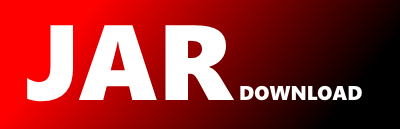
com.google.api.services.contactcenterinsights.v1.model.GoogleCloudContactcenterinsightsV1Settings Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.contactcenterinsights.v1.model;
/**
* The settings resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Contact Center AI Insights API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudContactcenterinsightsV1Settings extends com.google.api.client.json.GenericJson {
/**
* Default analysis settings.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1SettingsAnalysisConfig analysisConfig;
/**
* The default TTL for newly-created conversations. If a conversation has a specified expiration,
* that value will be used instead. Changing this value will not change the expiration of existing
* conversations. Conversations with no expire time persist until they are deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String conversationTtl;
/**
* Output only. The time at which the settings was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* A language code to be applied to each transcript segment unless the segment already specifies a
* language code. Language code defaults to "en-US" if it is neither specified on the segment nor
* here.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/**
* Immutable. The resource name of the settings resource. Format:
* projects/{project}/locations/{location}/settings
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* A map that maps a notification trigger to a Pub/Sub topic. Each time a specified trigger
* occurs, Insights will notify the corresponding Pub/Sub topic. Keys are notification triggers.
* Supported keys are: * "all-triggers": Notify each time any of the supported triggers occurs. *
* "create-analysis": Notify each time an analysis is created. * "create-conversation": Notify
* each time a conversation is created. * "export-insights-data": Notify each time an export is
* complete. * "ingest-conversations": Notify each time an IngestConversations LRO completes. *
* "update-conversation": Notify each time a conversation is updated via UpdateConversation. *
* "upload-conversation": Notify when an UploadConversation LRO completes. Values are Pub/Sub
* topics. The format of each Pub/Sub topic is: projects/{project}/topics/{topic}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map pubsubNotificationSettings;
/**
* Default DLP redaction resources to be applied while ingesting conversations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1RedactionConfig redactionConfig;
/**
* Optional. Default Speech-to-Text resources to be used while ingesting audio files. Optional,
* CCAI Insights will create a default if not provided.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudContactcenterinsightsV1SpeechConfig speechConfig;
/**
* Output only. The time at which the settings were last updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Default analysis settings.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1SettingsAnalysisConfig getAnalysisConfig() {
return analysisConfig;
}
/**
* Default analysis settings.
* @param analysisConfig analysisConfig or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setAnalysisConfig(GoogleCloudContactcenterinsightsV1SettingsAnalysisConfig analysisConfig) {
this.analysisConfig = analysisConfig;
return this;
}
/**
* The default TTL for newly-created conversations. If a conversation has a specified expiration,
* that value will be used instead. Changing this value will not change the expiration of existing
* conversations. Conversations with no expire time persist until they are deleted.
* @return value or {@code null} for none
*/
public String getConversationTtl() {
return conversationTtl;
}
/**
* The default TTL for newly-created conversations. If a conversation has a specified expiration,
* that value will be used instead. Changing this value will not change the expiration of existing
* conversations. Conversations with no expire time persist until they are deleted.
* @param conversationTtl conversationTtl or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setConversationTtl(String conversationTtl) {
this.conversationTtl = conversationTtl;
return this;
}
/**
* Output only. The time at which the settings was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The time at which the settings was created.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* A language code to be applied to each transcript segment unless the segment already specifies a
* language code. Language code defaults to "en-US" if it is neither specified on the segment nor
* here.
* @return value or {@code null} for none
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* A language code to be applied to each transcript segment unless the segment already specifies a
* language code. Language code defaults to "en-US" if it is neither specified on the segment nor
* here.
* @param languageCode languageCode or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Immutable. The resource name of the settings resource. Format:
* projects/{project}/locations/{location}/settings
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Immutable. The resource name of the settings resource. Format:
* projects/{project}/locations/{location}/settings
* @param name name or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* A map that maps a notification trigger to a Pub/Sub topic. Each time a specified trigger
* occurs, Insights will notify the corresponding Pub/Sub topic. Keys are notification triggers.
* Supported keys are: * "all-triggers": Notify each time any of the supported triggers occurs. *
* "create-analysis": Notify each time an analysis is created. * "create-conversation": Notify
* each time a conversation is created. * "export-insights-data": Notify each time an export is
* complete. * "ingest-conversations": Notify each time an IngestConversations LRO completes. *
* "update-conversation": Notify each time a conversation is updated via UpdateConversation. *
* "upload-conversation": Notify when an UploadConversation LRO completes. Values are Pub/Sub
* topics. The format of each Pub/Sub topic is: projects/{project}/topics/{topic}
* @return value or {@code null} for none
*/
public java.util.Map getPubsubNotificationSettings() {
return pubsubNotificationSettings;
}
/**
* A map that maps a notification trigger to a Pub/Sub topic. Each time a specified trigger
* occurs, Insights will notify the corresponding Pub/Sub topic. Keys are notification triggers.
* Supported keys are: * "all-triggers": Notify each time any of the supported triggers occurs. *
* "create-analysis": Notify each time an analysis is created. * "create-conversation": Notify
* each time a conversation is created. * "export-insights-data": Notify each time an export is
* complete. * "ingest-conversations": Notify each time an IngestConversations LRO completes. *
* "update-conversation": Notify each time a conversation is updated via UpdateConversation. *
* "upload-conversation": Notify when an UploadConversation LRO completes. Values are Pub/Sub
* topics. The format of each Pub/Sub topic is: projects/{project}/topics/{topic}
* @param pubsubNotificationSettings pubsubNotificationSettings or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setPubsubNotificationSettings(java.util.Map pubsubNotificationSettings) {
this.pubsubNotificationSettings = pubsubNotificationSettings;
return this;
}
/**
* Default DLP redaction resources to be applied while ingesting conversations.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1RedactionConfig getRedactionConfig() {
return redactionConfig;
}
/**
* Default DLP redaction resources to be applied while ingesting conversations.
* @param redactionConfig redactionConfig or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setRedactionConfig(GoogleCloudContactcenterinsightsV1RedactionConfig redactionConfig) {
this.redactionConfig = redactionConfig;
return this;
}
/**
* Optional. Default Speech-to-Text resources to be used while ingesting audio files. Optional,
* CCAI Insights will create a default if not provided.
* @return value or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1SpeechConfig getSpeechConfig() {
return speechConfig;
}
/**
* Optional. Default Speech-to-Text resources to be used while ingesting audio files. Optional,
* CCAI Insights will create a default if not provided.
* @param speechConfig speechConfig or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setSpeechConfig(GoogleCloudContactcenterinsightsV1SpeechConfig speechConfig) {
this.speechConfig = speechConfig;
return this;
}
/**
* Output only. The time at which the settings were last updated.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The time at which the settings were last updated.
* @param updateTime updateTime or {@code null} for none
*/
public GoogleCloudContactcenterinsightsV1Settings setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public GoogleCloudContactcenterinsightsV1Settings set(String fieldName, Object value) {
return (GoogleCloudContactcenterinsightsV1Settings) super.set(fieldName, value);
}
@Override
public GoogleCloudContactcenterinsightsV1Settings clone() {
return (GoogleCloudContactcenterinsightsV1Settings) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy