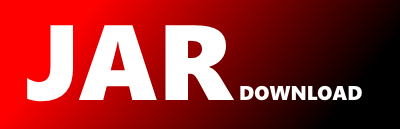
com.google.api.services.content.model.RateGroup Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Model definition for RateGroup.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RateGroup extends com.google.api.client.json.GenericJson {
/**
* A list of shipping labels defining the products to which this rate group applies to. This is a
* disjunction: only one of the labels has to match for the rate group to apply. May only be empty
* for the last rate group of a service. Required.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List applicableShippingLabels;
/**
* A list of carrier rates that can be referred to by mainTable or singleValue.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List carrierRates;
static {
// hack to force ProGuard to consider CarrierRate used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(CarrierRate.class);
}
/**
* A table defining the rate group, when singleValue is not expressive enough. Can only be set if
* singleValue is not set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Table mainTable;
/**
* Name of the rate group. Optional. If set has to be unique within shipping service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The value of the rate group (e.g. flat rate $10). Can only be set if mainTable and subtables
* are not set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Value singleValue;
/**
* A list of subtables referred to by mainTable. Can only be set if mainTable is set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List subtables;
/**
* A list of shipping labels defining the products to which this rate group applies to. This is a
* disjunction: only one of the labels has to match for the rate group to apply. May only be empty
* for the last rate group of a service. Required.
* @return value or {@code null} for none
*/
public java.util.List getApplicableShippingLabels() {
return applicableShippingLabels;
}
/**
* A list of shipping labels defining the products to which this rate group applies to. This is a
* disjunction: only one of the labels has to match for the rate group to apply. May only be empty
* for the last rate group of a service. Required.
* @param applicableShippingLabels applicableShippingLabels or {@code null} for none
*/
public RateGroup setApplicableShippingLabels(java.util.List applicableShippingLabels) {
this.applicableShippingLabels = applicableShippingLabels;
return this;
}
/**
* A list of carrier rates that can be referred to by mainTable or singleValue.
* @return value or {@code null} for none
*/
public java.util.List getCarrierRates() {
return carrierRates;
}
/**
* A list of carrier rates that can be referred to by mainTable or singleValue.
* @param carrierRates carrierRates or {@code null} for none
*/
public RateGroup setCarrierRates(java.util.List carrierRates) {
this.carrierRates = carrierRates;
return this;
}
/**
* A table defining the rate group, when singleValue is not expressive enough. Can only be set if
* singleValue is not set.
* @return value or {@code null} for none
*/
public Table getMainTable() {
return mainTable;
}
/**
* A table defining the rate group, when singleValue is not expressive enough. Can only be set if
* singleValue is not set.
* @param mainTable mainTable or {@code null} for none
*/
public RateGroup setMainTable(Table mainTable) {
this.mainTable = mainTable;
return this;
}
/**
* Name of the rate group. Optional. If set has to be unique within shipping service.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the rate group. Optional. If set has to be unique within shipping service.
* @param name name or {@code null} for none
*/
public RateGroup setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The value of the rate group (e.g. flat rate $10). Can only be set if mainTable and subtables
* are not set.
* @return value or {@code null} for none
*/
public Value getSingleValue() {
return singleValue;
}
/**
* The value of the rate group (e.g. flat rate $10). Can only be set if mainTable and subtables
* are not set.
* @param singleValue singleValue or {@code null} for none
*/
public RateGroup setSingleValue(Value singleValue) {
this.singleValue = singleValue;
return this;
}
/**
* A list of subtables referred to by mainTable. Can only be set if mainTable is set.
* @return value or {@code null} for none
*/
public java.util.List getSubtables() {
return subtables;
}
/**
* A list of subtables referred to by mainTable. Can only be set if mainTable is set.
* @param subtables subtables or {@code null} for none
*/
public RateGroup setSubtables(java.util.List subtables) {
this.subtables = subtables;
return this;
}
@Override
public RateGroup set(String fieldName, Object value) {
return (RateGroup) super.set(fieldName, value);
}
@Override
public RateGroup clone() {
return (RateGroup) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy