer() {
return faqAnswer;
}
/**
* Agent Assist FAQ answer data.
* @param faqAnswer faqAnswer or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setFaqAnswer(GoogleCloudContactcenterinsightsV1FaqAnswerData faqAnswer) {
this.faqAnswer = faqAnswer;
return this;
}
/**
* The generator suggestion result.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1GetGeneratorSuggestionResponse getGeneratorSuggestionResult() {
return generatorSuggestionResult;
}
/**
* The generator suggestion result.
* @param generatorSuggestionResult generatorSuggestionResult or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setGeneratorSuggestionResult(GoogleCloudContactcenterinsightsV1GetGeneratorSuggestionResponse generatorSuggestionResult) {
this.generatorSuggestionResult = generatorSuggestionResult;
return this;
}
/**
* The Knowledge Assist result.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1GetKnowledgeAssistResponse getKnowledgeAssistResult() {
return knowledgeAssistResult;
}
/**
* The Knowledge Assist result.
* @param knowledgeAssistResult knowledgeAssistResult or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setKnowledgeAssistResult(GoogleCloudContactcenterinsightsV1GetKnowledgeAssistResponse knowledgeAssistResult) {
this.knowledgeAssistResult = knowledgeAssistResult;
return this;
}
/**
* The Knowledge Search result.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1SearchKnowledgeAnswer getKnowledgeSearchResult() {
return knowledgeSearchResult;
}
/**
* The Knowledge Search result.
* @param knowledgeSearchResult knowledgeSearchResult or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setKnowledgeSearchResult(GoogleCloudContactcenterinsightsV1SearchKnowledgeAnswer knowledgeSearchResult) {
this.knowledgeSearchResult = knowledgeSearchResult;
return this;
}
/**
* Agent Assist Smart Compose suggestion data.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1SmartComposeSuggestionData getSmartComposeSuggestion() {
return smartComposeSuggestion;
}
/**
* Agent Assist Smart Compose suggestion data.
* @param smartComposeSuggestion smartComposeSuggestion or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setSmartComposeSuggestion(GoogleCloudContactcenterinsightsV1SmartComposeSuggestionData smartComposeSuggestion) {
this.smartComposeSuggestion = smartComposeSuggestion;
return this;
}
/**
* Agent Assist Smart Reply data.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1SmartReplyData getSmartReply() {
return smartReply;
}
/**
* Agent Assist Smart Reply data.
* @param smartReply smartReply or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setSmartReply(GoogleCloudContactcenterinsightsV1SmartReplyData smartReply) {
this.smartReply = smartReply;
return this;
}
/**
* The boundary in the conversation where the annotation starts, inclusive.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1AnnotationBoundary getStartBoundary() {
return startBoundary;
}
/**
* The boundary in the conversation where the annotation starts, inclusive.
* @param startBoundary startBoundary or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation setStartBoundary(GoogleCloudContactcenterinsightsV1AnnotationBoundary startBoundary) {
this.startBoundary = startBoundary;
return this;
}
@Override
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation set(String fieldName, Object value) {
return (GoogleCloudContactcenterinsightsV1RuntimeAnnotation) super.set(fieldName, value);
}
@Override
public GoogleCloudContactcenterinsightsV1RuntimeAnnotation clone() {
return (GoogleCloudContactcenterinsightsV1RuntimeAnnotation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy