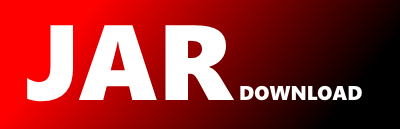
com.google.api.services.containeranalysis.v1.model.Signature Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.containeranalysis.v1.model;
/**
* Verifiers (e.g. Kritis implementations) MUST verify signatures with respect to the trust anchors
* defined in policy (e.g. a Kritis policy). Typically this means that the verifier has been
* configured with a map from `public_key_id` to public key material (and any required parameters,
* e.g. signing algorithm). In particular, verification implementations MUST NOT treat the signature
* `public_key_id` as anything more than a key lookup hint. The `public_key_id` DOES NOT validate or
* authenticate a public key; it only provides a mechanism for quickly selecting a public key
* ALREADY CONFIGURED on the verifier through a trusted channel. Verification implementations MUST
* reject signatures in any of the following circumstances: * The `public_key_id` is not recognized
* by the verifier. * The public key that `public_key_id` refers to does not verify the signature
* with respect to the payload. The `signature` contents SHOULD NOT be "attached" (where the payload
* is included with the serialized `signature` bytes). Verifiers MUST ignore any "attached" payload
* and only verify signatures with respect to explicitly provided payload (e.g. a `payload` field on
* the proto message that holds this Signature, or the canonical serialization of the proto message
* that holds this signature).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Container Analysis API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Signature extends com.google.api.client.json.GenericJson {
/**
* The identifier for the public key that verifies this signature. * The `public_key_id` is
* required. * The `public_key_id` SHOULD be an RFC3986 conformant URI. * When possible, the
* `public_key_id` SHOULD be an immutable reference, such as a cryptographic digest. Examples of
* valid `public_key_id`s: OpenPGP V4 public key fingerprint: *
* "openpgp4fpr:74FAF3B861BDA0870C7B6DEF607E48D2A663AEEA" See
* https://www.iana.org/assignments/uri-schemes/prov/openpgp4fpr for more details on this scheme.
* RFC6920 digest-named SubjectPublicKeyInfo (digest of the DER serialization): *
* "ni:sha-256;cD9o9Cq6LG3jD0iKXqEi_vdjJGecm_iXkbqVoScViaU" *
* "nih:sha-256;703f68f42aba2c6de30f488a5ea122fef76324679c9bf89791ba95a1271589a5"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String publicKeyId;
/**
* The content of the signature, an opaque bytestring. The payload that this signature verifies
* MUST be unambiguously provided with the Signature during verification. A wrapper message might
* provide the payload explicitly. Alternatively, a message might have a canonical serialization
* that can always be unambiguously computed to derive the payload.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String signature;
/**
* The identifier for the public key that verifies this signature. * The `public_key_id` is
* required. * The `public_key_id` SHOULD be an RFC3986 conformant URI. * When possible, the
* `public_key_id` SHOULD be an immutable reference, such as a cryptographic digest. Examples of
* valid `public_key_id`s: OpenPGP V4 public key fingerprint: *
* "openpgp4fpr:74FAF3B861BDA0870C7B6DEF607E48D2A663AEEA" See
* https://www.iana.org/assignments/uri-schemes/prov/openpgp4fpr for more details on this scheme.
* RFC6920 digest-named SubjectPublicKeyInfo (digest of the DER serialization): *
* "ni:sha-256;cD9o9Cq6LG3jD0iKXqEi_vdjJGecm_iXkbqVoScViaU" *
* "nih:sha-256;703f68f42aba2c6de30f488a5ea122fef76324679c9bf89791ba95a1271589a5"
* @return value or {@code null} for none
*/
public java.lang.String getPublicKeyId() {
return publicKeyId;
}
/**
* The identifier for the public key that verifies this signature. * The `public_key_id` is
* required. * The `public_key_id` SHOULD be an RFC3986 conformant URI. * When possible, the
* `public_key_id` SHOULD be an immutable reference, such as a cryptographic digest. Examples of
* valid `public_key_id`s: OpenPGP V4 public key fingerprint: *
* "openpgp4fpr:74FAF3B861BDA0870C7B6DEF607E48D2A663AEEA" See
* https://www.iana.org/assignments/uri-schemes/prov/openpgp4fpr for more details on this scheme.
* RFC6920 digest-named SubjectPublicKeyInfo (digest of the DER serialization): *
* "ni:sha-256;cD9o9Cq6LG3jD0iKXqEi_vdjJGecm_iXkbqVoScViaU" *
* "nih:sha-256;703f68f42aba2c6de30f488a5ea122fef76324679c9bf89791ba95a1271589a5"
* @param publicKeyId publicKeyId or {@code null} for none
*/
public Signature setPublicKeyId(java.lang.String publicKeyId) {
this.publicKeyId = publicKeyId;
return this;
}
/**
* The content of the signature, an opaque bytestring. The payload that this signature verifies
* MUST be unambiguously provided with the Signature during verification. A wrapper message might
* provide the payload explicitly. Alternatively, a message might have a canonical serialization
* that can always be unambiguously computed to derive the payload.
* @see #decodeSignature()
* @return value or {@code null} for none
*/
public java.lang.String getSignature() {
return signature;
}
/**
* The content of the signature, an opaque bytestring. The payload that this signature verifies
* MUST be unambiguously provided with the Signature during verification. A wrapper message might
* provide the payload explicitly. Alternatively, a message might have a canonical serialization
* that can always be unambiguously computed to derive the payload.
* @see #getSignature()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeSignature() {
return com.google.api.client.util.Base64.decodeBase64(signature);
}
/**
* The content of the signature, an opaque bytestring. The payload that this signature verifies
* MUST be unambiguously provided with the Signature during verification. A wrapper message might
* provide the payload explicitly. Alternatively, a message might have a canonical serialization
* that can always be unambiguously computed to derive the payload.
* @see #encodeSignature()
* @param signature signature or {@code null} for none
*/
public Signature setSignature(java.lang.String signature) {
this.signature = signature;
return this;
}
/**
* The content of the signature, an opaque bytestring. The payload that this signature verifies
* MUST be unambiguously provided with the Signature during verification. A wrapper message might
* provide the payload explicitly. Alternatively, a message might have a canonical serialization
* that can always be unambiguously computed to derive the payload.
* @see #setSignature()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public Signature encodeSignature(byte[] signature) {
this.signature = com.google.api.client.util.Base64.encodeBase64URLSafeString(signature);
return this;
}
@Override
public Signature set(String fieldName, Object value) {
return (Signature) super.set(fieldName, value);
}
@Override
public Signature clone() {
return (Signature) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy