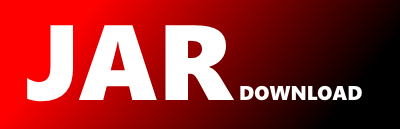
com.google.api.services.content.model.Product Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2014-04-15 19:10:39 UTC)
* on 2014-06-04 at 14:54:25 UTC
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Product data.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* http://code.google.com/p/google-api-java-client/wiki/Json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Product extends com.google.api.client.json.GenericJson {
/**
* Additional URLs of images of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List additionalImageLinks;
/**
* Set to true if the item is targeted towards adults.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean adult;
/**
* Used to group items in an arbitrary way. Only for CPA%, discouraged otherwise.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adwordsGrouping;
/**
* Similar to adwords_grouping, but only works on CPC.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List adwordsLabels;
/**
* Allows advertisers to override the item URL when the product is shown within the context of
* Product Ads.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adwordsRedirect;
/**
* Target age group of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ageGroup;
/**
* Availability status of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String availability;
/**
* Brand of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String brand;
/**
* The item's channel (online or local).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String channel;
/**
* Color of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String color;
/**
* Condition or state of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String condition;
/**
* The two-letter ISO 639-1 language code for the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contentLanguage;
/**
* A list of custom (merchant-provided) attributes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List customAttributes;
static {
// hack to force ProGuard to consider ProductCustomAttribute used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(ProductCustomAttribute.class);
}
/**
* A list of custom (merchant-provided) custom attribute groups.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List customGroups;
static {
// hack to force ProGuard to consider ProductCustomGroup used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(ProductCustomGroup.class);
}
/**
* Custom label 0 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel0;
/**
* Custom label 1 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel1;
/**
* Custom label 2 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel2;
/**
* Custom label 3 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel3;
/**
* Custom label 4 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel4;
/**
* Description of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Specifies the intended destinations for the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List destinations;
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String energyEfficiencyClass;
/**
* Date that an item will expire.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String expirationDate;
/**
* Target gender of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gender;
/**
* Google's category of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String googleProductCategory;
/**
* Global Trade Item Number (GTIN) of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gtin;
/**
* The REST id of the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* False when the item does not have unique product identifiers appropriate to its category, such
* as GTIN, MPN, and brand. Required according to the Unique Product Identifier Rules for all
* target countries except for Canada.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean identifierExists;
/**
* URL of an image of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imageLink;
/**
* Number and amount of installments to pay for an item. Brazil only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductInstallment installment;
/**
* Shared identifier for all variants of the same product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String itemGroupId;
/**
* Identifies what kind of resource this is. Value: the fixed string "content#product".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* URL directly linking to your item's page on your website.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String link;
/**
* Loyalty points that users receive after purchasing the item. Japan only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LoyaltyPoints loyaltyPoints;
/**
* The material of which the item is made.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String material;
/**
* The number of identical products in a merchant-defined multipack.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger merchantMultipackQuantity;
/**
* Manufacturer Part Number (MPN) of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mpn;
/**
* An identifier of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String offerId;
/**
* Whether an item is available for purchase only online.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean onlineOnly;
/**
* The item's pattern (e.g. polka dots).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pattern;
/**
* Price of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Price price;
/**
* Your category of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productType;
/**
* Advertised sale price of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Price salePrice;
/**
* Date range during which the item is on sale.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String salePriceEffectiveDate;
/**
* Shipping rules.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List shipping;
/**
* Weight of the item for shipping.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductShippingWeight shippingWeight;
/**
* Size of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sizes;
/**
* The two-letter ISO 3166 country code for the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String targetCountry;
/**
* Tax information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductTax tax;
/**
* Title of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The preference of the denominator of the unit price.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String unitPricingBaseMeasure;
/**
* The measure and dimension of an item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String unitPricingMeasure;
/**
* The read-only list of intended destinations which passed validation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List validatedDestinations;
/**
* Read-only warnings.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List warnings;
/**
* Additional URLs of images of the item.
* @return value or {@code null} for none
*/
public java.util.List getAdditionalImageLinks() {
return additionalImageLinks;
}
/**
* Additional URLs of images of the item.
* @param additionalImageLinks additionalImageLinks or {@code null} for none
*/
public Product setAdditionalImageLinks(java.util.List additionalImageLinks) {
this.additionalImageLinks = additionalImageLinks;
return this;
}
/**
* Set to true if the item is targeted towards adults.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAdult() {
return adult;
}
/**
* Set to true if the item is targeted towards adults.
* @param adult adult or {@code null} for none
*/
public Product setAdult(java.lang.Boolean adult) {
this.adult = adult;
return this;
}
/**
* Used to group items in an arbitrary way. Only for CPA%, discouraged otherwise.
* @return value or {@code null} for none
*/
public java.lang.String getAdwordsGrouping() {
return adwordsGrouping;
}
/**
* Used to group items in an arbitrary way. Only for CPA%, discouraged otherwise.
* @param adwordsGrouping adwordsGrouping or {@code null} for none
*/
public Product setAdwordsGrouping(java.lang.String adwordsGrouping) {
this.adwordsGrouping = adwordsGrouping;
return this;
}
/**
* Similar to adwords_grouping, but only works on CPC.
* @return value or {@code null} for none
*/
public java.util.List getAdwordsLabels() {
return adwordsLabels;
}
/**
* Similar to adwords_grouping, but only works on CPC.
* @param adwordsLabels adwordsLabels or {@code null} for none
*/
public Product setAdwordsLabels(java.util.List adwordsLabels) {
this.adwordsLabels = adwordsLabels;
return this;
}
/**
* Allows advertisers to override the item URL when the product is shown within the context of
* Product Ads.
* @return value or {@code null} for none
*/
public java.lang.String getAdwordsRedirect() {
return adwordsRedirect;
}
/**
* Allows advertisers to override the item URL when the product is shown within the context of
* Product Ads.
* @param adwordsRedirect adwordsRedirect or {@code null} for none
*/
public Product setAdwordsRedirect(java.lang.String adwordsRedirect) {
this.adwordsRedirect = adwordsRedirect;
return this;
}
/**
* Target age group of the item.
* @return value or {@code null} for none
*/
public java.lang.String getAgeGroup() {
return ageGroup;
}
/**
* Target age group of the item.
* @param ageGroup ageGroup or {@code null} for none
*/
public Product setAgeGroup(java.lang.String ageGroup) {
this.ageGroup = ageGroup;
return this;
}
/**
* Availability status of the item.
* @return value or {@code null} for none
*/
public java.lang.String getAvailability() {
return availability;
}
/**
* Availability status of the item.
* @param availability availability or {@code null} for none
*/
public Product setAvailability(java.lang.String availability) {
this.availability = availability;
return this;
}
/**
* Brand of the item.
* @return value or {@code null} for none
*/
public java.lang.String getBrand() {
return brand;
}
/**
* Brand of the item.
* @param brand brand or {@code null} for none
*/
public Product setBrand(java.lang.String brand) {
this.brand = brand;
return this;
}
/**
* The item's channel (online or local).
* @return value or {@code null} for none
*/
public java.lang.String getChannel() {
return channel;
}
/**
* The item's channel (online or local).
* @param channel channel or {@code null} for none
*/
public Product setChannel(java.lang.String channel) {
this.channel = channel;
return this;
}
/**
* Color of the item.
* @return value or {@code null} for none
*/
public java.lang.String getColor() {
return color;
}
/**
* Color of the item.
* @param color color or {@code null} for none
*/
public Product setColor(java.lang.String color) {
this.color = color;
return this;
}
/**
* Condition or state of the item.
* @return value or {@code null} for none
*/
public java.lang.String getCondition() {
return condition;
}
/**
* Condition or state of the item.
* @param condition condition or {@code null} for none
*/
public Product setCondition(java.lang.String condition) {
this.condition = condition;
return this;
}
/**
* The two-letter ISO 639-1 language code for the item.
* @return value or {@code null} for none
*/
public java.lang.String getContentLanguage() {
return contentLanguage;
}
/**
* The two-letter ISO 639-1 language code for the item.
* @param contentLanguage contentLanguage or {@code null} for none
*/
public Product setContentLanguage(java.lang.String contentLanguage) {
this.contentLanguage = contentLanguage;
return this;
}
/**
* A list of custom (merchant-provided) attributes.
* @return value or {@code null} for none
*/
public java.util.List getCustomAttributes() {
return customAttributes;
}
/**
* A list of custom (merchant-provided) attributes.
* @param customAttributes customAttributes or {@code null} for none
*/
public Product setCustomAttributes(java.util.List customAttributes) {
this.customAttributes = customAttributes;
return this;
}
/**
* A list of custom (merchant-provided) custom attribute groups.
* @return value or {@code null} for none
*/
public java.util.List getCustomGroups() {
return customGroups;
}
/**
* A list of custom (merchant-provided) custom attribute groups.
* @param customGroups customGroups or {@code null} for none
*/
public Product setCustomGroups(java.util.List customGroups) {
this.customGroups = customGroups;
return this;
}
/**
* Custom label 0 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel0() {
return customLabel0;
}
/**
* Custom label 0 for custom grouping of items in a Shopping campaign.
* @param customLabel0 customLabel0 or {@code null} for none
*/
public Product setCustomLabel0(java.lang.String customLabel0) {
this.customLabel0 = customLabel0;
return this;
}
/**
* Custom label 1 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel1() {
return customLabel1;
}
/**
* Custom label 1 for custom grouping of items in a Shopping campaign.
* @param customLabel1 customLabel1 or {@code null} for none
*/
public Product setCustomLabel1(java.lang.String customLabel1) {
this.customLabel1 = customLabel1;
return this;
}
/**
* Custom label 2 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel2() {
return customLabel2;
}
/**
* Custom label 2 for custom grouping of items in a Shopping campaign.
* @param customLabel2 customLabel2 or {@code null} for none
*/
public Product setCustomLabel2(java.lang.String customLabel2) {
this.customLabel2 = customLabel2;
return this;
}
/**
* Custom label 3 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel3() {
return customLabel3;
}
/**
* Custom label 3 for custom grouping of items in a Shopping campaign.
* @param customLabel3 customLabel3 or {@code null} for none
*/
public Product setCustomLabel3(java.lang.String customLabel3) {
this.customLabel3 = customLabel3;
return this;
}
/**
* Custom label 4 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel4() {
return customLabel4;
}
/**
* Custom label 4 for custom grouping of items in a Shopping campaign.
* @param customLabel4 customLabel4 or {@code null} for none
*/
public Product setCustomLabel4(java.lang.String customLabel4) {
this.customLabel4 = customLabel4;
return this;
}
/**
* Description of the item.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Description of the item.
* @param description description or {@code null} for none
*/
public Product setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Specifies the intended destinations for the product.
* @return value or {@code null} for none
*/
public java.util.List getDestinations() {
return destinations;
}
/**
* Specifies the intended destinations for the product.
* @param destinations destinations or {@code null} for none
*/
public Product setDestinations(java.util.List destinations) {
this.destinations = destinations;
return this;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @return value or {@code null} for none
*/
public java.lang.String getEnergyEfficiencyClass() {
return energyEfficiencyClass;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @param energyEfficiencyClass energyEfficiencyClass or {@code null} for none
*/
public Product setEnergyEfficiencyClass(java.lang.String energyEfficiencyClass) {
this.energyEfficiencyClass = energyEfficiencyClass;
return this;
}
/**
* Date that an item will expire.
* @return value or {@code null} for none
*/
public java.lang.String getExpirationDate() {
return expirationDate;
}
/**
* Date that an item will expire.
* @param expirationDate expirationDate or {@code null} for none
*/
public Product setExpirationDate(java.lang.String expirationDate) {
this.expirationDate = expirationDate;
return this;
}
/**
* Target gender of the item.
* @return value or {@code null} for none
*/
public java.lang.String getGender() {
return gender;
}
/**
* Target gender of the item.
* @param gender gender or {@code null} for none
*/
public Product setGender(java.lang.String gender) {
this.gender = gender;
return this;
}
/**
* Google's category of the item.
* @return value or {@code null} for none
*/
public java.lang.String getGoogleProductCategory() {
return googleProductCategory;
}
/**
* Google's category of the item.
* @param googleProductCategory googleProductCategory or {@code null} for none
*/
public Product setGoogleProductCategory(java.lang.String googleProductCategory) {
this.googleProductCategory = googleProductCategory;
return this;
}
/**
* Global Trade Item Number (GTIN) of the item.
* @return value or {@code null} for none
*/
public java.lang.String getGtin() {
return gtin;
}
/**
* Global Trade Item Number (GTIN) of the item.
* @param gtin gtin or {@code null} for none
*/
public Product setGtin(java.lang.String gtin) {
this.gtin = gtin;
return this;
}
/**
* The REST id of the product.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The REST id of the product.
* @param id id or {@code null} for none
*/
public Product setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* False when the item does not have unique product identifiers appropriate to its category, such
* as GTIN, MPN, and brand. Required according to the Unique Product Identifier Rules for all
* target countries except for Canada.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIdentifierExists() {
return identifierExists;
}
/**
* False when the item does not have unique product identifiers appropriate to its category, such
* as GTIN, MPN, and brand. Required according to the Unique Product Identifier Rules for all
* target countries except for Canada.
* @param identifierExists identifierExists or {@code null} for none
*/
public Product setIdentifierExists(java.lang.Boolean identifierExists) {
this.identifierExists = identifierExists;
return this;
}
/**
* URL of an image of the item.
* @return value or {@code null} for none
*/
public java.lang.String getImageLink() {
return imageLink;
}
/**
* URL of an image of the item.
* @param imageLink imageLink or {@code null} for none
*/
public Product setImageLink(java.lang.String imageLink) {
this.imageLink = imageLink;
return this;
}
/**
* Number and amount of installments to pay for an item. Brazil only.
* @return value or {@code null} for none
*/
public ProductInstallment getInstallment() {
return installment;
}
/**
* Number and amount of installments to pay for an item. Brazil only.
* @param installment installment or {@code null} for none
*/
public Product setInstallment(ProductInstallment installment) {
this.installment = installment;
return this;
}
/**
* Shared identifier for all variants of the same product.
* @return value or {@code null} for none
*/
public java.lang.String getItemGroupId() {
return itemGroupId;
}
/**
* Shared identifier for all variants of the same product.
* @param itemGroupId itemGroupId or {@code null} for none
*/
public Product setItemGroupId(java.lang.String itemGroupId) {
this.itemGroupId = itemGroupId;
return this;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "content#product".
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "content#product".
* @param kind kind or {@code null} for none
*/
public Product setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* URL directly linking to your item's page on your website.
* @return value or {@code null} for none
*/
public java.lang.String getLink() {
return link;
}
/**
* URL directly linking to your item's page on your website.
* @param link link or {@code null} for none
*/
public Product setLink(java.lang.String link) {
this.link = link;
return this;
}
/**
* Loyalty points that users receive after purchasing the item. Japan only.
* @return value or {@code null} for none
*/
public LoyaltyPoints getLoyaltyPoints() {
return loyaltyPoints;
}
/**
* Loyalty points that users receive after purchasing the item. Japan only.
* @param loyaltyPoints loyaltyPoints or {@code null} for none
*/
public Product setLoyaltyPoints(LoyaltyPoints loyaltyPoints) {
this.loyaltyPoints = loyaltyPoints;
return this;
}
/**
* The material of which the item is made.
* @return value or {@code null} for none
*/
public java.lang.String getMaterial() {
return material;
}
/**
* The material of which the item is made.
* @param material material or {@code null} for none
*/
public Product setMaterial(java.lang.String material) {
this.material = material;
return this;
}
/**
* The number of identical products in a merchant-defined multipack.
* @return value or {@code null} for none
*/
public java.math.BigInteger getMerchantMultipackQuantity() {
return merchantMultipackQuantity;
}
/**
* The number of identical products in a merchant-defined multipack.
* @param merchantMultipackQuantity merchantMultipackQuantity or {@code null} for none
*/
public Product setMerchantMultipackQuantity(java.math.BigInteger merchantMultipackQuantity) {
this.merchantMultipackQuantity = merchantMultipackQuantity;
return this;
}
/**
* Manufacturer Part Number (MPN) of the item.
* @return value or {@code null} for none
*/
public java.lang.String getMpn() {
return mpn;
}
/**
* Manufacturer Part Number (MPN) of the item.
* @param mpn mpn or {@code null} for none
*/
public Product setMpn(java.lang.String mpn) {
this.mpn = mpn;
return this;
}
/**
* An identifier of the item.
* @return value or {@code null} for none
*/
public java.lang.String getOfferId() {
return offerId;
}
/**
* An identifier of the item.
* @param offerId offerId or {@code null} for none
*/
public Product setOfferId(java.lang.String offerId) {
this.offerId = offerId;
return this;
}
/**
* Whether an item is available for purchase only online.
* @return value or {@code null} for none
*/
public java.lang.Boolean getOnlineOnly() {
return onlineOnly;
}
/**
* Whether an item is available for purchase only online.
* @param onlineOnly onlineOnly or {@code null} for none
*/
public Product setOnlineOnly(java.lang.Boolean onlineOnly) {
this.onlineOnly = onlineOnly;
return this;
}
/**
* The item's pattern (e.g. polka dots).
* @return value or {@code null} for none
*/
public java.lang.String getPattern() {
return pattern;
}
/**
* The item's pattern (e.g. polka dots).
* @param pattern pattern or {@code null} for none
*/
public Product setPattern(java.lang.String pattern) {
this.pattern = pattern;
return this;
}
/**
* Price of the item.
* @return value or {@code null} for none
*/
public Price getPrice() {
return price;
}
/**
* Price of the item.
* @param price price or {@code null} for none
*/
public Product setPrice(Price price) {
this.price = price;
return this;
}
/**
* Your category of the item.
* @return value or {@code null} for none
*/
public java.lang.String getProductType() {
return productType;
}
/**
* Your category of the item.
* @param productType productType or {@code null} for none
*/
public Product setProductType(java.lang.String productType) {
this.productType = productType;
return this;
}
/**
* Advertised sale price of the item.
* @return value or {@code null} for none
*/
public Price getSalePrice() {
return salePrice;
}
/**
* Advertised sale price of the item.
* @param salePrice salePrice or {@code null} for none
*/
public Product setSalePrice(Price salePrice) {
this.salePrice = salePrice;
return this;
}
/**
* Date range during which the item is on sale.
* @return value or {@code null} for none
*/
public java.lang.String getSalePriceEffectiveDate() {
return salePriceEffectiveDate;
}
/**
* Date range during which the item is on sale.
* @param salePriceEffectiveDate salePriceEffectiveDate or {@code null} for none
*/
public Product setSalePriceEffectiveDate(java.lang.String salePriceEffectiveDate) {
this.salePriceEffectiveDate = salePriceEffectiveDate;
return this;
}
/**
* Shipping rules.
* @return value or {@code null} for none
*/
public java.util.List getShipping() {
return shipping;
}
/**
* Shipping rules.
* @param shipping shipping or {@code null} for none
*/
public Product setShipping(java.util.List shipping) {
this.shipping = shipping;
return this;
}
/**
* Weight of the item for shipping.
* @return value or {@code null} for none
*/
public ProductShippingWeight getShippingWeight() {
return shippingWeight;
}
/**
* Weight of the item for shipping.
* @param shippingWeight shippingWeight or {@code null} for none
*/
public Product setShippingWeight(ProductShippingWeight shippingWeight) {
this.shippingWeight = shippingWeight;
return this;
}
/**
* Size of the item.
* @return value or {@code null} for none
*/
public java.util.List getSizes() {
return sizes;
}
/**
* Size of the item.
* @param sizes sizes or {@code null} for none
*/
public Product setSizes(java.util.List sizes) {
this.sizes = sizes;
return this;
}
/**
* The two-letter ISO 3166 country code for the item.
* @return value or {@code null} for none
*/
public java.lang.String getTargetCountry() {
return targetCountry;
}
/**
* The two-letter ISO 3166 country code for the item.
* @param targetCountry targetCountry or {@code null} for none
*/
public Product setTargetCountry(java.lang.String targetCountry) {
this.targetCountry = targetCountry;
return this;
}
/**
* Tax information.
* @return value or {@code null} for none
*/
public ProductTax getTax() {
return tax;
}
/**
* Tax information.
* @param tax tax or {@code null} for none
*/
public Product setTax(ProductTax tax) {
this.tax = tax;
return this;
}
/**
* Title of the item.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Title of the item.
* @param title title or {@code null} for none
*/
public Product setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* The preference of the denominator of the unit price.
* @return value or {@code null} for none
*/
public java.lang.String getUnitPricingBaseMeasure() {
return unitPricingBaseMeasure;
}
/**
* The preference of the denominator of the unit price.
* @param unitPricingBaseMeasure unitPricingBaseMeasure or {@code null} for none
*/
public Product setUnitPricingBaseMeasure(java.lang.String unitPricingBaseMeasure) {
this.unitPricingBaseMeasure = unitPricingBaseMeasure;
return this;
}
/**
* The measure and dimension of an item.
* @return value or {@code null} for none
*/
public java.lang.String getUnitPricingMeasure() {
return unitPricingMeasure;
}
/**
* The measure and dimension of an item.
* @param unitPricingMeasure unitPricingMeasure or {@code null} for none
*/
public Product setUnitPricingMeasure(java.lang.String unitPricingMeasure) {
this.unitPricingMeasure = unitPricingMeasure;
return this;
}
/**
* The read-only list of intended destinations which passed validation.
* @return value or {@code null} for none
*/
public java.util.List getValidatedDestinations() {
return validatedDestinations;
}
/**
* The read-only list of intended destinations which passed validation.
* @param validatedDestinations validatedDestinations or {@code null} for none
*/
public Product setValidatedDestinations(java.util.List validatedDestinations) {
this.validatedDestinations = validatedDestinations;
return this;
}
/**
* Read-only warnings.
* @return value or {@code null} for none
*/
public java.util.List getWarnings() {
return warnings;
}
/**
* Read-only warnings.
* @param warnings warnings or {@code null} for none
*/
public Product setWarnings(java.util.List warnings) {
this.warnings = warnings;
return this;
}
@Override
public Product set(String fieldName, Object value) {
return (Product) super.set(fieldName, value);
}
@Override
public Product clone() {
return (Product) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy