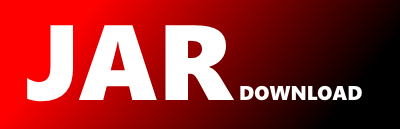
com.google.api.services.containeranalysis.v1.model.VexAssessment Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.containeranalysis.v1.model;
/**
* VexAssessment provides all publisher provided Vex information that is related to this
* vulnerability.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Container Analysis API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class VexAssessment extends com.google.api.client.json.GenericJson {
/**
* Holds the MITRE standard Common Vulnerabilities and Exposures (CVE) tracking number for the
* vulnerability. Deprecated: Use vulnerability_id instead to denote CVEs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cve;
/**
* Contains information about the impact of this vulnerability, this will change with time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List impacts;
/**
* Justification provides the justification when the state of the assessment if NOT_AFFECTED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Justification justification;
/**
* The VulnerabilityAssessment note from which this VexAssessment was generated. This will be of
* the form: `projects/[PROJECT_ID]/notes/[NOTE_ID]`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String noteName;
/**
* Holds a list of references associated with this vulnerability item and assessment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List relatedUris;
static {
// hack to force ProGuard to consider RelatedUrl used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(RelatedUrl.class);
}
/**
* Specifies details on how to handle (and presumably, fix) a vulnerability.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List remediations;
static {
// hack to force ProGuard to consider Remediation used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Remediation.class);
}
/**
* Provides the state of this Vulnerability assessment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* The vulnerability identifier for this Assessment. Will hold one of common identifiers e.g. CVE,
* GHSA etc.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String vulnerabilityId;
/**
* Holds the MITRE standard Common Vulnerabilities and Exposures (CVE) tracking number for the
* vulnerability. Deprecated: Use vulnerability_id instead to denote CVEs.
* @return value or {@code null} for none
*/
public java.lang.String getCve() {
return cve;
}
/**
* Holds the MITRE standard Common Vulnerabilities and Exposures (CVE) tracking number for the
* vulnerability. Deprecated: Use vulnerability_id instead to denote CVEs.
* @param cve cve or {@code null} for none
*/
public VexAssessment setCve(java.lang.String cve) {
this.cve = cve;
return this;
}
/**
* Contains information about the impact of this vulnerability, this will change with time.
* @return value or {@code null} for none
*/
public java.util.List getImpacts() {
return impacts;
}
/**
* Contains information about the impact of this vulnerability, this will change with time.
* @param impacts impacts or {@code null} for none
*/
public VexAssessment setImpacts(java.util.List impacts) {
this.impacts = impacts;
return this;
}
/**
* Justification provides the justification when the state of the assessment if NOT_AFFECTED.
* @return value or {@code null} for none
*/
public Justification getJustification() {
return justification;
}
/**
* Justification provides the justification when the state of the assessment if NOT_AFFECTED.
* @param justification justification or {@code null} for none
*/
public VexAssessment setJustification(Justification justification) {
this.justification = justification;
return this;
}
/**
* The VulnerabilityAssessment note from which this VexAssessment was generated. This will be of
* the form: `projects/[PROJECT_ID]/notes/[NOTE_ID]`.
* @return value or {@code null} for none
*/
public java.lang.String getNoteName() {
return noteName;
}
/**
* The VulnerabilityAssessment note from which this VexAssessment was generated. This will be of
* the form: `projects/[PROJECT_ID]/notes/[NOTE_ID]`.
* @param noteName noteName or {@code null} for none
*/
public VexAssessment setNoteName(java.lang.String noteName) {
this.noteName = noteName;
return this;
}
/**
* Holds a list of references associated with this vulnerability item and assessment.
* @return value or {@code null} for none
*/
public java.util.List getRelatedUris() {
return relatedUris;
}
/**
* Holds a list of references associated with this vulnerability item and assessment.
* @param relatedUris relatedUris or {@code null} for none
*/
public VexAssessment setRelatedUris(java.util.List relatedUris) {
this.relatedUris = relatedUris;
return this;
}
/**
* Specifies details on how to handle (and presumably, fix) a vulnerability.
* @return value or {@code null} for none
*/
public java.util.List getRemediations() {
return remediations;
}
/**
* Specifies details on how to handle (and presumably, fix) a vulnerability.
* @param remediations remediations or {@code null} for none
*/
public VexAssessment setRemediations(java.util.List remediations) {
this.remediations = remediations;
return this;
}
/**
* Provides the state of this Vulnerability assessment.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Provides the state of this Vulnerability assessment.
* @param state state or {@code null} for none
*/
public VexAssessment setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* The vulnerability identifier for this Assessment. Will hold one of common identifiers e.g. CVE,
* GHSA etc.
* @return value or {@code null} for none
*/
public java.lang.String getVulnerabilityId() {
return vulnerabilityId;
}
/**
* The vulnerability identifier for this Assessment. Will hold one of common identifiers e.g. CVE,
* GHSA etc.
* @param vulnerabilityId vulnerabilityId or {@code null} for none
*/
public VexAssessment setVulnerabilityId(java.lang.String vulnerabilityId) {
this.vulnerabilityId = vulnerabilityId;
return this;
}
@Override
public VexAssessment set(String fieldName, Object value) {
return (VexAssessment) super.set(fieldName, value);
}
@Override
public VexAssessment clone() {
return (VexAssessment) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy