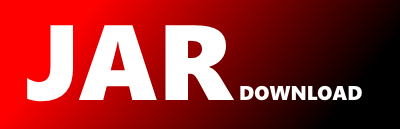
com.google.api.services.containeranalysis.v1.model.VulnerabilityNote Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.containeranalysis.v1.model;
/**
* A security vulnerability that can be found in resources.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Container Analysis API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class VulnerabilityNote extends com.google.api.client.json.GenericJson {
/**
* The CVSS score of this vulnerability. CVSS score is on a scale of 0 - 10 where 0 indicates low
* severity and 10 indicates high severity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float cvssScore;
/**
* The full description of the v2 CVSS for this vulnerability.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CVSS cvssV2;
/**
* The full description of the CVSSv3 for this vulnerability.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CVSSv3 cvssV3;
/**
* CVSS version used to populate cvss_score and severity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cvssVersion;
/**
* Details of all known distros and packages affected by this vulnerability.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List details;
static {
// hack to force ProGuard to consider Detail used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Detail.class);
}
/**
* The note provider assigned severity of this vulnerability.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String severity;
/**
* The time this information was last changed at the source. This is an upstream timestamp from
* the underlying information source - e.g. Ubuntu security tracker.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String sourceUpdateTime;
/**
* Windows details get their own format because the information format and model don't match a
* normal detail. Specifically Windows updates are done as patches, thus Windows vulnerabilities
* really are a missing package, rather than a package being at an incorrect version.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List windowsDetails;
/**
* The CVSS score of this vulnerability. CVSS score is on a scale of 0 - 10 where 0 indicates low
* severity and 10 indicates high severity.
* @return value or {@code null} for none
*/
public java.lang.Float getCvssScore() {
return cvssScore;
}
/**
* The CVSS score of this vulnerability. CVSS score is on a scale of 0 - 10 where 0 indicates low
* severity and 10 indicates high severity.
* @param cvssScore cvssScore or {@code null} for none
*/
public VulnerabilityNote setCvssScore(java.lang.Float cvssScore) {
this.cvssScore = cvssScore;
return this;
}
/**
* The full description of the v2 CVSS for this vulnerability.
* @return value or {@code null} for none
*/
public CVSS getCvssV2() {
return cvssV2;
}
/**
* The full description of the v2 CVSS for this vulnerability.
* @param cvssV2 cvssV2 or {@code null} for none
*/
public VulnerabilityNote setCvssV2(CVSS cvssV2) {
this.cvssV2 = cvssV2;
return this;
}
/**
* The full description of the CVSSv3 for this vulnerability.
* @return value or {@code null} for none
*/
public CVSSv3 getCvssV3() {
return cvssV3;
}
/**
* The full description of the CVSSv3 for this vulnerability.
* @param cvssV3 cvssV3 or {@code null} for none
*/
public VulnerabilityNote setCvssV3(CVSSv3 cvssV3) {
this.cvssV3 = cvssV3;
return this;
}
/**
* CVSS version used to populate cvss_score and severity.
* @return value or {@code null} for none
*/
public java.lang.String getCvssVersion() {
return cvssVersion;
}
/**
* CVSS version used to populate cvss_score and severity.
* @param cvssVersion cvssVersion or {@code null} for none
*/
public VulnerabilityNote setCvssVersion(java.lang.String cvssVersion) {
this.cvssVersion = cvssVersion;
return this;
}
/**
* Details of all known distros and packages affected by this vulnerability.
* @return value or {@code null} for none
*/
public java.util.List getDetails() {
return details;
}
/**
* Details of all known distros and packages affected by this vulnerability.
* @param details details or {@code null} for none
*/
public VulnerabilityNote setDetails(java.util.List details) {
this.details = details;
return this;
}
/**
* The note provider assigned severity of this vulnerability.
* @return value or {@code null} for none
*/
public java.lang.String getSeverity() {
return severity;
}
/**
* The note provider assigned severity of this vulnerability.
* @param severity severity or {@code null} for none
*/
public VulnerabilityNote setSeverity(java.lang.String severity) {
this.severity = severity;
return this;
}
/**
* The time this information was last changed at the source. This is an upstream timestamp from
* the underlying information source - e.g. Ubuntu security tracker.
* @return value or {@code null} for none
*/
public String getSourceUpdateTime() {
return sourceUpdateTime;
}
/**
* The time this information was last changed at the source. This is an upstream timestamp from
* the underlying information source - e.g. Ubuntu security tracker.
* @param sourceUpdateTime sourceUpdateTime or {@code null} for none
*/
public VulnerabilityNote setSourceUpdateTime(String sourceUpdateTime) {
this.sourceUpdateTime = sourceUpdateTime;
return this;
}
/**
* Windows details get their own format because the information format and model don't match a
* normal detail. Specifically Windows updates are done as patches, thus Windows vulnerabilities
* really are a missing package, rather than a package being at an incorrect version.
* @return value or {@code null} for none
*/
public java.util.List getWindowsDetails() {
return windowsDetails;
}
/**
* Windows details get their own format because the information format and model don't match a
* normal detail. Specifically Windows updates are done as patches, thus Windows vulnerabilities
* really are a missing package, rather than a package being at an incorrect version.
* @param windowsDetails windowsDetails or {@code null} for none
*/
public VulnerabilityNote setWindowsDetails(java.util.List windowsDetails) {
this.windowsDetails = windowsDetails;
return this;
}
@Override
public VulnerabilityNote set(String fieldName, Object value) {
return (VulnerabilityNote) super.set(fieldName, value);
}
@Override
public VulnerabilityNote clone() {
return (VulnerabilityNote) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy