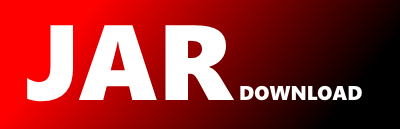
com.google.api.services.content.model.Datafeed Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-03-26 20:30:19 UTC)
* on 2015-06-05 at 00:40:03 UTC
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Datafeed data.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Datafeed extends com.google.api.client.json.GenericJson {
/**
* The two-letter ISO 639-1 language in which the attributes are defined in the data feed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String attributeLanguage;
/**
* The two-letter ISO 639-1 language of the items in the feed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contentLanguage;
/**
* The type of data feed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contentType;
/**
* Fetch schedule for the feed file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DatafeedFetchSchedule fetchSchedule;
/**
* The filename of the feed. All feeds must have a unique file name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fileName;
/**
* Format of the feed file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DatafeedFormat format;
/**
* The ID of the data feed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long id;
/**
* The list of intended destinations (corresponds to checked check boxes in Merchant Center).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List intendedDestinations;
/**
* Identifies what kind of resource this is. Value: the fixed string "content#datafeed".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* A descriptive name of the data feed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The two-letter ISO 3166 country where the items in the feed will be included in the search
* index.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String targetCountry;
/**
* The two-letter ISO 639-1 language in which the attributes are defined in the data feed.
* @return value or {@code null} for none
*/
public java.lang.String getAttributeLanguage() {
return attributeLanguage;
}
/**
* The two-letter ISO 639-1 language in which the attributes are defined in the data feed.
* @param attributeLanguage attributeLanguage or {@code null} for none
*/
public Datafeed setAttributeLanguage(java.lang.String attributeLanguage) {
this.attributeLanguage = attributeLanguage;
return this;
}
/**
* The two-letter ISO 639-1 language of the items in the feed.
* @return value or {@code null} for none
*/
public java.lang.String getContentLanguage() {
return contentLanguage;
}
/**
* The two-letter ISO 639-1 language of the items in the feed.
* @param contentLanguage contentLanguage or {@code null} for none
*/
public Datafeed setContentLanguage(java.lang.String contentLanguage) {
this.contentLanguage = contentLanguage;
return this;
}
/**
* The type of data feed.
* @return value or {@code null} for none
*/
public java.lang.String getContentType() {
return contentType;
}
/**
* The type of data feed.
* @param contentType contentType or {@code null} for none
*/
public Datafeed setContentType(java.lang.String contentType) {
this.contentType = contentType;
return this;
}
/**
* Fetch schedule for the feed file.
* @return value or {@code null} for none
*/
public DatafeedFetchSchedule getFetchSchedule() {
return fetchSchedule;
}
/**
* Fetch schedule for the feed file.
* @param fetchSchedule fetchSchedule or {@code null} for none
*/
public Datafeed setFetchSchedule(DatafeedFetchSchedule fetchSchedule) {
this.fetchSchedule = fetchSchedule;
return this;
}
/**
* The filename of the feed. All feeds must have a unique file name.
* @return value or {@code null} for none
*/
public java.lang.String getFileName() {
return fileName;
}
/**
* The filename of the feed. All feeds must have a unique file name.
* @param fileName fileName or {@code null} for none
*/
public Datafeed setFileName(java.lang.String fileName) {
this.fileName = fileName;
return this;
}
/**
* Format of the feed file.
* @return value or {@code null} for none
*/
public DatafeedFormat getFormat() {
return format;
}
/**
* Format of the feed file.
* @param format format or {@code null} for none
*/
public Datafeed setFormat(DatafeedFormat format) {
this.format = format;
return this;
}
/**
* The ID of the data feed.
* @return value or {@code null} for none
*/
public java.lang.Long getId() {
return id;
}
/**
* The ID of the data feed.
* @param id id or {@code null} for none
*/
public Datafeed setId(java.lang.Long id) {
this.id = id;
return this;
}
/**
* The list of intended destinations (corresponds to checked check boxes in Merchant Center).
* @return value or {@code null} for none
*/
public java.util.List getIntendedDestinations() {
return intendedDestinations;
}
/**
* The list of intended destinations (corresponds to checked check boxes in Merchant Center).
* @param intendedDestinations intendedDestinations or {@code null} for none
*/
public Datafeed setIntendedDestinations(java.util.List intendedDestinations) {
this.intendedDestinations = intendedDestinations;
return this;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "content#datafeed".
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "content#datafeed".
* @param kind kind or {@code null} for none
*/
public Datafeed setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* A descriptive name of the data feed.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* A descriptive name of the data feed.
* @param name name or {@code null} for none
*/
public Datafeed setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The two-letter ISO 3166 country where the items in the feed will be included in the search
* index.
* @return value or {@code null} for none
*/
public java.lang.String getTargetCountry() {
return targetCountry;
}
/**
* The two-letter ISO 3166 country where the items in the feed will be included in the search
* index.
* @param targetCountry targetCountry or {@code null} for none
*/
public Datafeed setTargetCountry(java.lang.String targetCountry) {
this.targetCountry = targetCountry;
return this;
}
@Override
public Datafeed set(String fieldName, Object value) {
return (Datafeed) super.set(fieldName, value);
}
@Override
public Datafeed clone() {
return (Datafeed) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy