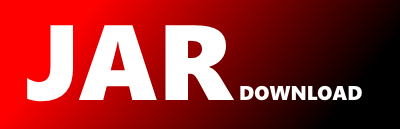
com.google.api.services.content.ShoppingContent Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-04-06 17:52:19 UTC)
* on 2018-05-01 at 17:01:15 UTC
* Modify at your own risk.
*/
package com.google.api.services.content;
/**
* Service definition for ShoppingContent (v2).
*
*
* Manages product items, inventory, and Merchant Center accounts for Google Shopping.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link ShoppingContentRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class ShoppingContent extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.21.0 of the Content API for Shopping library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "content/v2/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public ShoppingContent(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
ShoppingContent(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Accounts collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Accounts.List request = content.accounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounts accounts() {
return new Accounts();
}
/**
* The "accounts" collection of methods.
*/
public class Accounts {
/**
* Returns information about the authenticated user.
*
* Create a request for the method "accounts.authinfo".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Authinfo#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Authinfo authinfo() throws java.io.IOException {
Authinfo result = new Authinfo();
initialize(result);
return result;
}
public class Authinfo extends ShoppingContentRequest {
private static final String REST_PATH = "accounts/authinfo";
/**
* Returns information about the authenticated user.
*
* Create a request for the method "accounts.authinfo".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Authinfo#execute()} method to invoke the remote operation.
* {@link
* Authinfo#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Authinfo() {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.AccountsAuthInfoResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Authinfo setAlt(java.lang.String alt) {
return (Authinfo) super.setAlt(alt);
}
@Override
public Authinfo setFields(java.lang.String fields) {
return (Authinfo) super.setFields(fields);
}
@Override
public Authinfo setKey(java.lang.String key) {
return (Authinfo) super.setKey(key);
}
@Override
public Authinfo setOauthToken(java.lang.String oauthToken) {
return (Authinfo) super.setOauthToken(oauthToken);
}
@Override
public Authinfo setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Authinfo) super.setPrettyPrint(prettyPrint);
}
@Override
public Authinfo setQuotaUser(java.lang.String quotaUser) {
return (Authinfo) super.setQuotaUser(quotaUser);
}
@Override
public Authinfo setUserIp(java.lang.String userIp) {
return (Authinfo) super.setUserIp(userIp);
}
@Override
public Authinfo set(String parameterName, Object value) {
return (Authinfo) super.set(parameterName, value);
}
}
/**
* Claims the website of a Merchant Center sub-account.
*
* Create a request for the method "accounts.claimwebsite".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Claimwebsite#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account whose website is claimed.
* @return the request
*/
public Claimwebsite claimwebsite(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Claimwebsite result = new Claimwebsite(merchantId, accountId);
initialize(result);
return result;
}
public class Claimwebsite extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounts/{accountId}/claimwebsite";
/**
* Claims the website of a Merchant Center sub-account.
*
* Create a request for the method "accounts.claimwebsite".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Claimwebsite#execute()} method to invoke the remote operation.
* {@link
* Claimwebsite#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account whose website is claimed.
* @since 1.13
*/
protected Claimwebsite(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "POST", REST_PATH, null, com.google.api.services.content.model.AccountsClaimWebsiteResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Claimwebsite setAlt(java.lang.String alt) {
return (Claimwebsite) super.setAlt(alt);
}
@Override
public Claimwebsite setFields(java.lang.String fields) {
return (Claimwebsite) super.setFields(fields);
}
@Override
public Claimwebsite setKey(java.lang.String key) {
return (Claimwebsite) super.setKey(key);
}
@Override
public Claimwebsite setOauthToken(java.lang.String oauthToken) {
return (Claimwebsite) super.setOauthToken(oauthToken);
}
@Override
public Claimwebsite setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Claimwebsite) super.setPrettyPrint(prettyPrint);
}
@Override
public Claimwebsite setQuotaUser(java.lang.String quotaUser) {
return (Claimwebsite) super.setQuotaUser(quotaUser);
}
@Override
public Claimwebsite setUserIp(java.lang.String userIp) {
return (Claimwebsite) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Claimwebsite setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account whose website is claimed. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account whose website is claimed.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account whose website is claimed. */
public Claimwebsite setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/**
* Only available to selected merchants. When set to True, this flag removes any existing
* claim on the requested website by another account and replaces it with a claim from this
* account.
*/
@com.google.api.client.util.Key
private java.lang.Boolean overwrite;
/** Only available to selected merchants. When set to True, this flag removes any existing claim on the
requested website by another account and replaces it with a claim from this account.
*/
public java.lang.Boolean getOverwrite() {
return overwrite;
}
/**
* Only available to selected merchants. When set to True, this flag removes any existing
* claim on the requested website by another account and replaces it with a claim from this
* account.
*/
public Claimwebsite setOverwrite(java.lang.Boolean overwrite) {
this.overwrite = overwrite;
return this;
}
@Override
public Claimwebsite set(String parameterName, Object value) {
return (Claimwebsite) super.set(parameterName, value);
}
}
/**
* Retrieves, inserts, updates, and deletes multiple Merchant Center (sub-)accounts in a single
* request.
*
* Create a request for the method "accounts.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.AccountsCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.AccountsCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "accounts/batch";
/**
* Retrieves, inserts, updates, and deletes multiple Merchant Center (sub-)accounts in a single
* request.
*
* Create a request for the method "accounts.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.AccountsCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.AccountsCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.AccountsCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Deletes a Merchant Center sub-account.
*
* Create a request for the method "accounts.delete".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. This must be a multi-client account, and accountId must be the ID of
* a sub-account of this account.
* @param accountId The ID of the account.
* @return the request
*/
public Delete delete(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Delete result = new Delete(merchantId, accountId);
initialize(result);
return result;
}
public class Delete extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounts/{accountId}";
/**
* Deletes a Merchant Center sub-account.
*
* Create a request for the method "accounts.delete".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. This must be a multi-client account, and accountId must be the ID of
* a sub-account of this account.
* @param accountId The ID of the account.
* @since 1.13
*/
protected Delete(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "DELETE", REST_PATH, null, Void.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the managing account. This must be a multi-client account, and accountId must be
* the ID of a sub-account of this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. This must be a multi-client account, and accountId must be the ID
of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. This must be a multi-client account, and accountId must be
* the ID of a sub-account of this account.
*/
public Delete setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account. */
public Delete setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Delete setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
/** Flag to delete sub-accounts with products. The default value is false. */
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Flag to delete sub-accounts with products. The default value is false. [default: false]
*/
public java.lang.Boolean getForce() {
return force;
}
/** Flag to delete sub-accounts with products. The default value is false. */
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Flag to delete sub-accounts with products. The default value is false.
*
*/
public boolean isForce() {
if (force == null || force == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return force;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a Merchant Center account.
*
* Create a request for the method "accounts.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Get result = new Get(merchantId, accountId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounts/{accountId}";
/**
* Retrieves a Merchant Center account.
*
* Create a request for the method "accounts.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.Account.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account. */
public Get setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a Merchant Center sub-account.
*
* Create a request for the method "accounts.insert".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @param content the {@link com.google.api.services.content.model.Account}
* @return the request
*/
public Insert insert(java.math.BigInteger merchantId, com.google.api.services.content.model.Account content) throws java.io.IOException {
Insert result = new Insert(merchantId, content);
initialize(result);
return result;
}
public class Insert extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounts";
/**
* Creates a Merchant Center sub-account.
*
* Create a request for the method "accounts.insert".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @param content the {@link com.google.api.services.content.model.Account}
* @since 1.13
*/
protected Insert(java.math.BigInteger merchantId, com.google.api.services.content.model.Account content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.Account.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Account.getName()");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the managing account. This must be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. This must be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. This must be a multi-client account. */
public Insert setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Insert setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists the sub-accounts in your Merchant Center account.
*
* Create a request for the method "accounts.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounts";
/**
* Lists the sub-accounts in your Merchant Center account.
*
* Create a request for the method "accounts.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.AccountsListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the managing account. This must be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. This must be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. This must be a multi-client account. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The maximum number of accounts to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of accounts to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of accounts to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a Merchant Center account. This method supports patch semantics.
*
* Create a request for the method "accounts.patch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @param content the {@link com.google.api.services.content.model.Account}
* @return the request
*/
public Patch patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.Account content) throws java.io.IOException {
Patch result = new Patch(merchantId, accountId, content);
initialize(result);
return result;
}
public class Patch extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounts/{accountId}";
/**
* Updates a Merchant Center account. This method supports patch semantics.
*
* Create a request for the method "accounts.patch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @param content the {@link com.google.api.services.content.model.Account}
* @since 1.13
*/
protected Patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.Account content) {
super(ShoppingContent.this, "PATCH", REST_PATH, content, com.google.api.services.content.model.Account.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Patch setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account. */
public Patch setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Patch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a Merchant Center account.
*
* Create a request for the method "accounts.update".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @param content the {@link com.google.api.services.content.model.Account}
* @return the request
*/
public Update update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.Account content) throws java.io.IOException {
Update result = new Update(merchantId, accountId, content);
initialize(result);
return result;
}
public class Update extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounts/{accountId}";
/**
* Updates a Merchant Center account.
*
* Create a request for the method "accounts.update".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @param content the {@link com.google.api.services.content.model.Account}
* @since 1.13
*/
protected Update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.Account content) {
super(ShoppingContent.this, "PUT", REST_PATH, content, com.google.api.services.content.model.Account.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getId(), "Account.getId()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Account.getName()");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Update setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account. */
public Update setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Update setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Accountstatuses collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Accountstatuses.List request = content.accountstatuses().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accountstatuses accountstatuses() {
return new Accountstatuses();
}
/**
* The "accountstatuses" collection of methods.
*/
public class Accountstatuses {
/**
* Create a request for the method "accountstatuses.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.AccountstatusesCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.AccountstatusesCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "accountstatuses/batch";
/**
* Create a request for the method "accountstatuses.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.AccountstatusesCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.AccountstatusesCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.AccountstatusesCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Retrieves the status of a Merchant Center account.
*
* Create a request for the method "accountstatuses.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Get result = new Get(merchantId, accountId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accountstatuses/{accountId}";
/**
* Retrieves the status of a Merchant Center account.
*
* Create a request for the method "accountstatuses.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.AccountStatus.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account. */
public Get setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
@com.google.api.client.util.Key
private java.util.List destinations;
/** If set, only issues for the specified destinations are returned, otherwise only issues for the
Shopping destination.
*/
public java.util.List getDestinations() {
return destinations;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
public Get setDestinations(java.util.List destinations) {
this.destinations = destinations;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the statuses of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "accountstatuses.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accountstatuses";
/**
* Lists the statuses of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "accountstatuses.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.AccountstatusesListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the managing account. This must be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. This must be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. This must be a multi-client account. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
@com.google.api.client.util.Key
private java.util.List destinations;
/** If set, only issues for the specified destinations are returned, otherwise only issues for the
Shopping destination.
*/
public java.util.List getDestinations() {
return destinations;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
public List setDestinations(java.util.List destinations) {
this.destinations = destinations;
return this;
}
/** The maximum number of account statuses to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of account statuses to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of account statuses to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Accounttax collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Accounttax.List request = content.accounttax().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounttax accounttax() {
return new Accounttax();
}
/**
* The "accounttax" collection of methods.
*/
public class Accounttax {
/**
* Retrieves and updates tax settings of multiple accounts in a single request.
*
* Create a request for the method "accounttax.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.AccounttaxCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.AccounttaxCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "accounttax/batch";
/**
* Retrieves and updates tax settings of multiple accounts in a single request.
*
* Create a request for the method "accounttax.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.AccounttaxCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.AccounttaxCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.AccounttaxCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Retrieves the tax settings of the account.
*
* Create a request for the method "accounttax.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update account tax settings.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Get result = new Get(merchantId, accountId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounttax/{accountId}";
/**
* Retrieves the tax settings of the account.
*
* Create a request for the method "accounttax.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update account tax settings.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.AccountTax.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get/update account tax settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get/update account tax settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get/update account tax settings. */
public Get setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the tax settings of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "accounttax.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounttax";
/**
* Lists the tax settings of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "accounttax.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.AccounttaxListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the managing account. This must be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. This must be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. This must be a multi-client account. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The maximum number of tax settings to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of tax settings to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of tax settings to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the tax settings of the account. This method supports patch semantics.
*
* Create a request for the method "accounttax.patch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update account tax settings.
* @param content the {@link com.google.api.services.content.model.AccountTax}
* @return the request
*/
public Patch patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.AccountTax content) throws java.io.IOException {
Patch result = new Patch(merchantId, accountId, content);
initialize(result);
return result;
}
public class Patch extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounttax/{accountId}";
/**
* Updates the tax settings of the account. This method supports patch semantics.
*
* Create a request for the method "accounttax.patch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update account tax settings.
* @param content the {@link com.google.api.services.content.model.AccountTax}
* @since 1.13
*/
protected Patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.AccountTax content) {
super(ShoppingContent.this, "PATCH", REST_PATH, content, com.google.api.services.content.model.AccountTax.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Patch setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get/update account tax settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get/update account tax settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get/update account tax settings. */
public Patch setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Patch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the tax settings of the account.
*
* Create a request for the method "accounttax.update".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update account tax settings.
* @param content the {@link com.google.api.services.content.model.AccountTax}
* @return the request
*/
public Update update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.AccountTax content) throws java.io.IOException {
Update result = new Update(merchantId, accountId, content);
initialize(result);
return result;
}
public class Update extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/accounttax/{accountId}";
/**
* Updates the tax settings of the account.
*
* Create a request for the method "accounttax.update".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update account tax settings.
* @param content the {@link com.google.api.services.content.model.AccountTax}
* @since 1.13
*/
protected Update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.AccountTax content) {
super(ShoppingContent.this, "PUT", REST_PATH, content, com.google.api.services.content.model.AccountTax.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getAccountId(), "AccountTax.getAccountId()");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Update setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get/update account tax settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get/update account tax settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get/update account tax settings. */
public Update setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Update setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Datafeeds collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Datafeeds.List request = content.datafeeds().list(parameters ...)}
*
*
* @return the resource collection
*/
public Datafeeds datafeeds() {
return new Datafeeds();
}
/**
* The "datafeeds" collection of methods.
*/
public class Datafeeds {
/**
* Create a request for the method "datafeeds.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.DatafeedsCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.DatafeedsCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "datafeeds/batch";
/**
* Create a request for the method "datafeeds.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.DatafeedsCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.DatafeedsCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.DatafeedsCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Deletes a datafeed configuration from your Merchant Center account.
*
* Create a request for the method "datafeeds.delete".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @return the request
*/
public Delete delete(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) throws java.io.IOException {
Delete result = new Delete(merchantId, datafeedId);
initialize(result);
return result;
}
public class Delete extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeeds/{datafeedId}";
/**
* Deletes a datafeed configuration from your Merchant Center account.
*
* Create a request for the method "datafeeds.delete".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @since 1.13
*/
protected Delete(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) {
super(ShoppingContent.this, "DELETE", REST_PATH, null, Void.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.datafeedId = com.google.api.client.util.Preconditions.checkNotNull(datafeedId, "Required parameter datafeedId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeed. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
public Delete setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the datafeed. */
@com.google.api.client.util.Key
private java.math.BigInteger datafeedId;
/** The ID of the datafeed.
*/
public java.math.BigInteger getDatafeedId() {
return datafeedId;
}
/** The ID of the datafeed. */
public Delete setDatafeedId(java.math.BigInteger datafeedId) {
this.datafeedId = datafeedId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Delete setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Invokes a fetch for the datafeed in your Merchant Center account.
*
* Create a request for the method "datafeeds.fetchnow".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Fetchnow#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed to be fetched.
* @return the request
*/
public Fetchnow fetchnow(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) throws java.io.IOException {
Fetchnow result = new Fetchnow(merchantId, datafeedId);
initialize(result);
return result;
}
public class Fetchnow extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeeds/{datafeedId}/fetchNow";
/**
* Invokes a fetch for the datafeed in your Merchant Center account.
*
* Create a request for the method "datafeeds.fetchnow".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Fetchnow#execute()} method to invoke the remote operation.
* {@link
* Fetchnow#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed to be fetched.
* @since 1.13
*/
protected Fetchnow(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) {
super(ShoppingContent.this, "POST", REST_PATH, null, com.google.api.services.content.model.DatafeedsFetchNowResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.datafeedId = com.google.api.client.util.Preconditions.checkNotNull(datafeedId, "Required parameter datafeedId must be specified.");
}
@Override
public Fetchnow setAlt(java.lang.String alt) {
return (Fetchnow) super.setAlt(alt);
}
@Override
public Fetchnow setFields(java.lang.String fields) {
return (Fetchnow) super.setFields(fields);
}
@Override
public Fetchnow setKey(java.lang.String key) {
return (Fetchnow) super.setKey(key);
}
@Override
public Fetchnow setOauthToken(java.lang.String oauthToken) {
return (Fetchnow) super.setOauthToken(oauthToken);
}
@Override
public Fetchnow setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Fetchnow) super.setPrettyPrint(prettyPrint);
}
@Override
public Fetchnow setQuotaUser(java.lang.String quotaUser) {
return (Fetchnow) super.setQuotaUser(quotaUser);
}
@Override
public Fetchnow setUserIp(java.lang.String userIp) {
return (Fetchnow) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeed. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
public Fetchnow setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the datafeed to be fetched. */
@com.google.api.client.util.Key
private java.math.BigInteger datafeedId;
/** The ID of the datafeed to be fetched.
*/
public java.math.BigInteger getDatafeedId() {
return datafeedId;
}
/** The ID of the datafeed to be fetched. */
public Fetchnow setDatafeedId(java.math.BigInteger datafeedId) {
this.datafeedId = datafeedId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Fetchnow setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Fetchnow set(String parameterName, Object value) {
return (Fetchnow) super.set(parameterName, value);
}
}
/**
* Retrieves a datafeed configuration from your Merchant Center account.
*
* Create a request for the method "datafeeds.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) throws java.io.IOException {
Get result = new Get(merchantId, datafeedId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeeds/{datafeedId}";
/**
* Retrieves a datafeed configuration from your Merchant Center account.
*
* Create a request for the method "datafeeds.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.Datafeed.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.datafeedId = com.google.api.client.util.Preconditions.checkNotNull(datafeedId, "Required parameter datafeedId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeed. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the datafeed. */
@com.google.api.client.util.Key
private java.math.BigInteger datafeedId;
/** The ID of the datafeed.
*/
public java.math.BigInteger getDatafeedId() {
return datafeedId;
}
/** The ID of the datafeed. */
public Get setDatafeedId(java.math.BigInteger datafeedId) {
this.datafeedId = datafeedId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Registers a datafeed configuration with your Merchant Center account.
*
* Create a request for the method "datafeeds.insert".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param content the {@link com.google.api.services.content.model.Datafeed}
* @return the request
*/
public Insert insert(java.math.BigInteger merchantId, com.google.api.services.content.model.Datafeed content) throws java.io.IOException {
Insert result = new Insert(merchantId, content);
initialize(result);
return result;
}
public class Insert extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeeds";
/**
* Registers a datafeed configuration with your Merchant Center account.
*
* Create a request for the method "datafeeds.insert".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param content the {@link com.google.api.services.content.model.Datafeed}
* @since 1.13
*/
protected Insert(java.math.BigInteger merchantId, com.google.api.services.content.model.Datafeed content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.Datafeed.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getContentType(), "Datafeed.getContentType()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getFileName(), "Datafeed.getFileName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Datafeed.getName()");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeed. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
public Insert setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Insert setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists the configurations for datafeeds in your Merchant Center account.
*
* Create a request for the method "datafeeds.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeeds. This account cannot be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeeds";
/**
* Lists the configurations for datafeeds in your Merchant Center account.
*
* Create a request for the method "datafeeds.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeeds. This account cannot be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.DatafeedsListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeeds. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeeds. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeeds. This account cannot be a multi-client
* account.
*/
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The maximum number of products to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of products to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of products to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a datafeed configuration of your Merchant Center account. This method supports patch
* semantics.
*
* Create a request for the method "datafeeds.patch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @param content the {@link com.google.api.services.content.model.Datafeed}
* @return the request
*/
public Patch patch(java.math.BigInteger merchantId, java.math.BigInteger datafeedId, com.google.api.services.content.model.Datafeed content) throws java.io.IOException {
Patch result = new Patch(merchantId, datafeedId, content);
initialize(result);
return result;
}
public class Patch extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeeds/{datafeedId}";
/**
* Updates a datafeed configuration of your Merchant Center account. This method supports patch
* semantics.
*
* Create a request for the method "datafeeds.patch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @param content the {@link com.google.api.services.content.model.Datafeed}
* @since 1.13
*/
protected Patch(java.math.BigInteger merchantId, java.math.BigInteger datafeedId, com.google.api.services.content.model.Datafeed content) {
super(ShoppingContent.this, "PATCH", REST_PATH, content, com.google.api.services.content.model.Datafeed.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.datafeedId = com.google.api.client.util.Preconditions.checkNotNull(datafeedId, "Required parameter datafeedId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeed. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
public Patch setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the datafeed. */
@com.google.api.client.util.Key
private java.math.BigInteger datafeedId;
/** The ID of the datafeed.
*/
public java.math.BigInteger getDatafeedId() {
return datafeedId;
}
/** The ID of the datafeed. */
public Patch setDatafeedId(java.math.BigInteger datafeedId) {
this.datafeedId = datafeedId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Patch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a datafeed configuration of your Merchant Center account.
*
* Create a request for the method "datafeeds.update".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @param content the {@link com.google.api.services.content.model.Datafeed}
* @return the request
*/
public Update update(java.math.BigInteger merchantId, java.math.BigInteger datafeedId, com.google.api.services.content.model.Datafeed content) throws java.io.IOException {
Update result = new Update(merchantId, datafeedId, content);
initialize(result);
return result;
}
public class Update extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeeds/{datafeedId}";
/**
* Updates a datafeed configuration of your Merchant Center account.
*
* Create a request for the method "datafeeds.update".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @param content the {@link com.google.api.services.content.model.Datafeed}
* @since 1.13
*/
protected Update(java.math.BigInteger merchantId, java.math.BigInteger datafeedId, com.google.api.services.content.model.Datafeed content) {
super(ShoppingContent.this, "PUT", REST_PATH, content, com.google.api.services.content.model.Datafeed.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.datafeedId = com.google.api.client.util.Preconditions.checkNotNull(datafeedId, "Required parameter datafeedId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getContentType(), "Datafeed.getContentType()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getFileName(), "Datafeed.getFileName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getId(), "Datafeed.getId()");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeed. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
public Update setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the datafeed. */
@com.google.api.client.util.Key
private java.math.BigInteger datafeedId;
/** The ID of the datafeed.
*/
public java.math.BigInteger getDatafeedId() {
return datafeedId;
}
/** The ID of the datafeed. */
public Update setDatafeedId(java.math.BigInteger datafeedId) {
this.datafeedId = datafeedId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Update setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Datafeedstatuses collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Datafeedstatuses.List request = content.datafeedstatuses().list(parameters ...)}
*
*
* @return the resource collection
*/
public Datafeedstatuses datafeedstatuses() {
return new Datafeedstatuses();
}
/**
* The "datafeedstatuses" collection of methods.
*/
public class Datafeedstatuses {
/**
* Create a request for the method "datafeedstatuses.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.DatafeedstatusesCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.DatafeedstatusesCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "datafeedstatuses/batch";
/**
* Create a request for the method "datafeedstatuses.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.DatafeedstatusesCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.DatafeedstatusesCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.DatafeedstatusesCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Retrieves the status of a datafeed from your Merchant Center account.
*
* Create a request for the method "datafeedstatuses.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) throws java.io.IOException {
Get result = new Get(merchantId, datafeedId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeedstatuses/{datafeedId}";
/**
* Retrieves the status of a datafeed from your Merchant Center account.
*
* Create a request for the method "datafeedstatuses.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeed. This account cannot be a multi-client account.
* @param datafeedId The ID of the datafeed.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger datafeedId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.DatafeedStatus.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.datafeedId = com.google.api.client.util.Preconditions.checkNotNull(datafeedId, "Required parameter datafeedId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeed. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeed. This account cannot be a multi-client
* account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the datafeed. */
@com.google.api.client.util.Key
private java.math.BigInteger datafeedId;
/** The ID of the datafeed.
*/
public java.math.BigInteger getDatafeedId() {
return datafeedId;
}
/** The ID of the datafeed. */
public Get setDatafeedId(java.math.BigInteger datafeedId) {
this.datafeedId = datafeedId;
return this;
}
/**
* The country for which to get the datafeed status. If this parameter is provided then
* language must also be provided. Note that this parameter is required for feeds targeting
* multiple countries and languages, since a feed may have a different status for each target.
*/
@com.google.api.client.util.Key
private java.lang.String country;
/** The country for which to get the datafeed status. If this parameter is provided then language must
also be provided. Note that this parameter is required for feeds targeting multiple countries and
languages, since a feed may have a different status for each target.
*/
public java.lang.String getCountry() {
return country;
}
/**
* The country for which to get the datafeed status. If this parameter is provided then
* language must also be provided. Note that this parameter is required for feeds targeting
* multiple countries and languages, since a feed may have a different status for each target.
*/
public Get setCountry(java.lang.String country) {
this.country = country;
return this;
}
/**
* The language for which to get the datafeed status. If this parameter is provided then
* country must also be provided. Note that this parameter is required for feeds targeting
* multiple countries and languages, since a feed may have a different status for each target.
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language for which to get the datafeed status. If this parameter is provided then country must
also be provided. Note that this parameter is required for feeds targeting multiple countries and
languages, since a feed may have a different status for each target.
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language for which to get the datafeed status. If this parameter is provided then
* country must also be provided. Note that this parameter is required for feeds targeting
* multiple countries and languages, since a feed may have a different status for each target.
*/
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the statuses of the datafeeds in your Merchant Center account.
*
* Create a request for the method "datafeedstatuses.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the datafeeds. This account cannot be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/datafeedstatuses";
/**
* Lists the statuses of the datafeeds in your Merchant Center account.
*
* Create a request for the method "datafeedstatuses.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the datafeeds. This account cannot be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.DatafeedstatusesListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The ID of the account that manages the datafeeds. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the datafeeds. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that manages the datafeeds. This account cannot be a multi-client
* account.
*/
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The maximum number of products to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of products to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of products to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Inventory collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Inventory.List request = content.inventory().list(parameters ...)}
*
*
* @return the resource collection
*/
public Inventory inventory() {
return new Inventory();
}
/**
* The "inventory" collection of methods.
*/
public class Inventory {
/**
* Updates price and availability for multiple products or stores in a single request. This
* operation does not update the expiration date of the products.
*
* Create a request for the method "inventory.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.InventoryCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.InventoryCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "inventory/batch";
/**
* Updates price and availability for multiple products or stores in a single request. This
* operation does not update the expiration date of the products.
*
* Create a request for the method "inventory.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.InventoryCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.InventoryCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.InventoryCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Updates price and availability of a product in your Merchant Center account.
*
* Create a request for the method "inventory.set".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Set#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param storeCode The code of the store for which to update price and availability. Use online to update price and
* availability of an online product.
* @param productId The REST id of the product for which to update price and availability.
* @param content the {@link com.google.api.services.content.model.InventorySetRequest}
* @return the request
*/
public Set set(java.math.BigInteger merchantId, java.lang.String storeCode, java.lang.String productId, com.google.api.services.content.model.InventorySetRequest content) throws java.io.IOException {
Set result = new Set(merchantId, storeCode, productId, content);
initialize(result);
return result;
}
public class Set extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/inventory/{storeCode}/products/{productId}";
/**
* Updates price and availability of a product in your Merchant Center account.
*
* Create a request for the method "inventory.set".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Set#execute()} method to invoke the remote operation. {@link
* Set#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param storeCode The code of the store for which to update price and availability. Use online to update price and
* availability of an online product.
* @param productId The REST id of the product for which to update price and availability.
* @param content the {@link com.google.api.services.content.model.InventorySetRequest}
* @since 1.13
*/
protected Set(java.math.BigInteger merchantId, java.lang.String storeCode, java.lang.String productId, com.google.api.services.content.model.InventorySetRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.InventorySetResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.storeCode = com.google.api.client.util.Preconditions.checkNotNull(storeCode, "Required parameter storeCode must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public Set setAlt(java.lang.String alt) {
return (Set) super.setAlt(alt);
}
@Override
public Set setFields(java.lang.String fields) {
return (Set) super.setFields(fields);
}
@Override
public Set setKey(java.lang.String key) {
return (Set) super.setKey(key);
}
@Override
public Set setOauthToken(java.lang.String oauthToken) {
return (Set) super.setOauthToken(oauthToken);
}
@Override
public Set setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Set) super.setPrettyPrint(prettyPrint);
}
@Override
public Set setQuotaUser(java.lang.String quotaUser) {
return (Set) super.setQuotaUser(quotaUser);
}
@Override
public Set setUserIp(java.lang.String userIp) {
return (Set) super.setUserIp(userIp);
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that contains the product. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
public Set setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* The code of the store for which to update price and availability. Use online to update
* price and availability of an online product.
*/
@com.google.api.client.util.Key
private java.lang.String storeCode;
/** The code of the store for which to update price and availability. Use online to update price and
availability of an online product.
*/
public java.lang.String getStoreCode() {
return storeCode;
}
/**
* The code of the store for which to update price and availability. Use online to update
* price and availability of an online product.
*/
public Set setStoreCode(java.lang.String storeCode) {
this.storeCode = storeCode;
return this;
}
/** The REST id of the product for which to update price and availability. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The REST id of the product for which to update price and availability.
*/
public java.lang.String getProductId() {
return productId;
}
/** The REST id of the product for which to update price and availability. */
public Set setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Set setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Set set(String parameterName, Object value) {
return (Set) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Liasettings collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Liasettings.List request = content.liasettings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Liasettings liasettings() {
return new Liasettings();
}
/**
* The "liasettings" collection of methods.
*/
public class Liasettings {
/**
* Retrieves and/or updates the LIA settings of multiple accounts in a single request.
*
* Create a request for the method "liasettings.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.LiasettingsCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.LiasettingsCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "liasettings/batch";
/**
* Retrieves and/or updates the LIA settings of multiple accounts in a single request.
*
* Create a request for the method "liasettings.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.LiasettingsCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.LiasettingsCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.LiasettingsCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Retrieves the LIA settings of the account.
*
* Create a request for the method "liasettings.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get or update LIA settings.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Get result = new Get(merchantId, accountId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings/{accountId}";
/**
* Retrieves the LIA settings of the account.
*
* Create a request for the method "liasettings.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get or update LIA settings.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.LiaSettings.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get or update LIA settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get or update LIA settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get or update LIA settings. */
public Get setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the list of accessible Google My Business accounts.
*
* Create a request for the method "liasettings.getaccessiblegmbaccounts".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Getaccessiblegmbaccounts#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to retrieve accessible Google My Business accounts.
* @return the request
*/
public Getaccessiblegmbaccounts getaccessiblegmbaccounts(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Getaccessiblegmbaccounts result = new Getaccessiblegmbaccounts(merchantId, accountId);
initialize(result);
return result;
}
public class Getaccessiblegmbaccounts extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings/{accountId}/accessiblegmbaccounts";
/**
* Retrieves the list of accessible Google My Business accounts.
*
* Create a request for the method "liasettings.getaccessiblegmbaccounts".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Getaccessiblegmbaccounts#execute()} method to invoke the remote
* operation. {@link Getaccessiblegmbaccounts#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to retrieve accessible Google My Business accounts.
* @since 1.13
*/
protected Getaccessiblegmbaccounts(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.LiasettingsGetAccessibleGmbAccountsResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Getaccessiblegmbaccounts setAlt(java.lang.String alt) {
return (Getaccessiblegmbaccounts) super.setAlt(alt);
}
@Override
public Getaccessiblegmbaccounts setFields(java.lang.String fields) {
return (Getaccessiblegmbaccounts) super.setFields(fields);
}
@Override
public Getaccessiblegmbaccounts setKey(java.lang.String key) {
return (Getaccessiblegmbaccounts) super.setKey(key);
}
@Override
public Getaccessiblegmbaccounts setOauthToken(java.lang.String oauthToken) {
return (Getaccessiblegmbaccounts) super.setOauthToken(oauthToken);
}
@Override
public Getaccessiblegmbaccounts setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Getaccessiblegmbaccounts) super.setPrettyPrint(prettyPrint);
}
@Override
public Getaccessiblegmbaccounts setQuotaUser(java.lang.String quotaUser) {
return (Getaccessiblegmbaccounts) super.setQuotaUser(quotaUser);
}
@Override
public Getaccessiblegmbaccounts setUserIp(java.lang.String userIp) {
return (Getaccessiblegmbaccounts) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Getaccessiblegmbaccounts setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to retrieve accessible Google My Business accounts. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to retrieve accessible Google My Business accounts.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to retrieve accessible Google My Business accounts. */
public Getaccessiblegmbaccounts setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
@Override
public Getaccessiblegmbaccounts set(String parameterName, Object value) {
return (Getaccessiblegmbaccounts) super.set(parameterName, value);
}
}
/**
* Lists the LIA settings of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "liasettings.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings";
/**
* Lists the LIA settings of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "liasettings.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.LiasettingsListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the managing account. This must be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. This must be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. This must be a multi-client account. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The maximum number of LIA settings to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of LIA settings to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of LIA settings to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the LIA settings of the account. This method supports patch semantics.
*
* Create a request for the method "liasettings.patch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get or update LIA settings.
* @param content the {@link com.google.api.services.content.model.LiaSettings}
* @return the request
*/
public Patch patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.LiaSettings content) throws java.io.IOException {
Patch result = new Patch(merchantId, accountId, content);
initialize(result);
return result;
}
public class Patch extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings/{accountId}";
/**
* Updates the LIA settings of the account. This method supports patch semantics.
*
* Create a request for the method "liasettings.patch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get or update LIA settings.
* @param content the {@link com.google.api.services.content.model.LiaSettings}
* @since 1.13
*/
protected Patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.LiaSettings content) {
super(ShoppingContent.this, "PATCH", REST_PATH, content, com.google.api.services.content.model.LiaSettings.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Patch setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get or update LIA settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get or update LIA settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get or update LIA settings. */
public Patch setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Patch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Requests access to a specified Google My Business account.
*
* Create a request for the method "liasettings.requestgmbaccess".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Requestgmbaccess#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which GMB access is requested.
* @return the request
*/
public Requestgmbaccess requestgmbaccess(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Requestgmbaccess result = new Requestgmbaccess(merchantId, accountId);
initialize(result);
return result;
}
public class Requestgmbaccess extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings/{accountId}/requestgmbaccess";
/**
* Requests access to a specified Google My Business account.
*
* Create a request for the method "liasettings.requestgmbaccess".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Requestgmbaccess#execute()} method to invoke the remote operation.
* {@link Requestgmbaccess#initialize(com.google.api.client.googleapis.services.AbstractGoogle
* ClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which GMB access is requested.
* @since 1.13
*/
protected Requestgmbaccess(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "POST", REST_PATH, null, com.google.api.services.content.model.LiasettingsRequestGmbAccessResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Requestgmbaccess setAlt(java.lang.String alt) {
return (Requestgmbaccess) super.setAlt(alt);
}
@Override
public Requestgmbaccess setFields(java.lang.String fields) {
return (Requestgmbaccess) super.setFields(fields);
}
@Override
public Requestgmbaccess setKey(java.lang.String key) {
return (Requestgmbaccess) super.setKey(key);
}
@Override
public Requestgmbaccess setOauthToken(java.lang.String oauthToken) {
return (Requestgmbaccess) super.setOauthToken(oauthToken);
}
@Override
public Requestgmbaccess setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Requestgmbaccess) super.setPrettyPrint(prettyPrint);
}
@Override
public Requestgmbaccess setQuotaUser(java.lang.String quotaUser) {
return (Requestgmbaccess) super.setQuotaUser(quotaUser);
}
@Override
public Requestgmbaccess setUserIp(java.lang.String userIp) {
return (Requestgmbaccess) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Requestgmbaccess setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which GMB access is requested. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which GMB access is requested.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which GMB access is requested. */
public Requestgmbaccess setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** The email of the Google My Business account. */
@com.google.api.client.util.Key
private java.lang.String gmbEmail;
/** The email of the Google My Business account.
*/
public java.lang.String getGmbEmail() {
return gmbEmail;
}
/** The email of the Google My Business account. */
public Requestgmbaccess setGmbEmail(java.lang.String gmbEmail) {
this.gmbEmail = gmbEmail;
return this;
}
@Override
public Requestgmbaccess set(String parameterName, Object value) {
return (Requestgmbaccess) super.set(parameterName, value);
}
}
/**
* Requests inventory validation for the specified country.
*
* Create a request for the method "liasettings.requestinventoryverification".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Requestinventoryverification#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account that manages the order. This cannot be a multi-client account.
* @param country The country for which inventory validation is requested.
* @return the request
*/
public Requestinventoryverification requestinventoryverification(java.math.BigInteger merchantId, java.math.BigInteger accountId, java.lang.String country) throws java.io.IOException {
Requestinventoryverification result = new Requestinventoryverification(merchantId, accountId, country);
initialize(result);
return result;
}
public class Requestinventoryverification extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings/{accountId}/requestinventoryverification/{country}";
/**
* Requests inventory validation for the specified country.
*
* Create a request for the method "liasettings.requestinventoryverification".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Requestinventoryverification#execute()} method to invoke the remote
* operation. {@link Requestinventoryverification#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account that manages the order. This cannot be a multi-client account.
* @param country The country for which inventory validation is requested.
* @since 1.13
*/
protected Requestinventoryverification(java.math.BigInteger merchantId, java.math.BigInteger accountId, java.lang.String country) {
super(ShoppingContent.this, "POST", REST_PATH, null, com.google.api.services.content.model.LiasettingsRequestInventoryVerificationResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.country = com.google.api.client.util.Preconditions.checkNotNull(country, "Required parameter country must be specified.");
}
@Override
public Requestinventoryverification setAlt(java.lang.String alt) {
return (Requestinventoryverification) super.setAlt(alt);
}
@Override
public Requestinventoryverification setFields(java.lang.String fields) {
return (Requestinventoryverification) super.setFields(fields);
}
@Override
public Requestinventoryverification setKey(java.lang.String key) {
return (Requestinventoryverification) super.setKey(key);
}
@Override
public Requestinventoryverification setOauthToken(java.lang.String oauthToken) {
return (Requestinventoryverification) super.setOauthToken(oauthToken);
}
@Override
public Requestinventoryverification setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Requestinventoryverification) super.setPrettyPrint(prettyPrint);
}
@Override
public Requestinventoryverification setQuotaUser(java.lang.String quotaUser) {
return (Requestinventoryverification) super.setQuotaUser(quotaUser);
}
@Override
public Requestinventoryverification setUserIp(java.lang.String userIp) {
return (Requestinventoryverification) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Requestinventoryverification setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Requestinventoryverification setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** The country for which inventory validation is requested. */
@com.google.api.client.util.Key
private java.lang.String country;
/** The country for which inventory validation is requested.
*/
public java.lang.String getCountry() {
return country;
}
/** The country for which inventory validation is requested. */
public Requestinventoryverification setCountry(java.lang.String country) {
this.country = country;
return this;
}
@Override
public Requestinventoryverification set(String parameterName, Object value) {
return (Requestinventoryverification) super.set(parameterName, value);
}
}
/**
* Sets the inventory verification contract for the specified country.
*
* Create a request for the method "liasettings.setinventoryverificationcontact".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Setinventoryverificationcontact#execute()} method to invoke the
* remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account that manages the order. This cannot be a multi-client account.
* @return the request
*/
public Setinventoryverificationcontact setinventoryverificationcontact(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Setinventoryverificationcontact result = new Setinventoryverificationcontact(merchantId, accountId);
initialize(result);
return result;
}
public class Setinventoryverificationcontact extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings/{accountId}/setinventoryverificationcontact";
/**
* Sets the inventory verification contract for the specified country.
*
* Create a request for the method "liasettings.setinventoryverificationcontact".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Setinventoryverificationcontact#execute()} method to invoke the
* remote operation. {@link Setinventoryverificationcontact#initialize(com.google.api.client.g
* oogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account that manages the order. This cannot be a multi-client account.
* @since 1.13
*/
protected Setinventoryverificationcontact(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "POST", REST_PATH, null, com.google.api.services.content.model.LiasettingsSetInventoryVerificationContactResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Setinventoryverificationcontact setAlt(java.lang.String alt) {
return (Setinventoryverificationcontact) super.setAlt(alt);
}
@Override
public Setinventoryverificationcontact setFields(java.lang.String fields) {
return (Setinventoryverificationcontact) super.setFields(fields);
}
@Override
public Setinventoryverificationcontact setKey(java.lang.String key) {
return (Setinventoryverificationcontact) super.setKey(key);
}
@Override
public Setinventoryverificationcontact setOauthToken(java.lang.String oauthToken) {
return (Setinventoryverificationcontact) super.setOauthToken(oauthToken);
}
@Override
public Setinventoryverificationcontact setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Setinventoryverificationcontact) super.setPrettyPrint(prettyPrint);
}
@Override
public Setinventoryverificationcontact setQuotaUser(java.lang.String quotaUser) {
return (Setinventoryverificationcontact) super.setQuotaUser(quotaUser);
}
@Override
public Setinventoryverificationcontact setUserIp(java.lang.String userIp) {
return (Setinventoryverificationcontact) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Setinventoryverificationcontact setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Setinventoryverificationcontact setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** The email of the inventory verification contact. */
@com.google.api.client.util.Key
private java.lang.String contactEmail;
/** The email of the inventory verification contact.
*/
public java.lang.String getContactEmail() {
return contactEmail;
}
/** The email of the inventory verification contact. */
public Setinventoryverificationcontact setContactEmail(java.lang.String contactEmail) {
this.contactEmail = contactEmail;
return this;
}
/** The name of the inventory verification contact. */
@com.google.api.client.util.Key
private java.lang.String contactName;
/** The name of the inventory verification contact.
*/
public java.lang.String getContactName() {
return contactName;
}
/** The name of the inventory verification contact. */
public Setinventoryverificationcontact setContactName(java.lang.String contactName) {
this.contactName = contactName;
return this;
}
/** The country for which inventory verification is requested. */
@com.google.api.client.util.Key
private java.lang.String country;
/** The country for which inventory verification is requested.
*/
public java.lang.String getCountry() {
return country;
}
/** The country for which inventory verification is requested. */
public Setinventoryverificationcontact setCountry(java.lang.String country) {
this.country = country;
return this;
}
/** The language for which inventory verification is requested. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The language for which inventory verification is requested.
*/
public java.lang.String getLanguage() {
return language;
}
/** The language for which inventory verification is requested. */
public Setinventoryverificationcontact setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Setinventoryverificationcontact set(String parameterName, Object value) {
return (Setinventoryverificationcontact) super.set(parameterName, value);
}
}
/**
* Updates the LIA settings of the account.
*
* Create a request for the method "liasettings.update".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get or update LIA settings.
* @param content the {@link com.google.api.services.content.model.LiaSettings}
* @return the request
*/
public Update update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.LiaSettings content) throws java.io.IOException {
Update result = new Update(merchantId, accountId, content);
initialize(result);
return result;
}
public class Update extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/liasettings/{accountId}";
/**
* Updates the LIA settings of the account.
*
* Create a request for the method "liasettings.update".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get or update LIA settings.
* @param content the {@link com.google.api.services.content.model.LiaSettings}
* @since 1.13
*/
protected Update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.LiaSettings content) {
super(ShoppingContent.this, "PUT", REST_PATH, content, com.google.api.services.content.model.LiaSettings.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Update setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get or update LIA settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get or update LIA settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get or update LIA settings. */
public Update setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Update setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Orders collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Orders.List request = content.orders().list(parameters ...)}
*
*
* @return the resource collection
*/
public Orders orders() {
return new Orders();
}
/**
* The "orders" collection of methods.
*/
public class Orders {
/**
* Marks an order as acknowledged.
*
* Create a request for the method "orders.acknowledge".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Acknowledge#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersAcknowledgeRequest}
* @return the request
*/
public Acknowledge acknowledge(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersAcknowledgeRequest content) throws java.io.IOException {
Acknowledge result = new Acknowledge(merchantId, orderId, content);
initialize(result);
return result;
}
public class Acknowledge extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/acknowledge";
/**
* Marks an order as acknowledged.
*
* Create a request for the method "orders.acknowledge".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Acknowledge#execute()} method to invoke the remote operation.
* {@link
* Acknowledge#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersAcknowledgeRequest}
* @since 1.13
*/
protected Acknowledge(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersAcknowledgeRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersAcknowledgeResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Acknowledge setAlt(java.lang.String alt) {
return (Acknowledge) super.setAlt(alt);
}
@Override
public Acknowledge setFields(java.lang.String fields) {
return (Acknowledge) super.setFields(fields);
}
@Override
public Acknowledge setKey(java.lang.String key) {
return (Acknowledge) super.setKey(key);
}
@Override
public Acknowledge setOauthToken(java.lang.String oauthToken) {
return (Acknowledge) super.setOauthToken(oauthToken);
}
@Override
public Acknowledge setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Acknowledge) super.setPrettyPrint(prettyPrint);
}
@Override
public Acknowledge setQuotaUser(java.lang.String quotaUser) {
return (Acknowledge) super.setQuotaUser(quotaUser);
}
@Override
public Acknowledge setUserIp(java.lang.String userIp) {
return (Acknowledge) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Acknowledge setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Acknowledge setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Acknowledge set(String parameterName, Object value) {
return (Acknowledge) super.set(parameterName, value);
}
}
/**
* Sandbox only. Moves a test order from state "inProgress" to state "pendingShipment".
*
* Create a request for the method "orders.advancetestorder".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Advancetestorder#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the test order to modify.
* @return the request
*/
public Advancetestorder advancetestorder(java.math.BigInteger merchantId, java.lang.String orderId) throws java.io.IOException {
Advancetestorder result = new Advancetestorder(merchantId, orderId);
initialize(result);
return result;
}
public class Advancetestorder extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/testorders/{orderId}/advance";
/**
* Sandbox only. Moves a test order from state "inProgress" to state "pendingShipment".
*
* Create a request for the method "orders.advancetestorder".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Advancetestorder#execute()} method to invoke the remote operation.
* {@link Advancetestorder#initialize(com.google.api.client.googleapis.services.AbstractGoogle
* ClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the test order to modify.
* @since 1.13
*/
protected Advancetestorder(java.math.BigInteger merchantId, java.lang.String orderId) {
super(ShoppingContent.this, "POST", REST_PATH, null, com.google.api.services.content.model.OrdersAdvanceTestOrderResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Advancetestorder setAlt(java.lang.String alt) {
return (Advancetestorder) super.setAlt(alt);
}
@Override
public Advancetestorder setFields(java.lang.String fields) {
return (Advancetestorder) super.setFields(fields);
}
@Override
public Advancetestorder setKey(java.lang.String key) {
return (Advancetestorder) super.setKey(key);
}
@Override
public Advancetestorder setOauthToken(java.lang.String oauthToken) {
return (Advancetestorder) super.setOauthToken(oauthToken);
}
@Override
public Advancetestorder setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Advancetestorder) super.setPrettyPrint(prettyPrint);
}
@Override
public Advancetestorder setQuotaUser(java.lang.String quotaUser) {
return (Advancetestorder) super.setQuotaUser(quotaUser);
}
@Override
public Advancetestorder setUserIp(java.lang.String userIp) {
return (Advancetestorder) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Advancetestorder setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the test order to modify. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the test order to modify.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the test order to modify. */
public Advancetestorder setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Advancetestorder set(String parameterName, Object value) {
return (Advancetestorder) super.set(parameterName, value);
}
}
/**
* Cancels all line items in an order, making a full refund.
*
* Create a request for the method "orders.cancel".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order to cancel.
* @param content the {@link com.google.api.services.content.model.OrdersCancelRequest}
* @return the request
*/
public Cancel cancel(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelRequest content) throws java.io.IOException {
Cancel result = new Cancel(merchantId, orderId, content);
initialize(result);
return result;
}
public class Cancel extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/cancel";
/**
* Cancels all line items in an order, making a full refund.
*
* Create a request for the method "orders.cancel".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order to cancel.
* @param content the {@link com.google.api.services.content.model.OrdersCancelRequest}
* @since 1.13
*/
protected Cancel(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCancelResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUserIp(java.lang.String userIp) {
return (Cancel) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Cancel setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order to cancel. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order to cancel.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order to cancel. */
public Cancel setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Cancels a line item, making a full refund.
*
* Create a request for the method "orders.cancellineitem".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Cancellineitem#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersCancelLineItemRequest}
* @return the request
*/
public Cancellineitem cancellineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelLineItemRequest content) throws java.io.IOException {
Cancellineitem result = new Cancellineitem(merchantId, orderId, content);
initialize(result);
return result;
}
public class Cancellineitem extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/cancelLineItem";
/**
* Cancels a line item, making a full refund.
*
* Create a request for the method "orders.cancellineitem".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Cancellineitem#execute()} method to invoke the remote operation.
* {@link Cancellineitem#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersCancelLineItemRequest}
* @since 1.13
*/
protected Cancellineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelLineItemRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCancelLineItemResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Cancellineitem setAlt(java.lang.String alt) {
return (Cancellineitem) super.setAlt(alt);
}
@Override
public Cancellineitem setFields(java.lang.String fields) {
return (Cancellineitem) super.setFields(fields);
}
@Override
public Cancellineitem setKey(java.lang.String key) {
return (Cancellineitem) super.setKey(key);
}
@Override
public Cancellineitem setOauthToken(java.lang.String oauthToken) {
return (Cancellineitem) super.setOauthToken(oauthToken);
}
@Override
public Cancellineitem setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancellineitem) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancellineitem setQuotaUser(java.lang.String quotaUser) {
return (Cancellineitem) super.setQuotaUser(quotaUser);
}
@Override
public Cancellineitem setUserIp(java.lang.String userIp) {
return (Cancellineitem) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Cancellineitem setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Cancellineitem setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Cancellineitem set(String parameterName, Object value) {
return (Cancellineitem) super.set(parameterName, value);
}
}
/**
* Sandbox only. Creates a test order.
*
* Create a request for the method "orders.createtestorder".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Createtestorder#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that should manage the order. This cannot be a multi-client account.
* @param content the {@link com.google.api.services.content.model.OrdersCreateTestOrderRequest}
* @return the request
*/
public Createtestorder createtestorder(java.math.BigInteger merchantId, com.google.api.services.content.model.OrdersCreateTestOrderRequest content) throws java.io.IOException {
Createtestorder result = new Createtestorder(merchantId, content);
initialize(result);
return result;
}
public class Createtestorder extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/testorders";
/**
* Sandbox only. Creates a test order.
*
* Create a request for the method "orders.createtestorder".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Createtestorder#execute()} method to invoke the remote operation.
* {@link Createtestorder#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the account that should manage the order. This cannot be a multi-client account.
* @param content the {@link com.google.api.services.content.model.OrdersCreateTestOrderRequest}
* @since 1.13
*/
protected Createtestorder(java.math.BigInteger merchantId, com.google.api.services.content.model.OrdersCreateTestOrderRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCreateTestOrderResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public Createtestorder setAlt(java.lang.String alt) {
return (Createtestorder) super.setAlt(alt);
}
@Override
public Createtestorder setFields(java.lang.String fields) {
return (Createtestorder) super.setFields(fields);
}
@Override
public Createtestorder setKey(java.lang.String key) {
return (Createtestorder) super.setKey(key);
}
@Override
public Createtestorder setOauthToken(java.lang.String oauthToken) {
return (Createtestorder) super.setOauthToken(oauthToken);
}
@Override
public Createtestorder setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Createtestorder) super.setPrettyPrint(prettyPrint);
}
@Override
public Createtestorder setQuotaUser(java.lang.String quotaUser) {
return (Createtestorder) super.setQuotaUser(quotaUser);
}
@Override
public Createtestorder setUserIp(java.lang.String userIp) {
return (Createtestorder) super.setUserIp(userIp);
}
/**
* The ID of the account that should manage the order. This cannot be a multi-client account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that should manage the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that should manage the order. This cannot be a multi-client account.
*/
public Createtestorder setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
@Override
public Createtestorder set(String parameterName, Object value) {
return (Createtestorder) super.set(parameterName, value);
}
}
/**
* Retrieves or modifies multiple orders in a single request.
*
* Create a request for the method "orders.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.OrdersCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.OrdersCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "orders/batch";
/**
* Retrieves or modifies multiple orders in a single request.
*
* Create a request for the method "orders.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.OrdersCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.OrdersCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Retrieves an order from your Merchant Center account.
*
* Create a request for the method "orders.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.lang.String orderId) throws java.io.IOException {
Get result = new Get(merchantId, orderId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}";
/**
* Retrieves an order from your Merchant Center account.
*
* Create a request for the method "orders.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.lang.String orderId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.Order.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Get setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves an order using merchant order id.
*
* Create a request for the method "orders.getbymerchantorderid".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Getbymerchantorderid#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param merchantOrderId The merchant order id to be looked for.
* @return the request
*/
public Getbymerchantorderid getbymerchantorderid(java.math.BigInteger merchantId, java.lang.String merchantOrderId) throws java.io.IOException {
Getbymerchantorderid result = new Getbymerchantorderid(merchantId, merchantOrderId);
initialize(result);
return result;
}
public class Getbymerchantorderid extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/ordersbymerchantid/{merchantOrderId}";
/**
* Retrieves an order using merchant order id.
*
* Create a request for the method "orders.getbymerchantorderid".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Getbymerchantorderid#execute()} method to invoke the remote
* operation. {@link Getbymerchantorderid#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param merchantOrderId The merchant order id to be looked for.
* @since 1.13
*/
protected Getbymerchantorderid(java.math.BigInteger merchantId, java.lang.String merchantOrderId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.OrdersGetByMerchantOrderIdResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.merchantOrderId = com.google.api.client.util.Preconditions.checkNotNull(merchantOrderId, "Required parameter merchantOrderId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Getbymerchantorderid setAlt(java.lang.String alt) {
return (Getbymerchantorderid) super.setAlt(alt);
}
@Override
public Getbymerchantorderid setFields(java.lang.String fields) {
return (Getbymerchantorderid) super.setFields(fields);
}
@Override
public Getbymerchantorderid setKey(java.lang.String key) {
return (Getbymerchantorderid) super.setKey(key);
}
@Override
public Getbymerchantorderid setOauthToken(java.lang.String oauthToken) {
return (Getbymerchantorderid) super.setOauthToken(oauthToken);
}
@Override
public Getbymerchantorderid setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Getbymerchantorderid) super.setPrettyPrint(prettyPrint);
}
@Override
public Getbymerchantorderid setQuotaUser(java.lang.String quotaUser) {
return (Getbymerchantorderid) super.setQuotaUser(quotaUser);
}
@Override
public Getbymerchantorderid setUserIp(java.lang.String userIp) {
return (Getbymerchantorderid) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Getbymerchantorderid setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The merchant order id to be looked for. */
@com.google.api.client.util.Key
private java.lang.String merchantOrderId;
/** The merchant order id to be looked for.
*/
public java.lang.String getMerchantOrderId() {
return merchantOrderId;
}
/** The merchant order id to be looked for. */
public Getbymerchantorderid setMerchantOrderId(java.lang.String merchantOrderId) {
this.merchantOrderId = merchantOrderId;
return this;
}
@Override
public Getbymerchantorderid set(String parameterName, Object value) {
return (Getbymerchantorderid) super.set(parameterName, value);
}
}
/**
* Sandbox only. Retrieves an order template that can be used to quickly create a new order in
* sandbox.
*
* Create a request for the method "orders.gettestordertemplate".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Gettestordertemplate#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account that should manage the order. This cannot be a multi-client account.
* @param templateName The name of the template to retrieve.
* @return the request
*/
public Gettestordertemplate gettestordertemplate(java.math.BigInteger merchantId, java.lang.String templateName) throws java.io.IOException {
Gettestordertemplate result = new Gettestordertemplate(merchantId, templateName);
initialize(result);
return result;
}
public class Gettestordertemplate extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/testordertemplates/{templateName}";
/**
* Sandbox only. Retrieves an order template that can be used to quickly create a new order in
* sandbox.
*
* Create a request for the method "orders.gettestordertemplate".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Gettestordertemplate#execute()} method to invoke the remote
* operation. {@link Gettestordertemplate#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account that should manage the order. This cannot be a multi-client account.
* @param templateName The name of the template to retrieve.
* @since 1.13
*/
protected Gettestordertemplate(java.math.BigInteger merchantId, java.lang.String templateName) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.OrdersGetTestOrderTemplateResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.templateName = com.google.api.client.util.Preconditions.checkNotNull(templateName, "Required parameter templateName must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Gettestordertemplate setAlt(java.lang.String alt) {
return (Gettestordertemplate) super.setAlt(alt);
}
@Override
public Gettestordertemplate setFields(java.lang.String fields) {
return (Gettestordertemplate) super.setFields(fields);
}
@Override
public Gettestordertemplate setKey(java.lang.String key) {
return (Gettestordertemplate) super.setKey(key);
}
@Override
public Gettestordertemplate setOauthToken(java.lang.String oauthToken) {
return (Gettestordertemplate) super.setOauthToken(oauthToken);
}
@Override
public Gettestordertemplate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Gettestordertemplate) super.setPrettyPrint(prettyPrint);
}
@Override
public Gettestordertemplate setQuotaUser(java.lang.String quotaUser) {
return (Gettestordertemplate) super.setQuotaUser(quotaUser);
}
@Override
public Gettestordertemplate setUserIp(java.lang.String userIp) {
return (Gettestordertemplate) super.setUserIp(userIp);
}
/**
* The ID of the account that should manage the order. This cannot be a multi-client account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that should manage the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that should manage the order. This cannot be a multi-client account.
*/
public Gettestordertemplate setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The name of the template to retrieve. */
@com.google.api.client.util.Key
private java.lang.String templateName;
/** The name of the template to retrieve.
*/
public java.lang.String getTemplateName() {
return templateName;
}
/** The name of the template to retrieve. */
public Gettestordertemplate setTemplateName(java.lang.String templateName) {
this.templateName = templateName;
return this;
}
@Override
public Gettestordertemplate set(String parameterName, Object value) {
return (Gettestordertemplate) super.set(parameterName, value);
}
}
/**
* Notifies that item return and refund was handled directly in store.
*
* Create a request for the method "orders.instorerefundlineitem".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Instorerefundlineitem#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersInStoreRefundLineItemRequest}
* @return the request
*/
public Instorerefundlineitem instorerefundlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersInStoreRefundLineItemRequest content) throws java.io.IOException {
Instorerefundlineitem result = new Instorerefundlineitem(merchantId, orderId, content);
initialize(result);
return result;
}
public class Instorerefundlineitem extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/inStoreRefundLineItem";
/**
* Notifies that item return and refund was handled directly in store.
*
* Create a request for the method "orders.instorerefundlineitem".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Instorerefundlineitem#execute()} method to invoke the remote
* operation. {@link Instorerefundlineitem#initialize(com.google.api.client.googleapis.service
* s.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersInStoreRefundLineItemRequest}
* @since 1.13
*/
protected Instorerefundlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersInStoreRefundLineItemRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersInStoreRefundLineItemResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Instorerefundlineitem setAlt(java.lang.String alt) {
return (Instorerefundlineitem) super.setAlt(alt);
}
@Override
public Instorerefundlineitem setFields(java.lang.String fields) {
return (Instorerefundlineitem) super.setFields(fields);
}
@Override
public Instorerefundlineitem setKey(java.lang.String key) {
return (Instorerefundlineitem) super.setKey(key);
}
@Override
public Instorerefundlineitem setOauthToken(java.lang.String oauthToken) {
return (Instorerefundlineitem) super.setOauthToken(oauthToken);
}
@Override
public Instorerefundlineitem setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Instorerefundlineitem) super.setPrettyPrint(prettyPrint);
}
@Override
public Instorerefundlineitem setQuotaUser(java.lang.String quotaUser) {
return (Instorerefundlineitem) super.setQuotaUser(quotaUser);
}
@Override
public Instorerefundlineitem setUserIp(java.lang.String userIp) {
return (Instorerefundlineitem) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Instorerefundlineitem setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Instorerefundlineitem setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Instorerefundlineitem set(String parameterName, Object value) {
return (Instorerefundlineitem) super.set(parameterName, value);
}
}
/**
* Lists the orders in your Merchant Center account.
*
* Create a request for the method "orders.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders";
/**
* Lists the orders in your Merchant Center account.
*
* Create a request for the method "orders.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.OrdersListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* Obtains orders that match the acknowledgement status. When set to true, obtains orders that
* have been acknowledged. When false, obtains orders that have not been acknowledged. We
* recommend using this filter set to false, in conjunction with the acknowledge call, such
* that only un-acknowledged orders are returned.
*/
@com.google.api.client.util.Key
private java.lang.Boolean acknowledged;
/** Obtains orders that match the acknowledgement status. When set to true, obtains orders that have
been acknowledged. When false, obtains orders that have not been acknowledged. We recommend using
this filter set to false, in conjunction with the acknowledge call, such that only un-acknowledged
orders are returned.
*/
public java.lang.Boolean getAcknowledged() {
return acknowledged;
}
/**
* Obtains orders that match the acknowledgement status. When set to true, obtains orders that
* have been acknowledged. When false, obtains orders that have not been acknowledged. We
* recommend using this filter set to false, in conjunction with the acknowledge call, such
* that only un-acknowledged orders are returned.
*/
public List setAcknowledged(java.lang.Boolean acknowledged) {
this.acknowledged = acknowledged;
return this;
}
/**
* The maximum number of orders to return in the response, used for paging. The default value
* is 25 orders per page, and the maximum allowed value is 250 orders per page. Known issue:
* All List calls will return all Orders without limit regardless of the value of this field.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of orders to return in the response, used for paging. The default value is 25
orders per page, and the maximum allowed value is 250 orders per page. Known issue: All List calls
will return all Orders without limit regardless of the value of this field.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of orders to return in the response, used for paging. The default value
* is 25 orders per page, and the maximum allowed value is 250 orders per page. Known issue:
* All List calls will return all Orders without limit regardless of the value of this field.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The ordering of the returned list. The only supported value are placedDate desc and
* placedDate asc for now, which returns orders sorted by placement date. "placedDate desc"
* stands for listing orders by placement date, from oldest to most recent. "placedDate asc"
* stands for listing orders by placement date, from most recent to oldest. In future releases
* we'll support other sorting criteria.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** The ordering of the returned list. The only supported value are placedDate desc and placedDate asc
for now, which returns orders sorted by placement date. "placedDate desc" stands for listing orders
by placement date, from oldest to most recent. "placedDate asc" stands for listing orders by
placement date, from most recent to oldest. In future releases we'll support other sorting
criteria.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* The ordering of the returned list. The only supported value are placedDate desc and
* placedDate asc for now, which returns orders sorted by placement date. "placedDate desc"
* stands for listing orders by placement date, from oldest to most recent. "placedDate asc"
* stands for listing orders by placement date, from most recent to oldest. In future releases
* we'll support other sorting criteria.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Obtains orders placed before this date (exclusively), in ISO 8601 format. */
@com.google.api.client.util.Key
private java.lang.String placedDateEnd;
/** Obtains orders placed before this date (exclusively), in ISO 8601 format.
*/
public java.lang.String getPlacedDateEnd() {
return placedDateEnd;
}
/** Obtains orders placed before this date (exclusively), in ISO 8601 format. */
public List setPlacedDateEnd(java.lang.String placedDateEnd) {
this.placedDateEnd = placedDateEnd;
return this;
}
/** Obtains orders placed after this date (inclusively), in ISO 8601 format. */
@com.google.api.client.util.Key
private java.lang.String placedDateStart;
/** Obtains orders placed after this date (inclusively), in ISO 8601 format.
*/
public java.lang.String getPlacedDateStart() {
return placedDateStart;
}
/** Obtains orders placed after this date (inclusively), in ISO 8601 format. */
public List setPlacedDateStart(java.lang.String placedDateStart) {
this.placedDateStart = placedDateStart;
return this;
}
/**
* Obtains orders that match any of the specified statuses. Multiple values can be specified
* with comma separation. Additionally, please note that active is a shortcut for
* pendingShipment and partiallyShipped, and completed is a shortcut for shipped ,
* partiallyDelivered, delivered, partiallyReturned, returned, and canceled.
*/
@com.google.api.client.util.Key
private java.util.List statuses;
/** Obtains orders that match any of the specified statuses. Multiple values can be specified with
comma separation. Additionally, please note that active is a shortcut for pendingShipment and
partiallyShipped, and completed is a shortcut for shipped , partiallyDelivered, delivered,
partiallyReturned, returned, and canceled.
*/
public java.util.List getStatuses() {
return statuses;
}
/**
* Obtains orders that match any of the specified statuses. Multiple values can be specified
* with comma separation. Additionally, please note that active is a shortcut for
* pendingShipment and partiallyShipped, and completed is a shortcut for shipped ,
* partiallyDelivered, delivered, partiallyReturned, returned, and canceled.
*/
public List setStatuses(java.util.List statuses) {
this.statuses = statuses;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Refund a portion of the order, up to the full amount paid.
*
* Create a request for the method "orders.refund".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Refund#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order to refund.
* @param content the {@link com.google.api.services.content.model.OrdersRefundRequest}
* @return the request
*/
public Refund refund(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersRefundRequest content) throws java.io.IOException {
Refund result = new Refund(merchantId, orderId, content);
initialize(result);
return result;
}
public class Refund extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/refund";
/**
* Refund a portion of the order, up to the full amount paid.
*
* Create a request for the method "orders.refund".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Refund#execute()} method to invoke the remote operation. {@link
* Refund#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order to refund.
* @param content the {@link com.google.api.services.content.model.OrdersRefundRequest}
* @since 1.13
*/
protected Refund(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersRefundRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersRefundResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Refund setAlt(java.lang.String alt) {
return (Refund) super.setAlt(alt);
}
@Override
public Refund setFields(java.lang.String fields) {
return (Refund) super.setFields(fields);
}
@Override
public Refund setKey(java.lang.String key) {
return (Refund) super.setKey(key);
}
@Override
public Refund setOauthToken(java.lang.String oauthToken) {
return (Refund) super.setOauthToken(oauthToken);
}
@Override
public Refund setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Refund) super.setPrettyPrint(prettyPrint);
}
@Override
public Refund setQuotaUser(java.lang.String quotaUser) {
return (Refund) super.setQuotaUser(quotaUser);
}
@Override
public Refund setUserIp(java.lang.String userIp) {
return (Refund) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Refund setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order to refund. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order to refund.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order to refund. */
public Refund setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Refund set(String parameterName, Object value) {
return (Refund) super.set(parameterName, value);
}
}
/**
* Rejects return on an line item.
*
* Create a request for the method "orders.rejectreturnlineitem".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Rejectreturnlineitem#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersRejectReturnLineItemRequest}
* @return the request
*/
public Rejectreturnlineitem rejectreturnlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersRejectReturnLineItemRequest content) throws java.io.IOException {
Rejectreturnlineitem result = new Rejectreturnlineitem(merchantId, orderId, content);
initialize(result);
return result;
}
public class Rejectreturnlineitem extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/rejectReturnLineItem";
/**
* Rejects return on an line item.
*
* Create a request for the method "orders.rejectreturnlineitem".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Rejectreturnlineitem#execute()} method to invoke the remote
* operation. {@link Rejectreturnlineitem#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersRejectReturnLineItemRequest}
* @since 1.13
*/
protected Rejectreturnlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersRejectReturnLineItemRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersRejectReturnLineItemResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Rejectreturnlineitem setAlt(java.lang.String alt) {
return (Rejectreturnlineitem) super.setAlt(alt);
}
@Override
public Rejectreturnlineitem setFields(java.lang.String fields) {
return (Rejectreturnlineitem) super.setFields(fields);
}
@Override
public Rejectreturnlineitem setKey(java.lang.String key) {
return (Rejectreturnlineitem) super.setKey(key);
}
@Override
public Rejectreturnlineitem setOauthToken(java.lang.String oauthToken) {
return (Rejectreturnlineitem) super.setOauthToken(oauthToken);
}
@Override
public Rejectreturnlineitem setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rejectreturnlineitem) super.setPrettyPrint(prettyPrint);
}
@Override
public Rejectreturnlineitem setQuotaUser(java.lang.String quotaUser) {
return (Rejectreturnlineitem) super.setQuotaUser(quotaUser);
}
@Override
public Rejectreturnlineitem setUserIp(java.lang.String userIp) {
return (Rejectreturnlineitem) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Rejectreturnlineitem setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Rejectreturnlineitem setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Rejectreturnlineitem set(String parameterName, Object value) {
return (Rejectreturnlineitem) super.set(parameterName, value);
}
}
/**
* Returns a line item.
*
* Create a request for the method "orders.returnlineitem".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Returnlineitem#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersReturnLineItemRequest}
* @return the request
*/
public Returnlineitem returnlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersReturnLineItemRequest content) throws java.io.IOException {
Returnlineitem result = new Returnlineitem(merchantId, orderId, content);
initialize(result);
return result;
}
public class Returnlineitem extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/returnLineItem";
/**
* Returns a line item.
*
* Create a request for the method "orders.returnlineitem".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Returnlineitem#execute()} method to invoke the remote operation.
* {@link Returnlineitem#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersReturnLineItemRequest}
* @since 1.13
*/
protected Returnlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersReturnLineItemRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersReturnLineItemResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Returnlineitem setAlt(java.lang.String alt) {
return (Returnlineitem) super.setAlt(alt);
}
@Override
public Returnlineitem setFields(java.lang.String fields) {
return (Returnlineitem) super.setFields(fields);
}
@Override
public Returnlineitem setKey(java.lang.String key) {
return (Returnlineitem) super.setKey(key);
}
@Override
public Returnlineitem setOauthToken(java.lang.String oauthToken) {
return (Returnlineitem) super.setOauthToken(oauthToken);
}
@Override
public Returnlineitem setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Returnlineitem) super.setPrettyPrint(prettyPrint);
}
@Override
public Returnlineitem setQuotaUser(java.lang.String quotaUser) {
return (Returnlineitem) super.setQuotaUser(quotaUser);
}
@Override
public Returnlineitem setUserIp(java.lang.String userIp) {
return (Returnlineitem) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Returnlineitem setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Returnlineitem setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Returnlineitem set(String parameterName, Object value) {
return (Returnlineitem) super.set(parameterName, value);
}
}
/**
* Returns and refunds a line item. Note that this method can only be called on fully shipped
* orders.
*
* Create a request for the method "orders.returnrefundlineitem".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Returnrefundlineitem#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersReturnRefundLineItemRequest}
* @return the request
*/
public Returnrefundlineitem returnrefundlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersReturnRefundLineItemRequest content) throws java.io.IOException {
Returnrefundlineitem result = new Returnrefundlineitem(merchantId, orderId, content);
initialize(result);
return result;
}
public class Returnrefundlineitem extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/returnRefundLineItem";
/**
* Returns and refunds a line item. Note that this method can only be called on fully shipped
* orders.
*
* Create a request for the method "orders.returnrefundlineitem".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Returnrefundlineitem#execute()} method to invoke the remote
* operation. {@link Returnrefundlineitem#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersReturnRefundLineItemRequest}
* @since 1.13
*/
protected Returnrefundlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersReturnRefundLineItemRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersReturnRefundLineItemResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Returnrefundlineitem setAlt(java.lang.String alt) {
return (Returnrefundlineitem) super.setAlt(alt);
}
@Override
public Returnrefundlineitem setFields(java.lang.String fields) {
return (Returnrefundlineitem) super.setFields(fields);
}
@Override
public Returnrefundlineitem setKey(java.lang.String key) {
return (Returnrefundlineitem) super.setKey(key);
}
@Override
public Returnrefundlineitem setOauthToken(java.lang.String oauthToken) {
return (Returnrefundlineitem) super.setOauthToken(oauthToken);
}
@Override
public Returnrefundlineitem setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Returnrefundlineitem) super.setPrettyPrint(prettyPrint);
}
@Override
public Returnrefundlineitem setQuotaUser(java.lang.String quotaUser) {
return (Returnrefundlineitem) super.setQuotaUser(quotaUser);
}
@Override
public Returnrefundlineitem setUserIp(java.lang.String userIp) {
return (Returnrefundlineitem) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Returnrefundlineitem setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Returnrefundlineitem setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Returnrefundlineitem set(String parameterName, Object value) {
return (Returnrefundlineitem) super.set(parameterName, value);
}
}
/**
* Sets (overrides) merchant provided annotations on the line item.
*
* Create a request for the method "orders.setlineitemmetadata".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Setlineitemmetadata#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersSetLineItemMetadataRequest}
* @return the request
*/
public Setlineitemmetadata setlineitemmetadata(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersSetLineItemMetadataRequest content) throws java.io.IOException {
Setlineitemmetadata result = new Setlineitemmetadata(merchantId, orderId, content);
initialize(result);
return result;
}
public class Setlineitemmetadata extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/setLineItemMetadata";
/**
* Sets (overrides) merchant provided annotations on the line item.
*
* Create a request for the method "orders.setlineitemmetadata".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Setlineitemmetadata#execute()} method to invoke the remote
* operation. {@link Setlineitemmetadata#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersSetLineItemMetadataRequest}
* @since 1.13
*/
protected Setlineitemmetadata(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersSetLineItemMetadataRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersSetLineItemMetadataResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Setlineitemmetadata setAlt(java.lang.String alt) {
return (Setlineitemmetadata) super.setAlt(alt);
}
@Override
public Setlineitemmetadata setFields(java.lang.String fields) {
return (Setlineitemmetadata) super.setFields(fields);
}
@Override
public Setlineitemmetadata setKey(java.lang.String key) {
return (Setlineitemmetadata) super.setKey(key);
}
@Override
public Setlineitemmetadata setOauthToken(java.lang.String oauthToken) {
return (Setlineitemmetadata) super.setOauthToken(oauthToken);
}
@Override
public Setlineitemmetadata setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Setlineitemmetadata) super.setPrettyPrint(prettyPrint);
}
@Override
public Setlineitemmetadata setQuotaUser(java.lang.String quotaUser) {
return (Setlineitemmetadata) super.setQuotaUser(quotaUser);
}
@Override
public Setlineitemmetadata setUserIp(java.lang.String userIp) {
return (Setlineitemmetadata) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Setlineitemmetadata setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Setlineitemmetadata setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Setlineitemmetadata set(String parameterName, Object value) {
return (Setlineitemmetadata) super.set(parameterName, value);
}
}
/**
* Marks line item(s) as shipped.
*
* Create a request for the method "orders.shiplineitems".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Shiplineitems#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersShipLineItemsRequest}
* @return the request
*/
public Shiplineitems shiplineitems(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersShipLineItemsRequest content) throws java.io.IOException {
Shiplineitems result = new Shiplineitems(merchantId, orderId, content);
initialize(result);
return result;
}
public class Shiplineitems extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/shipLineItems";
/**
* Marks line item(s) as shipped.
*
* Create a request for the method "orders.shiplineitems".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Shiplineitems#execute()} method to invoke the remote operation.
* {@link Shiplineitems#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersShipLineItemsRequest}
* @since 1.13
*/
protected Shiplineitems(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersShipLineItemsRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersShipLineItemsResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Shiplineitems setAlt(java.lang.String alt) {
return (Shiplineitems) super.setAlt(alt);
}
@Override
public Shiplineitems setFields(java.lang.String fields) {
return (Shiplineitems) super.setFields(fields);
}
@Override
public Shiplineitems setKey(java.lang.String key) {
return (Shiplineitems) super.setKey(key);
}
@Override
public Shiplineitems setOauthToken(java.lang.String oauthToken) {
return (Shiplineitems) super.setOauthToken(oauthToken);
}
@Override
public Shiplineitems setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Shiplineitems) super.setPrettyPrint(prettyPrint);
}
@Override
public Shiplineitems setQuotaUser(java.lang.String quotaUser) {
return (Shiplineitems) super.setQuotaUser(quotaUser);
}
@Override
public Shiplineitems setUserIp(java.lang.String userIp) {
return (Shiplineitems) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Shiplineitems setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Shiplineitems setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Shiplineitems set(String parameterName, Object value) {
return (Shiplineitems) super.set(parameterName, value);
}
}
/**
* Updates ship by and delivery by dates for a line item.
*
* Create a request for the method "orders.updatelineitemshippingdetails".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Updatelineitemshippingdetails#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateLineItemShippingDetailsRequest}
* @return the request
*/
public Updatelineitemshippingdetails updatelineitemshippingdetails(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateLineItemShippingDetailsRequest content) throws java.io.IOException {
Updatelineitemshippingdetails result = new Updatelineitemshippingdetails(merchantId, orderId, content);
initialize(result);
return result;
}
public class Updatelineitemshippingdetails extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/updateLineItemShippingDetails";
/**
* Updates ship by and delivery by dates for a line item.
*
* Create a request for the method "orders.updatelineitemshippingdetails".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Updatelineitemshippingdetails#execute()} method to invoke the
* remote operation. {@link Updatelineitemshippingdetails#initialize(com.google.api.client.goo
* gleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateLineItemShippingDetailsRequest}
* @since 1.13
*/
protected Updatelineitemshippingdetails(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateLineItemShippingDetailsRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersUpdateLineItemShippingDetailsResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Updatelineitemshippingdetails setAlt(java.lang.String alt) {
return (Updatelineitemshippingdetails) super.setAlt(alt);
}
@Override
public Updatelineitemshippingdetails setFields(java.lang.String fields) {
return (Updatelineitemshippingdetails) super.setFields(fields);
}
@Override
public Updatelineitemshippingdetails setKey(java.lang.String key) {
return (Updatelineitemshippingdetails) super.setKey(key);
}
@Override
public Updatelineitemshippingdetails setOauthToken(java.lang.String oauthToken) {
return (Updatelineitemshippingdetails) super.setOauthToken(oauthToken);
}
@Override
public Updatelineitemshippingdetails setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Updatelineitemshippingdetails) super.setPrettyPrint(prettyPrint);
}
@Override
public Updatelineitemshippingdetails setQuotaUser(java.lang.String quotaUser) {
return (Updatelineitemshippingdetails) super.setQuotaUser(quotaUser);
}
@Override
public Updatelineitemshippingdetails setUserIp(java.lang.String userIp) {
return (Updatelineitemshippingdetails) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Updatelineitemshippingdetails setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Updatelineitemshippingdetails setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Updatelineitemshippingdetails set(String parameterName, Object value) {
return (Updatelineitemshippingdetails) super.set(parameterName, value);
}
}
/**
* Updates the merchant order ID for a given order.
*
* Create a request for the method "orders.updatemerchantorderid".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Updatemerchantorderid#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest}
* @return the request
*/
public Updatemerchantorderid updatemerchantorderid(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest content) throws java.io.IOException {
Updatemerchantorderid result = new Updatemerchantorderid(merchantId, orderId, content);
initialize(result);
return result;
}
public class Updatemerchantorderid extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/updateMerchantOrderId";
/**
* Updates the merchant order ID for a given order.
*
* Create a request for the method "orders.updatemerchantorderid".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Updatemerchantorderid#execute()} method to invoke the remote
* operation. {@link Updatemerchantorderid#initialize(com.google.api.client.googleapis.service
* s.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest}
* @since 1.13
*/
protected Updatemerchantorderid(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersUpdateMerchantOrderIdResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Updatemerchantorderid setAlt(java.lang.String alt) {
return (Updatemerchantorderid) super.setAlt(alt);
}
@Override
public Updatemerchantorderid setFields(java.lang.String fields) {
return (Updatemerchantorderid) super.setFields(fields);
}
@Override
public Updatemerchantorderid setKey(java.lang.String key) {
return (Updatemerchantorderid) super.setKey(key);
}
@Override
public Updatemerchantorderid setOauthToken(java.lang.String oauthToken) {
return (Updatemerchantorderid) super.setOauthToken(oauthToken);
}
@Override
public Updatemerchantorderid setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Updatemerchantorderid) super.setPrettyPrint(prettyPrint);
}
@Override
public Updatemerchantorderid setQuotaUser(java.lang.String quotaUser) {
return (Updatemerchantorderid) super.setQuotaUser(quotaUser);
}
@Override
public Updatemerchantorderid setUserIp(java.lang.String userIp) {
return (Updatemerchantorderid) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Updatemerchantorderid setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Updatemerchantorderid setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Updatemerchantorderid set(String parameterName, Object value) {
return (Updatemerchantorderid) super.set(parameterName, value);
}
}
/**
* Updates a shipment's status, carrier, and/or tracking ID.
*
* Create a request for the method "orders.updateshipment".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Updateshipment#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateShipmentRequest}
* @return the request
*/
public Updateshipment updateshipment(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateShipmentRequest content) throws java.io.IOException {
Updateshipment result = new Updateshipment(merchantId, orderId, content);
initialize(result);
return result;
}
public class Updateshipment extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/updateShipment";
/**
* Updates a shipment's status, carrier, and/or tracking ID.
*
* Create a request for the method "orders.updateshipment".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Updateshipment#execute()} method to invoke the remote operation.
* {@link Updateshipment#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the account that manages the order. This cannot be a multi-client account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateShipmentRequest}
* @since 1.13
*/
protected Updateshipment(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateShipmentRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersUpdateShipmentResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Updateshipment setAlt(java.lang.String alt) {
return (Updateshipment) super.setAlt(alt);
}
@Override
public Updateshipment setFields(java.lang.String fields) {
return (Updateshipment) super.setFields(fields);
}
@Override
public Updateshipment setKey(java.lang.String key) {
return (Updateshipment) super.setKey(key);
}
@Override
public Updateshipment setOauthToken(java.lang.String oauthToken) {
return (Updateshipment) super.setOauthToken(oauthToken);
}
@Override
public Updateshipment setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Updateshipment) super.setPrettyPrint(prettyPrint);
}
@Override
public Updateshipment setQuotaUser(java.lang.String quotaUser) {
return (Updateshipment) super.setQuotaUser(quotaUser);
}
@Override
public Updateshipment setUserIp(java.lang.String userIp) {
return (Updateshipment) super.setUserIp(userIp);
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that manages the order. This cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account that manages the order. This cannot be a multi-client account. */
public Updateshipment setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Updateshipment setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Updateshipment set(String parameterName, Object value) {
return (Updateshipment) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Pos collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Pos.List request = content.pos().list(parameters ...)}
*
*
* @return the resource collection
*/
public Pos pos() {
return new Pos();
}
/**
* The "pos" collection of methods.
*/
public class Pos {
/**
* Batches multiple POS-related calls in a single request.
*
* Create a request for the method "pos.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.PosCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.PosCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "pos/batch";
/**
* Batches multiple POS-related calls in a single request.
*
* Create a request for the method "pos.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.PosCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.PosCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.PosCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Deletes a store for the given merchant.
*
* Create a request for the method "pos.delete".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param storeCode A store code that is unique per merchant.
* @return the request
*/
public Delete delete(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, java.lang.String storeCode) throws java.io.IOException {
Delete result = new Delete(merchantId, targetMerchantId, storeCode);
initialize(result);
return result;
}
public class Delete extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/pos/{targetMerchantId}/store/{storeCode}";
/**
* Deletes a store for the given merchant.
*
* Create a request for the method "pos.delete".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param storeCode A store code that is unique per merchant.
* @since 1.13
*/
protected Delete(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, java.lang.String storeCode) {
super(ShoppingContent.this, "DELETE", REST_PATH, null, Void.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.targetMerchantId = com.google.api.client.util.Preconditions.checkNotNull(targetMerchantId, "Required parameter targetMerchantId must be specified.");
this.storeCode = com.google.api.client.util.Preconditions.checkNotNull(storeCode, "Required parameter storeCode must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** The ID of the POS or inventory data provider. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the POS or inventory data provider.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the POS or inventory data provider. */
public Delete setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the target merchant. */
@com.google.api.client.util.Key
private java.math.BigInteger targetMerchantId;
/** The ID of the target merchant.
*/
public java.math.BigInteger getTargetMerchantId() {
return targetMerchantId;
}
/** The ID of the target merchant. */
public Delete setTargetMerchantId(java.math.BigInteger targetMerchantId) {
this.targetMerchantId = targetMerchantId;
return this;
}
/** A store code that is unique per merchant. */
@com.google.api.client.util.Key
private java.lang.String storeCode;
/** A store code that is unique per merchant.
*/
public java.lang.String getStoreCode() {
return storeCode;
}
/** A store code that is unique per merchant. */
public Delete setStoreCode(java.lang.String storeCode) {
this.storeCode = storeCode;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Delete setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves information about the given store.
*
* Create a request for the method "pos.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param storeCode A store code that is unique per merchant.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, java.lang.String storeCode) throws java.io.IOException {
Get result = new Get(merchantId, targetMerchantId, storeCode);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/pos/{targetMerchantId}/store/{storeCode}";
/**
* Retrieves information about the given store.
*
* Create a request for the method "pos.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param storeCode A store code that is unique per merchant.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, java.lang.String storeCode) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.PosStore.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.targetMerchantId = com.google.api.client.util.Preconditions.checkNotNull(targetMerchantId, "Required parameter targetMerchantId must be specified.");
this.storeCode = com.google.api.client.util.Preconditions.checkNotNull(storeCode, "Required parameter storeCode must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the POS or inventory data provider. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the POS or inventory data provider.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the POS or inventory data provider. */
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the target merchant. */
@com.google.api.client.util.Key
private java.math.BigInteger targetMerchantId;
/** The ID of the target merchant.
*/
public java.math.BigInteger getTargetMerchantId() {
return targetMerchantId;
}
/** The ID of the target merchant. */
public Get setTargetMerchantId(java.math.BigInteger targetMerchantId) {
this.targetMerchantId = targetMerchantId;
return this;
}
/** A store code that is unique per merchant. */
@com.google.api.client.util.Key
private java.lang.String storeCode;
/** A store code that is unique per merchant.
*/
public java.lang.String getStoreCode() {
return storeCode;
}
/** A store code that is unique per merchant. */
public Get setStoreCode(java.lang.String storeCode) {
this.storeCode = storeCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a store for the given merchant.
*
* Create a request for the method "pos.insert".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param content the {@link com.google.api.services.content.model.PosStore}
* @return the request
*/
public Insert insert(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, com.google.api.services.content.model.PosStore content) throws java.io.IOException {
Insert result = new Insert(merchantId, targetMerchantId, content);
initialize(result);
return result;
}
public class Insert extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/pos/{targetMerchantId}/store";
/**
* Creates a store for the given merchant.
*
* Create a request for the method "pos.insert".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param content the {@link com.google.api.services.content.model.PosStore}
* @since 1.13
*/
protected Insert(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, com.google.api.services.content.model.PosStore content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.PosStore.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.targetMerchantId = com.google.api.client.util.Preconditions.checkNotNull(targetMerchantId, "Required parameter targetMerchantId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getStoreAddress(), "PosStore.getStoreAddress()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getStoreCode(), "PosStore.getStoreCode()");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the POS or inventory data provider. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the POS or inventory data provider.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the POS or inventory data provider. */
public Insert setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the target merchant. */
@com.google.api.client.util.Key
private java.math.BigInteger targetMerchantId;
/** The ID of the target merchant.
*/
public java.math.BigInteger getTargetMerchantId() {
return targetMerchantId;
}
/** The ID of the target merchant. */
public Insert setTargetMerchantId(java.math.BigInteger targetMerchantId) {
this.targetMerchantId = targetMerchantId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Insert setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Submit inventory for the given merchant.
*
* Create a request for the method "pos.inventory".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Inventory#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param content the {@link com.google.api.services.content.model.PosInventoryRequest}
* @return the request
*/
public Inventory inventory(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, com.google.api.services.content.model.PosInventoryRequest content) throws java.io.IOException {
Inventory result = new Inventory(merchantId, targetMerchantId, content);
initialize(result);
return result;
}
public class Inventory extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/pos/{targetMerchantId}/inventory";
/**
* Submit inventory for the given merchant.
*
* Create a request for the method "pos.inventory".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Inventory#execute()} method to invoke the remote operation.
* {@link
* Inventory#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param content the {@link com.google.api.services.content.model.PosInventoryRequest}
* @since 1.13
*/
protected Inventory(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, com.google.api.services.content.model.PosInventoryRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.PosInventoryResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.targetMerchantId = com.google.api.client.util.Preconditions.checkNotNull(targetMerchantId, "Required parameter targetMerchantId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getContentLanguage(), "PosInventoryRequest.getContentLanguage()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getItemId(), "PosInventoryRequest.getItemId()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getQuantity(), "PosInventoryRequest.getQuantity()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getStoreCode(), "PosInventoryRequest.getStoreCode()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getTargetCountry(), "PosInventoryRequest.getTargetCountry()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getTimestamp(), "PosInventoryRequest.getTimestamp()");
}
@Override
public Inventory setAlt(java.lang.String alt) {
return (Inventory) super.setAlt(alt);
}
@Override
public Inventory setFields(java.lang.String fields) {
return (Inventory) super.setFields(fields);
}
@Override
public Inventory setKey(java.lang.String key) {
return (Inventory) super.setKey(key);
}
@Override
public Inventory setOauthToken(java.lang.String oauthToken) {
return (Inventory) super.setOauthToken(oauthToken);
}
@Override
public Inventory setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Inventory) super.setPrettyPrint(prettyPrint);
}
@Override
public Inventory setQuotaUser(java.lang.String quotaUser) {
return (Inventory) super.setQuotaUser(quotaUser);
}
@Override
public Inventory setUserIp(java.lang.String userIp) {
return (Inventory) super.setUserIp(userIp);
}
/** The ID of the POS or inventory data provider. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the POS or inventory data provider.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the POS or inventory data provider. */
public Inventory setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the target merchant. */
@com.google.api.client.util.Key
private java.math.BigInteger targetMerchantId;
/** The ID of the target merchant.
*/
public java.math.BigInteger getTargetMerchantId() {
return targetMerchantId;
}
/** The ID of the target merchant. */
public Inventory setTargetMerchantId(java.math.BigInteger targetMerchantId) {
this.targetMerchantId = targetMerchantId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Inventory setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Inventory set(String parameterName, Object value) {
return (Inventory) super.set(parameterName, value);
}
}
/**
* Lists the stores of the target merchant.
*
* Create a request for the method "pos.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @return the request
*/
public List list(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId) throws java.io.IOException {
List result = new List(merchantId, targetMerchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/pos/{targetMerchantId}/store";
/**
* Lists the stores of the target merchant.
*
* Create a request for the method "pos.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.PosListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.targetMerchantId = com.google.api.client.util.Preconditions.checkNotNull(targetMerchantId, "Required parameter targetMerchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the POS or inventory data provider. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the POS or inventory data provider.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the POS or inventory data provider. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the target merchant. */
@com.google.api.client.util.Key
private java.math.BigInteger targetMerchantId;
/** The ID of the target merchant.
*/
public java.math.BigInteger getTargetMerchantId() {
return targetMerchantId;
}
/** The ID of the target merchant. */
public List setTargetMerchantId(java.math.BigInteger targetMerchantId) {
this.targetMerchantId = targetMerchantId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Submit a sale event for the given merchant.
*
* Create a request for the method "pos.sale".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Sale#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param content the {@link com.google.api.services.content.model.PosSaleRequest}
* @return the request
*/
public Sale sale(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, com.google.api.services.content.model.PosSaleRequest content) throws java.io.IOException {
Sale result = new Sale(merchantId, targetMerchantId, content);
initialize(result);
return result;
}
public class Sale extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/pos/{targetMerchantId}/sale";
/**
* Submit a sale event for the given merchant.
*
* Create a request for the method "pos.sale".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Sale#execute()} method to invoke the remote operation. {@link
* Sale#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the POS or inventory data provider.
* @param targetMerchantId The ID of the target merchant.
* @param content the {@link com.google.api.services.content.model.PosSaleRequest}
* @since 1.13
*/
protected Sale(java.math.BigInteger merchantId, java.math.BigInteger targetMerchantId, com.google.api.services.content.model.PosSaleRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.PosSaleResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.targetMerchantId = com.google.api.client.util.Preconditions.checkNotNull(targetMerchantId, "Required parameter targetMerchantId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getContentLanguage(), "PosSaleRequest.getContentLanguage()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getItemId(), "PosSaleRequest.getItemId()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getQuantity(), "PosSaleRequest.getQuantity()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getStoreCode(), "PosSaleRequest.getStoreCode()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getTargetCountry(), "PosSaleRequest.getTargetCountry()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getTimestamp(), "PosSaleRequest.getTimestamp()");
}
@Override
public Sale setAlt(java.lang.String alt) {
return (Sale) super.setAlt(alt);
}
@Override
public Sale setFields(java.lang.String fields) {
return (Sale) super.setFields(fields);
}
@Override
public Sale setKey(java.lang.String key) {
return (Sale) super.setKey(key);
}
@Override
public Sale setOauthToken(java.lang.String oauthToken) {
return (Sale) super.setOauthToken(oauthToken);
}
@Override
public Sale setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Sale) super.setPrettyPrint(prettyPrint);
}
@Override
public Sale setQuotaUser(java.lang.String quotaUser) {
return (Sale) super.setQuotaUser(quotaUser);
}
@Override
public Sale setUserIp(java.lang.String userIp) {
return (Sale) super.setUserIp(userIp);
}
/** The ID of the POS or inventory data provider. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the POS or inventory data provider.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the POS or inventory data provider. */
public Sale setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the target merchant. */
@com.google.api.client.util.Key
private java.math.BigInteger targetMerchantId;
/** The ID of the target merchant.
*/
public java.math.BigInteger getTargetMerchantId() {
return targetMerchantId;
}
/** The ID of the target merchant. */
public Sale setTargetMerchantId(java.math.BigInteger targetMerchantId) {
this.targetMerchantId = targetMerchantId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Sale setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Sale set(String parameterName, Object value) {
return (Sale) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Products collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Products.List request = content.products().list(parameters ...)}
*
*
* @return the resource collection
*/
public Products products() {
return new Products();
}
/**
* The "products" collection of methods.
*/
public class Products {
/**
* Retrieves, inserts, and deletes multiple products in a single request.
*
* Create a request for the method "products.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.ProductsCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.ProductsCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "products/batch";
/**
* Retrieves, inserts, and deletes multiple products in a single request.
*
* Create a request for the method "products.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.ProductsCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.ProductsCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.ProductsCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Deletes a product from your Merchant Center account.
*
* Create a request for the method "products.delete".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param productId The REST id of the product.
* @return the request
*/
public Delete delete(java.math.BigInteger merchantId, java.lang.String productId) throws java.io.IOException {
Delete result = new Delete(merchantId, productId);
initialize(result);
return result;
}
public class Delete extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/products/{productId}";
/**
* Deletes a product from your Merchant Center account.
*
* Create a request for the method "products.delete".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param productId The REST id of the product.
* @since 1.13
*/
protected Delete(java.math.BigInteger merchantId, java.lang.String productId) {
super(ShoppingContent.this, "DELETE", REST_PATH, null, Void.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that contains the product. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
public Delete setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The REST id of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The REST id of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The REST id of the product. */
public Delete setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Delete setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a product from your Merchant Center account.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param productId The REST id of the product.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.lang.String productId) throws java.io.IOException {
Get result = new Get(merchantId, productId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/products/{productId}";
/**
* Retrieves a product from your Merchant Center account.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param productId The REST id of the product.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.lang.String productId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.Product.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that contains the product. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The REST id of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The REST id of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The REST id of the product. */
public Get setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Uploads a product to your Merchant Center account. If an item with the same channel,
* contentLanguage, offerId, and targetCountry already exists, this method updates that entry.
*
* Create a request for the method "products.insert".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param content the {@link com.google.api.services.content.model.Product}
* @return the request
*/
public Insert insert(java.math.BigInteger merchantId, com.google.api.services.content.model.Product content) throws java.io.IOException {
Insert result = new Insert(merchantId, content);
initialize(result);
return result;
}
public class Insert extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/products";
/**
* Uploads a product to your Merchant Center account. If an item with the same channel,
* contentLanguage, offerId, and targetCountry already exists, this method updates that entry.
*
* Create a request for the method "products.insert".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param content the {@link com.google.api.services.content.model.Product}
* @since 1.13
*/
protected Insert(java.math.BigInteger merchantId, com.google.api.services.content.model.Product content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.Product.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getChannel(), "Product.getChannel()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getContentLanguage(), "Product.getContentLanguage()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getOfferId(), "Product.getOfferId()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getTargetCountry(), "Product.getTargetCountry()");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that contains the product. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
public Insert setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Insert setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists the products in your Merchant Center account.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that contains the products. This account cannot be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/products";
/**
* Lists the products in your Merchant Center account.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that contains the products. This account cannot be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.ProductsListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The ID of the account that contains the products. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that contains the products. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that contains the products. This account cannot be a multi-client
* account.
*/
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* Flag to include the invalid inserted items in the result of the list request. By default
* the invalid items are not shown (the default value is false).
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeInvalidInsertedItems;
/** Flag to include the invalid inserted items in the result of the list request. By default the
invalid items are not shown (the default value is false).
*/
public java.lang.Boolean getIncludeInvalidInsertedItems() {
return includeInvalidInsertedItems;
}
/**
* Flag to include the invalid inserted items in the result of the list request. By default
* the invalid items are not shown (the default value is false).
*/
public List setIncludeInvalidInsertedItems(java.lang.Boolean includeInvalidInsertedItems) {
this.includeInvalidInsertedItems = includeInvalidInsertedItems;
return this;
}
/** The maximum number of products to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of products to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of products to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Productstatuses collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Productstatuses.List request = content.productstatuses().list(parameters ...)}
*
*
* @return the resource collection
*/
public Productstatuses productstatuses() {
return new Productstatuses();
}
/**
* The "productstatuses" collection of methods.
*/
public class Productstatuses {
/**
* Gets the statuses of multiple products in a single request.
*
* Create a request for the method "productstatuses.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.ProductstatusesCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.ProductstatusesCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "productstatuses/batch";
/**
* Gets the statuses of multiple products in a single request.
*
* Create a request for the method "productstatuses.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.ProductstatusesCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.ProductstatusesCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.ProductstatusesCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/**
* Flag to include full product data in the results of this request. The default value is
* false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeAttributes;
/** Flag to include full product data in the results of this request. The default value is false.
*/
public java.lang.Boolean getIncludeAttributes() {
return includeAttributes;
}
/**
* Flag to include full product data in the results of this request. The default value is
* false.
*/
public Custombatch setIncludeAttributes(java.lang.Boolean includeAttributes) {
this.includeAttributes = includeAttributes;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Gets the status of a product from your Merchant Center account.
*
* Create a request for the method "productstatuses.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param productId The REST id of the product.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.lang.String productId) throws java.io.IOException {
Get result = new Get(merchantId, productId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/productstatuses/{productId}";
/**
* Gets the status of a product from your Merchant Center account.
*
* Create a request for the method "productstatuses.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that contains the product. This account cannot be a multi-client account.
* @param productId The REST id of the product.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.lang.String productId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.ProductStatus.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that contains the product. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that contains the product. This account cannot be a multi-client
* account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The REST id of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The REST id of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The REST id of the product. */
public Get setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
@com.google.api.client.util.Key
private java.util.List destinations;
/** If set, only issues for the specified destinations are returned, otherwise only issues for the
Shopping destination.
*/
public java.util.List getDestinations() {
return destinations;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
public Get setDestinations(java.util.List destinations) {
this.destinations = destinations;
return this;
}
/**
* Flag to include full product data in the result of this get request. The default value is
* false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeAttributes;
/** Flag to include full product data in the result of this get request. The default value is false.
*/
public java.lang.Boolean getIncludeAttributes() {
return includeAttributes;
}
/**
* Flag to include full product data in the result of this get request. The default value is
* false.
*/
public Get setIncludeAttributes(java.lang.Boolean includeAttributes) {
this.includeAttributes = includeAttributes;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the statuses of the products in your Merchant Center account.
*
* Create a request for the method "productstatuses.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the account that contains the products. This account cannot be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/productstatuses";
/**
* Lists the statuses of the products in your Merchant Center account.
*
* Create a request for the method "productstatuses.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the account that contains the products. This account cannot be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.ProductstatusesListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The ID of the account that contains the products. This account cannot be a multi-client
* account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account that contains the products. This account cannot be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the account that contains the products. This account cannot be a multi-client
* account.
*/
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
@com.google.api.client.util.Key
private java.util.List destinations;
/** If set, only issues for the specified destinations are returned, otherwise only issues for the
Shopping destination.
*/
public java.util.List getDestinations() {
return destinations;
}
/**
* If set, only issues for the specified destinations are returned, otherwise only issues for
* the Shopping destination.
*/
public List setDestinations(java.util.List destinations) {
this.destinations = destinations;
return this;
}
/**
* Flag to include full product data in the results of the list request. The default value is
* false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeAttributes;
/** Flag to include full product data in the results of the list request. The default value is false.
*/
public java.lang.Boolean getIncludeAttributes() {
return includeAttributes;
}
/**
* Flag to include full product data in the results of the list request. The default value is
* false.
*/
public List setIncludeAttributes(java.lang.Boolean includeAttributes) {
this.includeAttributes = includeAttributes;
return this;
}
/**
* Flag to include the invalid inserted items in the result of the list request. By default
* the invalid items are not shown (the default value is false).
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeInvalidInsertedItems;
/** Flag to include the invalid inserted items in the result of the list request. By default the
invalid items are not shown (the default value is false).
*/
public java.lang.Boolean getIncludeInvalidInsertedItems() {
return includeInvalidInsertedItems;
}
/**
* Flag to include the invalid inserted items in the result of the list request. By default
* the invalid items are not shown (the default value is false).
*/
public List setIncludeInvalidInsertedItems(java.lang.Boolean includeInvalidInsertedItems) {
this.includeInvalidInsertedItems = includeInvalidInsertedItems;
return this;
}
/** The maximum number of product statuses to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of product statuses to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of product statuses to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Shippingsettings collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Shippingsettings.List request = content.shippingsettings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Shippingsettings shippingsettings() {
return new Shippingsettings();
}
/**
* The "shippingsettings" collection of methods.
*/
public class Shippingsettings {
/**
* Retrieves and updates the shipping settings of multiple accounts in a single request.
*
* Create a request for the method "shippingsettings.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.ShippingsettingsCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.ShippingsettingsCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "shippingsettings/batch";
/**
* Retrieves and updates the shipping settings of multiple accounts in a single request.
*
* Create a request for the method "shippingsettings.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.ShippingsettingsCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.ShippingsettingsCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.ShippingsettingsCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Custombatch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Retrieves the shipping settings of the account.
*
* Create a request for the method "shippingsettings.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update shipping settings.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.math.BigInteger accountId) throws java.io.IOException {
Get result = new Get(merchantId, accountId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/shippingsettings/{accountId}";
/**
* Retrieves the shipping settings of the account.
*
* Create a request for the method "shippingsettings.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update shipping settings.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.math.BigInteger accountId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.ShippingSettings.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get/update shipping settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get/update shipping settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get/update shipping settings. */
public Get setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves supported carriers and carrier services for an account.
*
* Create a request for the method "shippingsettings.getsupportedcarriers".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Getsupportedcarriers#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account for which to retrieve the supported carriers.
* @return the request
*/
public Getsupportedcarriers getsupportedcarriers(java.math.BigInteger merchantId) throws java.io.IOException {
Getsupportedcarriers result = new Getsupportedcarriers(merchantId);
initialize(result);
return result;
}
public class Getsupportedcarriers extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/supportedCarriers";
/**
* Retrieves supported carriers and carrier services for an account.
*
* Create a request for the method "shippingsettings.getsupportedcarriers".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Getsupportedcarriers#execute()} method to invoke the remote
* operation. {@link Getsupportedcarriers#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account for which to retrieve the supported carriers.
* @since 1.13
*/
protected Getsupportedcarriers(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.ShippingsettingsGetSupportedCarriersResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Getsupportedcarriers setAlt(java.lang.String alt) {
return (Getsupportedcarriers) super.setAlt(alt);
}
@Override
public Getsupportedcarriers setFields(java.lang.String fields) {
return (Getsupportedcarriers) super.setFields(fields);
}
@Override
public Getsupportedcarriers setKey(java.lang.String key) {
return (Getsupportedcarriers) super.setKey(key);
}
@Override
public Getsupportedcarriers setOauthToken(java.lang.String oauthToken) {
return (Getsupportedcarriers) super.setOauthToken(oauthToken);
}
@Override
public Getsupportedcarriers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Getsupportedcarriers) super.setPrettyPrint(prettyPrint);
}
@Override
public Getsupportedcarriers setQuotaUser(java.lang.String quotaUser) {
return (Getsupportedcarriers) super.setQuotaUser(quotaUser);
}
@Override
public Getsupportedcarriers setUserIp(java.lang.String userIp) {
return (Getsupportedcarriers) super.setUserIp(userIp);
}
/** The ID of the account for which to retrieve the supported carriers. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account for which to retrieve the supported carriers.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account for which to retrieve the supported carriers. */
public Getsupportedcarriers setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
@Override
public Getsupportedcarriers set(String parameterName, Object value) {
return (Getsupportedcarriers) super.set(parameterName, value);
}
}
/**
* Retrieves supported holidays for an account.
*
* Create a request for the method "shippingsettings.getsupportedholidays".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Getsupportedholidays#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the account for which to retrieve the supported holidays.
* @return the request
*/
public Getsupportedholidays getsupportedholidays(java.math.BigInteger merchantId) throws java.io.IOException {
Getsupportedholidays result = new Getsupportedholidays(merchantId);
initialize(result);
return result;
}
public class Getsupportedholidays extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/supportedHolidays";
/**
* Retrieves supported holidays for an account.
*
* Create a request for the method "shippingsettings.getsupportedholidays".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Getsupportedholidays#execute()} method to invoke the remote
* operation. {@link Getsupportedholidays#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the account for which to retrieve the supported holidays.
* @since 1.13
*/
protected Getsupportedholidays(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.ShippingsettingsGetSupportedHolidaysResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Getsupportedholidays setAlt(java.lang.String alt) {
return (Getsupportedholidays) super.setAlt(alt);
}
@Override
public Getsupportedholidays setFields(java.lang.String fields) {
return (Getsupportedholidays) super.setFields(fields);
}
@Override
public Getsupportedholidays setKey(java.lang.String key) {
return (Getsupportedholidays) super.setKey(key);
}
@Override
public Getsupportedholidays setOauthToken(java.lang.String oauthToken) {
return (Getsupportedholidays) super.setOauthToken(oauthToken);
}
@Override
public Getsupportedholidays setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Getsupportedholidays) super.setPrettyPrint(prettyPrint);
}
@Override
public Getsupportedholidays setQuotaUser(java.lang.String quotaUser) {
return (Getsupportedholidays) super.setQuotaUser(quotaUser);
}
@Override
public Getsupportedholidays setUserIp(java.lang.String userIp) {
return (Getsupportedholidays) super.setUserIp(userIp);
}
/** The ID of the account for which to retrieve the supported holidays. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the account for which to retrieve the supported holidays.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the account for which to retrieve the supported holidays. */
public Getsupportedholidays setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
@Override
public Getsupportedholidays set(String parameterName, Object value) {
return (Getsupportedholidays) super.set(parameterName, value);
}
}
/**
* Lists the shipping settings of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "shippingsettings.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/shippingsettings";
/**
* Lists the shipping settings of the sub-accounts in your Merchant Center account.
*
* Create a request for the method "shippingsettings.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. This must be a multi-client account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.ShippingsettingsListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the managing account. This must be a multi-client account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. This must be a multi-client account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. This must be a multi-client account. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The maximum number of shipping settings to return in the response, used for paging. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of shipping settings to return in the response, used for paging.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of shipping settings to return in the response, used for paging. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the shipping settings of the account. This method supports patch semantics.
*
* Create a request for the method "shippingsettings.patch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update shipping settings.
* @param content the {@link com.google.api.services.content.model.ShippingSettings}
* @return the request
*/
public Patch patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.ShippingSettings content) throws java.io.IOException {
Patch result = new Patch(merchantId, accountId, content);
initialize(result);
return result;
}
public class Patch extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/shippingsettings/{accountId}";
/**
* Updates the shipping settings of the account. This method supports patch semantics.
*
* Create a request for the method "shippingsettings.patch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update shipping settings.
* @param content the {@link com.google.api.services.content.model.ShippingSettings}
* @since 1.13
*/
protected Patch(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.ShippingSettings content) {
super(ShoppingContent.this, "PATCH", REST_PATH, content, com.google.api.services.content.model.ShippingSettings.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Patch setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get/update shipping settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get/update shipping settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get/update shipping settings. */
public Patch setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Patch setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the shipping settings of the account.
*
* Create a request for the method "shippingsettings.update".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update shipping settings.
* @param content the {@link com.google.api.services.content.model.ShippingSettings}
* @return the request
*/
public Update update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.ShippingSettings content) throws java.io.IOException {
Update result = new Update(merchantId, accountId, content);
initialize(result);
return result;
}
public class Update extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/shippingsettings/{accountId}";
/**
* Updates the shipping settings of the account.
*
* Create a request for the method "shippingsettings.update".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account. If this parameter is not the same as accountId, then this account
* must be a multi-client account and accountId must be the ID of a sub-account of this
* account.
* @param accountId The ID of the account for which to get/update shipping settings.
* @param content the {@link com.google.api.services.content.model.ShippingSettings}
* @since 1.13
*/
protected Update(java.math.BigInteger merchantId, java.math.BigInteger accountId, com.google.api.services.content.model.ShippingSettings content) {
super(ShoppingContent.this, "PUT", REST_PATH, content, com.google.api.services.content.model.ShippingSettings.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account. If this parameter is not the same as accountId, then this account
must be a multi-client account and accountId must be the ID of a sub-account of this account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/**
* The ID of the managing account. If this parameter is not the same as accountId, then this
* account must be a multi-client account and accountId must be the ID of a sub-account of
* this account.
*/
public Update setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the account for which to get/update shipping settings. */
@com.google.api.client.util.Key
private java.math.BigInteger accountId;
/** The ID of the account for which to get/update shipping settings.
*/
public java.math.BigInteger getAccountId() {
return accountId;
}
/** The ID of the account for which to get/update shipping settings. */
public Update setAccountId(java.math.BigInteger accountId) {
this.accountId = accountId;
return this;
}
/** Flag to run the request in dry-run mode. */
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Flag to run the request in dry-run mode.
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/** Flag to run the request in dry-run mode. */
public Update setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link ShoppingContent}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link ShoppingContent}. */
@Override
public ShoppingContent build() {
return new ShoppingContent(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link ShoppingContentRequestInitializer}.
*
* @since 1.12
*/
public Builder setShoppingContentRequestInitializer(
ShoppingContentRequestInitializer shoppingcontentRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(shoppingcontentRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}